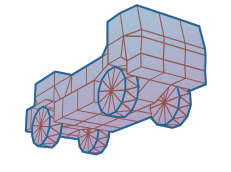 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
40 #include <OgreUTFString.h>
41 #include <rapidjson/document.h>
624 Ogre::BillboardSet *
bbs =
nullptr;
866 std::shared_ptr<rapidjson::Document>
888 std::shared_ptr<rapidjson::Document>
int16_t nd_coll_bbox_id
Optional attribute (-1 = none) - multiple collision bounding boxes defined in truckfile.
float solid_ground_level
how deep the solid ground is
static const int MAX_COMMANDS
maximum number of commands per actor
node_t * wh_rim_nodes[50]
int last_debug_state
smart debug output
float fx_particle_max_velo
maximum velocity to display sparks
RoR::CmdKeyInertia command_inertia
bool nd_contacter
Attr; User-defined.
SoundScriptInstancePtr ssi
bool asr_free_position
Disables the automatic spawn position adjustment.
bool cmb_is_force_restricted
Attribute defined in truckfile.
Global forward declarations.
bool nd_immovable
Attr; User-defined.
@ NETWORK
Remote controlled.
std::array< command_t, MAX_COMMANDS+1 > m_commandkey
BEWARE: commandkeys are indexed 1-MAX_COMMANDS!
float strength
ground strength
Ogre::BillboardSet * bbs
This remains nullptr if removed via addonpart_unwanted_flare or Tuning UI.
NodeNum_t ffc_target_node
@ TRUCK
its a truck (or other land vehicle)
std::vector< int > rotators
Ogre::Real nd_avg_collision_slip
Physics state; average slip velocity across the last few physics frames.
ground_model_t * nd_last_collision_gm
Physics state; last collision 'ground model' (surface definition)
float va
adhesion velocity
@ SHOCK_FLAG_TRG_BLOCKER_A
float springin
shocks2 & shocks3
@ EVENT_AIRPLANE
'airplane' ~ Triggered by any node of airplane (ActorType::AIRPLANE)
@ CONFIG_FILE
'Preselected vehicle' in RoR.cfg or command line
Ogre::Vector3 AbsPosition
absolute position in the world (shaky)
bool trigger_enabled
general trigger,switch and blocker state
int dashboard_link
Only 'd' type flares, valid values are DD_*.
@ EVENT_TRUCK_WHEELS
'truck_wheels' ~ Triggered only by wheel nodes of land vehicle (ActorType::TRUCK)
Estabilishing a physics linkage between 2 actors modifies a global linkage table and triggers immedia...
CollisionEventFilter
Specified in terrain object (.ODEF) file, syntax: 'event <type> <filter>'.
bool nd_under_water
State; GFX hint.
Ogre::ParticleSystem * smoker
This remains nullptr if removed via addonpart_unwanted_exhaust or Tuning UI.
float hb_ref_length
Idle length in meters.
static const NodeNum_t NODENUM_INVALID
bool nd_contactable
Attr; This node will be treated as contacter on inter truck collisions.
static const int8_t INVALID_BBOX
Ogre::Vector3 lo
absolute collision box
bool nd_override_mass
User defined attr; mass is user-specified rather than calculated (override the calculation)
@ DISPOSED
removed from simulation, still in memory to satisfy pointers.
ActorLinkingRequestType alr_type
bool uses_inertia
Only 'flares3'.
float fx_particle_fade
fade coefficient
command_t & operator[](int index)
int pos
Index into ar_ropables.
float cmb_engine_coupling
Attr from truckfile.
float trigger_boundary_t
optional value to tune trigger_switch_state autorelease
float ffc_force_magnitude
bool trigger_cmdkeyblock_state
identifies blocked F-commands for triggers
Ogre::Vector3 hi
absolute collision box
@ EVENT_BOAT
'boat' ~ Triggered by any node of boats (ActorType::BOAT)
ActorPtr bm_locked_actor
in case p2 is on another actor
std::vector< collision_box_t * > CollisionBoxPtrVec
User input state for animated props with 'source:event'.
float hk_min_length
Absolute value in meters.
@ EVENT_AVATAR
'avatar' ~ Triggered by the character only
float dampout
shocks2 & shocks3
TuneupDefPtr asr_working_tuneup
Only filled when editing tuneup via Tuning menu.
Ogre::Real wh_mass
Total rotational mass of the wheel.
ActorPtr ffc_target_actor
Designed to be run on main/rendering loop (FPS)
@ LOCAL_SIMULATED
simulated (local) actor
std::string asr_filename
Can be in "Bundle-qualified" format, i.e. "mybundle.zip:myactor.truck".
Ogre::Vector3 RelPosition
relative to the local physics origin (one origin per actor) (shaky)
@ NOT_DRIVEABLE
not drivable at all
float fx_particle_velo_factor
velocity factor
std::shared_ptr< commandbeam_state_t > cmb_state
int8_t auto_moving_mode
State.
