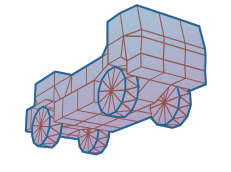 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
76 #include <OgreMaterialManager.h>
77 #include <OgreSceneManager.h>
78 #include <OgreMovableObject.h>
79 #include <OgreParticleSystem.h>
80 #include <OgreEntity.h>
82 #include <fmt/format.h>
93 if (sectionconfig !=
"")
95 auto result = def->user_modules.find(sectionconfig);
97 if (result != def->user_modules.end())
100 LOG(
" == ActorSpawner: Module added to configuration: " + sectionconfig);
128 LOG(
" == ActorSpawner: Addon part added to configuration: " + addonpart);
158 for (
auto& def: module_def->
nodes)
264 for (
size_t i = 0; i < req.
num_nodes; ++i)
329 #ifdef USE_ANGELSCRIPT
331 #endif // USE_ANGELSCRIPT
401 "Failed to load built-in material 'tracks/simple'; disabling 'SimpleMaterials'");
471 float proped_wheels_radius_sum = 0.0f;
511 if ((a1 == i - 1) && (a2 == i - 0))
544 float max_dist = 0.0f;
558 float offset = atan2(dir.dotProduct(Ogre::Vector3::UNIT_Z), dir.dotProduct(Ogre::Vector3::UNIT_X));
578 Ogre::Vector3 cross = dir_node_offset.crossProduct(roll_node_offset);
632 if (wcent.x>prop.x && wcent.x<prop.x+15.0)
635 if (wcent.y>prop.y-radius && wcent.y<prop.y+radius)
640 float pleft=prop.z+radius;
641 float pright=prop.z-radius;
643 if (pleft<aleft) aleft=pleft;
645 if (pright>aright) aright=pright;
649 float wratio=(aleft-aright)/(wleft-wright);
673 Ogre::Entity* afterburn_ent =
nullptr;
683 propname, nozzle_ent, afterburn_ent);
782 Ogre::String
const & airfoil,
791 this->
ComposeName(
"turboprop", aeroengine_index).c_str(),
880 this->
ComposeName(
"airbrake", airbrake_idx).c_str(),
911 ab->
offset = Ogre::Vector3::ZERO;
944 this->GetNodeIndexOrThrow(def.
nodes[2]),
945 this->GetNodeIndexOrThrow(def.
nodes[3]),
946 this->GetNodeIndexOrThrow(def.
nodes[4]),
947 this->GetNodeIndexOrThrow(def.
nodes[5]),
948 this->GetNodeIndexOrThrow(def.
nodes[6]),
949 this->GetNodeIndexOrThrow(def.
nodes[7]),
964 Ogre::Entity* entity =
nullptr;
992 if (node1 != previous_wing.
fa->
nfld)
1027 left_green_prop.
pp_beacon_bbs[0]->setMaterialName(
"tracks/greenflare");
1031 left_green_prop.
pp_beacon_bbs[0]->setDefaultDimensions(0.5, 0.5);
1049 left_flash_prop.
pp_beacon_light[0]->setDiffuseColour( Ogre::ColourValue(1.0, 1.0, 1.0));
1050 left_flash_prop.
pp_beacon_light[0]->setSpecularColour( Ogre::ColourValue(1.0, 1.0, 1.0));
1051 left_flash_prop.
pp_beacon_light[0]->setAttenuation(50.0, 1.0, 0.3, 0.0);
1061 left_flash_prop.
pp_beacon_bbs[0]->setMaterialName(
"tracks/flare");
1065 left_flash_prop.
pp_beacon_bbs[0]->setDefaultDimensions(1.0, 1.0);
1090 right_red_prop.
pp_beacon_bbs[0]->setMaterialName(
"tracks/redflare");
1094 right_red_prop.
pp_beacon_bbs[0]->setDefaultDimensions(0.5, 0.5);
1112 right_flash_prop.
pp_beacon_light[0]->setDiffuseColour( Ogre::ColourValue(1.0, 1.0, 1.0));
1113 right_flash_prop.
pp_beacon_light[0]->setSpecularColour( Ogre::ColourValue(1.0, 1.0, 1.0));
1114 right_flash_prop.
pp_beacon_light[0]->setAttenuation(50.0, 1.0, 0.3, 0.0);
1124 right_flash_prop.
pp_beacon_bbs[0]->setMaterialName(
"tracks/flare");
1128 right_flash_prop.
pp_beacon_bbs[0]->setDefaultDimensions(1.0, 1.0);
1159 return (((
x-ref).crossProduct(
y-ref)).length()+((
x-aref).crossProduct(
y-aref)).length())*0.5f;
1176 #endif // USE_OPENAL
1183 #endif // USE_OPENAL
1193 if (sound_script ==
nullptr)
1213 #endif // USE_OPENAL
1218 auto itor = def.
nodes.begin();
1219 auto end = def.
nodes.end();
1220 for(; itor != end; ++itor)
1242 if (def.
key ==
"helpMaterial")
1246 else if (def.
key ==
"speedoMax")
1249 if (maxKph > 10 && maxKph < 32000)
1260 else if (def.
key ==
"useMaxRPM")
1264 else if (def.
key ==
"shifterAnimTime")
1291 if (template_name ==
"" || template_name ==
"default")
1293 template_name =
"tracks/Smoke";
1301 if (exhaust.
smoker ==
nullptr)
1303 std::stringstream msg;
1304 msg <<
"Failed to create particle system '" << name <<
"' (template: '" << template_name <<
"')";
1324 for (; module_itor != module_end; ++module_itor)
1326 if (! module_itor->get()->submesh_groundmodel.empty())
1328 return module_itor->get()->submesh_groundmodel[0];
1331 return std::string();
1343 std::vector<RigDef::Texcoord>::iterator texcoord_itor = def.
texcoords.begin();
1344 for ( ; texcoord_itor != def.
texcoords.end(); texcoord_itor++)
1362 for ( ; cab_itor != cab_itor_end; ++cab_itor)
1370 std::stringstream msg;
1371 msg <<
"Collcab limit (" <<
MAX_CABS <<
") exceeded";
1376 bool mk_buoyance =
false;
1419 std::stringstream msg;
1420 msg <<
"Collcab limit (" <<
MAX_CABS <<
") exceeded";
1426 std::stringstream msg;
1427 msg <<
"Buoycab limit (" <<
MAX_CABS <<
") exceeded";
1529 std::vector<unsigned int> node_indices;
1530 bool nodes_found =
true;
1536 nodes_found =
false;
1539 node_indices.push_back(node);
1571 catch (Ogre::Exception& e)
1594 pprop.
pp_id = prop_id;
1603 prop.
pp_id = prop_id;
1610 prop.
pp_rot = Ogre::Quaternion(Ogre::Degree(prop.
pp_rota.z), Ogre::Vector3::UNIT_Z)
1611 * Ogre::Quaternion(Ogre::Degree(prop.
pp_rota.y), Ogre::Vector3::UNIT_Y)
1612 * Ogre::Quaternion(Ogre::Degree(prop.
pp_rota.x), Ogre::Vector3::UNIT_X);
1632 Ogre::Vector3 steering_wheel_offset = Ogre::Vector3::ZERO;
1635 steering_wheel_offset = Ogre::Vector3(-0.67, -0.61,0.24);
1639 steering_wheel_offset = Ogre::Vector3(0.67, -0.61,0.24);
1641 if (steering_wheel_offset != Ogre::Vector3::ZERO)
1654 this->ComposeName(
"steering wheel entity @ prop", prop_id),
1666 this->ComposeName(
"prop entity", prop_id),
1715 pp_beacon_light->setType(Ogre::Light::LT_SPOTLIGHT);
1718 pp_beacon_light->setAttenuation(50.0, 1.0, 0.3, 0.0);
1719 pp_beacon_light->setSpotlightRange( Ogre::Degree(35), Ogre::Degree(45) );
1720 pp_beacon_light->setCastShadows(
false);
1721 pp_beacon_light->setVisible(
false);
1726 if (flare_billboard_sys)
1728 flare_billboard_sys->createBillboard(0,0,0);
1729 flare_billboard_sys->setMaterialName(prop.
pp_media[1]);
1731 flare_scene_node->attachObject(flare_billboard_sys);
1733 flare_scene_node->setVisible(
false);
1747 pp_beacon_light->setType(Ogre::Light::LT_POINT);
1748 pp_beacon_light->setDiffuseColour( Ogre::ColourValue(1.0, 0.0, 0.0));
1749 pp_beacon_light->setSpecularColour( Ogre::ColourValue(1.0, 0.0, 0.0));
1750 pp_beacon_light->setAttenuation(50.0, 1.0, 0.3, 0.0);
1751 pp_beacon_light->setCastShadows(
false);
1752 pp_beacon_light->setVisible(
false);
1756 if (flare_billboard_sys)
1758 flare_billboard_sys->createBillboard(0,0,0);
1759 flare_billboard_sys->setMaterialName(
"tracks/redbeaconflare");
1761 flare_billboard_sys->setDefaultDimensions(1.0, 1.0);
1762 flare_scene_node->attachObject(flare_billboard_sys);
1764 flare_scene_node->setVisible(
false);
1776 for (
int k=0; k<4; k++)
1786 prop.
pp_beacon_light[k]->setDiffuseColour( Ogre::ColourValue(1.0, 0.0, 0.0));
1787 prop.
pp_beacon_light[k]->setSpecularColour( Ogre::ColourValue(1.0, 0.0, 0.0));
1791 prop.
pp_beacon_light[k]->setDiffuseColour( Ogre::ColourValue(0.0, 0.5, 1.0));
1792 prop.
pp_beacon_light[k]->setSpecularColour( Ogre::ColourValue(0.0, 0.5, 1.0));
1795 prop.
pp_beacon_light[k]->setSpotlightRange( Ogre::Degree(35), Ogre::Degree(45) );
1806 prop.
pp_beacon_bbs[k]->setMaterialName(
"tracks/brightredflare");
1810 prop.
pp_beacon_bbs[k]->setMaterialName(
"tracks/brightblueflare");
1839 if (anim_def.
ratio == 0)
1841 std::stringstream msg;
1842 msg <<
"Prop (mesh: " << def.
mesh_name <<
") has invalid animation ratio (0), using it anyway (compatibility)...";
1970 std::list<RigDef::Animation::MotorSource>::iterator source_itor = anim_def.
motor_sources.begin();
1971 for ( ; source_itor != anim_def.
motor_sources.end(); source_itor++)
1976 anim.
animOpt3 =
static_cast<float>(source_itor->motor);
1980 anim.
animOpt3 =
static_cast<float>(source_itor->motor);
1984 anim.
animOpt3 =
static_cast<float>(source_itor->motor);
1988 anim.
animOpt3 =
static_cast<float>(source_itor->motor);
1992 anim.
animOpt3 =
static_cast<float>(source_itor->motor);
1998 anim.
animOpt3 =
static_cast<float>(source_itor->motor);
2033 anim.
animOpt3 =
static_cast<float>(link_id);
2071 const bool use_default_lower_limit = (anim_def.
lower_limit == 0.f);
2072 const bool use_default_upper_limit = (anim_def.
upper_limit == 0.f);
2134 float size = def.
size;
2139 if (blink_delay == -2)
2163 flare.
blinkdelay = (blink_delay == -1) ? 0.5f : blink_delay / 1000.f;
2185 fmt::format(
"Bad flare control num {}, must be 1-{}, using 1.",
2192 fmt::format(
"Bad flare control num {}, must be 1-{}, using {}.",
2214 std::string flare_name = this->
ComposeName(
"Flare", flare_id);
2215 if (!is_placeholder)
2229 if (flare.
bbs ==
nullptr)
2235 flare.
bbs->createBillboard(0,0,0);
2238 if (using_default_material)
2242 material_name =
"tracks/brakeflare";
2246 material_name =
"tracks/blinkflare";
2250 material_name =
"tracks/greenflare";
2254 material_name =
"tracks/redflare";
2258 material_name =
"tracks/flare";
2263 if (!material.isNull())
2265 flare.
bbs->setMaterial(material);
2266 flare.
snode->attachObject(flare.
bbs);
2270 flare.
light =
nullptr;
2277 flare.
light->setType(Ogre::Light::LT_SPOTLIGHT);
2278 flare.
light->setDiffuseColour( Ogre::ColourValue(1, 1, 1));
2279 flare.
light->setSpecularColour( Ogre::ColourValue(1, 1, 1));
2280 flare.
light->setAttenuation(200, 0.9, 0, 0);
2281 flare.
light->setSpotlightRange( Ogre::Degree(35), Ogre::Degree(45) );
2282 flare.
light->setCastShadows(
false);
2287 flare.
light->setType(Ogre::Light::LT_SPOTLIGHT);
2288 flare.
light->setDiffuseColour(Ogre::ColourValue(1, 1, 1));
2289 flare.
light->setSpecularColour(Ogre::ColourValue(1, 1, 1));
2290 flare.
light->setAttenuation(400, 0.9, 0, 0);
2291 flare.
light->setSpotlightRange(Ogre::Degree(35), Ogre::Degree(45));
2292 flare.
light->setCastShadows(
false);
2297 flare.
light->setType(Ogre::Light::LT_SPOTLIGHT);
2298 flare.
light->setDiffuseColour(Ogre::ColourValue(1, 1, 1));
2299 flare.
light->setSpecularColour(Ogre::ColourValue(1, 1, 1));
2300 flare.
light->setAttenuation(400, 0.9, 0, 0);
2301 flare.
light->setSpotlightRange(Ogre::Degree(35), Ogre::Degree(45));
2302 flare.
light->setCastShadows(
false);
2310 flare.
light->setDiffuseColour( Ogre::ColourValue(1.0, 0, 0));
2311 flare.
light->setSpecularColour( Ogre::ColourValue(1.0, 0, 0));
2312 flare.
light->setAttenuation(10.0, 1.0, 0, 0);
2317 flare.
light->setDiffuseColour(Ogre::ColourValue(1, 1, 1));
2318 flare.
light->setSpecularColour(Ogre::ColourValue(1, 1, 1));
2319 flare.
light->setAttenuation(20.0, 1, 0, 0);
2324 flare.
light->setDiffuseColour( Ogre::ColourValue(1.0, 0, 0));
2325 flare.
light->setSpecularColour( Ogre::ColourValue(1.0, 0, 0));
2326 flare.
light->setAttenuation(10.0, 1.0, 0, 0);
2331 flare.
light->setDiffuseColour( Ogre::ColourValue(1, 1, 0));
2332 flare.
light->setSpecularColour( Ogre::ColourValue(1, 1, 0));
2333 flare.
light->setAttenuation(10.0, 1, 1, 0);
2338 flare.
light->setDiffuseColour( Ogre::ColourValue(1, 1, 1));
2339 flare.
light->setSpecularColour( Ogre::ColourValue(1, 1, 1));
2340 flare.
light->setAttenuation(1.0, 1.0, 1, 0.2);
2345 flare.
light->setDiffuseColour(Ogre::ColourValue(1, 1, 1));
2346 flare.
light->setSpecularColour(Ogre::ColourValue(1, 1, 1));
2347 flare.
light->setAttenuation(5.0, 1.0, 1, 0.2);
2352 if (flare.
light !=
nullptr)
2354 flare.
light->setType(Ogre::Light::LT_SPOTLIGHT);
2355 flare.
light->setSpotlightRange( Ogre::Degree(35), Ogre::Degree(45) );
2356 flare.
light->setCastShadows(
false);
2363 Ogre::MaterialPtr src_mat = Ogre::MaterialManager::getSingleton().getByName(source_name, rg_name);
2364 if (src_mat.isNull())
2366 std::stringstream msg;
2367 msg <<
"Built-in material '" << source_name <<
"' missing! Skipping...";
2369 return Ogre::MaterialPtr();
2372 return src_mat->clone(clone_name);
2391 if (!Ogre::ResourceGroupManager::getSingleton().resourceExists(resource_group, def.
diffuse_map))
2398 !Ogre::ResourceGroupManager::getSingleton().resourceExists(resource_group, def.
damaged_diffuse_map))
2405 !Ogre::ResourceGroupManager::getSingleton().resourceExists(resource_group, def.
specular_map))
2418 if (Ogre::MaterialManager::getSingleton().getByName(def.
name, module_rg).isNull())
2420 LOG(
fmt::format(
"[RoR] DBG ActorSpawner::ProcessManagedMaterial(): Creating placeholder for material '{}' in group '{}'", def.
name, module_rg));
2425 LOG(
fmt::format(
"[RoR] DBG ActorSpawner::ProcessManagedMaterial(): Placeholder already exists: '{}' in group '{}'", def.
name, module_rg));
2430 Ogre::MaterialPtr material;
2434 material = Ogre::MaterialManager::getSingleton().getByName(
"tracks/transred")->clone(custom_name,
true, resource_group);
2438 std::string mat_name_base
2440 ?
