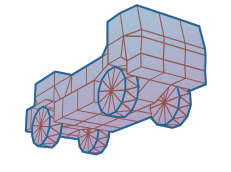 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
37 #include <rapidjson/document.h>
41 #define CACHE_FILE "mods.cache"
42 #define CACHE_FILE_FORMAT 14
43 #define CACHE_FILE_FRESHNESS 86400 // 60*60*24 = one day
355 void AddFile(Ogre::String group, Ogre::FileInfo f, Ogre::String ext);
380 bool Match(
size_t& out_score, std::string data, std::string
const& query,
size_t );
396 {108,
_LC(
"ModCategory",
"Other Land Vehicles")},
398 {146,
_LC(
"ModCategory",
"Street Cars")},
399 {147,
_LC(
"ModCategory",
"Light Racing Cars")},
400 {148,
_LC(
"ModCategory",
"Offroad Cars")},
401 {149,
_LC(
"ModCategory",
"Fantasy Cars")},
402 {150,
_LC(
"ModCategory",
"Bikes")},
403 {155,
_LC(
"ModCategory",
"Crawlers")},
405 {152,
_LC(
"ModCategory",
"Towercranes")},
406 {153,
_LC(
"ModCategory",
"Mobile Cranes")},
407 {154,
_LC(
"ModCategory",
"Other cranes")},
409 {107,
_LC(
"ModCategory",
"Buses")},
410 {151,
_LC(
"ModCategory",
"Tractors")},
411 {156,
_LC(
"ModCategory",
"Forklifts")},
412 {159,
_LC(
"ModCategory",
"Fantasy Trucks")},
413 {160,
_LC(
"ModCategory",
"Transport Trucks")},
414 {161,
_LC(
"ModCategory",
"Racing Trucks")},
415 {162,
_LC(
"ModCategory",
"Offroad Trucks")},
417 {110,
_LC(
"ModCategory",
"Boats")},
419 {113,
_LC(
"ModCategory",
"Helicopters")},
420 {114,
_LC(
"ModCategory",
"Aircraft")},
422 {117,
_LC(
"ModCategory",
"Trailers")},
423 {118,
_LC(
"ModCategory",
"Other Loads")},
426 {129,
_LC(
"ModCategory",
"Addon Terrains")},
428 {859,
_LC(
"ModCategory",
"Container")},
430 {875,
_LC(
"ModCategory",
"Submarine")},
433 {5000,
_LC(
"ModCategory",
"Official Terrains")},
434 {5001,
_LC(
"ModCategory",
"Night Terrains")},
int CacheEntryID_t
index to CacheSystem::m_cache_entries, use RoR::CACHEENTRYNUM_INVALID as empty value.
std::string resource_bundle_type
Archive type recognized by OGRE resource system: 'FileSystem' or 'Zip'.
std::set< std::string > addonpart_guids
GUIDs of all vehicles this addonpart is used with.
std::vector< Ogre::String > m_known_extensions
the extensions we track in the cache system
void LoadAssetPack(CacheEntryPtr &t_dest, Ogre::String const &assetpack_filename)
Adds asset pack to the requesting cache entry's resource group.
const std::vector< CacheEntryPtr > & GetEntries() const
void UnLoadResource(CacheEntryPtr &t)
Unloads associated bundle, destroying all spawned actors.
std::map< int, Ogre::String > CategoryIdNameMap
void FillTerrainDetailInfo(CacheEntryPtr &entry, Ogre::DataStreamPtr ds, Ogre::String fname)
int categoryid
category id
Ogre::String dname
name parsed from the file
@ GUID
Partial match in: guid.
@ TUNEUP_FORCEREMOVE_PROP_SET
'subject_id' is prop ID.
std::string cpr_description
Optional, implemented for tuneups.
bool deleted
is this mod deleted?
void DeleteProject(CacheEntryPtr &entry)
int cqy_filter_category_id
@ TUNEUP_PROTECTED_FLEXBODY_SET
'subject_id' is flexbody ID.
RefCountingObjectPtr< CacheEntry > CacheEntryPtr
@ TUNEUP_FORCEREMOVE_PROP_RESET
'subject_id' is prop ID.
std::shared_ptr< RoR::SkinDef > skin_def
Cached skin info, added on first use or during cache rebuild.
int version
file's version
void ClearResourceGroups()
@ TUNEUP_FORCEREMOVE_FLEXBODY_SET
'subject_id' is flexbody ID.
void RemoveFileCache(CacheEntryPtr &entry)
CacheSearchMethod cqy_search_method
@ SAVE_TUNEUP
Dumps .tuneup file with CID_Tuneup from source actor, will not overwrite existing unless explicitly i...
std::time_t m_update_time
Ensures that all inserted files share the same timestamp.
void LoadModCache(CacheValidity validity)
void AddFile(Ogre::String group, Ogre::FileInfo f, Ogre::String ext)
CacheEntryID_t number
Sequential number, assigned internally, used by Selector-GUI.
