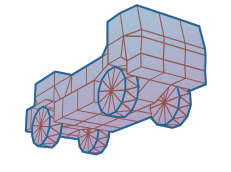 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
37 #include <OgreMeshManager.h>
38 #include <OgreSceneManager.h>
39 #include <MeshLodGenerator/OgreMeshLodGenerator.h>
43 #ifdef FLEXFACTORY_DEBUG_LOGGING
44 # include "RoRPrerequisites.h"
45 # define FLEX_DEBUG_LOG(TEXT) LOG("FlexFactory | " TEXT)
47 # define FLEX_DEBUG_LOG(TEXT)
48 #endif // FLEXFACTORY_DEBUG_LOGGING
56 m_rig_spawner(rig_spawner),
57 m_is_flexbody_cache_loaded(false),
59 m_flexbody_cache_next_index(0)
69 Ogre::Vector3 rotation,
70 std::vector<unsigned int> & node_indices,
71 const std::string& mesh_name,
72 const std::string& resource_group_name)
74 Ogre::MeshPtr common_mesh = Ogre::MeshManager::getSingleton().load(mesh_name, resource_group_name);
76 Ogre::MeshPtr mesh = common_mesh->clone(mesh_unique_name);
90 Ogre::Quaternion rot=Ogre::Quaternion(Ogre::Degree(rotation.z), Ogre::Vector3::UNIT_Z);
91 rot=rot*Ogre::Quaternion(Ogre::Degree(rotation.y), Ogre::Vector3::UNIT_Y);
92 rot=rot*Ogre::Quaternion(Ogre::Degree(rotation.x), Ogre::Vector3::UNIT_X);
109 new_flexbody->
m_id = flexbody_id;
115 unsigned int wheel_index,
116 int axis_node_1_index,
117 int axis_node_2_index,
122 std::string
const & rim_mesh_name,
123 std::string
const & rim_mesh_rg,
124 std::string
const & tire_material_name,
125 std::string
const & tire_material_rg)
141 tire_material_name, tire_material_rg, rim_radius, rim_reverse);
150 return flex_mesh_wheel;
155 size_t num_written = fwrite(source, length, 1,
m_file);
156 if (num_written != 1)
165 size_t num_written = fread(dest, length, 1,
m_file);
166 if (num_written != 1)
260 data->
src_normals=(Ogre::Vector3*)malloc(
sizeof(Ogre::Vector3) * vertex_count);
276 data->
dst_pos=(Ogre::Vector3*)malloc(
sizeof(Ogre::Vector3) * vertex_count);
299 data->
src_colors=(Ogre::ARGB*)malloc(
sizeof(Ogre::ARGB) * vertex_count);
313 m_file = fopen(path, fopen_mode);
337 for (; itor != end; ++itor)
403 m_fileformat_version(0),
404 m_cache_entry_number(-1)
#define ROR_ASSERT(_EXPR)
unsigned int num_flexbodies
FlexBodyRecordHeader header
@ RESULT_CODE_FREAD_OUTPUT_INCOMPLETE
void WriteFlexbodyColorsBuffer(FlexBody *flexbody)
void OpenFile(const char *fopen_mode)
static const BitMask_t HAS_TEXTURE
bool m_uses_shared_vertex_data
void ReadFlexbodyHeader(FlexBodyCacheData *flexbody)
void CheckAndLoadFlexbodyCache()
static const BitMask_t USES_SHARED_VERTEX_DATA
std::string m_orig_mesh_name
std::string ComposeName(const std::string &object, int number=-1)
Creates name containing actor ID token, i.e. "Object#1 (filename.truck [Instance ID 1])".
FlexBodyCacheData * GetLoadedItem(unsigned index)
Truck file format(technical spec)
Consists of static mesh, representing the rim, and dynamic mesh, representing the tire.