"managed/flexmesh_standard"
2441 :
"managed/flexmesh_transparent";
2457 if (material.isNull())
2480 if (material.isNull())
2502 if (material.isNull())
2523 if (material.isNull())
2533 Ogre::String mat_name_base
2535 ?
"managed/mesh_standard"
2536 :
"managed/mesh_transparent";
2550 if (material.isNull())
2571 if (material.isNull())
2585 material->getTechnique(
"BaseTechnique")->getPass(
"BaseRender")->setCullingMode(Ogre::CULL_NONE);
2590 material->getTechnique(
"BaseTechnique")->getPass(
"SpecularMapping1")->setCullingMode(Ogre::CULL_NONE);
2594 material->getTechnique(
"BaseTechnique")->getPass(
"Specular")->setCullingMode(Ogre::CULL_NONE);
2600 material->compile();
2617 RoR::LogFormat(
"[RoR|Spawner] Collision box: re-assigning node '%s' from box ID '%d' to '%d'",
2646 _out_axle_wheel = i;
2669 std::stringstream msg;
2670 msg <<
"Couldn't find wheel with axis nodes '" << def.
wheels[0][0].
ToString()
2677 std::stringstream msg;
2678 msg <<
"Couldn't find wheel with axis nodes '" << def.
wheels[1][0].
ToString()
2692 for (
auto itor = def.
options.begin(); itor != end; ++itor)
2732 AddMessage(
Message::TYPE_ERROR,
"You cannot have both an inter-axle differential and a transfercase between the same two axles, skipping...");
2819 std::stringstream msg;
2821 <<
") must be positive nonzero number. Using it anyway (compatibility)";
2849 std::vector<RigDef::TorqueCurve::Sample>::iterator itor = def.
samples.begin();
2850 for ( ; itor != def.
samples.end(); itor++)
2852 target_torque_curve->
AddCurveSample(itor->power, itor->torque_percent);
2872 if (particle.
psys ==
nullptr)
2874 std::stringstream msg;
2875 msg <<
"Failed to create particle system '" << name <<
"' (template: '" << def.
particle_system_name <<
"')";
2881 particle.
snode->attachObject(particle.
psys);
2886 for (
unsigned int i = 0; i < particle.
psys->getNumEmitters(); i++)
2888 particle.
psys->getEmitter(i)->setEnabled(
false);
3026 if (rail_group ==
nullptr)
3028 std::stringstream msg;
3029 msg <<
"Specified rail group id '" << def.
railgroup_id <<
"' not found. Ignoring slidenode...";
3037 if (rail_group !=
nullptr)
3056 std::stringstream msg;
3057 msg <<
"Failed to find node by reference: " << node_ref.
ToString();
3064 std::vector<RigDef::Node::Range> & node_ranges,
3065 std::vector<NodeNum_t> & out_node_indices
3068 std::vector<RigDef::Node::Range>::iterator itor = node_ranges.begin();
3069 for ( ; itor != node_ranges.end(); itor++)
3071 if (itor->IsRange())
3085 std::stringstream msg;
3086 msg <<
"Encountered non-existent node '" << itor->end.ToString() <<
"' in range [" << itor->start.ToString() <<
" - " << itor->end.ToString() <<
"], "
3089 if (itor->end.Str().empty())
3091 msg <<
" However, this node must be accepted anyway for backwards compatibility."
3092 <<
" Please fix this as soon as possible.";
3093 end = itor->end.Num();
3110 for (
NodeNum_t i = start; i <= end; i++)
3112 out_node_indices.push_back(i);
3126 std::vector<NodeNum_t> node_indices;
3131 for (
unsigned int i = 0; i < node_indices.size() - 1; i++)
3134 if (beam ==
nullptr)
3136 std::stringstream msg;
3137 msg <<
"No beam between nodes indexed '" << node_indices[i] <<
"' and '" << node_indices[i + 1] <<
"'";
3147 for (
size_t i = 1; i < (num_seg - 1); ++i)
3154 const bool is_loop = (node_indices.front() == node_indices.back());
3191 if (node ==
nullptr)
3201 if (itor->hk_hook_node == node)
3208 if (hook ==
nullptr)
3210 std::stringstream msg;
3211 msg <<
"Node '" << def.
node.
ToString() <<
"' is not a hook-node (not marked with flag 'h'), ignoring...";
3242 int beam_index = -1;
3259 auto itor = lockgroup.
nodes.begin();
3260 auto end = lockgroup.
nodes.end();
3261 for (; itor != end; ++itor)
3274 bool invisible =
false;
3276 bool shock_trigger_enabled =
true;
3277 bool triggerblocker =
false;
3278 bool triggerblocker_inverted =
false;
3279 bool cmdkeyblock =
false;
3280 bool hooktoggle =
false;
3281 bool enginetrigger =
false;
3283 bool trigger_cmdkeyblock_state_short =
false;
3284 bool trigger_cmdkeyblock_state_long =
true;
3294 shock_trigger_enabled =
false;
3299 triggerblocker =
true;
3311 sbound = abs(sbound-1);
3317 triggerblocker_inverted =
true;
3336 enginetrigger =
true;
3339 if (!triggerblocker && !triggerblocker_inverted && !hooktoggle && !enginetrigger)
3348 else if (!hooktoggle && !enginetrigger)
3357 else if (enginetrigger)
3393 LOG(
"Trigger added. BeamID " +
TOSTRING(beam_index));
3397 beam.
shock = &shock;
3398 shock.
beamid = beam_index;
3400 if (!triggerblocker && !triggerblocker_inverted)
3410 if (!triggerblocker_inverted)
3426 if (cmdkeyblock && !triggerblocker)
3428 trigger_cmdkeyblock_state_short =
true;
3436 shock.
flags = shockflag;
3465 for (
unsigned int i = 0; i < 4; i++)
3501 for (
unsigned int i = 0; i < 4; i++)
3537 std::string start_function;
3538 std::string stop_function;
3565 Ogre::String start_function;
3566 Ogre::String stop_function;
3634 float center_length = 0.f;
3660 contract_command->
beams.push_back(cmd_beam);
3670 extend_command->
beams.push_back(cmd_beam);
3689 bool must_insert_qpair =
true;
3696 must_insert_qpair =
false;
3704 if (must_insert_qpair)
3717 float anim_option = 0;
3875 std::shared_ptr<RigDef::BeamDefaults> & beam_defaults,
3885 float strength = beam_defaults->breaking_threshold;
3891 float plastic_coef = beam_defaults->plastic_deform_coef;
3904 bool invisible =
false;
3905 unsigned int hydro_flags = 0;
3995 short_bound /= beam_length;
3996 long_bound /= beam_length;
4001 short_bound = (beam_length - short_bound) / beam_length;
4002 long_bound = (long_bound - beam_length) / beam_length;
4004 if (long_bound < 0.f)
4008 "Metric shock length calculation failed, 'short_bound' less than beams spawn length. Resetting to beam's spawn length (short_bound = 0)"
4013 if (short_bound > 1.f)
4017 "Metric shock length calculation failed, 'short_bound' less than 0 meters. Resetting to 0 meters (short_bound = 1)"
4044 shock.
flags = shock_flags;
4058 beam.
shock = & shock;
4059 shock.
beamid = beam_index;
4078 short_bound /= beam_length;
4079 long_bound /= beam_length;
4084 short_bound = (beam_length - short_bound) / beam_length;
4085 long_bound = (long_bound - beam_length) / beam_length;
4087 if (long_bound < 0.f)
4091 "Metric shock length calculation failed, 'short_bound' less than beams spawn length. Resetting to beam's spawn length (short_bound = 0)"
4096 if (short_bound > 1.f)
4100 "Metric shock length calculation failed, 'short_bound' less than 0 meters. Resetting to 0 meters (short_bound = 1)"
4127 shock.
flags = shock_flags;
4139 beam.
shock = & shock;
4140 shock.
beamid = beam_index;
4166 short_bound /= beam_length;
4167 long_bound /= beam_length;
4186 shock.
flags = shock_flags;
4195 beam.
shock = & shock;
4196 shock.
beamid = beam_index;
4210 node_t* swap = axis_node_1;
4211 axis_node_1 = axis_node_2;
4216 node_t *rigidity_node =
nullptr;
4217 node_t *axis_node_closest_to_rigidity_node =
nullptr;
4223 axis_node_closest_to_rigidity_node = ((distance_1 < distance_2)) ? axis_node_1 : axis_node_2;
4232 wheel.
wh_width = axis_vector.length();
4233 axis_vector.normalise();
4234 Ogre::Vector3 rim_ray_vector = axis_vector.perpendicular() * override_rim_radius;
4235 Ogre::Quaternion rim_ray_rotator = Ogre::Quaternion(Ogre::Degree(-360.f / (def.
num_rays * 2)), axis_vector);
4238 for (
unsigned int i = 0; i < def.
num_rays; i++)
4243 Ogre::Vector3 ray_point = axis_node_1->
RelPosition + rim_ray_vector;
4244 rim_ray_vector = rim_ray_rotator * rim_ray_vector;
4249 outer_node.
mass = node_mass;
4258 ray_point = axis_node_2->
RelPosition + rim_ray_vector;
4259 rim_ray_vector = rim_ray_rotator * rim_ray_vector;
4264 inner_node.
mass = node_mass;
4277 Ogre::Vector3 tyre_ray_vector = axis_vector.perpendicular() * override_tire_radius;
4278 Ogre::Quaternion& tyre_ray_rotator = rim_ray_rotator;
4279 tyre_ray_vector = tyre_ray_rotator * tyre_ray_vector;
4282 for (
unsigned int i = 0; i < def.
num_rays; i++)
4286 Ogre::Vector3 ray_point = axis_node_1->
RelPosition + tyre_ray_vector;
4287 tyre_ray_vector = tyre_ray_rotator * tyre_ray_vector;
4291 outer_node.
mass = node_mass;
4302 ray_point = axis_node_2->
RelPosition + tyre_ray_vector;
4303 tyre_ray_vector = tyre_ray_rotator * tyre_ray_vector;
4307 inner_node.
mass = node_mass;
4318 wheel.
wh_nodes[i * 2] = & outer_node;
4319 wheel.
wh_nodes[(i * 2) + 1] = & inner_node;
4330 for (
unsigned int i = 0; i < def.
num_rays; i++)
4335 unsigned int rim_outer_node_index = base_node_index + (i * 2);
4345 unsigned int rim_next_outer_node_index = base_node_index + (((i + 1) % def.
num_rays) * 2);
4357 for (
unsigned int i = 0; i < def.
num_rays; i++)
4359 int rim_node_index = base_node_index + i*2;
4360 int tyre_node_index = base_node_index + i*2 + def.
num_rays*2;
4365 int tyre_base_index = (i == 0) ? tyre_node_index + (def.
num_rays * 2) : tyre_node_index;
4374 int index = (i == 0) ? tyre_node_index + (def.
num_rays * 2) - 1 : tyre_node_index - 1;
4383 int rimnode = rim_node_index;
4391 if (rigidity_node !=
nullptr)
4393 if (axis_node_closest_to_rigidity_node == axis_node_1)
4395 axis_node_closest_to_rigidity_node = &
m_actor->
ar_nodes[base_node_index+i*2+rays*2];
4398 axis_node_closest_to_rigidity_node = &
m_actor->
ar_nodes[base_node_index+i*2+1+rays*2];
4400 unsigned int beam_index =
AddWheelBeam(rigidity_node, axis_node_closest_to_rigidity_node, tyre_spring, tyre_damp, def.
beam_defaults);
4409 float support_beams_short_bound = 1.0f - ((override_rim_radius / override_tire_radius) * 0.95f);
4411 for (
unsigned int i=0; i<def.
num_rays; i++)
4414 unsigned int tirenode = base_node_index + i*2 + def.
num_rays*2;
4415 unsigned int beam_index;
4456 override_rim_radius,
4489 out_node_1 = def_node_2;
4490 out_node_2 = def_node_1;
4494 out_node_1 = def_node_1;
4495 out_node_2 = def_node_2;
4503 node_t* axis_node_1 =
nullptr;
4504 node_t* axis_node_2 =
nullptr;
4508 this->BuildWheelObjectAndNodes(
4559 node_t* axis_node_1 =
nullptr;
4560 node_t* axis_node_2 =
nullptr;
4565 this->BuildWheelObjectAndNodes(
4582 float tyre_spring = def.
spring;
4583 float tyre_damp = def.
damping;
4625 unsigned int num_rays,
4627 Ogre::String mesh_name,
4628 Ogre::String mesh_rg,
4629 Ogre::String material_name,
4630 Ogre::String material_rg,
4661 catch (Ogre::Exception& e)
4670 unsigned int num_rays,
4673 node_t *reference_arm_node,
4674 unsigned int reserve_nodes,
4675 unsigned int reserve_beams,
4679 std::shared_ptr<RigDef::NodeDefaults> node_defaults,
4688 float axis_length = axis_vector.length();
4689 axis_vector.normalise();
4698 wheel.
wh_width = (wheel_width < 0) ? axis_length : wheel_width;
4714 Ogre::Vector3 ray_vector = axis_vector.perpendicular() * wheel_radius;
4715 Ogre::Quaternion ray_rotator = Ogre::Quaternion(Ogre::Degree(-360.0 / (num_rays * 2)), axis_vector);
4717 for (
unsigned int i = 0; i < num_rays; i++)
4720 Ogre::Vector3 ray_point = axis_node_1->
RelPosition + ray_vector;
4721 ray_vector = ray_rotator * ray_vector;
4724 InitNode(outer_node, ray_point, node_defaults);
4725 outer_node.
mass = wheel_mass / (2.f * num_rays);
4733 ray_point = axis_node_2->
RelPosition + ray_vector;
4734 ray_vector = ray_rotator * ray_vector;
4737 InitNode(inner_node, ray_point, node_defaults);
4738 inner_node.
mass = wheel_mass / (2.f * num_rays);
4746 wheel.
wh_nodes[i * 2] = & outer_node;
4747 wheel.
wh_nodes[(i * 2) + 1] = & inner_node;
4753 unsigned int options = (defaults->options | node_def.
