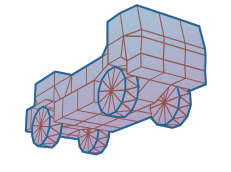 |
RigsofRods
2023.09
Soft-body Physics Simulation
|
Go to the documentation of this file.
157 Ogre::Quaternion
pp_rot = Ogre::Quaternion::IDENTITY;
339 Ogre::ParticleSystem*
psys =
nullptr;
Ogre::Quaternion vcam_rotation
const PropAnimFlag_t PROP_ANIM_FLAG_AIRSPEED
const PropAnimFlag_t PROP_ANIM_FLAG_TORQUE
const PropAnimFlag_t PROP_ANIM_FLAG_SHIFTER
'shifterman1, shifterman2, sequential, shifterlin, autoshifterlin'; animOpt3: see RoR::ShifterPropAni...
WheelSide
Used by rig-def/addonpart/tuneup formats to specify wheel rim mesh orientation.
float upper_limit
The upper limit for the animation.
const PropAnimMode_t PROP_ANIM_MODE_OFFSET_X
CameraMode_t pp_camera_mode_orig
Dynamic visibility mode {0 and higher = cinecam index}.
const PropAnimFlag_t PROP_ANIM_FLAG_AIRBRAKE
const PropAnimFlag_t PROP_ANIM_FLAG_AOA
std::string fbr_mesh_name
Visuals of softbody beam (beam_t struct); Partially updated along with SimBuffer.
Ogre::MaterialPtr vcam_material
const PropAnimFlag_t PROP_ANIM_FLAG_BRAKE
float pp_beacon_rot_angle[4]
Radians.
const PropAnimMode_t PROP_ANIM_MODE_ROTA_Z
int FreeBeamGfxID_t
Index into GfxScene::m_gfx_freebeams, use RoR::FREEBEAMGFXID_INVALID as empty value.
float lower_limit
The lower limit for the animation.
const PropAnimFlag_t PROP_ANIM_FLAG_DASHBOARD
Used with dashboard system inputs, see enum DashData in file DashBoardManager.h.
Ogre::TexturePtr vcam_render_tex
const PropAnimMode_t PROP_ANIM_MODE_ROTA_X
static const NodeNum_t NODENUM_INVALID
static const PropID_t PROPID_INVALID
bool nx_no_particles
User-defined attr; disable all particles.
FreeForceID_t fbx_freeforce_primary
Required.
Ogre::SceneNode * wx_scenenode
std::string fbr_material_name
static const FreeBeamGfxID_t FREEBEAMGFXID_INVALID
NodeNum_t vcam_node_center
const PropAnimMode_t PROP_ANIM_MODE_NOFLIP
Ogre::Vector3 pp_offset_orig
Used with ANIM_FLAG_OFFSET*.
std::string vcam_mat_name_orig
For display in Tuning UI: Original material name from rig-def file, without per-actor stamping.
static const float DEFAULT_BEAM_DIAMETER
5 centimeters default beam width
Ogre::RenderTexture * vcam_render_target
NodeGfx(NodeNum_t node_idx)
Used by MSG_EDI_[ADD/MODIFY]_FREEBEAMGFX_REQUESTED; tailored for use with AngelScript thru GameScript...
bool nx_no_sparks
User-defined attr;.
const PropAnimFlag_t PROP_ANIM_FLAG_PBRAKE
NodeNum_t vcam_node_dir_y
const PropAnimFlag_t PROP_ANIM_FLAG_ARUDDER
const PropAnimFlag_t PROP_ANIM_FLAG_RPM
char pp_beacon_type
Special prop: beacon {0 = none, 'b' = user-specified, 'r' = red, 'p' = police lightbar,...
Ogre::SceneNode * vcam_debug_node
const PropAnimFlag_t PROP_ANIM_FLAG_ALTIMETER
Ogre::Vector3 pp_wheel_pos
const PropAnimMode_t PROP_ANIM_MODE_ROTA_Y
bool pp_aero_propeller_spin
Special - blurred spinning propeller effect.
double fbr_diameter
meters
float animratio
A coefficient for the animation, prop degree if used with mode: rotation and propoffset if used with ...
ActorPtr rod_target_actor
Ogre::RenderWindow * vcam_render_window
Visuals of a 'freebeam' (a pair of HALFBEAM_ freeforces)
const PropAnimFlag_t PROP_ANIM_FLAG_STEERING
const PropAnimFlag_t PROP_ANIM_FLAG_GEAR
'gearreverse' (animOpt3=-1), 'gearneutral' (animOpt3=0), 'gear#' (animOpt3=#)
Ogre::SceneNode * pp_wheel_scene_node
@ VCSTATE_ENABLED_OFFLINE
MeshObject * pp_wheel_mesh_obj
Ogre::ParticleSystem * psys
static const FreeForceID_t FREEFORCEID_INVALID
Ogre::SceneNode * fbx_scenenode
NodeNum_t rod_node1
Node index - may change during simulation!
const PropAnimFlag_t PROP_ANIM_FLAG_AETORQUE
Ogre::SceneNode * smokeNode
uint16_t NodeNum_t
Node position within Actor::ar_nodes; use RoR::NODENUM_INVALID as empty value.
const PropAnimFlag_t PROP_ANIM_FLAG_PERMANENT
Gfx attributes/state of a softbody node.
const PropAnimMode_t PROP_ANIM_MODE_AUTOANIMATE
static const WheelID_t WHEELID_INVALID
Ogre::Light * pp_beacon_light[4]
std::string vcam_off_tex_name
Used when videocamera is offline.
const PropAnimFlag_t PROP_ANIM_FLAG_FLAP
const PropAnimFlag_t PROP_ANIM_FLAG_DIFFLOCK
int WheelID_t
Index to Actor::ar_wheels, use RoR::WHEELID_INVALID as empty value.
const PropAnimFlag_t PROP_ANIM_FLAG_AESTATUS
Ogre::Camera * vcam_ogre_camera
int FreeForceID_t
Unique sequentially generated ID of FreeForce; use ActorManager::GetFreeForceNextId().