CreateProjectRequestType cpr_type
std::vector< CacheEntryPtr > m_entries
@ FILENAME
Partial match in file name.
@ TUNEUP_USE_ADDONPART_RESET
'subject' is addonpart filename.
RoR::TuneupDefPtr tuneup_def
Cached tuning info, added on first use or during cache rebuild.
@ TUNEUP_PROTECTED_WHEEL_RESET
'subject_id' is wheel ID.
CacheEntryPtr GetEntryByNumber(int modid)
@ TUNEUP_PROTECTED_FLEXBODY_RESET
'subject_id' is flexbody ID.
@ CID_Projects
For truck files under 'projects/' directory, to allow listing from editors.
std::string tuneup_associated_filename
Value of 'filename' field in the tuneup file; always lowercase.
void FillAddonPartDetailInfo(CacheEntryPtr &entry, Ogre::DataStreamPtr ds)
Ogre::String resource_group
Resource group of the loaded bundle. Empty if not loaded yet.
void ModifyProject(ModifyProjectRequest *request)
@ TUNEUP_USE_ADDONPART_SET
'subject' is addonpart filename.
@ TUNEUP_PROTECTED_EXHAUST_RESET
'subject_id' is exhaust ID.
@ TUNEUP_PROTECTED_MANAGEDMAT_SET
'subject' is managed material name.
std::vector< Ogre::String > sectionconfigs
void ExportEntryToJson(rapidjson::Value &j_entries, rapidjson::Document &j_doc, CacheEntryPtr const &entry)
static Ogre::String StripSHA1fromString(Ogre::String sha1str)
void ImportEntryFromJson(rapidjson::Value &j_entry, CacheEntryPtr &out_entry)
void FillSkinDetailInfo(CacheEntryPtr &entry, std::shared_ptr< SkinDef > &skin_def)
A content database MOTIVATION: RoR users usually have A LOT of content installed.
CacheEntryPtr cpr_source_entry
The original mod to copy files from.
std::string cpr_name
Directory and also the mod file (without extension).
@ TUNEUP_FORCEREMOVE_MANAGEDMAT_SET
'subject' is managed material name.
bool IsPathContentDirRoot(const std::string &path) const
@ NONE
Ignore the search string and find all.
std::set< std::string > addonpart_filenames
File names of all vehicles this addonpart is used with. If empty, any filename goes.
bool operator<(CacheQueryResult const &other) const
CacheValidity LoadCacheFileJson()
@ TUNEUP_FORCED_WHEEL_SIDE_SET
'subject_id' is wheel ID, 'value_int' is RoR::WheelSide
@ TUNEUP_PROTECTED_FLARE_RESET
'subject_id' is flare ID.
std::map< int, size_t > cqy_res_category_usage
Total usage (ignores search params + category filter)
void LoadAssociatedSkinDef(CacheEntryPtr &cache_entry)
Loads+parses the .skin file and updates all related CacheEntries.
@ AUTHORS
Partial match in: author name/email.
@ TUNEUP_FORCEREMOVE_FLARE_RESET
'subject_id' is flare ID.
void FillTruckDetailInfo(CacheEntryPtr &entry, Ogre::DataStreamPtr ds, Ogre::String fname, Ogre::String group)
@ TUNEUP_PROTECTED_FLARE_SET
'subject_id' is flare ID.
CacheEntryPtr FindEntryByFilename(RoR::LoaderType type, bool partial, const std::string &_filename_maybe_bundlequalified)
Returns NULL if none found; "Bundle-qualified" format also specifies the ZIP/directory in modcache,...
Core data structures for simulation; Everything affected by by either physics, network or user intera...
CacheEntry()
default constructor resets the data.
bool Match(size_t &out_score, std::string data, std::string const &query, size_t)
std::vector< AuthorInfo > authors
authors
Ogre::String filecachename
preview image filename
void ParseZipArchives(Ogre::String group)
@ TUNEUP_PROTECTED_EXHAUST_SET
'subject_id' is exhaust ID.
const CategoryIdNameMap & GetCategories() const
const std::vector< std::string > & GetContentDirs() const
std::time_t addtimestamp
timestamp when this file was added to the cache
void ParseSingleZip(Ogre::String path)
void FillTuneupDetailInfo(CacheEntryPtr &entry, TuneupDefPtr &tuneup_def)
void LoadAssociatedTuneupDef(CacheEntryPtr &cache_entry)
Loads+parses the .tuneup file and updates all related CacheEntries.
Ogre::String GetPrettyName(Ogre::String fname)
bool ParseKnownFiles(Ogre::String group)
RigDef::DocumentPtr actor_def
Cached actor definition (aka truckfile) after first spawn.
@ TUNEUP_FORCEREMOVE_MANAGEDMAT_RESET
'subject' is managed material name.