options);
4763 unsigned int num_rays,
4771 std::shared_ptr<RigDef::BeamDefaults> beam_defaults,
4777 bool rigidity_beam_side_1 =
false;
4778 node_t *rigidity_node =
nullptr;
4784 rigidity_beam_side_1 = distance_1 < distance_2;
4787 for (
unsigned int i = 0; i < num_rays; i++)
4790 unsigned int outer_ring_node_index = base_node_index + (i * 2);
4794 AddWheelBeam(axis_node_1, outer_ring_node, tyre_spring, tyre_damping, beam_defaults, 0.66f, max_extension);
4795 AddWheelBeam(axis_node_2, inner_ring_node, tyre_spring, tyre_damping, beam_defaults, 0.66f, max_extension);
4796 AddWheelBeam(axis_node_2, outer_ring_node, tyre_spring, tyre_damping, beam_defaults);
4797 AddWheelBeam(axis_node_1, inner_ring_node, tyre_spring, tyre_damping, beam_defaults);
4800 unsigned int next_outer_ring_node_index = base_node_index + (((i + 1) % num_rays) * 2);
4804 AddWheelBeam(outer_ring_node, inner_ring_node, rim_spring, rim_damping, beam_defaults);
4805 AddWheelBeam(outer_ring_node, next_outer_ring_node, rim_spring, rim_damping, beam_defaults);
4806 AddWheelBeam(inner_ring_node, next_inner_ring_node, rim_spring, rim_damping, beam_defaults);
4807 AddWheelBeam(inner_ring_node, next_outer_ring_node, rim_spring, rim_damping, beam_defaults);
4810 if (rigidity_node !=
nullptr)
4812 node_t *target_node = (rigidity_beam_side_1) ? outer_ring_node : inner_ring_node;
4813 unsigned int beam_index =
AddWheelBeam(rigidity_node, target_node, tyre_spring, tyre_damping, beam_defaults, -1.f, -1.f,
BEAM_VIRTUAL);
4823 node_t* axis_node_1 =
nullptr;
4824 node_t* axis_node_2 =
nullptr;
4829 this->BuildWheelObjectAndNodes(
4886 node_t* axis_node_1 =
nullptr;
4887 node_t* axis_node_2 =
nullptr;
4893 bool rigidity_beam_side_1 =
false;
4899 rigidity_beam_side_1 = distance_1 < distance_2;
4909 axis_vector.normalise();
4910 Ogre::Vector3 rim_ray_vector = Ogre::Vector3(0, override_rim_radius, 0);
4911 Ogre::Quaternion rim_ray_rotator = Ogre::Quaternion(Ogre::Degree(-360.f / wheel_2_def.
num_rays), axis_vector);
4914 wheel.
wh_width = axis_vector.length();
4917 for (
unsigned int i = 0; i < wheel_2_def.
num_rays; i++)
4919 float node_mass = wheel_2_def.
mass / (4.f * wheel_2_def.
num_rays);
4922 Ogre::Vector3 ray_point = axis_node_1->
RelPosition + rim_ray_vector;
4926 outer_node.
mass = node_mass;
4934 ray_point = axis_node_2->
RelPosition + rim_ray_vector;
4938 inner_node.
mass = node_mass;
4949 rim_ray_vector = rim_ray_rotator * rim_ray_vector;
4952 Ogre::Vector3 tyre_ray_vector = Ogre::Vector3(0, override_tire_radius, 0);
4953 Ogre::Quaternion tyre_ray_rotator = Ogre::Quaternion(Ogre::Degree(-180.f / wheel_2_def.
num_rays), axis_vector);
4954 tyre_ray_vector = tyre_ray_rotator * tyre_ray_vector;
4957 for (
unsigned int i = 0; i < wheel_2_def.
num_rays; i++)
4960 Ogre::Vector3 ray_point = axis_node_1->
RelPosition + tyre_ray_vector;
4964 outer_node.
mass = (0.67f * wheel_2_def.
mass) / (2.f * wheel_2_def.
num_rays);
4974 ray_point = axis_node_2->
RelPosition + tyre_ray_vector;
4978 inner_node.
mass = (0.33f * wheel_2_def.
mass) / (2.f * wheel_2_def.
num_rays);
4988 wheel.
wh_nodes[i * 2] = & outer_node;
4989 wheel.
wh_nodes[(i * 2) + 1] = & inner_node;
4991 tyre_ray_vector = rim_ray_rotator * tyre_ray_vector;
4995 for (
unsigned int i = 0; i < wheel_2_def.
num_rays; i++)
5000 unsigned int rim_outer_node_index = base_node_index + (i * 2);
5004 unsigned int beam_index;
5005 beam_index =
AddWheelRimBeam(wheel_2_def, axis_node_1, rim_outer_node);
5007 beam_index =
AddWheelRimBeam(wheel_2_def, axis_node_2, rim_inner_node);
5013 unsigned int rim_next_outer_node_index = base_node_index + (((i + 1) % wheel_2_def.
num_rays) * 2);
5029 (rigidity_beam_side_1) ? rim_outer_node : rim_inner_node
5036 unsigned int tyre_node_index = rim_outer_node_index + (2 * wheel_2_def.
num_rays);
5039 unsigned int tyre_next_node_index = rim_next_outer_node_index + (2 * wheel_2_def.
num_rays);
5044 AddTyreBeam(wheel_2_def, tyre_outer_node, tyre_next_outer_node);
5045 AddTyreBeam(wheel_2_def, tyre_outer_node, tyre_next_inner_node);
5046 AddTyreBeam(wheel_2_def, tyre_inner_node, tyre_next_outer_node);
5047 AddTyreBeam(wheel_2_def, tyre_inner_node, tyre_next_inner_node);
5049 AddTyreBeam(wheel_2_def, tyre_outer_node, rim_outer_node);
5050 AddTyreBeam(wheel_2_def, tyre_outer_node, rim_next_outer_node);
5051 AddTyreBeam(wheel_2_def, tyre_inner_node, rim_inner_node);
5052 AddTyreBeam(wheel_2_def, tyre_inner_node, rim_next_inner_node);
5054 AddTyreBeam(wheel_2_def, tyre_outer_node, rim_inner_node);
5055 AddTyreBeam(wheel_2_def, tyre_outer_node, rim_next_inner_node);
5056 AddTyreBeam(wheel_2_def, tyre_inner_node, rim_outer_node);
5057 AddTyreBeam(wheel_2_def, tyre_inner_node, rim_next_outer_node);
5059 AddTyreBeam(wheel_2_def, axis_node_1, tyre_outer_node);
5060 AddTyreBeam(wheel_2_def, axis_node_2, tyre_inner_node);
5097 override_rim_radius / override_tire_radius
5107 unsigned int num_rays,
5108 Ogre::String
const& face_material_name,
5109 Ogre::String
const& face_material_rg,
5110 Ogre::String
const& band_material_name,
5111 Ogre::String
const& band_material_rg,
5124 const std::string wheel_mesh_name = this->
ComposeName(
"mesh @ wheel*", wheel_index);
5130 static_cast<NodeNum_t>(node_base_index),
5132 face_material_name, face_material_rg,
5133 band_material_name, band_material_rg,
5138 const std::string instance_name = this->
ComposeName(
"entity @ wheel*", wheel_index);
5146 catch (Ogre::Exception& e)
5160 std::string rim_mesh_name,
5161 std::string rim_mesh_rg,
5162 std::string tire_mesh_name,
5163 std::string tire_mesh_rg)
5181 int num_nodes = num_rays * 4;
5182 std::vector<unsigned int> node_indices;
5183 node_indices.reserve(num_nodes);
5184 for (
int i = 0; i < num_nodes; ++i)
5186 node_indices.push_back( node_base_index + i );
5196 Ogre::Vector3(0.5f, 0.5f, 0.f),
5197 Ogre::Vector3(0.f, 0.f, 0.f),
5203 if (flexbody ==
nullptr)
5210 catch (Ogre::Exception& e)
5213 "Failed to create flexbodywheel visuals '" + tire_mesh_name +
"', reason:" + e.getDescription());
5222 std::shared_ptr<RigDef::BeamDefaults> beam_defaults,
5223 float max_contraction,
5224 float max_extension,
5233 if (max_contraction > 0.f)
5304 if (force < 1.f || force > 20.f)
5306 std::stringstream msg;
5307 msg <<
"Clamping 'regulating_force' value '" << force <<
"' to allowed range <1 - 20>";
5309 force = (force < 1.f) ? 1.f : 20.f;
5321 if (pulse <= 1.0f || pulse >= 2000.0f)
5337 if (force < 1.f || force > 20.f)
5339 std::stringstream msg;
5340 msg <<
"Clamping 'regulating_force' value '" << force <<
"' to allowed range <1 - 20>";
5342 force = (force < 1.f) ? 1.f : 20.f;
5353 if (pulse <= 1.0f || pulse >= 2000.0f)
5384 m_actor->
ar_engine->
SetTurboOptions(def.
version, def.
tinertiaFactor, def.
nturbos, def.
param1, def.
param2, def.
param3, def.
param4, def.
param5, def.
param6, def.
param7, def.
param8, def.
param9, def.
param10, def.
param11);
5424 std::vector<float> gears_compat;
5428 std::vector<float>::iterator itor = def.
gear_ratios.begin();
5431 gears_compat.push_back(*itor);
5469 std::stringstream msg;
5470 msg <<
"Failed to retrieve required node: " << node_ref.
ToString();
5499 if (node !=
nullptr)
5509 node_t* ar_nodes[] = {
nullptr,
nullptr};
5511 if (ar_nodes[0] ==
nullptr)
5517 if (ar_nodes[1] ==
nullptr)
5527 beam.
k = def.
defaults->GetScaledSpringiness();
5528 beam.
d = def.
defaults->GetScaledDamping();
5536 float beam_strength = def.
defaults->GetScaledBreakingThreshold();
5655 if (beam_defaults->_is_user_defined)
5657 default_deform = beam_defaults->deformation_threshold;
5658 if (!beam_defaults->_enable_advanced_deformation && default_deform <
BEAM_DEFORM)
5663 if (beam_defaults->_is_plastic_deform_coef_user_defined && beam_defaults->plastic_deform_coef >= 0.f)
5670 if (default_deform < beam_creak)
5672 default_deform = beam_creak;
5675 float deformation_threshold = default_deform * beam_defaults->scale.deformation_threshold_constant;
5684 std::string material_name = material_override;
5685 if (material_name.empty())
5689 material_name =
"tracks/Chrome";
5693 material_name = beam_defaults->beam_material_name;
5698 auto material = it->second;
5699 if (!material.isNull())
5701 material_name = material->getName();
5715 entity->setMaterialName(material_name);
5718 beamx.
rod_diameter_mm = uint16_t(beam_defaults->visual_beam_diameter * 1000.f);
5728 beamx.
rod_scenenode->setScale(beam_defaults->visual_beam_diameter, -1, beam_defaults->visual_beam_diameter);
5732 catch (Ogre::Exception& e)
5741 beam.
L = beam_length;
5742 beam.
refL = beam_length;
5765 txt <<
": " << text;
5770 cm_type = RoR::Console::MessageType::CONSOLE_SYSTEM_ERROR;
5774 cm_type = RoR::Console::MessageType::CONSOLE_SYSTEM_WARNING;
5778 cm_type = RoR::Console::MessageType::CONSOLE_SYSTEM_NOTICE;
5792 bool is_imported = node_ref.GetImportState_IsValid();
5793 bool is_named = (is_imported ? node_ref.GetImportState_IsResolvedNamed() : node_ref.GetRegularState_IsNamed());
5802 std::stringstream msg;
5803 msg <<
"Failed to resolve node-ref (node not found):" << node_ref.
ToString();
5814 std::stringstream msg;
5840 if (node ==
nullptr)
5842 std::stringstream msg;
5843 msg <<
"Required node not found: " << node_ref.
ToString();
5853 std::stringstream msg;
5854 msg <<
"Attempt to add node with 'INVALID' flag: " <<
id.ToString() <<
" (number of nodes at this point: " <<
m_actor->
ar_num_nodes <<
")";
5856 return std::make_pair(0,
false);
5859 if (
id.IsTypeNamed())
5862 auto insert_result =
m_named_nodes.insert(std::make_pair(
id.
Str(), new_index));
5863 if (! insert_result.second)
5865 std::stringstream msg;
5866 msg <<
"Ignoring named node! Duplicate name: " <<
id.Str() <<
" (number of nodes at this point: " <<
m_actor->
ar_num_nodes <<
")";
5868 return std::make_pair(0,
false);
5874 return std::make_pair(new_index,
true);
5876 if (
id.IsTypeNumbered())
5880 std::stringstream msg;
5881 msg <<
"Duplicate node number, previous definition will be overriden! - " <<
id.ToString() <<
" (number of nodes at this point: " <<
m_actor->
ar_num_nodes <<
")";
5887 return std::make_pair(new_index,
true);
5890 throw Exception(
"Invalid Node::Id without type flags!");
5895 std::pair<unsigned int, bool> inserted_node =
AddNode(def.
id);
5896 if (! inserted_node.second)
5902 node.
pos = inserted_node.first;
6032 if (exhaust.
smoker ==
nullptr)
6040 exhaust.
smoker->setMaterialName(mat->getName(), mat->getGroup());
6070 for (
unsigned int i = 0; i < 8; i++)
6091 Ogre::Vector3
const & position,
6092 std::shared_ptr<RigDef::NodeDefaults> node_defaults
6109 Ogre::MaterialPtr mat = Ogre::MaterialManager::getSingleton().getByName(def.
material_name);
6116 std::stringstream msg;
6117 msg <<
"Material '" << def.
material_name <<
"' defined in section 'globals' not found. Trying material 'tracks/transred'";
6133 std::stringstream msg;
6145 std::stringstream msg;
6146 msg <<
"Axle limit (" <<
MAX_WHEELS/2 <<
") exceeded";
6157 std::stringstream msg;
6169 std::stringstream msg;
6182 std::stringstream msg;
6194 std::stringstream msg;
6195 msg <<
"Cab limit (" <<
MAX_CABS <<
") exceeded";
6206 std::stringstream msg;
6218 std::stringstream msg;
6230 std::stringstream msg;
6416 Ogre::String index_str =
TOSTRING(i+1);
6484 for (
auto& def: module->materialflarebindings)
6486 if (def.material_name == material_name)
6499 for (
auto& def: module->videocameras)
6501 if (def.material_name == material_name)
6519 return lookup_res->second.material;
6525 if (mat_lookup_name ==
"mirror")
6530 static int mirror_counter = 0;
6531 const std::string new_mat_name = this->
ComposeName(
"RenderMaterial", mirror_counter);
6533 lookup_entry.
material = Ogre::MaterialManager::getSingleton().getByName(
"mirror")->clone(new_mat_name,
true, mat_lookup_rg);
6541 if (videocam_def !=
nullptr)
6543 Ogre::MaterialPtr video_mat_shared;
6547 video_mat_shared = found_managedmat->second;
6551 video_mat_shared = Ogre::MaterialManager::getSingleton().getByName(mat_lookup_name);
6554 if (!video_mat_shared.isNull())
6558 lookup_entry.
material = video_mat_shared->clone(video_mat_name,
true, mat_lookup_rg);
6564 std::stringstream msg;
6565 msg <<
"VideoCamera material '" << mat_lookup_name <<
"' not found! Ignoring videocamera.";
6572 if (mat_flare_def !=
nullptr)
6582 auto skin_res = skin_def->replace_materials.find(mat_lookup_name);
6583 if (skin_res != skin_def->replace_materials.end())
6585 Ogre::MaterialPtr skin_mat = Ogre::MaterialManager::getSingleton().getByName(
6587 if (!skin_mat.isNull())
6589 lookup_entry.
material = skin_mat->clone(this->
ComposeName(skin_mat->getName()),
true, mat_lookup_rg);
6595 std::stringstream buf;
6599 <<
"')! Ignoring it...";
6610 lookup_entry.
material = mmat_res->second;
6615 Ogre::MaterialPtr orig_mat = Ogre::MaterialManager::getSingleton().getByName(mat_lookup_name, mat_lookup_rg);
6616 if (orig_mat.isNull())
6618 std::stringstream buf;
6619 buf <<
"Material doesn't exist:" << mat_lookup_name;
6621 return Ogre::MaterialPtr();
6624 lookup_entry.
material = orig_mat->clone(this->
ComposeName(orig_mat->getName()),
true, mat_lookup_rg);
6636 for (
auto& technique: lookup_entry.
