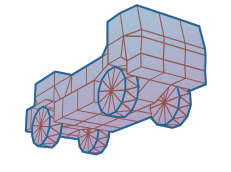 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
29 #include <OgreResourceGroupManager.h>
30 #include <OgreScriptCompiler.h>
31 #include <rapidjson/document.h>
36 public Ogre::ResourceLoadingListener,
37 public Ogre::ScriptCompilerListener
84 bool DeleteDiskFile(std::string
const& filename, std::string
const& rg_name);
87 bool LoadAndParseJson(std::string
const& filename, std::string
const& rg_name, rapidjson::Document& j_doc);
88 bool SerializeAndWriteJson(std::string
const& filename, std::string
const& rg_name, rapidjson::Document& j_doc);
93 Ogre::DataStreamPtr
resourceLoading(
const Ogre::String& name,
const Ogre::String& group, Ogre::Resource* resource)
override;
94 void resourceStreamOpened(
const Ogre::String& name,
const Ogre::String& group, Ogre::Resource* resource, Ogre::DataStreamPtr& dataStream)
override;
95 bool resourceCollision(Ogre::Resource* resource, Ogre::ResourceManager* resourceManager)
override;
98 bool handleEvent(Ogre::ScriptCompiler *compiler, Ogre::ScriptCompilerEvent *evt,
void *retval)
override;
static const ResourcePack HYDRAX
void InitContentManager()
static const ResourcePack CUBEMAPS
bool DeleteDiskFile(std::string const &filename, std::string const &rg_name)
static const ResourcePack WALLPAPERS
Ogre::DataStreamPtr resourceLoading(const Ogre::String &name, const Ogre::String &group, Ogre::Resource *resource) override
void InitManagedMaterials(std::string const &rg_name)
static const ResourcePack PAGED
bool handleEvent(Ogre::ScriptCompiler *compiler, Ogre::ScriptCompilerEvent *evt, void *retval) override
bool resourceCollision(Ogre::Resource *resource, Ogre::ResourceManager *resourceManager) override
static const ResourcePack CAELUM
bool SerializeAndWriteJson(std::string const &filename, std::string const &rg_name, rapidjson::Document &j_doc)
static const ResourcePack DASHBOARDS
static const ResourcePack BEAM_OBJECTS
ResourcePack(const char *name, const char *resource_group_name)
A database of user-installed content alias 'mods' (vehicles, terrains...)
static const ResourcePack FLAGS
static const ResourcePack SCRIPTS
static const ResourcePack AIRFOILS
static const ResourcePack TEXTURES
void resourceStreamOpened(const Ogre::String &name, const Ogre::String &group, Ogre::Resource *resource, Ogre::DataStreamPtr &dataStream) override
bool LoadAndParseJson(std::string const &filename, std::string const &rg_name, rapidjson::Document &j_doc)
Central state/object manager and communications hub.
static const ResourcePack MATERIALS
static const ResourcePack ICONS
static const ResourcePack PARTICLES
void AddResourcePack(ResourcePack const &resource_pack, std::string const &override_rgn="")
Loads resources if not already loaded.
static const ResourcePack OVERLAYS
static const ResourcePack OGRE_CORE
static const ResourcePack SOUNDS
void LoadGameplayResources()
Checks GVar settings and loads required resources.
static const ResourcePack PSSM
const char * resource_group_name
void InitModCache(CacheValidity validity)
static const ResourcePack RTSHADER
static const ResourcePack SKYX
static const ResourcePack FAMICONS
static const ResourcePack MYGUI
std::string ListAllUserContent()
Used by ModCache for quick detection of added/removed content.
bool m_base_resource_loaded
static const ResourcePack FONTS
static const ResourcePack MESHES