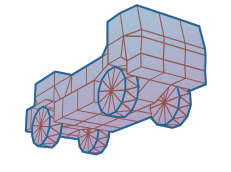 |
RigsofRods
2023.09
Soft-body Physics Simulation
|
Go to the documentation of this file.
30 #include <OgreEntity.h>
31 #include <OgreMaterialManager.h>
33 #include <OgreSubEntity.h>
34 #include <OgreTechnique.h>
96 out_val = itor->second;
110 out_val = itor->second;
242 out_tweak = &itor->second;
285 out_tweak = &itor->second;
300 out_tweak = &itor->second;
393 out_tweak = &itor->second;
484 out_tweak = &itor->second;
520 out_tweak = &itor->second;
574 LOG(
fmt::format(
"[RoR|Tuneup] WARN managedmaterial '{}': Addonpart '{}' not found in modcache!", material_name, tweak->
tmt_origin));
591 std::vector<TuneupDefPtr> result;
595 while(!stream->eof())
600 if (!line.length() || line.substr(0, 2) ==
"//")
608 Ogre::StringUtil::trim(line);
610 curr_tuneup->
name = line;
611 stream->skipLine(
"{");
618 result.push_back(curr_tuneup);
619 curr_tuneup =
nullptr;
632 fmt::format(
"Tuneup '{}' in file '{}' not properly closed with '}}'",
633 curr_tuneup->
name, stream->getName()));
634 result.push_back(curr_tuneup);
635 curr_tuneup =
nullptr;
638 catch (Ogre::Exception& e)
642 fmt::format(
"Error parsing tuneup file '{}', message: {}",
643 stream->getName(), e.getFullDescription()));
650 Ogre::StringVector params = Ogre::StringUtil::split(line,
"\t=,;\n");
651 for (
unsigned int i=0; i < params.size(); i++)
653 Ogre::StringUtil::trim(params[i]);
655 Ogre::String& attrib = params[0];
656 Ogre::StringUtil::toLowerCase(attrib);
659 if (attrib ==
"preview" && params.size() >= 2) { tuneup_def->
thumbnail = params[1];
return; }
660 if (attrib ==
"description" && params.size() >= 2) { tuneup_def->
description = params[1];
return; }
661 if (attrib ==
"author_name" && params.size() >= 2) { tuneup_def->
author_name = params[1];
return; }
662 if (attrib ==
"author_id" && params.size() == 2) { tuneup_def->
author_id =
PARSEINT(params[1]);
return; }
664 if (attrib ==
"guid" && params.size() >= 2) { tuneup_def->
guid = params[1]; Ogre::StringUtil::trim(tuneup_def->
guid); Ogre::StringUtil::toLowerCase(tuneup_def->
guid);
return; }
665 if (attrib ==
"name" && params.size() >= 2) { tuneup_def->
name = params[1]; Ogre::StringUtil::trim(tuneup_def->
name);
return; }
666 if (attrib ==
"filename" && params.size() >= 2) { tuneup_def->
filename = params[1]; Ogre::StringUtil::trim(tuneup_def->
filename);
return; }
669 if (attrib ==
"use_addonpart" && params.size() == 2) { tuneup_def->
use_addonparts.insert(params[1]);
return; }
670 if (attrib ==
"unwanted_prop" && params.size() == 2) { tuneup_def->
unwanted_props.insert(
PARSEINT(params[1]));
return; }
672 if (attrib ==
"protected_prop" && params.size() == 2) { tuneup_def->
protected_props.insert(
PARSEINT(params[1]));
return; }
677 if (attrib ==
"force_remove_prop" && params.size() == 2) { tuneup_def->
force_remove_props.insert(
PARSEINT(params[1]));
return; }
684 buf << tuneup->
name <<
"\n";
688 buf <<
"\tpreview = " << tuneup->
thumbnail <<
"\n";
689 buf <<
"\tdescription = " << tuneup->
description <<
"\n";
690 buf <<
"\tauthor_name = " << tuneup->
author_name <<
"\n";
691 buf <<
"\tauthor_id = " << tuneup->
author_id <<
"\n";
692 buf <<
"\tcategory_id = " << (int)tuneup->
category_id <<
"\n";
693 buf <<
"\tguid = " << tuneup->
guid <<
"\n";
694 buf <<
"\tfilename = " << tuneup->
filename <<
"\n";
700 buf <<
"\tuse_addonpart = " << addonpart <<
"\n";
704 buf <<
"\tunwanted_prop = " << (int)unwanted_prop <<
"\n";
708 buf <<
"\tunwanted_flexbody = " << (int)unwanted_flexbody <<
"\n";
712 buf <<
"\tprotected_prop = " << (int)protected_prop <<
"\n";
716 buf <<
"\tprotected_flexbody = " << (int)protected_flexbody <<
"\n";
722 buf <<
"\tforce_remove_prop = " << (int)prop <<
"\n";
726 buf <<
"\tforce_remove_flexbody = " << (int)flexbody <<
"\n";
730 buf <<
"\tforced_wheel_side = " << pair.first <<
", " << (int)pair.second <<
"\n";
738 fmt::format(
"Error writing file '{}': only written {}/{} bytes", stream->getName(), written, buf.