material->getTechniques())
6638 for (
auto& pass: technique->getPasses())
6640 for (
auto& tex_unit: pass->getTextureUnitStates())
6643 if (tex_unit->getTextureName() ==
"dashtexture")
6648 std::stringstream msg;
6649 msg <<
"Warning: '" << mat_lookup_name
6650 <<
"' references 'dashtexture', but Renderdash isn't created yet! Texture will be blank.";
6661 const size_t num_frames = tex_unit->getNumFrames();
6662 for (
size_t i = 0; i < num_frames; ++i)
6671 Ogre::TexturePtr tex = Ogre::TextureManager::getSingleton().getByName(
6679 tex = Ogre::TextureManager::getSingleton().create(
6682 tex_unit->_setTexturePtr(tex, i);
6686 tex_unit->setFrameTextureName(query->second, (
unsigned int)i);
6698 catch (Ogre::Exception& e)
6700 std::stringstream msg;
6701 msg <<
"Exception while customizing material \"" << mat_lookup_name <<
"\", message: " << e.getFullDescription();
6704 return Ogre::MaterialPtr();
6711 static unsigned int simple_mat_counter = 0;
6713 newmat->getTechnique(0)->getPass(0)->setAmbient(color);
6749 size_t num_sub_entities = ent->getNumSubEntities();
6750 for (
size_t i = 0; i < num_sub_entities; i++)
6752 Ogre::SubEntity* subent = ent->getSubEntity(i);
6753 subent->setMaterial(mat);
6760 size_t subent_max = ent->getNumSubEntities();
6761 for (
size_t i = 0; i < subent_max; ++i)
6763 Ogre::SubEntity* subent = ent->getSubEntity(i);
6765 if (!subent->getMaterial().isNull())
6768 if (!own_mat.isNull())
6770 subent->setMaterial(own_mat);
6784 if (entry.second.material_flare_def !=
nullptr)
6787 entry.second.material_flare_def->flare_number, entry.second.material);
6792 entry.second.material, entry.second.mirror_prop_type, entry.second.mirror_prop_scenenode);
6794 else if (entry.second.video_camera_def !=
nullptr)
6810 for (
auto& gs: module->guisettings)
6812 if (gs.key ==
"dashboard")
6816 else if (gs.key ==
"texturedashboard")
6903 mat->getNumTechniques() > 0 &&
6904 mat->getTechnique(0)->getNumPasses() > 0 &&
6905 mat->getTechnique(0)->getPass(0)->getNumTextureUnitStates() > 0 &&
6906 mat->getTechnique(0)->getPass(0)->getTextureUnitState(0)->getNumFrames() > 0)
6909 Ogre::TextureManager::getSingleton().getByName(
6913 catch (Ogre::Exception& e)
6916 "Failed to load `help` material '" +
m_help_material_name +
"', message:" + e.getFullDescription());
6925 const float eps = 0.001f;
6928 Ogre::Plane pl = Ogre::Plane((ax1 - ax2).normalisedCopy(), 0);
6934 float a1len = a1.normalise();
6935 float a2len = a2.normalise();
6936 float a3len = a3.normalise();
6937 float a4len = a4.normalise();
6938 if ((std::max(a1len, a3len) / std::min(a1len, a3len) > 1.f + eps) ||
6939 (std::max(a2len, a4len) / std::min(a2len, a4len) > 1.f + eps))
6949 float b1len = b1.normalise();
6950 float b2len = b2.normalise();
6951 float b3len = b3.normalise();
6952 float b4len = b4.normalise();
6953 if ((std::max(b1len, b3len) / std::min(b1len, b3len) > 1.f + eps) ||
6954 (std::max(b2len, b4len) / std::min(b2len, b4len) > 1.f + eps))
6960 float rot1 = a1.dotProduct(b1);
6961 float rot2 = a2.dotProduct(b2);
6962 float rot3 = a3.dotProduct(b3);
6963 float rot4 = a4.dotProduct(b4);
6964 if ((std::max(rot1, rot2) / std::min(rot1, rot2) > 1.f + eps) ||
6965 (std::max(rot2, rot3) / std::min(rot2, rot3) > 1.f + eps) ||
6966 (std::max(rot3, rot4) / std::min(rot3, rot4) > 1.f + eps) ||
6967 (std::max(rot4, rot1) / std::min(rot4, rot1) > 1.f + eps))
6978 Ogre::MaterialPtr mat = Ogre::MaterialManager::getSingleton().create(
6979 "VideoCamDebugMat-" +
TOSTRING(
counter), Ogre::ResourceGroupManager::DEFAULT_RESOURCE_GROUP_NAME);
6981 mat->getTechnique(0)->getPass(0)->createTextureUnitState();
6982 mat->getTechnique(0)->getPass(0)->getTextureUnitState(0)->setTextureFiltering(Ogre::TFO_ANISOTROPIC);
6983 mat->getTechnique(0)->getPass(0)->getTextureUnitState(0)->setTextureAnisotropy(3);
6984 mat->setLightingEnabled(
false);
6985 mat->setReceiveShadows(
false);
6988 mo->begin(mat->getName(), Ogre::RenderOperation::OT_LINE_LIST);
6989 Ogre::ColourValue pos_mark_col(1.f, 0.82f, 0.26f);
6990 Ogre::ColourValue dir_mark_col(0.f, 1.f, 1.f);
6991 const float pos_mark_len = 0.8f;
6992 const float dir_mark_len = 4.f;
6994 mo->position(pos_mark_len,0,0);
6995 mo->colour(pos_mark_col);
6996 mo->position(-pos_mark_len,0,0);
6997 mo->colour(pos_mark_col);
6999 mo->position(0,pos_mark_len,0);
7000 mo->colour(pos_mark_col);
7001 mo->position(0,-pos_mark_len,0);
7002 mo->colour(pos_mark_col);
7004 mo->position(0,0,pos_mark_len);
7005 mo->colour(pos_mark_col);
7006 mo->position(0,0,0);
7007 mo->colour(pos_mark_col);
7009 mo->position(0,0,-dir_mark_len);
7010 mo->colour(dir_mark_col);
7011 mo->position(0,0,0);
7012 mo->colour(dir_mark_col);
7049 = Ogre::Quaternion(Ogre::Degree(rotation_z), Ogre::Vector3::UNIT_Z)
7050 * Ogre::Quaternion(Ogre::Degree(def->
rotation.y), Ogre::Vector3::UNIT_Y)
7051 * Ogre::Quaternion(Ogre::Degree(def->
rotation.x), Ogre::Vector3::UNIT_X);
7076 vcam.
vcam_render_tex = Ogre::TextureManager::getSingleton().createManual(
7078 Ogre::ResourceGroupManager::DEFAULT_RESOURCE_GROUP_NAME,
7084 Ogre::TU_RENDERTARGET);
7103 vcam.
vcam_material->getTechnique(0)->getPass(0)->setLightingEnabled(
false);
7109 vp->setClearEveryFrame(
true);
7113 vp->setOverlaysEnabled(
false);
7119 vcam.
vcam_material->getTechnique(0)->getPass(0)->getTextureUnitState(0)->setTextureUScale(-1);
7125 vp->setClearEveryFrame(
true);
7129 vp->setOverlaysEnabled(
false);
7143 catch (std::exception & ex)
7156 static int mprop_counter = 0;
7179 vcam.
vcam_render_tex = Ogre::TextureManager::getSingleton().createManual(
7180 this->
ComposeName(
"texture @ mirror", mprop_counter)
7187 , Ogre::TU_RENDERTARGET);
7201 v->setClearEveryFrame(
true);
7203 v->setOverlaysEnabled(
false);
7208 vcam.
vcam_material->getTechnique(0)->getPass(0)->setLightingEnabled(
false);
7213 catch (std::exception & ex)
7228 catch (Ogre::Exception& ogre_e)
7233 <<
") " << ogre_e.getFullDescription();
7237 catch (std::exception& std_e)
7252 Ogre::NameValuePairList params;
7254 params[
"templateName"] = template_name;
7257 name, Ogre::ParticleSystemFactory::FACTORY_TYPE_NAME, ¶ms);
7258 Ogre::ParticleSystem* psys =
static_cast<Ogre::ParticleSystem*
>(obj);
7279 Ogre::MaterialPtr mat = Ogre::MaterialManager::getSingleton().getByName(
m_cab_material_name);
7288 char transmatname[256];
7289 static int trans_counter = 0;
7291 Ogre::MaterialPtr transmat=mat->clone(transmatname);
7292 if (mat->getTechnique(0)->getNumPasses()>1)
7294 transmat->getTechnique(0)->removePass(1);
7296 transmat->getTechnique(0)->getPass(0)->setAlphaRejectSettings(Ogre::CMPF_LESS_EQUAL, 128);
7297 transmat->getTechnique(0)->getPass(0)->setDepthWriteEnabled(
false);
7298 if (transmat->getTechnique(0)->getPass(0)->getNumTextureUnitStates()>0)
7300 transmat->getTechnique(0)->getPass(0)->getTextureUnitState(0)->setTextureFiltering(Ogre::TFO_NONE);
7302 transmat->compile();
7306 char backmatname[256];
7307 static int back_counter = 0;
7309 Ogre::MaterialPtr backmat=mat->clone(backmatname);
7310 if (mat->getTechnique(0)->getNumPasses()>1)
7312 backmat->getTechnique(0)->removePass(1);
7314 if (transmat->getTechnique(0)->getPass(0)->getNumTextureUnitStates()>0)
7316 backmat->getTechnique(0)->getPass(0)->getTextureUnitState(0)->setColourOperationEx(
7320 Ogre::ColourValue(0,0,0),
7321 Ogre::ColourValue(0,0,0)
7326 backmat->setReceiveShadows(
false);
7332 char cab_material_name_cstr[1000] = {};
7334 std::string mesh_name = this->
ComposeName(
"mesh @ cab");
7342 cab_material_name_cstr,
7349 Ogre::Entity *ec =
nullptr;
7356 cab_scene_node->attachObject(ec);
7369 catch (Ogre::Exception& e)
7387 Ogre::Technique* tech = m->getTechnique(0);
7390 Ogre::Pass* p = tech->getPass(0);
7395 p->setSelfIllumination(Ogre::ColourValue::ZERO);
7425 Ogre::TexturePtr tex = Ogre::TextureManager::getSingleton().load(
7431 tus->setTexture(tex);
static const BitMask_t OPTION_u_INPUT_AILERON_ELEVATOR
unsigned int contract_key
#define ROR_ASSERT(_EXPR)
Game state manager and message-queue provider.
int16_t nd_coll_bbox_id
Optional attribute (-1 = none) - multiple collision bounding boxes defined in truckfile.
static const BitMask64_t SOURCE_EVENT
std::shared_ptr< BeamDefaults > beam_defaults
float ar_anim_previous_crank
For 'animator' with flag 'torque'.
void ResolveUnwantedAndTweakedElements(TuneupDefPtr &tuneup, CacheEntryPtr &addonpart_entry)
Evaluates 'addonpart_unwanted_*' elements, respecting 'protected_*' directives in the tuneup.
void setInertialReferences(node_t *refl, node_t *refr, node_t *refb, node_t *refc)
DifferentialTypeVec options
Order matters!
void ProcessWing(RigDef::Wing &def)
std::string * ar_nodes_name
Name in truck file, only if defined with 'nodes2'.
static const int MAX_COMMANDS
maximum number of commands per actor
int tr_ax_2
This axle is only driven in 4WD mode.
node_t * wh_rim_nodes[50]
void LoadAssetPack(CacheEntryPtr &t_dest, Ogre::String const &assetpack_filename)
Adds asset pack to the requesting cache entry's resource group.
float spring_out
spring value applied when shock extending
static const BitMask64_t SOURCE_ANGLE_OF_ATTACK
static const BitMask_t OPTION_c_COMMAND_STYLE
std::vector< Ogre::AxisAlignedBox > ar_collision_bounding_boxes
smart bounding boxes, used for determining the state of an actor (every box surrounds only a subset o...
std::shared_ptr< RigDef::Document::Module > m_current_module
For resolving addonparts.
bool cc_mode
Cruise Control.
Ogre::Quaternion vcam_rotation
static const BitMask_t OPTION_PITCH
const PropAnimFlag_t PROP_ANIM_FLAG_AIRSPEED
static const BitMask_t OPTION_e_INPUT_ELEVATOR
const PropAnimFlag_t PROP_ANIM_FLAG_TORQUE
int last_debug_state
smart debug output
int ar_num_contactable_nodes
Total number of nodes which can contact ground or cabs.
std::shared_ptr< BeamDefaults > beam_defaults
SoundScriptManager * GetSoundScriptManager()
static const BitMask_t CONSTRAINT_ATTACH_FOREIGN
BitMask_t constraint_flags
static const BitMask64_t SOURCE_FLAP
RoR::CmdKeyInertia command_inertia
const PropAnimFlag_t PROP_ANIM_FLAG_SHIFTER
'shifterman1, shifterman2, sequential, shifterlin, autoshifterlin'; animOpt3: see RoR::ShifterPropAni...
static const BitMask64_t SOURCE_VERTICAL_VELOCITY
float m_odometer_user
GUI state.
static const BitMask_t OPTION_A_INV_TRIGGER_BLOCKER
float damp_out_fast
Damping value applied when shock is commpressing faster than split out velocity.
bool nd_contacter
Attr; User-defined.
SoundScriptInstancePtr ssi
NodeNum_t ar_main_camera_node_pos
Sim attr; ar_camera_node_pos[0] >= 0 ? ar_camera_node_pos[0] : 0.
VehicleAIPtr ar_vehicle_ai
bool CheckSubmeshLimit(unsigned int count)
float upper_limit
The upper limit for the animation.
float extension_break_limit
Node::Ref blade_tip_nodes[4]
static const BitMask_t OPTION_p_10xTOUGHER
Ogre::String dname
name parsed from the file
std::vector< SlideNode > m_slidenodes
all the SlideNodes available on this actor
std::vector< Node::Ref > nodes
const PropAnimMode_t PROP_ANIM_MODE_OFFSET_X
void ProcessHook(RigDef::Hook &def)
CVar * gfx_speedo_imperial
bool cmb_is_force_restricted
Attribute defined in truckfile.
static const BitMask_t SOURCE_AERO_TORQUE
static void AddSoundSource(ActorPtr const &vehicle, SoundScriptInstancePtr sound_script, NodeNum_t node_index, int type=-2)
NodeNum_t ar_camera_node_dir[MAX_CAMERAS]
Physics attr; 'camera' = frame of reference; back node.
void ProcessHydro(RigDef::Hydro &def)
std::shared_ptr< BeamDefaults > beam_defaults
CameraSettings camera_settings
static const BitMask_t OPTION_x_EXHAUST_POINT
Legacy parser resolved references on-the-fly and the condition to check named nodes was "are there an...
RigDef::Keyword m_current_keyword
For error reports.
std::shared_ptr< Inertia > inertia_defaults
CameraMode_t pp_camera_mode_orig
Dynamic visibility mode {0 and higher = cinecam index}.
const PropAnimFlag_t PROP_ANIM_FLAG_AIRBRAKE
bool nd_immovable
Attr; User-defined.
const PropAnimFlag_t PROP_ANIM_FLAG_AOA
void ProcessSubmesh(RigDef::Submesh &def)
int ar_buoycabs[MAX_CABS]
void ProcessGlobals(RigDef::Globals &def)
static const BitMask_t OPTION_SHIFT_BACK_FORTH
std::string m_custom_resource_group
Ogre::BillboardSet * bbs
This remains nullptr if removed via addonpart_unwanted_flare or Tuning UI.
std::shared_ptr< Inertia > inertia_defaults
static const float NODE_FRICTION_COEF_DEFAULT
static const BitMask64_t SOURCE_AUTOSHIFTERLIN
void ProcessFlare2(RigDef::Flare2 &def)
float default_braking_force
bool option_o_1press_center
static const BitMask_t SOURCE_AERO_PITCH
node_t * m_fusealge_back
Physics attr; defined in truckfile.
collcab_rate_t ar_inter_collcabrate[MAX_CABS]
beam_t & GetBeam(unsigned int index)
NodeNum_t ar_extern_camera_node
std::shared_ptr< NodeDefaults > node_defaults
void ProcessRope(RigDef::Rope &def)
WingControlSurface control_surface
bool CheckCameraRailLimit(unsigned int count)
void UpdateSimDataBuffer()
Copies sim. data from Actor to GfxActor for later update.