Ogre::Quaternion unrot
rotation
@ SAVEGAME
User spawned and part of a savegame.
int fx_particle_amount
amount of particles
@ EVENT_TRUCK
'truck' ~ Triggered by any node of land vehicle (ActorType::TRUCK)
Ogre::Real wh_alb_coef
Sim state; Current anti-lock brake modulation ratio.
float mc
sliding friction coefficient
Ogre::Vector3 center
center of rotation
int hb_anim_flags
Animators (beams updating length based on simulation variables)
float vs
stribeck velocity (m/s)
float refL
reference length
float initial_beam_strength
for reset
Ogre::Vector3 ffr_force_const_direction
std::unordered_map< int, command_t > m_virtualkey
Negative-indexed commandkeys.
ActorInstanceID_t amr_actor
Ogre::Real wh_last_retorque
Last external forces (friction, ...)
CollisionEventFilter event_filter
Ogre::Vector3 debug_force
Simulation: An edge in the softbody structure.
static const FreeForceID_t FREEFORCEID_INVALID
@ PRELOCK
prelocking, attraction forces in action
@ SHOCK_FLAG_TRG_HOOK_LOCK
Ogre::Vector3 rehi
relative collision box
int CommandkeyID_t
Index into Actor::ar_commandkeys (BEWARE: indexed 1-MAX_COMMANDKEYS, 0 is invalid value,...
@ TOWARDS_COORDS
Constant force directed towards ffc_target_coords
int ActorInstanceID_t
Unique sequentially generated ID of an actor in session. Use ActorManager::GetActorById()
Ogre::Real wh_torque
Internal physics state; Do not read from this.
ropable_t * rp_locked_ropable
bool cmb_is_contraction
Attribute defined at spawn.
uint16_t NodeNum_t
Node position within Actor::ar_nodes; use RoR::NODENUM_INVALID as empty value.
@ UNLOCKED
lock not locked
static const int DEFAULT_DETACHER_GROUP
float ti_min_length
Proportional to orig; length.
Ogre::Vector3 ffc_force_const_direction
Expected to be normalized; only effective with FreeForceType::CONSTANT
float sbd_damp
set beam default for damping
Ogre::Vector3 selfcenter
center of self rotation
int trigger_cmdshort
F-key for trigger injection shortbound-check.
Ogre::Vector3 relo
relative collision box
float cmb_center_length
Attr computed at spawn.
bool cmb_needs_engine
Attribute defined in truckfile.
CacheEntryPtr asr_tuneup_entry
Only filled when user selected a saved/downloaded .tuneup mod in SelectorUI.
static const ActorInstanceID_t ACTORINSTANCEID_INVALID
CacheEntryPtr asr_cache_entry
Optional, overrides 'asr_filename' and 'asr_cache_entry_num'.
RoR::CmdKeyInertia hb_inertia
float trigger_switch_state
needed to avoid doubleswitch, bool and timer in one
@ LOCAL_SLEEPING
sleeping (local) actor
bool nd_cab_node
Attr; This node is part of collision triangle.
float cmb_speed
Attr; Rate of contraction/extension.
@ BEAM_VIRTUAL
Excluded from mass calculations, visuals permanently disabled.
bool nd_no_mouse_grab
Attr; User-defined.
int FreeForceID_t
Unique sequentially generated ID of FreeForce; use ActorManager::GetFreeForceNextId().
int trigger_cmdlong
F-key for trigger injection longbound-check.
@ CONSTANT
Constant force given by direction and magnitude.
NodeNum_t pos
This node's index in Actor::ar_nodes array.
Ogre::Vector3 ffr_target_coords
bool nd_tyre_node
Attr; This node is part of a tyre (note some wheel types don't use rim nodes at all)
std::string amr_addonpart_fname
Fallback method in case CacheEntry doesn't exist anymore - that means mod was uninstalled in the mean...
float debug_k
debug shock spring_rate
RoR::CmdKeyInertia rotator_inertia
node_t * wh_near_attach_node
bool nd_loaded_mass
User defined attr; mass is calculated from 'globals/loaded-mass' rather than 'globals/dry-mass'.
Ogre::Vector3 nd_last_collision_force
Physics state; last collision force.
@ SHOCK_FLAG_TRG_CONTINUOUS
Physics: A vertex in the softbody structure.
float cmb_boundary_length
Attr; Maximum/minimum length proportional to orig. len.
@ EVENT_NONE
Invalid value.
BrakeCombo
Wheels are braked by three mechanisms: A footbrake, a handbrake/parkingbrake, and directional brakes ...
float springout
shocks2 & shocks3
Designed to be run in physics loop (2khz)
@ AIRPLANE
its an airplane
double ffr_force_magnitude
bool pressed_center_mode
State.