Ogre::Entity * abx_entity
const PropAnimFlag_t PROP_ANIM_FLAG_SIGNALSTALK
Turn indicator stalk position (-1=left, 0=off, 1=right)
A mesh attached to vehicle frame via 3 nodes.
const PropAnimFlag_t PROP_ANIM_FLAG_VVI
int64_t fbr_freeforce_primary
Required.
const PropAnimMode_t PROP_ANIM_MODE_OFFSET_Z
const PropAnimMode_t PROP_ANIM_MODE_BOUNCE
const PropAnimFlag_t PROP_ANIM_FLAG_ACCEL
NodeNum_t vcam_node_lookat
Only for VCAM_ROLE_TRACK_CAM.
const PropAnimFlag_t PROP_ANIM_FLAG_THROTTLE
Ogre::MaterialPtr mat_instance
An Ogre::Camera mounted on the actor and rendering into either in-scene texture or external window.
Ogre::SceneNode * abx_scenenode
const PropAnimFlag_t PROP_ANIM_FLAG_PITCH
const PropAnimFlag_t PROP_ANIM_FLAG_TURBO
Ogre::SceneNode * vcam_prop_scenenode
Only for VCAM_ROLE_MIRROR_PROP_*.
Ogre::SceneNode * rod_scenenode
bool nx_may_get_wet
Attr; enables water drip and vapour.
NodeNum_t vcam_node_alt_pos
const PropAnimFlag_t PROP_ANIM_FLAG_HEADING
std::string pp_media[2]
Redundant, for Tuning UI. Media1 = prop mesh name, Media2 = steeringwheel mesh/beaconprop flare mat.
BitMask64_t PropAnimFlag_t
bool nx_is_hot
User-defined attr; emits vapour particles when in contact with water.
float animOpt3
MULTIPURPOSE.
static CameraMode_t CAMERA_MODE_ALWAYS_VISIBLE
std::vector< PropAnim > pp_animations
void setPropMeshesVisible(bool visible)
int64_t fbr_id
ID of the freebeam gfx, use GfxScene::GetFreeBeamGfxNextId()
CameraMode_t pp_camera_mode_active
Dynamic visibility mode {0 and higher = cinecam index}.
NodeNum_t rod_node2
Node index - may change during simulation!
NodeNum_t vcam_node_dir_z
bool pp_aero_propeller_blade
Special - single blade mesh.
const PropAnimFlag_t PROP_ANIM_FLAG_AEPITCH
const PropAnimFlag_t PROP_ANIM_FLAG_ELEVATORS
const PropAnimFlag_t PROP_ANIM_FLAG_BRUDDER
const PropAnimFlag_t PROP_ANIM_FLAG_SPEEDO
#define BITMASK64(OFFSET)
Ogre::Vector3 vcam_pos_offset
std::string wx_rim_mesh_name
Redundant, for Tuning UI. Only for 'meshwheels[2]' and 'flexbodywheels'.
MeshObject * pp_mesh_obj
Optional; NULL if removed via tuneup/addonpart.
FreeForceID_t fbx_freeforce_secondary
Not required for fixed-end beams.
float pp_beacon_rot_rate[4]
Radians per second.
std::string particleSystemName
Name in .particle file ~ for display in Tuning UI.
float pp_wheel_rot_degree
Ogre::BillboardSet * pp_beacon_bbs[4]
Ogre::SceneNode * pp_scene_node
The pivot scene node (parented to root-node).
const PropAnimFlag_t PROP_ANIM_FLAG_CLUTCH
Ogre::ColourValue emissive_color
const PropAnimFlag_t PROP_ANIM_FLAG_ROLL
Ogre::ParticleSystem * smoker
This remains nullptr if removed via addonpart_unwanted_exhaust or Tuning UI.
float nx_wet_time_sec
'Wet' means "already out of water, producing dripping particles". Set to -1 when not 'wet'.
const PropAnimFlag_t PROP_ANIM_FLAG_AILERONS
Ogre::SceneNode * pp_beacon_scene_node[4]
int PropID_t
Index to GfxActor::m_props, use RoR::PROPID_INVALID as empty value.
const PropAnimFlag_t PROP_ANIM_FLAG_BTHROTTLE
const PropAnimFlag_t PROP_ANIM_FLAG_EVENT
int64_t fbr_freeforce_secondary
Not required for fixed-end beams.
const PropAnimMode_t PROP_ANIM_MODE_OFFSET_Y
const PropAnimFlag_t PROP_ANIM_FLAG_TACHO
bool nx_under_water_prev
State.
FreeBeamGfxID_t fbx_id
ID of the freebeam gfx, use GfxScene::GetFreeBeamGfxNextId()
int pp_aero_engine_idx
Special - a turboprop/pistonprop reference.
ShifterPropAnim
< PropAnim::animOpt3 values for PROP_ANIM_FLAG_SHIFTER