@ TRUCK
its a truck (or other land vehicle)
ExtCameraMode ar_extern_camera_mode
static const BitMask_t OPTION_s_SOFT_BUMP_BOUNDS
Visuals of softbody beam (beam_t struct); Partially updated along with SimBuffer.
Ogre::MaterialPtr vcam_material
void SetSimpleDelay(RoR::CmdKeyInertiaConfig &cfg, float start_delay, float stop_delay, std::string start_function, std::string stop_function)
Ogre::RenderWindow * CreateCustomRenderWindow(std::string const &name, int width, int height)
void ProcessSoundSource(RigDef::SoundSource &def)
Ogre::String start_function
static const int MAX_CLIGHTS
See RoRnet::Lightmask and enum events in InputEngine.h.
unsigned int texture_width
float alb_pulse_time
Anti-lock brake attribute;.
@ SHOCK_FLAG_TRG_BLOCKER_A
int spaceCurveEvenly(Ogre::SimpleSpline *spline)
Spaces the points of a spline evenly; this is needed for the correct calculation of the Ogre simple s...
std::shared_ptr< BeamDefaults > beam_defaults
RigDef::MaterialFlareBinding * FindFlareBindingForMaterial(std::string const &material_name)
Returns NULL if none found.
void ConfigureSections(Ogre::String const §ionconfig, RigDef::DocumentPtr def)
float springin
shocks2 & shocks3
cparticle_t ar_custom_particles[MAX_CPARTICLES]
const PropAnimFlag_t PROP_ANIM_FLAG_BRAKE
int m_num_wheel_diffs
Physics attr.
WheelID_t AddWheel2(RigDef::Wheel2 &wheel_2_def)
float post_shift_time
Seconds.
static const BitMask_t OPTION_R_ACTIVE_RIGHT
CameraManager * GetCameraManager()
static const BitMask_t SOURCE_AERO_RPM
Ogre::Vector3 AbsPosition
absolute position in the world (shaky)
const std::string & Str() const
float pp_beacon_rot_angle[4]
Radians.
void ProcessMinimass(RigDef::Minimass &def)
bool m_trigger_debug_enabled
Logging state.
float long_bound
Maximum extension. The longest length a shock can be, as a proportion of its original length....
void ProcessEngine(RigDef::Engine &def)
void ProcessGuiSettings(RigDef::GuiSettings &def)
const PropAnimMode_t PROP_ANIM_MODE_ROTA_Z
int mode
A special constant or cinecam index.
void setMaterialName(Ogre::String m)
static const BitMask_t OPTION_SEQUENTIAL_SHIFT
static const float BEAM_DEFORM
RigDef::DocumentPtr m_file
float lower_limit
The lower limit for the animation.
bool trigger_enabled
general trigger,switch and blocker state
std::vector< Wheel > wheels
std::shared_ptr< RoR::SkinDef > skin_def
Cached skin info, added on first use or during cache rebuild.
int dashboard_link
Only 'd' type flares, valid values are DD_*.
static const BitMask_t OPTION_ALTIMETER_1K
const PropAnimFlag_t PROP_ANIM_FLAG_DASHBOARD
Used with dashboard system inputs, see enum DashData in file DashBoardManager.h.
std::vector< float > gear_ratios
bool ar_collision_relevant
Physics state;.
void ProcessBrakes(RigDef::Brakes &def)
Ogre::TexturePtr vcam_render_tex
bool sn_attach_foreign
Attach/detach to rails only on other vehicles.
Ogre::Entity * GetTireEntity()
std::string ToString() const
Ogre::SimpleSpline * getUsedSpline()
Returns the used spline.
ActorInstanceID_t ar_instance_id
Static attr; session-unique ID.
CacheEntryPtr FindEntryByFilename(RoR::LoaderType type, bool partial, const std::string &filename)
Returns NULL if none found.
float cc_target_rpm
Cruise Control.
A series of RailSegment-s for SlideNode to slide along. Can be closed in a loop.
Ogre::SceneNode * m_particles_parent_scenenode
this isn't used for moving/hiding things, just helps developers inspect the scene graph.
CameraSettings camera_settings
bool m_engine_has_air
Engine attribute.
float split_vel_out
Split velocity in (m/s) - threshold for slow / fast damping during extension.
void CheckAndLoadFlexbodyCache()
static const BitMask_t OPTION_SHIFT_LEFT_RIGHT
Ogre::SceneNode * m_actor_grouping_scenenode
Topmost common parent; this isn't used for moving things, just helps developers inspect the scene gra...
float tc_ratio
Traction control attribute: Regulating force.
static const BitMask_t MODE_BOUNCE
Ogre::Entity * getEntity()
int loadDashBoard(Ogre::String filename, bool textureLayer)
std::vector< Ogre::SceneNode * > m_deletion_scene_nodes
For unloading vehicle; filled at spawn.
std::vector< Shock2 > shocks2
static const BitMask64_t SOURCE_TACHO
std::vector< Command2 > commands2
static bool isPropAnyhowRemoved(TuneupDefPtr &tuneup_def, PropID_t prop_id)
static const BitMask_t OPTION_PITCH
Ogre::ParticleSystem * smoker
This remains nullptr if removed via addonpart_unwanted_exhaust or Tuning UI.
std::vector< exhaust_t > exhausts
const PropAnimMode_t PROP_ANIM_MODE_ROTA_X
int m_num_axle_diffs
Physics attr.
bool sn_attach_self
Attach/detach to rails on the current vehicle only.
Differential * m_wheel_diffs[MAX_WHEELS/2]
Physics.
float m_fusealge_width
Physics attr; defined in truckfile.
float hb_ref_length
Idle length in meters.
Ogre::String damaged_diffuse_map
static const BitMask_t OPTION_CLUTCH
AppContext * GetAppContext()
static const NodeNum_t NODENUM_INVALID
std::vector< UniqueCommandKeyPair > ar_unique_commandkey_pairs
UI helper for displaying command control keys to user (must be built at spawn).
std::string ComposeName(const std::string &object, int number=-1)
Creates name containing actor ID token, i.e. "Object#1 (filename.truck [Instance ID 1])".
void ProcessExtCamera(RigDef::ExtCamera &def)
bool nd_contactable
Attr; This node will be treated as contacter on inter truck collisions.
void ProcessRotator(RigDef::Rotator &def)
std::vector< Wing > wings
ground_model_t * defaultgm
void ProcessHelp(RigDef::Help &def)
static const int8_t INVALID_BBOX
Truck file format(technical spec)
void SetBeamDeformationThreshold(beam_t &beam, std::shared_ptr< RigDef::BeamDefaults > beam_defaults)
void BuildWheelBeams(unsigned int num_rays, NodeNum_t base_node_index, node_t *axis_node_1, node_t *axis_node_2, float tyre_spring, float tyre_damping, float rim_spring, float rim_damping, std::shared_ptr< RigDef::BeamDefaults > beam_defaults, RigDef::Node::Ref const &rigidity_node_id, float max_extension=0.f)
'wheels', 'meshwheels'
bool nd_override_mass
User defined attr; mass is user-specified rather than calculated (override the calculation)
static const BitMask_t OPTION_FLAP
bool nx_no_particles
User-defined attr; disable all particles.
CVar * diag_simple_materials
Ogre::String particle_system_name
void AddExhaust(NodeNum_t emitter_node_idx, NodeNum_t direction_node_idx)
void ProcessShock(RigDef::Shock &def)
std::shared_ptr< BeamDefaults > beam_defaults
static const BitMask_t CONSTRAINT_ATTACH_SELF
static const BitMask_t OPTION_ANGLE_OF_ATTACK
static const BitMask64_t SOURCE_PARKING
int longbound_trigger_action
int ar_buoycab_types[MAX_CABS]
void ProcessLockgroup(RigDef::Lockgroup &lockgroup)
int m_proped_wheel_pairs[MAX_WHEELS]
Physics attr; For inter-differential locking.
void CreateWheelSkidmarks(WheelID_t wheel_index)
Ogre::SceneNode * wx_scenenode
Consists of static mesh, representing the rim, and dynamic mesh, representing the tire.
Ogre::MaterialPtr m_simple_material_base
bool uses_inertia
Only 'flares3'.
RigDef::DocumentPtr m_definition
CVar * gfx_particles_mode
static const BitMask64_t SOURCE_BOAT_RUDDER
bool sl_enabled
Speed limiter;.
NodeNum_t FindNodeIndex(RigDef::Node::Ref &node_ref, bool silent=false)
BackmeshType backmesh_type
float spring_in
Spring value applied when the shock is compressing.
void AddCurveSample(float rpm, float progress, Ogre::String const &model=customModel)
Adds a point to the torque curve graph.
CVar * gfx_enable_videocams
int pos
Index into ar_ropables.
const char * KeywordToString(Keyword keyword)
void ProcessCamera(RigDef::Camera &def)
std::vector< Rope > ropes
float cmb_engine_coupling
Attr from truckfile.
float tc_pulse_time
Traction control attribute;.
int m_masscount
Physics attr; Number of nodes loaded with l option.
static const BitMask_t OPTION_AIR_BRAKE
float trigger_boundary_t
optional value to tune trigger_switch_state autorelease
NodeNum_t vcam_node_center
static const BitMask_t OPTION_m_METRIC
void SetVisible(bool visible)
void ProcessContacter(RigDef::Node::Ref &node_ref)
void SetCurrentKeyword(RigDef::Keyword keyword)
static const BitMask_t OPTION_L_ACTIVE_LEFT
void LogFormat(const char *format,...)
Improved logging utility. Uses fixed 2Kb buffer.
std::vector< Node::Range > rail_node_ranges
static const BitMask64_t SOURCE_PITCH
std::vector< Airbrake > airbrakes
void ProcessMeshWheel2(RigDef::MeshWheel2 &def)
static const BitMask_t SOURCE_AERO_STATUS
static const BitMask_t OPTION_BOAT_THROTTLE
void ProcessFusedrag(RigDef::Fusedrag &def)
const PropAnimMode_t PROP_ANIM_MODE_NOFLIP
std::vector< Node::Ref > nodes
Ogre::TexturePtr getTexture()
float precompression
Changes compression or extension of the suspension when the truck spawns. This can be used to "level"...
std::vector< Node > nodes
Ogre::Vector3 pp_offset_orig
Used with ANIM_FLAG_OFFSET*.
System integration layer; inspired by OgreBites::ApplicationContext.
static const BitMask_t OPTION_n_INPUT_NORMAL
void ProcessAirbrake(RigDef::Airbrake &def)
void ProcessTorqueCurve(RigDef::TorqueCurve &def)
void putMessage(MessageArea area, MessageType type, std::string const &msg, std::string icon="")
std::vector< hook_t > ar_hooks
User input state for animated props with 'source:event'.
static const BitMask64_t SOURCE_ALTIMETER_10K
CVar * sim_no_self_collisions
A land vehicle engine + transmission.
float hk_min_length
Absolute value in meters.
unsigned int spin_right_key
std::shared_ptr< RigDef::Document::Module > TransformToRigDefModule(CacheEntryPtr &addonpart_entry)
transforms the addonpart to RigDef::File::Module (fake 'section/end_section') used for spawning.
bool WasDashboardLoaded() const
Ogre::SceneNode * mirror_prop_scenenode
This class loads and processes a torque curve for a vehicle.
bool tr_4wd_mode
Enables 4WD mode.
std::vector< wheeldetacher_t > ar_wheeldetachers
float ComputeWingArea(Ogre::Vector3 const &ref, Ogre::Vector3 const &x, Ogre::Vector3 const &y, Ogre::Vector3 const &aref)
Ogre::String resource_group
Resource group of the loaded bundle. Empty if not loaded yet.
static const BitMask_t OPTION_p_NO_PARTICLES
static const BitMask_t OPTION_u_INVULNERABLE
bool m_generate_wing_position_lights
std::vector< tie_t > ar_ties
Ogre::RenderTexture * vcam_render_target
static CameraMode_t CAMERA_MODE_ALWAYS_HIDDEN
float dampout
shocks2 & shocks3
float sl_speed_limit
Speed limiter;.
float cc_target_speed_lower_limit
Cruise Control.
void ConfigureAddonParts(TuneupDefPtr &tuneup_def)
A visual mesh, forming a chassis for softbody actor At most one instance is created per actor.
void SetAutoMode(RoR::SimGearboxMode mode)
bool nx_no_sparks
User-defined attr;.
Ogre::Real wh_mass
Total rotational mass of the wheel.
std::string getTruckFileResourceGroup()
static const BitMask_t OPTION_TORQUE
NodeNum_t ar_main_camera_node_dir
Sim attr; ar_camera_node_dir[0] >= 0 ? ar_camera_node_dir[0] : 0.
uint16_t rod_diameter_mm
Diameter in millimeters.
const PropAnimFlag_t PROP_ANIM_FLAG_PBRAKE
RoR::FlexFactory m_flex_factory
bool ar_hide_in_actor_list
Hide in list of spawned actors (available in top menubar). Useful for fixed-place machinery,...
std::set< std::string > use_addonparts
Addonpart filenames.
Designed to be run on main/rendering loop (FPS)
static const BitMask_t MODE_EVENT_LOCK
static const BitMask_t OPTION_SPEEDO
TorqueCurve * getTorqueCurve()
NodeNum_t vcam_node_dir_y
static Ogre::Vector3 getTweakedPropOffset(TuneupDefPtr &tuneup_entry, PropID_t prop_id, Ogre::Vector3 orig_val)
static Ogre::Vector3 getTweakedPropRotation(TuneupDefPtr &tuneup_entry, PropID_t prop_id, Ogre::Vector3 orig_val)
const PropAnimFlag_t PROP_ANIM_FLAG_ARUDDER
std::map< std::string, Ogre::MaterialPtr > m_managed_materials
Ogre::Vector3 RelPosition
relative to the local physics origin (one origin per actor) (shaky)
const PropAnimFlag_t PROP_ANIM_FLAG_RPM
static const BitMask_t OPTION_BRAKES
void SetBeamSpring(beam_t &beam, float spring)
char pp_beacon_type
Special prop: beacon {0 = none, 'b' = user-specified, 'r' = red, 'p' = police lightbar,...
std::shared_ptr< commandbeam_state_t > cmb_state
static const BitMask64_t SOURCE_SHIFTERLIN
static const float HOOK_SPEED_DEFAULT
Ogre::SceneNode * vcam_debug_node
void ValidateRotator(int id, int axis1, int axis2, NodeNum_t *nodes1, NodeNum_t *nodes2)
static const BitMask64_t SOURCE_AIR_RUDDER
RigDef::VideoCamera * FindVideoCameraByMaterial(std::string const &material_name)
Returns NULL if none found.
float long_bound
Maximum extension limit, in percentage ( 1.00 = 100% )
const PropAnimFlag_t PROP_ANIM_FLAG_ALTIMETER
void CalcMemoryRequirements(ActorMemoryRequirements &req, RigDef::Document::Module *module_def)
bool IsValidAnyState() const
DashBoardManager * ar_dashboard
static const BitMask_t OPTION_m_NO_MOUSE_GRAB
static const BitMask64_t SOURCE_AIRSPEED
Skidmark * m_skid_trails[MAX_WHEELS *2]
Ogre::Vector3 pp_wheel_pos
const PropAnimMode_t PROP_ANIM_MODE_ROTA_Y
int di_idx_2
array location of wheel / axle 2
static const BitMask_t OPTION_B_TRIGGER_BLOCKER
bool pp_aero_propeller_spin
Special - blurred spinning propeller effect.
void _ProcessKeyInertia(RigDef::Inertia &inertia, RigDef::Inertia &inertia_defaults, RoR::CmdKeyInertia &contract_key, RoR::CmdKeyInertia &extend_key)
std::shared_ptr< BeamDefaults > beam_defaults
node_t & GetAndInitFreeNode(Ogre::Vector3 const &position)
float m_avg_proped_wheel_radius
Physics attr, filled at spawn - Average proped wheel radius.
static const BitMask64_t SOURCE_ELEVATOR
char m_engine_type
Engine attribute {'t' = truck (default), 'c' = car}.
void AddDifferentialType(DiffType diff)
float animratio
A coefficient for the animation, prop degree if used with mode: rotation and propoffset if used with ...
void _ProcessSimpleInertia(RigDef::Inertia &def, RoR::SimpleInertia &obj)
int ExhaustID_t
Index into Actor::exhausts, use RoR::EXHAUSTID_INVALID as empty value.