Ogre::String fpath
filepath relative to the .zip file
@ FULLTEXT
Partial match in: name, filename, description, author name/mail.
@ WHEELS
Wheel configuration, i.e. 4x4.
LoaderType
< Search mode for ModCache::Query() & Operation mode for GUI::MainSelector
@ TUNEUP_FORCEREMOVE_EXHAUST_RESET
'subject_id' is exhaust ID.
ActorPtr mpr_target_actor
Central state/object manager and communications hub.
std::string m_filenames_hash_loaded
hash from cachefile, for quick update detection
CacheQueryResult(CacheEntryPtr entry, size_t score)
std::string cqy_search_string
std::vector< std::string > m_content_dirs
the various mod directories we track in the cache system
std::map< int, Ogre::String > m_categories
std::string m_filenames_hash_generated
stores hash over the content, for quick update detection
std::time_t cqy_res_last_update
void GenerateFileCache(CacheEntryPtr &entry, Ogre::String group)
Ogre::String uniqueid
file's unique id
CacheValidity EvaluateCacheValidity()
@ TUNEUP_FORCEREMOVE_EXHAUST_SET
'subject_id' is exhaust ID.
CacheEntryPtr CreateProject(CreateProjectRequest *request)
Creates subdirectory in 'My Games\Rigs of Rods\projects', pre-populates it with files and adds modcac...
void LoadSupplementaryDocuments(CacheEntryPtr &t)
Loads the associated .truck*, .skin and .tuneup files.
@ TUNEUP_FORCEREMOVE_FLARE_SET
'subject_id' is flare ID.
Creates subdirectory in 'My Games\Rigs of Rods\projects', pre-populates it with files and adds modcac...
@ PROJECT_LOAD_TUNEUP
'subject' is tuneup filename. This overwrites the auto-generated tuneup with the save.
std::string ActorTypeToName(ActorType driveable)
static std::string ComposeResourceGroupName(const CacheEntryPtr &entry)
@ TUNEUP_PROTECTED_PROP_SET
'subject_id' is prop ID.
ActorPtr cpr_source_actor
Only for type SAVE_TUNEUP
CacheEntryPtr FetchSkinByName(std::string const &skin_name)
@ CID_Tuneups
For unsorted tuneup files.
int usagecounter
how much it was used already
Ogre::String fext
file's extension
RoR::LoaderType cqy_filter_type
void LoadResource(CacheEntryPtr &t)
Loads the associated resource bundle if not already done.
void WriteCacheFileJson()
@ CID_Max
SPECIAL VALUE - Maximum allowed to be present in any mod files.
@ TUNEUP_FORCEREMOVE_FLEXBODY_RESET
'subject_id' is flexbody ID.
std::string resource_bundle_path
Path of ZIP or directory which contains the media. Shared between CacheEntries, loaded only once.
@ TUNEUP_PROTECTED_PROP_RESET
'subject_id' is prop ID.
@ TUNEUP_PROTECTED_MANAGEDMAT_RESET
'subject' is managed material name.
void ReLoadResource(CacheEntryPtr &t)
Forces reloading the associated bundle.
Ogre::String categoryname
category name
@ TUNEUP_PROTECTED_WHEEL_SET
'subject_id' is wheel ID.
@ PROJECT_RESET_TUNEUP
'subject' is empty. This resets the auto-generated tuneup to orig. values.
std::string cqy_filter_guid
Exact match (case-insensitive); leave empty to disable.
std::shared_ptr< Document > DocumentPtr
Self reference-counting objects, as requred by AngelScript garbage collector.
ModifyProjectRequestType mpr_type
ActorType
< Aka 'Driveable'
@ TUNEUP_FORCED_WHEEL_SIDE_RESET
'subject_id' is wheel ID.
std::time_t filetime
filetime
void FillAssetPackDetailInfo(CacheEntryPtr &entry, Ogre::DataStreamPtr ds)
@ DEFAULT
Copy files from source mod. Source mod Determines mod file extension.
std::string cqy_filter_target_filename
Exact match (case-insensitive); leave empty to disable (currently only used with addonparts)
Data structures representing 'truck' file format, see https://docs.rigsofrods.org/vehicle-creation/fi...
size_t Query(CacheQuery &query)
RoR::TuneupDefPtr addonpart_data_only
Cached addonpart data (dummy tuneup), only used for evaluating conflicts, see AddonPartUtility::Recor...
std::vector< CacheQueryResult > cqy_results
void GenerateHashFromFilenames()
For quick detection of added/removed content.
Ogre::String guid
global unique id; Type "addonpart" leaves this empty and uses addonpart_guids; Always lowercase.
std::set< Ogre::String > m_resource_paths
A temporary list of existing resource paths.
static Ogre::String StripUIDfromString(Ogre::String uidstr)
Ogre::String fname
filename
Ogre::String fname_without_uid
filename