GetLength()));
#define ROR_ASSERT(_EXPR)
bool isFlexbodyForceRemoved(FlexbodyID_t flexbodyid)
WheelSide
Used by rig-def/addonpart/tuneup formats to specify wheel rim mesh orientation.
std::set< FlexbodyID_t > force_remove_flexbodies
UI overrides.
std::string twt_origin
Addonpart filename.
static bool isAddonPartUsed(TuneupDefPtr &tuneup_entry, const std::string &filename)
std::set< FlareID_t > protected_flares
Flares which cannot be altered via 'addonpart_unwanted_flare' directive.
static std::vector< TuneupDefPtr > ParseTuneups(Ogre::DataStreamPtr &stream)
static Ogre::Vector3 getTweakedCineCameraPosition(TuneupDefPtr &tuneup_entry, CineCameraID_t cinecamid, Ogre::Vector3 orig_val)
static bool isWheelTweaked(TuneupDefPtr &tuneup_entry, WheelID_t wheel_id, TuneupWheelTweak *&out_tweak)
float twt_tire_radius
Arg#5, optional.
std::set< PropID_t > force_remove_props
UI overrides.
std::set< PropID_t > unwanted_props
'addonpart_unwanted_prop' directives.
< Data of 'addonpart_tweak_prop <prop ID> <offsetX> <offsetY> <offsetZ> <rotX> <rotY> <rotZ> <media1>...
float twt_rim_radius
Arg#6, optional, only applies to some wheel types.
Ogre::Vector3 tft_rotation
int CineCameraID_t
Index into Actor::ar_cinecam_node and Actor::ar_camera_node_* arrays; use RoR::CINECAMERAID_INVALID a...
static bool isPropTweaked(TuneupDefPtr &tuneup_entry, PropID_t flexbody_id, TuneupPropTweak *&out_tweak)
std::string tft_origin
Addonpart filename.
std::string tmt_type
Arg#2, required.
static bool isPropAnyhowRemoved(TuneupDefPtr &tuneup_def, PropID_t prop_id)
std::map< PropID_t, TuneupPropTweak > prop_tweaks
Mesh name(s), offset and rotation overrides via 'addonpart_tweak_prop'.
Truck file format(technical spec)
std::set< FlexbodyID_t > unwanted_flexbodies
'addonpart_unwanted_flexbody' directives.
std::string SanitizeUtf8String(std::string const &str_in)
static void ExportTuneup(Ogre::DataStreamPtr &stream, TuneupDefPtr &tuneup)
void putMessage(MessageArea area, MessageType type, std::string const &msg, std::string icon="")
std::set< NodeNum_t > protected_nodes
Nodes that cannot be altered via 'addonpart_tweak_node'.
Ogre::String resource_group
Resource group of the loaded bundle. Empty if not loaded yet.
std::set< std::string > use_addonparts
Addonpart filenames.
bool isExhaustUnwanted(ExhaustID_t exhaustid)
bool isPropUnwanted(PropID_t propid)
static Ogre::Vector3 getTweakedPropOffset(TuneupDefPtr &tuneup_entry, PropID_t prop_id, Ogre::Vector3 orig_val)
static Ogre::Vector3 getTweakedPropRotation(TuneupDefPtr &tuneup_entry, PropID_t prop_id, Ogre::Vector3 orig_val)
Ogre::Vector3 tpt_rotation
int ExhaustID_t
Index into GfxActor::m_exhausts, use RoR::EXHAUSTID_INVALID as empty value.
std::set< PropID_t > protected_props
Props which cannot be altered via 'addonpart_tweak_prop' or 'addonpart_unwanted_prop' directive.