Ogre::String predefined_func_name
ActorPtr rod_target_actor
void ProcessAxle(RigDef::Axle &def)
Ogre::RenderWindow * vcam_render_window
int hb_anim_flags
Animators (beams updating length based on simulation variables)
Ogre::SceneManager * GetSceneManager()
static const BitMask64_t SOURCE_ROLL
void ProcessEngturbo(RigDef::Engturbo &def)
bool tc_nodash
Traction control attribute; Hide the dashboard indicator?
static const BitMask_t SOURCE_GEAR_FORWARD
Ogre::MaterialPtr m_cab_trans_material
const PropAnimFlag_t PROP_ANIM_FLAG_STEERING
static const BitMask_t OPTION_INVISIBLE
float damp_in
Damping value applied when the shock is compressing.
const PropAnimFlag_t PROP_ANIM_FLAG_GEAR
'gearreverse' (animOpt3=-1), 'gearneutral' (animOpt3=0), 'gear#' (animOpt3=#)
float damp_out
damping value applied when shock extending
float clutch_time
Seconds.
float spring_out
Spring value applied when shock extending.
void ProcessEngoption(RigDef::Engoption &def)
int ar_num_contacters
Total number of nodes which can selfcontact cabs.
float refL
reference length
bool CheckCabLimit(unsigned int count)
Airfoil * m_fusealge_airfoil
Physics attr; defined in truckfile.
@ VCTYPE_MIRROR_PROP_LEFT
The classic 'special prop/rear view mirror'.
@ FAULTY_MESH_PLACEHOLDER
static const BitMask_t MODE_ROTATION_Y
static const BitMask_t OPTION_RPM
Ogre::SceneNode * pp_wheel_scene_node
bool tc_pulse_state
Traction control state;.
static const BitMask64_t SOURCE_AILERON
static const BitMask_t OPTION_g_INPUT_ELEVATOR_RUDDER
void ProcessShock3(RigDef::Shock3 &def)
bool m_has_command_beams
Physics attr;.
float ar_posnode_spawn_height
Ogre::String tyre_mesh_name
void AddMessage(Message type, Ogre::String const &text)
Maintenance.
std::vector< MeshWheel2 > meshwheels2
void ProcessAnimator(RigDef::Animator &def)
float short_bound
Maximum contraction. The shortest length the shock can be, as a proportion of its original length....
void addwash(int propid, float ratio)
static const BitMask_t OPTION_m_METRIC
Node::Ref alt_reference_node
float spring_rate
The 'stiffness' of the shock. The higher the value, the less the shock will move for a given bump.
void SetupNewEntity(Ogre::Entity *e, Ogre::ColourValue simple_color)
Full texture and material setup.
Ogre::ManualObject * CreateVideocameraDebugMesh()
static std::string getTweakedFlexbodyMediaRG(TuneupDefPtr &tuneup_def, FlexbodyID_t flexbody_id, int media_idx, const std::string &orig_val)
Ogre::MaterialPtr InstantiateManagedMaterial(Ogre::String const &rg_name, Ogre::String const &source_name, Ogre::String const &clone_name)
#define BITMASK_SET_0(VAR, FLAGS)
MeshObject * pp_wheel_mesh_obj
Simulation: An edge in the softbody structure.
AeroEngine * ar_aeroengines[MAX_AEROENGINES]
static const BitMask_t MODE_AUTO_ANIMATE
void ProcessShock2(RigDef::Shock2 &def)
static const BitMask_t OPTION_l_LOAD_WEIGHT
Ogre::Camera * GetCamera()
ManagedMaterialsOptions options
Ogre::MaterialPtr FindOrCreateCustomizedMaterial(const std::string &mat_lookup_name, const std::string &mat_lookup_rg)
@ SHOCK_FLAG_TRG_HOOK_LOCK
bool CheckParticleLimit(unsigned int count)
static void ResetUnwantedAndTweakedElements(TuneupDefPtr &tuneup)
int * ar_nodes_id
Number in truck file, -1 for nodes generated by wheels/cinecam.
RoR::Renderdash * m_oldstyle_renderdash
#define BITMASK_IS_0(VAR, FLAGS)
Screwprop * ar_screwprops[MAX_SCREWPROPS]
CVar * gfx_window_videocams
Ogre::String material_name
void CreateVideoCamera(RigDef::VideoCamera *def)
@ DD_PARKINGBRAKE
chassis pitch
static const BitMask_t OPTION_TORQUE
NodeNum_t ResolveNodeRef(RigDef::Node::Ref const &node_ref)
bool CheckAeroEngineLimit(unsigned int count)
void ProcessMeshWheel(RigDef::MeshWheel &def)
ropable_t * rp_locked_ropable
std::vector< Cinecam > cinecam
NodeNum_t rod_node1
Node index - may change during simulation!
static const float DEFAULT_COLLISION_RANGE
CVar * gfx_alt_actor_materials
bool _has_load_weight_override
std::string dashboard_link
Only 'd' type flares.
std::vector< Wheel2 > wheels2
bool cmb_is_contraction
Attribute defined at spawn.
const PropAnimFlag_t PROP_ANIM_FLAG_AETORQUE
void SetTurboOptions(int type, float tinertiaFactor, int nturbos, float param1, float param2, float param3, float param4, float param5, float param6, float param7, float param8, float param9, float param10, float param11)
Sets turbo options.
void ProcessRopable(RigDef::Ropable &def)
static const BitMask_t OPTION_i_INVISIBLE
std::vector< authorinfo_t > authors
static const BitMask_t OPTION_LONG_LIMIT
CVar * gfx_speedo_digital
static const BitMask_t OPTION_i_INVISIBLE
virtual int getNoderef()=0
uint16_t NodeNum_t
Node position within Actor::ar_nodes; use RoR::NODENUM_INVALID as empty value.
static const BitMask64_t SOURCE_BOAT_THROTTLE
CVar * diag_log_beam_break
@ UNLOCKED
lock not locked
const PropAnimFlag_t PROP_ANIM_FLAG_PERMANENT
int ar_airbrake_intensity
Physics state; values 0-5.
static bool isManagedMatAnyhowRemoved(TuneupDefPtr &tuneup_def, const std::string &matname)
static const BitMask_t OPTION_ACCEL
Wrapper for classic c-string (local buffer) Refresher: strlen() excludes '\0' terminator; strncat() A...
node_t * m_fusealge_front
Physics attr; defined in truckfile.
NodeNum_t ar_camera_node_roll[MAX_CAMERAS]
Physics attr; 'camera' = frame of reference; left node.
TransferCase * m_transfer_case
Physics.
Ogre::Vector3 offset
gfx attribute
unsigned int texture_height
static const int DEFAULT_DETACHER_GROUP
std::vector< flare_t > ar_flares
float ti_min_length
Proportional to orig; length.
Ogre::String band_material_name
bool tc_notoggle
Traction control attribute; Disable in-game toggle?
static bool isFlexbodyAnyhowRemoved(TuneupDefPtr &tuneup_def, FlexbodyID_t flexbody_id)
static const BitMask64_t SOURCE_DIFFLOCK
static const BitMask64_t SOURCE_AIR_BRAKE
static const float HOOK_FORCE_DEFAULT
unsigned int AddTyreBeam(RigDef::Wheel2 &wheel_2_def, node_t *node_1, node_t *node_2)
static const BitMask_t OPTION_M_ABSOLUTE_METRIC
std::vector< CabTexcoord > m_oldstyle_cab_texcoords
void ProcessTurboprop2(RigDef::Turboprop2 &def)
wheel_t::BrakeCombo TranslateBrakingDef(RigDef::WheelBraking def)
int m_num_command_beams
TODO: Remove! Spawner context only; likely unused feature.
WheelID_t AddWheel(RigDef::Wheel &wheel)
Gfx attributes/state of a softbody node.
@ FAULTY_FORSET_PLACEHOLDER
@ ALL_VEHICLES_ALL_LIGHTS
All vehicles, all lights.
collcab_rate_t ar_intra_collcabrate[MAX_CABS]
float sbd_damp
set beam default for damping
void ProcessAuthor(RigDef::Author &def)
bool CheckTexcoordLimit(unsigned int count)
std::vector< Rotator2 > rotators2
A database of user-installed content alias 'mods' (vehicles, terrains...)
const PropAnimMode_t PROP_ANIM_MODE_AUTOANIMATE
int trigger_cmdshort
F-key for trigger injection shortbound-check.
float cmb_center_length
Attr computed at spawn.
bool cmb_needs_engine
Attribute defined in truckfile.
Ogre::ParticleSystem * CreateParticleSystem(std::string const &name, std::string const &template_name)
float cc_target_speed
Cruise Control.
Ogre::SceneNode * m_props_parent_scenenode
this isn't used for moving/hiding things, just helps developers inspect the scene graph.
float load_weight_override
void ConfigureAssetPacks(ActorPtr actor, RigDef::DocumentPtr def)
static const BitMask_t OPTION_x_START_DISABLED
Ogre::Light * pp_beacon_light[4]
static const BitMask_t OPTION_a_INPUT_AILERON
void ProcessSpeedLimiter(RigDef::SpeedLimiter &def)
#define BITMASK_IS_1(VAR, FLAGS)
static const BitMask_t MODE_OFFSET_Z
std::string m_help_material_name
int ar_num_custom_particles
std::string vcam_off_tex_name
Used when videocamera is offline.
static const int MAX_CPARTICLES
maximum number of custom particles per actor
PointColDetector * m_inter_point_col_detector
Physics.
int setTorqueModel(Ogre::String name)
Sets the torque model which is used for the vehicle.
RigDef::VideoCamera * video_camera_def
static std::string getTweakedPropMedia(TuneupDefPtr &tuneup_entry, PropID_t prop_id, int media_idx, const std::string &orig_val)
std::shared_ptr< Inertia > inertia_defaults
std::vector< Animator > animators
TyrePressure & getTyrePressure()
const PropAnimFlag_t PROP_ANIM_FLAG_FLAP
static const BitMask_t OPTION_c_CONTACT
Node::Ref alt_orientation_node
const PropAnimFlag_t PROP_ANIM_FLAG_DIFFLOCK
std::vector< Trigger > triggers
void GetWheelAxisNodes(RigDef::BaseWheel &def, node_t *&out_node_1, node_t *&out_node_2)
static const float ROTATOR_TOLERANCE_DEFAULT
CVar * diag_log_beam_trigger
#define BITMASK_SET_1(VAR, FLAGS)
RoR::CmdKeyInertia hb_inertia
int WheelID_t
Index to Actor::ar_wheels, use RoR::WHEELID_INVALID as empty value.
void ProcessFlare3(RigDef::Flare3 &def)
Ogre::String specular_map
const PropAnimFlag_t PROP_ANIM_FLAG_AESTATUS
static const int MAX_SUBMESHES
maximum number of submeshes per actor
float trigger_switch_state
needed to avoid doubleswitch, bool and timer in one
std::vector< RailSegment > rg_segments
static const int LOCKGROUP_NOLOCK
Ogre::Vector3 ar_origin
Physics state; base position for softbody nodes.
const char * ToCStr() const
void ProcessFlexbody(RigDef::Flexbody &def)
static const BitMask_t OPTION_ALTIMETER_10K
@ LOCAL_SLEEPING
sleeping (local) actor
CameraMode_t fb_camera_mode_active
Dynamic visibility mode {0 and higher = cinecam index}.
static const BitMask_t OPTION_STATUS
bool nd_cab_node
Attr; This node is part of collision triangle.
Ogre::Camera * vcam_ogre_camera
float cmb_speed
Attr; Rate of contraction/extension.
float short_bound
Maximum contraction limit, in percentage ( 1.00 = 100% )
@ BEAM_VIRTUAL
Excluded from mass calculations, visuals permanently disabled.
bool nd_no_mouse_grab
Attr; User-defined.
static const int MAX_CAMERAS
maximum number of cameras per actor
Ogre::String rim_mesh_name
static const BitMask_t OPTION_ROLL
int trigger_cmdlong
F-key for trigger injection longbound-check.
void ProcessFixedNode(RigDef::Node::Ref node_ref)
float spring_in
Spring value applied when the shock is compressing.
CmdKeyArray ar_command_key
BEWARE: commandkeys are indexed 1-MAX_COMMANDS!
Ogre::Entity * abx_entity
float precompression
Changes compression or extension of the suspension when the truck spawns. This can be used to "level"...
Collisions * GetCollisions()
static const float HOOK_RANGE_DEFAULT
std::string getTruckFileName()
static const BitMask_t MODE_OFFSET_X
static const BitMask_t OPTION_BOAT_RUDDER
void SetNodes(NodeNum_t front, NodeNum_t back, NodeNum_t ref)
NodeNum_t pos
This node's index in Actor::ar_nodes array.
std::vector< std::string > m_description
bool nd_tyre_node
Attr; This node is part of a tyre (note some wheel types don't use rim nodes at all)
static const BitMask_t MODE_ROTATION_Z
beam_t & GetAndInitFreeBeam(node_t &node_1, node_t &node_2)
void ProcessScrewprop(RigDef::Screwprop &def)
static const BitMask_t OPTION_THROTTLE
static const BitMask_t OPTION_v_INPUT_InvAILERON_ELEVATOR
std::map< std::string, CustomMaterial > m_material_substitutions
Maps original material names (shared) to their actor-specific substitutes; There's 1 substitute per 1...
std::vector< Sample > samples
CVar * diag_log_beam_deform
#define SOUND_START(_ACTOR_, _TRIG_)
static const BitMask64_t SOURCE_STEERING_WHEEL
static const BitMask_t OPTION_TURBO
std::vector< Rotator > rotators
unsigned int AddWheelBeam(node_t *node_1, node_t *node_2, float spring, float damping, std::shared_ptr< RigDef::BeamDefaults > beam_defaults, float max_contraction=-1.f, float max_extension=-1.f, BeamType type=BEAM_NORMAL)
'wheels', 'meshwheels', 'meshwheels2'
const PropAnimFlag_t PROP_ANIM_FLAG_SIGNALSTALK
Turn indicator stalk position (-1=left, 0=off, 1=right)
int ar_collcabs[MAX_CABS]
node_t * wh_near_attach_node
A mesh attached to vehicle frame via 3 nodes.
std::map< Ogre::String, unsigned int > m_named_nodes
std::vector< float > ar_minimass
minimum node mass in Kg
const PropAnimFlag_t PROP_ANIM_FLAG_VVI
float progress_factor_spring_out
Progression factor springout, 0 = disabled, 1...x as multipliers, example:maximum springrate == sprin...
float expansion_trigger_limit
bool nd_loaded_mass
User defined attr; mass is calculated from 'globals/loaded-mass' rather than 'globals/dry-mass'.
