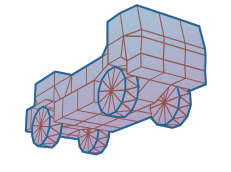 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
35 #include <OgreVector3.h>
36 #include <OgreColourValue.h>
117 std::vector<FlexBody*> &
GetList();
130 void OpenFile(
const char* fopen_mode);
175 Ogre::Vector3 offset,
176 Ogre::Vector3 rotation,
177 std::vector<unsigned int> & node_indices,
178 const std::string& mesh_name,
179 const std::string& resource_group_name);
182 unsigned int wheel_index,
183 int axis_node_1_index,
184 int axis_node_2_index,
189 std::string
const & rim_mesh_name,
190 std::string
const & rim_mesh_rg,
191 std::string
const & tire_material_name,
192 std::string
const & tire_material_rg);
unsigned int num_flexbodies
FlexBodyRecordHeader header
Global forward declarations.
@ RESULT_CODE_FREAD_OUTPUT_INCOMPLETE
void WriteFlexbodyColorsBuffer(FlexBody *flexbody)
void OpenFile(const char *fopen_mode)
static const BitMask_t HAS_TEXTURE
void ReadFlexbodyHeader(FlexBodyCacheData *flexbody)
void CheckAndLoadFlexbodyCache()
static const BitMask_t USES_SHARED_VERTEX_DATA
FlexBodyCacheData * GetLoadedItem(unsigned index)
Consists of static mesh, representing the rim, and dynamic mesh, representing the tire.
static const BitMask_t HAS_TEXTURE_BLEND
void ReadFlexbodyLocatorList(FlexBodyCacheData *flexbody)
ActorSpawner * m_rig_spawner
static const BitMask_t IS_FAULTY
bool m_is_flexbody_cache_loaded
Processes a RigDef::Document (parsed from 'truck' file format) into a simulated gameplay object (Acto...
void ReadFlexbodyNormalsBuffer(FlexBodyCacheData *flexbody)
unsigned int m_flexbody_cache_next_index
FlexBodyFileIO m_flexbody_cache
std::vector< FlexBody * > m_items_to_save
@ RESULT_CODE_ERR_FOPEN_FAILED
static const char * SIGNATURE
uint16_t NodeNum_t
Node position within Actor::ar_nodes; use RoR::NODENUM_INVALID as empty value.
void WriteToFile(void *source, size_t length)
@ RESULT_CODE_GENERAL_ERROR
Ogre::Vector3 center_offset
void WriteFlexbodyHeader(FlexBody *flexbody)
void ReadAndCheckSignature()
@ RESULT_CODE_ERR_SIGNATURE_MISMATCH
void ReadFromFile(void *dest, size_t length)
Ogre::Vector3 * src_normals
std::vector< FlexBodyCacheData > m_loaded_items
void SaveFlexbodiesToCache()
bool m_is_flexbody_cache_enabled
void ReadFlexbodyPositionsBuffer(FlexBodyCacheData *flexbody)
void WriteFlexbodyLocatorList(FlexBody *flexbody)
@ RESULT_CODE_ERR_VERSION_MISMATCH
unsigned int m_fileformat_version
void ReadFlexbodyColorsBuffer(FlexBodyCacheData *flexbody)
FlexMeshWheel * CreateFlexMeshWheel(unsigned int wheel_index, int axis_node_1_index, int axis_node_2_index, int nstart, int nrays, float rim_radius, bool rim_reverse, std::string const &rim_mesh_name, std::string const &rim_mesh_rg, std::string const &tire_material_name, std::string const &tire_material_rg)
void ReadMetadata(FlexBodyFileMetadata *meta)
void AddItemToSave(FlexBody *fb)
std::vector< FlexBody * > & GetList()
int FlexbodyID_t
Index to GfxActor::m_flexbodies, use RoR::FLEXBODYID_INVALID as empty value.
void WriteFlexbodyPositionsBuffer(FlexBody *flexbody)
Flexbody = A deformable mesh; updated on CPU every frame, then uploaded to video memory.
Locator_t * locators
1 loc per vertex
static const unsigned int FILE_FORMAT_VERSION
unsigned int file_format_version
@ RESULT_CODE_ERR_CACHE_NUMBER_UNDEFINED
Enables saving and loading flexbodies from/to binary file.
void WriteFlexbodyNormalsBuffer(FlexBody *flexbody)
@ RESULT_CODE_FWRITE_OUTPUT_INCOMPLETE
FlexBody * CreateFlexBody(FlexbodyID_t flexbody_id, const NodeNum_t ref_node, const NodeNum_t x_node, const NodeNum_t y_node, Ogre::Vector3 offset, Ogre::Vector3 rotation, std::vector< unsigned int > &node_indices, const std::string &mesh_name, const std::string &resource_group_name)