static bool isNodeTweaked(TuneupDefPtr &tuneup_entry, NodeNum_t nodenum, TuneupNodeTweak *&out_tweak)
bool isVideoCameraRoleForced(VideoCameraID_t camera_id, VideoCamRole &out_val) const
static std::string getTweakedFlexbodyMediaRG(TuneupDefPtr &tuneup_def, FlexbodyID_t flexbody_id, int media_idx, const std::string &orig_val)
< Data of 'addonpart_tweak_cinecam <cinecam ID> <posX> <posY> <posZ>'
std::map< std::string, TuneupManagedMatTweak > managedmat_tweaks
Managed material overrides via 'addonpart_tweak_managedmaterial'.
std::map< NodeNum_t, TuneupNodeTweak > node_tweaks
Node position overrides via 'addonpart_tweak_node'.
< Data of 'addonpart_tweak_managedmaterial <name> <type> <media1> <media2> [<media3>]'
int VideoCameraID_t
Index into GfxActor::m_videocameras, use RoR::VIDEOCAMERAID_INVALID as empty value.
std::string tmt_origin
Addonpart filename.
uint16_t NodeNum_t
Node position within Actor::ar_nodes; use RoR::NODENUM_INVALID as empty value.
static bool isManagedMatAnyhowRemoved(TuneupDefPtr &tuneup_def, const std::string &matname)
Wrapper for classic c-string (local buffer) Refresher: strlen() excludes '\0' terminator; strncat() A...
CacheEntryPtr FindEntryByFilename(RoR::LoaderType type, bool partial, const std::string &_filename_maybe_bundlequalified)
Returns NULL if none found; "Bundle-qualified" format also specifies the ZIP/directory in modcache,...
static bool isFlexbodyAnyhowRemoved(TuneupDefPtr &tuneup_def, FlexbodyID_t flexbody_id)
bool isManagedMatForceRemoved(const std::string &matname)
< Data of 'addonpart_tweak_node <nodenum> <posX> <posY> <posZ>'
A database of user-installed content alias 'mods' (vehicles, terrains...)
std::set< WheelID_t > protected_wheels
Wheels that cannot be altered via 'addonpart_tweak_wheel'.
WheelSide twt_side
Arg#4, optional, default LEFT (Only applicable to mesh/flexbody wheels)
static std::string getTweakedPropMedia(TuneupDefPtr &tuneup_entry, PropID_t prop_id, int media_idx, const std::string &orig_val)
std::map< FlexbodyID_t, TuneupFlexbodyTweak > flexbody_tweaks
Mesh name, offset and rotation overrides via 'addonpart_tweak_flexbody'.
int WheelID_t
Index to Actor::ar_wheels, use RoR::WHEELID_INVALID as empty value.
Ogre::Vector3 tnt_pos
Args#234, required.
std::set< std::string > protected_managedmats
Managed materials which cannot be altered via 'addonpart_tweak_managedmaterial' directive.
bool isFlareUnwanted(FlareID_t flareid)
Central state/object manager and communications hub.
std::string tpt_origin
Addonpart filename.
static bool isCineCameraTweaked(TuneupDefPtr &tuneup_entry, CineCameraID_t cinecamid, TuneupCineCameraTweak *&out_tweak)
static std::string getTweakedFlexbodyMedia(TuneupDefPtr &tuneup_entry, FlexbodyID_t flexbody_id, int media_idx, const std::string &orig_val)
static std::string getTweakedManagedMatMediaRG(TuneupDefPtr &tuneup_def, const std::string &matname, int media_idx, const std::string &orig_val)
static float getTweakedWheelRimRadius(TuneupDefPtr &tuneup_entry, WheelID_t wheel_id, float orig_val)
bool isFlareForceRemoved(FlareID_t flareid)
static bool isFlareAnyhowRemoved(TuneupDefPtr &tuneup_def, FlareID_t flare_id)
static Ogre::Vector3 getTweakedFlexbodyRotation(TuneupDefPtr &tuneup_entry, FlexbodyID_t flexbody_id, Ogre::Vector3 orig_val)
std::array< std::string, 2 > twt_media
twt_media[0] Arg#2, required ('wheels[2]': face material, 'meshwheels[2]/flexbodywheels': rim mesh) t...