NodeNum_t ar_exhaust_dir_node
Old-format exhaust (one per vehicle) backwards direction node.
const PropAnimMode_t PROP_ANIM_MODE_OFFSET_Z
Central state/object manager and communications hub.
float long_bound
Maximum extension limit, in percentage ( 1.00 = 100% )
void ProcessBeam(RigDef::Beam &def)
int getLinkIDForName(Ogre::String &str)
std::pair< unsigned int, bool > AddNode(RigDef::Node::Id &id)
unsigned int AddWheelRimBeam(RigDef::Wheel2 &wheel_2_def, node_t *node_1, node_t *node_2)
@ SHOCK_FLAG_TRG_CONTINUOUS
const PropAnimMode_t PROP_ANIM_MODE_BOUNCE
void ProcessCollisionRange(RigDef::CollisionRange &def)
static const BitMask64_t SOURCE_SHIFT_LEFT_RIGHT
@ CURR_VEHICLE_HEAD_ONLY
Only current vehicle, main lights.
Physics: A vertex in the softbody structure.
float cmb_boundary_length
Attr; Maximum/minimum length proportional to orig. len.
std::vector< float > gear_ratios
std::shared_ptr< Inertia > inertia_defaults
float damp_in_fast
Damping value applied when shock is commpressing faster than split in velocity.
std::string GetCurrentElementMediaRG()
Where to load media from (the addonpart's bundle or vehicle's bundle?)
float global_minimass
'minimass' - used where 'set_default_minimass' is not applied.
void AdjustNodeBuoyancy(node_t &node, RigDef::Node &node_def, std::shared_ptr< RigDef::NodeDefaults > defaults)
For user-defined nodes.
std::vector< Ogre::Entity * > m_deletion_entities
For unloading vehicle; filled at spawn.
GameContext * GetGameContext()
const PropAnimFlag_t PROP_ANIM_FLAG_ACCEL
float max_inclination_angle
void ProcessNode(RigDef::Node &def)
BrakeCombo
Wheels are braked by three mechanisms: A footbrake, a handbrake/parkingbrake, and directional brakes ...
AeroAnimator aero_animator
Ogre::MaterialPtr m_managedmat_placeholder_template
An 'error marker' material (bright magenta) to generate managedmaterial placeholders from.
static const BitMask_t OPTION_s_CMD_NUM_SWITCH
float springout
shocks2 & shocks3
static const BitMask64_t SOURCE_HEADING
DifferentialTypeVec options
Order matters!
std::vector< Shock > shocks
float tc_timer
Traction control state;.
static std::string getTweakedFlexbodyMedia(TuneupDefPtr &tuneup_entry, FlexbodyID_t flexbody_id, int media_idx, const std::string &orig_val)
float progress_factor_spring_in
Progression factor for springin. A value of 0 disables this option. 1...x as multipliers,...
Designed to be run in physics loop (2khz)
@ AIRPLANE
its an airplane
float m_dry_mass
Physics attr;.
void CreateMirrorPropVideoCam(Ogre::MaterialPtr custom_mat, CustomMaterial::MirrorPropType type, Ogre::SceneNode *prop_scenenode)
void ProcessSlidenode(RigDef::SlideNode &def)
int shortbound_trigger_action
void AddBeam(int beam_id)
NodeNum_t vcam_node_lookat
Only for VCTYPE_TRACK_CAM.
static const BitMask_t OPTION_y_INPUT_InvAILERON_RUDDER
std::vector< PropAnimKeyState > m_prop_anim_key_states
static const BitMask64_t SOURCE_PERMANENT
void ProcessRailGroup(RigDef::RailGroup &def)
static const BitMask_t OPTION_s_SUPPORT
static std::string getTweakedManagedMatMediaRG(TuneupDefPtr &tuneup_def, const std::string &matname, int media_idx, const std::string &orig_val)
static float getTweakedWheelRimRadius(TuneupDefPtr &tuneup_entry, WheelID_t wheel_id, float orig_val)
Ogre::SceneNode * m_flares_parent_scenenode
this isn't used for moving/hiding things, just helps developers inspect the scene graph.
bool cmb_is_1press
Attribute defined in truckfile.
bool ar_camera_node_roll_inv[MAX_CAMERAS]
Physics attr; 'camera' = frame of reference; indicates roll node is right instead of left.
const PropAnimFlag_t PROP_ANIM_FLAG_THROTTLE
GfxFlaresMode m_flares_mode
Snapshot of cvar 'gfx_flares_mode' on spawn.
int ar_nodes_name_top_length
For nicely formatted diagnostic output.
@ l_SKIP_LOADED
Only apply minimum mass to nodes without "L" option.
static const BitMask_t OPTION_b_KEY_BLOCKER
static const BitMask_t OPTION_AIRSPEED
Ogre::MaterialPtr mat_instance
An Ogre::Camera mounted on the actor and rendering into either in-scene texture or external window.
static const BitMask_t OPTION_i_INVISIBLE
static const BitMask64_t SOURCE_ALTIMETER_1K
Ogre::SceneNode * abx_scenenode
void SetBeamStrength(beam_t &beam, float strength)
uint16_t hb_beam_index
Index to Actor::ar_beams array.
virtual float getRadius()=0
std::vector< RailGroup * > m_railgroups
all the available RailGroups for this actor
Ogre::SceneNode * GetSceneNode()
static bool CheckSoundScriptLimit(ActorPtr const &vehicle, unsigned int count)
void ProcessDescription(Ogre::String const &line)
std::string m_cab_material_name
Original name defined in truckfile/globals.
void ProcessInterAxle(RigDef::InterAxle &def)
std::shared_ptr< BeamDefaults > defaults
int tr_ax_1
This axle is always driven.
void ProcessParticle(RigDef::Particle &def)
void ProcessCollisionBox(RigDef::CollisionBox &def)
@ VCTYPE_MIRROR_PROP_RIGHT
The classic 'special prop/rear view mirror'.
Ogre::String face_material_name
CacheEntryPtr & getUsedActorEntry()
The actor entry itself.
float parking_brake_force
bool tc_mode
Traction control state; Enabled? {1/0}.
const PropAnimFlag_t PROP_ANIM_FLAG_PITCH
void ProcessTie(RigDef::Tie &def)
void CreateMeshWheelVisuals(WheelID_t wheel_id, NodeNum_t base_node_index, NodeNum_t axis_node_1_index, NodeNum_t axis_node_2_index, unsigned int num_rays, WheelSide side, Ogre::String mesh_name, Ogre::String mesh_rg, Ogre::String material_name, Ogre::String material_rg, float rim_radius)
float option_min_range_meters
const PropAnimFlag_t PROP_ANIM_FLAG_TURBO
void UpdateCollcabContacterNodes()
@ VCTYPE_TRACKING_VIDEOCAM
Ogre::SceneNode * vcam_prop_scenenode
Only for VCTYPE_MIRROR_PROP_*.
Ogre::SceneNode * rod_scenenode
int ar_num_cinecams
Sim attr;.
static const BitMask_t OPTION_h_UNLOCKS_HOOK_GROUP
void SaveFlexbodiesToCache()
static const BitMask_t OPTION_D_CONTACT_BUOYANT
float ar_collision_range
Physics attr.
bool nx_may_get_wet
Attr; enables water drip and vapour.
static const BitMask_t OPTION_t_CONTINUOUS
void SetEngineOptions(float einertia, char etype, float eclutch, float ctime, float stime, float pstime, float irpm, float srpm, float maximix, float minimix, float ebraking)
Sets engine options.
void SetBeamDamping(beam_t &beam, float damping)
static const BitMask64_t SOURCE_GEAR_NEUTRAL
static const BitMask64_t SOURCE_ACCEL
std::vector< Texcoord > texcoords
@ SHOCK_FLAG_TRG_CMD_SWITCH
Ogre::SceneNode * m_curr_mirror_prop_scenenode
bool m_disable_smoke
Stops/starts smoke particles (i.e. exhausts, turbojets).
static const float WHEEL_FRICTION_COEF
std::vector< Cab > cab_triangles
float precompression
Changes compression or extension of the suspension when the truck spawns. This can be used to "level"...
static bool isFlareAnyhowRemoved(TuneupDefPtr &tuneup_def, FlareID_t flare_id)
NodeNum_t vcam_node_alt_pos
std::string uckp_description
std::shared_ptr< BeamDefaults > beam_defaults
const PropAnimFlag_t PROP_ANIM_FLAG_HEADING
bool CheckAxleLimit(unsigned int count)
std::string pp_media[2]
Redundant, for Tuning UI. Media1 = prop mesh name, Media2 = steeringwheel mesh/beaconprop flare mat.
float m_total_mass
Physics state; total mass in Kg.
void enableInducedDrag(float span, float area, bool l)
bool CollectNodesFromRanges(std::vector< RigDef::Node::Range > &node_ranges, std::vector< NodeNum_t > &out_node_indices)
Parses list of node-ranges into list of individual nodes.
static const BitMask_t OPTION_s_BUOYANT_NO_DRAG
static const int MAX_CAMERARAIL
maximum number of camera rail points
static Ogre::Vector3 getTweakedFlexbodyRotation(TuneupDefPtr &tuneup_entry, FlexbodyID_t flexbody_id, Ogre::Vector3 orig_val)
static const float DEFAULT_SPEEDO_MAX_KPH
@ TUNING_REMOVED_PLACEHOLDER
bool CreateNewCurve(Ogre::String const &name=customModel)
Creates new torque curve.
float ar_brake_force
Physics attr; filled at spawn.
#define SOUND_MODULATE(_ACTOR_, _MOD_, _VALUE_)
float global_min_mass_Kg
minimum node mass in Kg - only effective where DefaultMinimass was not set.
bool ar_is_police
Gfx/sfx attr.
bool cc_can_brake
Cruise Control.
float alb_timer
Anti-lock brake state;.
static const float HOOK_LOCK_TIMER_DEFAULT
static const BitMask_t OPTION_s_DISABLE_ON_HIGH_SPEED
bool alb_mode
Anti-lock brake state; Enabled? {1/0}.
void ProcessSoundSource2(RigDef::SoundSource2 &def)
static const BitMask_t OPTION_E_ENGINE_TRIGGER
static const BitMask_t OPTION_y_EXHAUST_DIRECTION
CacheSystem * GetCacheSystem()
void ProcessAntiLockBrakes(RigDef::AntiLockBrakes &def)
CameraMode_t fb_camera_mode_orig
Dynamic visibility mode {0 and higher = cinecam index}.
int m_num_proped_wheels
Physics attr, filled at spawn - Number of propelled wheels.
void BuildWheelObjectAndNodes(WheelID_t wheel_id, unsigned int num_rays, node_t *axis_node_1, node_t *axis_node_2, node_t *reference_arm_node, unsigned int reserve_nodes, unsigned int reserve_beams, float wheel_radius, RigDef::WheelPropulsion propulsion, RigDef::WheelBraking braking, std::shared_ptr< RigDef::NodeDefaults > node_defaults, float wheel_mass, float wheel_width=-1.f)
Sets up wheel and builds nodes for sections 'wheels', 'meshwheels' and 'meshwheels2'.
void ProcessWheel(RigDef::Wheel &def)
static const BitMask64_t SOURCE_TURBO
Node::Ref rotating_plate_nodes[4]
void ProcessCameraRail(RigDef::CameraRail &def)
int controlnumber
Only 'u' type flares, valid values 0-9, maps to EV_TRUCK_LIGHTTOGGLE01 to 10.
Ogre::String material_name
float animOpt3
MULTIPURPOSE.
std::vector< Node::Ref > node_list
static const BitMask_t OPTION_b_EXTRA_BUOYANCY
static void AddSoundSourceInstance(ActorPtr const &vehicle, Ogre::String const &sound_script_name, int node_index, int type=-2)
static const float ROTATOR_FORCE_DEFAULT
UI helper for displaying command control keys to user.
std::vector< PropAnim > pp_animations
std::vector< Shock3 > shocks3
CameraMode_t pp_camera_mode_active
Dynamic visibility mode {0 and higher = cinecam index}.
bool m_has_axles_section
Temporary (legacy parsing helper) until central diffs are implemented.
WheelPropulsion propulsion
int m_airplane_left_light
void RegisterCabMesh(Ogre::Entity *ent, Ogre::SceneNode *snode, FlexObj *flexobj)
void ProcessExhaust(RigDef::Exhaust &def)
static const BitMask_t OPTION_c_NO_GROUND_CONTACT
static const float BEAM_CREAK_DEFAULT
NodeNum_t rod_node2
Node index - may change during simulation!
Ogre::String particle_name
MirrorPropType mirror_prop_type
Ogre::ParticleSystem * psys
static Ogre::Vector3 getTweakedFlexbodyOffset(TuneupDefPtr &tuneup_entry, FlexbodyID_t flexbody_id, Ogre::Vector3 orig_val)
bool nd_rim_node
Attr; This node is part of a rim (only wheel types with separate rim nodes)
std::shared_ptr< BeamDefaults > beam_defaults
static const BitMask_t CONSTRAINT_ATTACH_NONE
static const BitMask_t OPTION_ALTIMETER_100K
static const BitMask_t OPTION_h_INPUT_InvELEVATOR_RUDDER
static std::string getTweakedPropMediaRG(TuneupDefPtr &tuneup_def, PropID_t prop_id, int media_idx, const std::string &orig_val)
CacheEntryPtr m_used_skin_entry
Graphics.
Abstract node ID (numbered or named) Node name is always available.
NodeNum_t vcam_node_dir_z
std::vector< FlexBodyWheel > flexbodywheels
static const BitMask_t OPTION_h_HOOK_POINT
float m_turbo_inertia_factor
void LoadResource(CacheEntryPtr &t)
Loads the associated resource bundle if not already done.
static const int MAX_SOUNDSCRIPTS_PER_TRUCK
maximum number of soundsscripts per actor
static const BitMask_t SOURCE_AERO_THROTTLE
ActorType ar_driveable
Sim attr; marks vehicle type and features.
virtual AeroEngineType getType()=0
node_t * GetNodePointer(RigDef::Node::Ref const &node_ref)
void ProcessProp(RigDef::Prop &def)
Resource group override is used with addonparts.
@ SHOCK_FLAG_TRG_CMD_BLOCKER
bool pp_aero_propeller_blade
Special - single blade mesh.
NodeNum_t ar_exhaust_pos_node
Old-format exhaust (one per vehicle) emitter node.
Differential * m_axle_diffs[1+MAX_WHEELS/2]
Physics.
static const BitMask64_t SOURCE_BRAKES
static bool isExhaustAnyhowRemoved(TuneupDefPtr &tuneup_def, ExhaustID_t exhaust_id)
bool alb_nodash
Anti-lock brake attribute: Hide the dashboard indicator?