CVar * gfx_flexbody_cache
static const BitMask_t HAS_TEXTURE_BLEND
void ReadFlexbodyLocatorList(FlexBodyCacheData *flexbody)
Ogre::Entity * m_tire_entity
Ogre::Vector3 * m_dst_pos
ActorSpawner * m_rig_spawner
static const BitMask_t IS_FAULTY
bool m_is_flexbody_cache_loaded
Ogre::SceneManager * GetSceneManager()
Processes a RigDef::Document (parsed from 'truck' file format) into a simulated gameplay object (Acto...
void ReadFlexbodyNormalsBuffer(FlexBodyCacheData *flexbody)
unsigned int m_flexbody_cache_next_index
FlexBodyFileIO m_flexbody_cache
void SetupNewEntity(Ogre::Entity *e, Ogre::ColourValue simple_color)
Full texture and material setup.
#define BITMASK_IS_0(VAR, FLAGS)
int m_camera_mode
Visibility control {-2 = always, -1 = 3rdPerson only, 0+ = cinecam index}.
std::vector< FlexBody * > m_items_to_save
@ RESULT_CODE_ERR_FOPEN_FAILED
static const char * SIGNATURE
uint16_t NodeNum_t
Node position within Actor::ar_nodes; use RoR::NODENUM_INVALID as empty value.
A database of user-installed content alias 'mods' (vehicles, terrains...)
void WriteToFile(void *source, size_t length)
Ogre::ARGB * m_src_colors
int m_shared_buf_num_verts
#define BITMASK_SET_1(VAR, FLAGS)
Ogre::Vector3 center_offset
void WriteFlexbodyHeader(FlexBody *flexbody)
Central state/object manager and communications hub.
void ReadAndCheckSignature()
@ RESULT_CODE_ERR_SIGNATURE_MISMATCH
void ReadFromFile(void *dest, size_t length)
Ogre::Vector3 * src_normals
std::vector< FlexBodyCacheData > m_loaded_items
void SaveFlexbodiesToCache()
bool m_is_flexbody_cache_enabled
void ReadFlexbodyPositionsBuffer(FlexBodyCacheData *flexbody)
void WriteFlexbodyLocatorList(FlexBody *flexbody)
@ RESULT_CODE_ERR_VERSION_MISMATCH
unsigned int m_fileformat_version
void ReadFlexbodyColorsBuffer(FlexBodyCacheData *flexbody)
Locator_t * m_locators
1 loc per vertex
FlexMeshWheel * CreateFlexMeshWheel(unsigned int wheel_index, int axis_node_1_index, int axis_node_2_index, int nstart, int nrays, float rim_radius, bool rim_reverse, std::string const &rim_mesh_name, std::string const &rim_mesh_rg, std::string const &tire_material_name, std::string const &tire_material_rg)
Ogre::SceneNode * m_wheels_parent_scenenode
this isn't used for moving/hiding things, just helps developers inspect the scene graph.
Ogre::Vector3 m_center_offset
Ogre::Vector3 * m_src_normals
void ReadMetadata(FlexBodyFileMetadata *meta)
void AddItemToSave(FlexBody *fb)
int FlexbodyID_t
Index to GfxActor::m_flexbodies, use RoR::FLEXBODYID_INVALID as empty value.
void WriteFlexbodyPositionsBuffer(FlexBody *flexbody)
Flexbody = A deformable mesh; updated on CPU every frame, then uploaded to video memory.
Locator_t * locators
1 loc per vertex
static const unsigned int FILE_FORMAT_VERSION
unsigned int file_format_version
Ogre::String GetResourceGroup()
Data structures representing 'truck' file format, see https://docs.rigsofrods.org/vehicle-creation/fi...
@ RESULT_CODE_ERR_CACHE_NUMBER_UNDEFINED
void WriteFlexbodyNormalsBuffer(FlexBody *flexbody)
#define FLEX_DEBUG_LOG(TEXT)
@ RESULT_CODE_FWRITE_OUTPUT_INCOMPLETE
FlexBody * CreateFlexBody(FlexbodyID_t flexbody_id, const NodeNum_t ref_node, const NodeNum_t x_node, const NodeNum_t y_node, Ogre::Vector3 offset, Ogre::Vector3 rotation, std::vector< unsigned int > &node_indices, const std::string &mesh_name, const std::string &resource_group_name)