CacheSystem * GetCacheSystem()
std::array< std::string, 2 > tpt_media
Media1 = prop mesh; Media2: Steering wheel mesh or beacon flare material.
std::map< VideoCameraID_t, VideoCamRole > force_video_cam_roles
UI overrides.
std::set< ExhaustID_t > protected_exhausts
Exhausts which cannot be altered via 'addonpart_unwanted_exhaust' directive.
static Ogre::Vector3 getTweakedFlexbodyOffset(TuneupDefPtr &tuneup_entry, FlexbodyID_t flexbody_id, Ogre::Vector3 orig_val)
std::set< FlexbodyID_t > protected_flexbodies
Flexbodies which cannot be altered via 'addonpart_tweak_flexbody' or 'addonpart_unwanted_flexbody' di...
static std::string getTweakedPropMediaRG(TuneupDefPtr &tuneup_def, PropID_t prop_id, int media_idx, const std::string &orig_val)
static std::string getTweakedManagedMatType(TuneupDefPtr &tuneup_def, const std::string &matname, const std::string &orig_val)
std::map< CineCameraID_t, TuneupCineCameraTweak > cinecam_tweaks
Cinecam position overrides via 'addonpart_tweak_cinecam'.
std::string filename
target vehicle filename
static bool isExhaustAnyhowRemoved(TuneupDefPtr &tuneup_def, ExhaustID_t exhaust_id)
static bool isManagedMatTweaked(TuneupDefPtr &tuneup_def, const std::string &matname, TuneupManagedMatTweak *&out_tweak)
static std::string getTweakedWheelMediaRG(TuneupDefPtr &tuneup_def, WheelID_t wheel_id, int media_idx, const std::string &orig_val)
int FlareID_t
Index into Actor::ar_flares, use RoR::FLAREID_INVALID as empty value.
static WheelSide getTweakedWheelSide(TuneupDefPtr &tuneup_entry, WheelID_t wheel_id, WheelSide orig_val)
static float getTweakedWheelTireRadius(TuneupDefPtr &tuneup_entry, WheelID_t wheel_id, float orig_val)
bool isExhaustForceRemoved(ExhaustID_t exhaustid)
static std::string getTweakedManagedMatMedia(TuneupDefPtr &tuneup_def, const std::string &matname, int media_idx, const std::string &orig_val)
CacheCategoryId category_id
< Data of 'addonpart_tweak_wheel <wheel ID> <media1> <media2> <side flag> <tire radius> <rim radius>'
static Ogre::Vector3 getTweakedNodePosition(TuneupDefPtr &tuneup_entry, NodeNum_t nodenum, Ogre::Vector3 orig_val)
@ CONSOLE_MSGTYPE_ACTOR
Parsing/spawn/simulation messages for actors.
std::map< WheelID_t, WheelSide > force_wheel_sides
UI overrides.
bool isFlexbodyUnwanted(FlexbodyID_t flexbodyid)
@ CONSOLE_MSGTYPE_INFO
Generic message.
int FlexbodyID_t
Index to GfxActor::m_flexbodies, use RoR::FLEXBODYID_INVALID as empty value.
bool isWheelSideForced(WheelID_t wheelid, WheelSide &out_val) const
static bool isFlexbodyTweaked(TuneupDefPtr &tuneup_entry, FlexbodyID_t flexbody_id, TuneupFlexbodyTweak *&out_tweak)
std::string guid
target vehicle GUID
static void ParseTuneupAttribute(const std::string &line, TuneupDefPtr &tuneup_def)
< Data of 'addonpart_tweak_flexbody <flexbody ID> <offsetX> <offsetY> <offsetZ> <rotX> <rotY> <rotZ> ...
static std::string getTweakedWheelMedia(TuneupDefPtr &tuneup_entry, WheelID_t wheel_id, int media_idx, const std::string &orig_val)
bool isPropForceRemoved(PropID_t propid)
static VideoCamRole getTweakedVideoCameraRole(TuneupDefPtr &tuneup_def, VideoCameraID_t camera_id, VideoCamRole orig_val)
std::array< std::string, 3 > tmt_media
Arg#3, required, Arg#4, optional, Arg#5, optional.
std::map< WheelID_t, TuneupWheelTweak > wheel_tweaks
Mesh name and radius overrides via 'addonpart_tweak_wheel'.
bool isManagedMatUnwanted(const std::string &matname)
int PropID_t
Index to GfxActor::m_props, use RoR::PROPID_INVALID as empty value.
Ogre::Vector3 tct_pos
Args#234, required.