PointColDetector * m_intra_point_col_detector
Physics.
float m_odometer_total
GUI state.
float alb_minspeed
Anti-lock brake attribute;.
float contraction_trigger_limit
std::shared_ptr< NodeDefaults > node_defaults
std::vector< rope_t > ar_ropes
static std::string getTweakedWheelMediaRG(TuneupDefPtr &tuneup_def, WheelID_t wheel_id, int media_idx, const std::string &orig_val)
const PropAnimFlag_t PROP_ANIM_FLAG_AEPITCH
float split_vel_in
Split velocity in (m/s) - threshold for slow / fast damping during compression.
const PropAnimFlag_t PROP_ANIM_FLAG_ELEVATORS
@ HYDRO_FLAG_REV_ELEVATOR
void ProcessPistonprop(RigDef::Pistonprop &def)
Ogre::String band_material_name
int FlareID_t
Index into Actor::ar_flares, use RoR::FLAREID_INVALID as empty value.
void CreateWheelVisuals(WheelID_t wheel_id, NodeNum_t node_base_index, unsigned int def_num_rays, Ogre::String const &face_material_name, Ogre::String const &face_material_rg, Ogre::String const &band_material_name, Ogre::String const &band_material_rg, bool separate_rim, float rim_ratio=1.f)
void ProcessTurbojet(RigDef::Turbojet &def)
float dampin
shocks2 & shocks3
int rg_id
Spawn context - matching separately defined rails with slidenodes.
float alb_ratio
Anti-lock brake attribute: Regulating force.
static const BitMask_t OPTION_j_INVISIBLE
float ar_anim_shift_timer
For 'animator' with flag 'shifter'.
static WheelSide getTweakedWheelSide(TuneupDefPtr &tuneup_entry, WheelID_t wheel_id, WheelSide orig_val)
void CalculateBeamLength(beam_t &beam)
float short_bound
Maximum contraction limit, in percentage ( 1.00 = 100% )
Ogre::Vector3 m_spawn_position
const PropAnimFlag_t PROP_ANIM_FLAG_BRUDDER
InputEngine * GetInputEngine()
static float getTweakedWheelTireRadius(TuneupDefPtr &tuneup_entry, WheelID_t wheel_id, float orig_val)
int detacher_group
Attribute: detacher group number (integer)
void ProcessCruiseControl(RigDef::CruiseControl &def)
Submesh for old-style actor body (the "cab")
float progress_factor_damp_in
Progression factor for dampin. 0 = disabled, 1...x as multipliers, example:maximum dampingrate == spr...
std::shared_ptr< Inertia > inertia_defaults
static const BitMask_t OPTION_M_ABSOLUTE_METRIC
ropable_t * ti_locked_ropable
std::shared_ptr< BeamDefaults > beam_defaults
@ SHOCK_FLAG_TRG_HOOK_UNLOCK
static const int LOCKGROUP_DEFAULT
unsigned int forum_account_id
std::vector< Ogre::AxisAlignedBox > ar_predicted_coll_bounding_boxes
RailGroup * CreateRail(std::vector< RigDef::Node::Range > &node_ranges)
static std::string getTweakedManagedMatMedia(TuneupDefPtr &tuneup_def, const std::string &matname, int media_idx, const std::string &orig_val)
static const BitMask_t CONSTRAINT_ATTACH_ALL
std::vector< commandbeam_t > beams
Texture coordinates for old-style actor body (the "cab")
bool nd_no_ground_contact
User-defined attr; node ignores contact with ground.
static const BitMask_t MODE_ROTATION_X
FlexMeshWheel * CreateFlexMeshWheel(unsigned int wheel_index, int axis_node_1_index, int axis_node_2_index, int nstart, int nrays, float rim_radius, bool rim_reverse, std::string const &rim_mesh_name, std::string const &rim_mesh_rg, std::string const &tire_material_name, std::string const &tire_material_rg)
std::vector< Hydro > hydros
static const int MAX_CABS
maximum number of cabs per actor
const PropAnimFlag_t PROP_ANIM_FLAG_SPEEDO
Ogre::SceneNode * m_wheels_parent_scenenode
this isn't used for moving/hiding things, just helps developers inspect the scene graph.
std::list< MotorSource > motor_sources
bool cmb_is_1press_center
Attribute defined in truckfile.
DashboardSpecial special_prop_dashboard
static const BitMask64_t SOURCE_DASHBOARD
Ogre::Vector3 vcam_pos_offset
RigDef::MaterialFlareBinding * material_flare_def
std::string wx_rim_mesh_name
Redundant, for Tuning UI. Only for 'meshwheels[2]' and 'flexbodywheels'.
static const BitMask64_t SOURCE_GEAR_REVERSE
float node_collision_range
ground_model_t * ar_submesh_ground_model
Node::Ref blade_tip_nodes[4]
bool m_apply_simple_materials
static const BitMask_t OPTION_r_ROPE
static const BitMask64_t SOURCE_CLUTCH
Represents an airfoil http://en.wikipedia.org/wiki/Airfoil.
Ogre::SceneNode * smokeNode
float hb_anim_param
Animators (beams updating length based on simulation variables)
static const BitMask_t MODE_OFFSET_Y
Ogre::String airfoil_name
float hb_speed
Rate of change.
static Ogre::Vector3 getTweakedNodePosition(TuneupDefPtr &tuneup_entry, NodeNum_t nodenum, Ogre::Vector3 orig_val)
static const BitMask64_t SOURCE_SIGNALSTALK
MeshObject * pp_mesh_obj
Optional; NULL if removed via tuneup/addonpart.
float sbd_spring
set beam default for spring
static const BitMask_t OPTION_F_10xTOUGHER_BUOYANT
int control_number
Only 'u' type flares.
float damp_in
Damping value applied when the shock is compressing.
std::vector< Node::Ref > nodes
static const BitMask_t OPTION_i_INVISIBLE
void ProcessRotator2(RigDef::Rotator2 &def)
void CreateMaterialFlare(int flare_index, Ogre::MaterialPtr mat)
bool _attachment_rate_set
void CreateFlexBodyWheelVisuals(WheelID_t wheel_id, NodeNum_t node_base_index, NodeNum_t axis_node_1, NodeNum_t axis_node_2, int num_rays, float radius, WheelSide side, std::string rim_mesh_name, std::string rim_mesh_rg, std::string tire_mesh_name, std::string tire_mesh_rg)
Manager for all visuals belonging to a single actor.
void CreateBeamVisuals(beam_t const &beam, int beam_index, bool visible, std::shared_ptr< RigDef::BeamDefaults > const &beam_defaults, std::string material_override="")
static void SetupDefaultSoundSources(ActorPtr const &actor)
bool AssignWheelToAxle(int &_out_axle_wheel, node_t *axis_node_1, node_t *axis_node_2)
Finds wheel with given axle nodes and returns it's index.
std::shared_ptr< BeamDefaults > beam_defaults
bool alb_notoggle
Anti-lock brake attribute: Disable in-game toggle?
CustomMaterial::MirrorPropType m_curr_mirror_prop_type
bool ti_no_self_lock
Attribute.
shock_t * ar_shocks
Shock absorbers.
void BuildAeroEngine(NodeNum_t ref_node_index, NodeNum_t back_node_index, NodeNum_t blade_1_node_index, NodeNum_t blade_2_node_index, NodeNum_t blade_3_node_index, NodeNum_t blade_4_node_index, NodeNum_t couplenode_index, bool is_turboprops, Ogre::String const &airfoil, float power, float pitch)
bool cmb_plays_sound
Attribute defined in truckfile.
@ CONSOLE_MSGTYPE_ACTOR
Parsing/spawn/simulation messages for actors.
std::vector< hydrobeam_t > ar_hydros
unsigned int _SectionWheels2AddBeam(RigDef::Wheel2 &wheel_2_def, node_t *node_1, node_t *node_2)
std::shared_ptr< Document > DocumentPtr
float pp_beacon_rot_rate[4]
Radians per second.
void ProcessCommand(RigDef::Command2 &def)
void setVisible(bool visibility)
static const BitMask_t OPTION_r_BUOYANT_ONLY_DRAG
RoR::CmdKeyInertiaConfig & GetInertiaConfig()
TurbojetVisual tjet_visual
static const int MAX_WHEELS
maximum number of wheels per actor
std::vector< Node::Range > node_list
static const BitMask_t OPTION_m_METRIC
NOTE: Modcache processes this format directly using RoR::GenericDocument, see RoR::CacheSystem::FillA...
static const BitMask_t OPTION_PARKING
WheelSide
Used by rig-def/addonpart/tuneup formats to specify wheel rim mesh orientation.
NodeNum_t GetNodeIndexOrThrow(RigDef::Node::Ref const &id)
ActorMemoryRequirements m_memory_requirements
float pp_wheel_rot_degree
std::shared_ptr< NodeDefaults > node_defaults
std::string particleSystemName
Name in .particle file ~ for display in Tuning UI.
static const BitMask64_t SOURCE_SHIFT_BACK_FORTH
int di_idx_1
array location of wheel / axle 1
static const BitMask_t OPTION_SHORT_LIMIT
int FlexbodyID_t
Index to GfxActor::m_flexbodies, use RoR::FLEXBODYID_INVALID as empty value.
void SetDefaultRail(RailGroup *rail)
Sets rail to initially use when spawned or reset.
static const BitMask_t OPTION_H_LOCKS_HOOK_GROUP
std::string GetSubmeshGroundmodelName()
wheel_t ar_wheels[MAX_WHEELS]
static const BitMask_t OPTION_S_INVULNERABLE_BUOYANT
static const BitMask_t OPTION_i_INVISIBLE
bool alb_pulse_state
Anti-lock brake state;.
int m_airplane_right_light
float damping
The 'resistance to motion' of the shock. The best value is given by this equation: 2 * sqrt(suspended...
NodeNum_t ar_camera_node_pos[MAX_CAMERAS]
Physics attr; 'camera' = frame of reference; origin node.
beam_t & AddBeam(node_t &node_1, node_t &node_2, std::shared_ptr< RigDef::BeamDefaults > &defaults, int detacher_group)
Flexbody = A deformable mesh; updated on CPU every frame, then uploaded to video memory.
void ProcessWheelDetacher(RigDef::WheelDetacher &def)
bool _max_attach_dist_set
std::shared_ptr< BeamDefaults > beam_defaults
void InitBeam(beam_t &beam, node_t *node_1, node_t *node_2)
Node::Ref base_plate_nodes[4]
@ NETWORKED_OK
not simulated (remote) actor
bool m_beam_break_debug_enabled
Logging state.
static const int MAX_SCREWPROPS
maximum number of boat screws per actor
float damp_out
Damping value applied when shock extending.
Ogre::BillboardSet * pp_beacon_bbs[4]
void AssignManagedMaterialTexture(Ogre::TextureUnitState *tus, const std::string &mm_name, int media_id, const std::string &tex_name)
Helper for ProcessManagedMaterial()
bool option_c_auto_center
Ogre::SceneNode * pp_scene_node
The pivot scene node (parented to root-node).
unsigned int spin_left_key
uint16_t cmb_beam_index
Index to Actor::ar_beams array.
bool cmb_is_autocentering
Attribute defined in truckfile.
const PropAnimFlag_t PROP_ANIM_FLAG_CLUTCH
float damp_in_slow
Damping value applied when shock is commpressing slower than split in velocity.
int16_t nd_lockgroup
Optional attribute (-1 = default, 9999 = deny lock) - used in the hook lock logic.
std::vector< ropable_t > ar_ropables
void ProcessFlexBodyWheel(RigDef::FlexBodyWheel &def)
int mode
0 and higher = cinecam index
Ogre::String material_name
static const BitMask_t OPTION_TACHO
beam_t * FindBeamInRig(NodeNum_t node_a, NodeNum_t node_b)
void ProcessTractionControl(RigDef::TractionControl &def)
float m_handbrake_force
Physics attr; defined in truckfile.
node_t * GetBeamNodePointer(RigDef::Node::Ref const &node_ref)
Ogre::MaterialPtr CreateSimpleMaterial(Ogre::ColourValue color)
static std::string getTweakedWheelMedia(TuneupDefPtr &tuneup_entry, WheelID_t wheel_id, int media_idx, const std::string &orig_val)
Ogre::ColourValue emissive_color
static const BitMask64_t SOURCE_TORQUE
const PropAnimFlag_t PROP_ANIM_FLAG_ROLL
Ogre::String stop_function
static const BitMask_t OPTION_s_DISABLE_SELF_LOCK
static const BitMask64_t SOURCE_ALTIMETER_100K
void ProcessTrigger(RigDef::Trigger &def)
std::vector< Beam > beams
std::vector< Airbrake * > ar_airbrakes
Ogre::String material_name
static const BitMask_t OPTION_r_INPUT_RUDDER
static const BitMask_t MODE_NO_FLIP
NodeNum_t ar_camera_rail[MAX_CAMERARAIL]
Nodes defining camera-movement spline.
TuneupDefPtr & getWorkingTuneupDef()
NodeNum_t ar_main_camera_node_roll
Sim attr; ar_camera_node_roll[0] >= 0 ? ar_camera_node_roll[0] : 0.
Node::Ref reference_arm_node
soundsource_t ar_soundsources[MAX_SOUNDSCRIPTS_PER_TRUCK]
std::shared_ptr< DefaultMinimass > default_minimass
int ar_num_shocks
Number of shock absorbers.
Ogre::String dash_link_name
bool ar_guisettings_use_engine_max_rpm
float m_load_mass
Physics attr; predefined load mass in Kg.
std::list< std::shared_ptr< RigDef::Document::Module > > m_selected_modules
std::unique_ptr< GfxActor > m_gfx_actor
bool m_beam_deform_debug_enabled
Logging state.
void setCastShadows(bool b)
void ProcessTransferCase(RigDef::TransferCase &def)
node_t * GetNodePointerOrThrow(RigDef::Node::Ref const &node_ref)
Ogre::Quaternion ar_main_camera_dir_corr
Sim attr;.
CVar * gfx_reduce_shadows
BeaconSpecial special_prop_beacon
const PropAnimFlag_t PROP_ANIM_FLAG_AILERONS
bool ar_has_active_shocks
Are there active stabilizer shocks?
Ogre::SceneNode * pp_beacon_scene_node[4]
float progress_factor_damp_out
Progression factor dampout, 0 = disabled, 1...x as multipliers, example:maximum dampingrate == spring...
ActorManager * GetActorManager()
void ProcessCinecam(RigDef::Cinecam &def)
float ar_guisettings_shifter_anim_time
Ogre::MaterialPtr material
int PropID_t
Index to GfxActor::m_props, use RoR::PROPID_INVALID as empty value.
static const BitMask_t OPTION_i_INVISIBLE
bool ar_minimass_skip_loaded_nodes
std::map< std::string, Ogre::MaterialPtr > ar_managed_materials
static const int MAX_AEROENGINES
maximum number of aero engines per actor
std::shared_ptr< BeamDefaults > beam_defaults
const PropAnimFlag_t PROP_ANIM_FLAG_BTHROTTLE
Ogre::String face_material_name
static const BitMask_t OPTION_b_BUOYANT
const PropAnimFlag_t PROP_ANIM_FLAG_EVENT
void InitNode(node_t &node, Ogre::Vector3 const &position)
static const BitMask_t OPTION_x_INPUT_AILERON_RUDDER
std::vector< MeshWheel > meshwheels
Ogre::String sound_script_name
NodeNum_t ar_cinecam_node[MAX_CAMERAS]
Sim attr; Cine-camera node indexes.
static const int MAX_TEXCOORDS
maximum number of texture coordinates per actor
void ProcessManagedMaterial(RigDef::ManagedMaterial &def)
std::unique_ptr< Buoyance > m_buoyance
Physics.
const PropAnimMode_t PROP_ANIM_MODE_OFFSET_Y
const PropAnimFlag_t PROP_ANIM_FLAG_TACHO
float damp_out_slow
Damping value applied when shock is commpressing slower than split out velocity.
Ogre::String flare_material_name
void SetupVisuals(RigDef::Turbojet &def, int num, std::string const &propname, Ogre::Entity *nozzle, Ogre::Entity *afterburner_flame)
void ProcessWheel2(RigDef::Wheel2 &def)
std::vector< Node::Ref > fixes
SimpleInertia inertia
Only 'flares3'.
float ar_guisettings_speedo_max_kph
std::list< Animation > animations
std::vector< CabSubmesh > m_oldstyle_cab_submeshes
const TerrainPtr & GetTerrain()
Ogre::String fname
filename
static const BitMask_t OPTION_f_NO_SPARKS
static const BitMask_t OPTION_VERTICAL_VELOCITY
static const BitMask_t OPTION_GEAR_SELECT
static const BitMask64_t SOURCE_SEQUENTIAL_SHIFT
FlexBody * CreateFlexBody(FlexbodyID_t flexbody_id, const NodeNum_t ref_node, const NodeNum_t x_node, const NodeNum_t y_node, Ogre::Vector3 offset, Ogre::Vector3 rotation, std::vector< unsigned int > &node_indices, const std::string &mesh_name, const std::string &resource_group_name)
int SetCmdKeyDelay(RoR::CmdKeyInertiaConfig &cfg, float start_delay, float stop_delay, std::string start_function, std::string stop_function)
int pp_aero_engine_idx
Special - a turboprop/pistonprop reference.
static const BitMask64_t SOURCE_SPEEDO
bool CheckScrewpropLimit(unsigned int count)