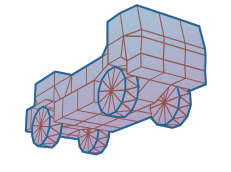 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
33 #include <OgreVector3.h>
53 Id(
unsigned int id_num);
54 Id(std::string
const & id_str);
57 void SetNum(
unsigned int id_num);
58 void setStr(std::string
const & id_str);
91 Ref(std::string
const & id_str,
unsigned int id_num,
unsigned flags,
unsigned line_number_defined);
94 inline std::string
const &
Str()
const {
return m_id; }
101 inline bool IsValidAnyState()
const {
return GetImportState_IsValid() || GetRegularState_IsValid(); }
BITMASK_PROPERTY_GET(m_flags, 1, IS_VALID, IsValid)
static const BitMask_t OPTION_x_EXHAUST_POINT
Legacy parser resolved references on-the-fly and the condition to check named nodes was "are there an...
Range(Node::Ref const &single)
std::shared_ptr< BeamDefaults > beam_defaults
bool operator!=(Ref const &rhs) const
const std::string & Str() const
std::string ToString() const
void SetNum(unsigned int id_num)
void SetSingle(Node::Ref const &ref)
static const BitMask_t OPTION_p_NO_PARTICLES
unsigned GetLineNumber() const
BITMASK_PROPERTY_GET(m_flags, 1, IMPORT_STATE_IS_VALID, GetImportState_IsValid)
bool IsValidAnyState() const
static const BitMask_t OPTION_m_NO_MOUSE_GRAB
static const BitMask_t OPTION_l_LOAD_WEIGHT
bool _has_load_weight_override
float load_weight_override
const std::string & Str() const
static const BitMask_t OPTION_L_LOG
unsigned int m_line_number
bool operator==(Ref const &rhs) const
static const BitMask_t OPTION_y_EXHAUST_DIRECTION
static const BitMask_t OPTION_b_EXTRA_BUOYANCY
static const BitMask_t OPTION_c_NO_GROUND_CONTACT
Abstract node ID (numbered or named) Node name is always available.
static const BitMask_t OPTION_h_HOOK_POINT
std::shared_ptr< NodeDefaults > node_defaults
void setStr(std::string const &id_str)
unsigned int m_id_as_number
static const BitMask_t OPTION_e_TERRAIN_EDIT_POINT
std::string ToString() const
Range(Node::Ref const &start, Node::Ref const &end)
std::shared_ptr< DefaultMinimass > default_minimass
static const BitMask_t OPTION_f_NO_SPARKS
bool Compare(Ref const &rhs) const