Global force affecting particular (base) node of particular (base) actor; added ad-hoc by scripts.
Ogre::Real wh_speed
Current wheel speed in m/s.
bool cmb_is_1press
Attribute defined in truckfile.
Ogre::Vector3 campos
camera position
@ TERRN_DEF
Preloaded with terrain.
bool asr_terrn_machine
This is a fixed machinery.
Ogre::Real wh_avg_speed
Internal physics state; Do not read from this.
CacheEntryPtr asr_skin_entry
float t2
hydrodynamic friction (s/m)
uint16_t hb_beam_index
Index to Actor::ar_beams array.
Ogre::Vector3 nd_last_collision_slip
Physics state; last collision slip vector.
std::shared_ptr< rapidjson::Document > amr_saved_state
Ogre::Vector3 debug_scaled_cforce
float ms
static friction coefficient
Ogre::Quaternion selfrot
self rotation
@ SHOCK_FLAG_TRG_CMD_SWITCH
bool nd_has_mesh_contact
Physics state.
std::string uckp_description
float debug_d
debug shock damping
bool bm_inter_actor
in case p2 is on another actor
int controlnumber
Only 'u' type flares, valid values 0-9, maps to EV_TRUCK_LIGHTTOGGLE01 to 10.
UI helper for displaying command control keys to user.
Common for ADD and MODIFY requests; tailored for use with AngelScript thru GameScript::pushMessage().
float flow_behavior_index
if flow_behavior_index<1 then liquid is Pseudoplastic (ketchup, whipped cream, paint) if =1 then liqu...
float debug_v
debug shock velocity
Ogre::ParticleSystem * psys
bool nd_rim_node
Attr; This node is part of a rim (only wheel types with separate rim nodes)
float fx_particle_timedelta
delta for particle animation
CacheEntryPtr amr_addonpart
Primary method of specifying cache entry.
Ogre::Real wh_last_torque
Last internal forces (engine / brakes / diffs)
bool nd_has_ground_contact
Physics state.
@ SHOCK_FLAG_TRG_CMD_BLOCKER
Ogre::UTFString asr_net_username
Ogre::Quaternion rot
rotation
@ HYDRO_FLAG_REV_ELEVATOR
@ TOWARDS_NODE
Constant force directed towards ffc_target_node
float fluid_density
Density of liquid.
Ogre::Quaternion selfunrot
self rotation
float dampin
shocks2 & shocks3
int detacher_group
Attribute: detacher group number (integer)
Surface friction properties.
float drag_anisotropy
Upwards/Downwards drag anisotropy.
ropable_t * ti_locked_ropable
@ SHOCK_FLAG_TRG_HOOK_UNLOCK
ActorInstanceID_t alr_actor_instance_id
bool auto_move_lock
State.
std::vector< commandbeam_t > beams
bool nd_no_ground_contact
User-defined attr; node ignores contact with ground.
bool cmb_is_1press_center
Attribute defined in truckfile.
Ogre::Real wh_tc_coef
Sim state; Current traction control modulation ratio.
NodeNum_t alr_hook_mousenode
Ogre::SceneNode * smokeNode
float hb_anim_param
Animators (beams updating length based on simulation variables)
float hb_speed
Rate of change.
@ EVENT_ALL
(default) ~ Triggered by any node on any vehicle
float sbd_spring
set beam default for spring
@ NETWORKED_HIDDEN
not simulated, not updated (remote)
Ogre::Vector3 debug_verts[8]
box corners in absolute world position
@ USER
Direct selection by user via GUI.
bool ti_no_self_lock
Attribute.
bool cmb_plays_sound
Attribute defined in truckfile.
float flow_consistency_index
general drag coefficient
std::string particleSystemName
Name in .particle file ~ for display in Tuning UI.
Ogre::Vector3 asr_position
ActorType
< Aka 'Driveable'
@ NETWORKED_OK
not simulated (remote) actor
uint16_t cmb_beam_index
Index to Actor::ar_beams array.
bool cmb_is_autocentering
Attribute defined in truckfile.
int16_t nd_lockgroup
Optional attribute (-1 = default, 9999 = deny lock) - used in the hook lock logic.
@ AI
machine controlled by an Artificial Intelligence
Ogre::Vector3 ffc_target_coords
collision_box_t * asr_spawnbox
Ogre::Quaternion asr_rotation
float default_beam_deform
for reset
static const CommandkeyID_t COMMANDKEYID_INVALID
float fx_particle_min_velo
minimum velocity to display sparks
Origin
< Enables special processing
SimpleInertia inertia
Only 'flares3'.
For backwards compatibility of the 'triggers' feature, the commandkey array must support negative ind...
std::shared_ptr< rapidjson::Document > asr_saved_state
Pushes msg MODIFY_ACTOR (type RESTORE_SAVED) after spawn.
Ogre::ColourValue fx_colour
@ RELOAD
Full reload from filesystem, requested by user.