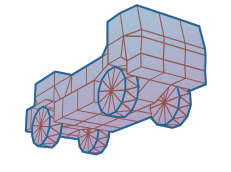 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
37 Engine::Engine(
float _min_rpm,
float _max_rpm,
float torque,
float reverse_gear,
float neutral_gear, std::vector<float> forward_gears,
float diff_ratio,
ActorPtr actor) :
39 , m_auto_cur_acc(0.0f)
42 , m_braking_torque(-torque / 5.0f)
43 , m_clutch_force(10000.0f)
48 , m_cur_clutch_torque(0.0f)
49 , m_cur_engine_rpm(0.0f)
50 , m_cur_engine_torque(0.0f)
53 , m_cur_wheel_revolutions(0.0f)
54 , m_ref_wheel_revolutions(0.0f)
55 , m_diff_ratio(diff_ratio)
57 , m_engine_torque(torque)
58 , m_engine_has_air(true)
59 , m_engine_has_turbo(true)
60 , m_hydropump_state(0.0f)
61 , m_engine_idle_rpm(std::min(std::abs(_min_rpm), 800.0f))
62 , m_engine_inertia(10.0f)
63 , m_kickdown_delay_counter(0)
64 , m_max_idle_mixture(0.1f)
65 , m_engine_max_rpm(std::abs(_max_rpm))
66 , m_min_idle_mixture(0.0f)
67 , m_engine_shiftup_rpm(std::abs(_min_rpm))
68 , m_num_gears((int)forward_gears.size())
69 , m_post_shift_time(0.2f)
70 , m_post_shift_clock(0.0f)
72 , m_engine_is_priming(false)
73 , m_engine_is_running(false)
74 , m_shift_behaviour(0.0f)
79 , m_engine_stall_rpm(300.0f)
83 , m_engine_turbo_mode(OLD)
85 , m_upshift_delay_counter(0)
86 , m_engine_is_electric(false)
87 , m_turbo_inertia_factor(1)
89 , m_max_turbo_rpm(200000.0f)
90 , m_turbo_engine_rpm_operation(0.0f)
93 , m_min_wastegate_psi(20)
94 , m_turbo_has_wastegate(false)
95 , m_turbo_has_bov(false)
96 , m_turbo_flutters(false)
97 , m_turbo_wg_threshold_p(0)
98 , m_turbo_wg_threshold_n(0)
99 , m_turbo_has_antilag(false)
100 , m_antilag_rand_chance(0.9975)
101 , m_antilag_min_rpm(3000)
102 , m_antilag_power_factor(170)
117 for (
float gear : forward_gears)
128 void Engine::SetTurboOptions(
int type,
float tinertiaFactor,
int nturbos,
float param1,
float param2,
float param3,
float param4,
float param5,
float param6,
float param7,
float param8,
float param9,
float param10,
float param11)
136 LOG(
"Turbo: No more than 4 turbos allowed");
154 float turbo_max_psi = param1;
196 void Engine::SetEngineOptions(
float einertia,
char etype,
float eclutch,
float ctime,
float stime,
float pstime,
float irpm,
float srpm,
float maximix,
float minimix,
float ebraking)
237 else if (etype ==
'e')
286 float turbotorque = 0.0f;
287 float turboInertia = 0.000003f;
311 float turbotorque = 0.0f;
312 float turboBOVtorque = 0.0f;
359 turboInertia = turboInertia * 0.7;
361 turboInertia = turboInertia * 1.3;
370 turbotorque += (turbotorque * 2.5);
425 float totaltorque = 0.0f;
586 else if (m_cur_engine_rpm < m_engine_shiftup_rpm && m_engine_shiftup_rpm > declutchRPM)
602 float torqueDiff = std::min(engineTorque, std::abs(reTorque));
603 float clutch = torqueDiff / reTorque;
617 if (hdir != Vector3::ZERO)
649 float avgRPM50 = 0.0f;
650 float avgRPM200 = 0.0f;
651 float avgAcc50 = 0.0f;
652 float avgAcc200 = 0.0f;
653 float avgBrake50 = 0.0f;
654 float avgBrake200 = 0.0f;
656 for (
unsigned int i = 0; i <
m_accs.size(); i++)
670 avgRPM50 /= std::min(
m_rpms.size(), (std::deque<float>::size_type)50);
671 avgAcc50 /= std::min(
m_accs.size(), (std::deque<float>::size_type)50);
672 avgBrake50 /= std::min(
m_brakes.size(), (std::deque<float>::size_type)50);
674 avgRPM200 /=
m_rpms.size();
675 avgAcc200 /=
m_accs.size();
678 if (avgAcc50 > 0.8f || avgAcc200 > 0.8f || avgBrake50 > 0.8f || avgBrake200 > 0.8f)
682 else if (acc < 0.5f && avgAcc50 < 0.5f && avgAcc200 < 0.5f && m_actor->ar_brake < 0.5f && avgBrake50 < 0.5f && avgBrake200 < 0.5)
753 if (newGear < m_cur_gear && m_kickdown_delay_counter > 0)
885 void Engine::pushNetworkState(
float rpm,
float acc,
float clutch,
int gear,
bool running,
bool contact,
char automode,
char autoselect)
897 if (autoselect != -1)
974 rpmRatio = std::max(0.0f, rpmRatio);
975 rpmRatio = std::min(rpmRatio, 1.0f);
977 float crankfactor = 5.0f * rpmRatio;
1190 val = std::max(0.0f, val);
1202 float atValue = 0.0f;
1222 float tqValue = 1.0f;
1245 if (crankfactor < 0.9f)
1250 else if (crankfactor < 1.0f)
1253 return 10.0f * (1.0f - crankfactor);
1268 float acclModifier = 0.0f;
1271 acclModifier += 0.25f;
1275 acclModifier += 0.50f;
1277 accl *= acclModifier;
1283 float brakeModifier = 0.0f;
1286 brakeModifier += 0.25f;
1290 brakeModifier += 0.50f;
1292 brake *= brakeModifier;
1305 if (this->hasContact() && !this->
isRunning() && (accl > 0 || brake > 0))
1331 if (velocity < 1.0f && brake > 0.5f && accl < 0.5f && this->
getGear() > 0)
1343 else if (velocity > -1.0f && brake < 0.5f && accl > 0.5f && this->
getGear() < 0)
1405 float clutchModifier = 0.0f;
1408 clutchModifier += 0.25f;
1412 clutchModifier += 0.50f;
1414 clutch *= clutchModifier;
1448 for (
int i = 1; i < 19; i++)
1460 bool gear_changed =
false;
1462 int curgear = this->
getGear();
1464 int gearoffset = std::max(0, curgear - curgearrange * 6);
1472 gear_changed =
true;
1477 gear_changed =
true;
1482 gear_changed =
true;
1487 gear_changed =
true;
1516 else if (curgear > 0 && curgear < 19)
1521 if (gear_changed || curgear == 0)
1537 for (
int i = 1; i < 19 && !found; i++)
1548 for (
int i = 1; i < 7 && !found; i++)
1552 this->
shiftTo(i + curgearrange * 6);
float ar_avg_wheel_speed
Physics state; avg wheel speed in m/s.
void toggleContact()
Ignition.
void autoShiftSet(int mode)
bool m_contact
Ignition switch is in ON/RUN position.
float m_cur_engine_torque
Engine.
void setGearRange(int v)
low level gear changing (bypasses shifting logic)
float m_turbo_wg_threshold_n
float getGearRatio(int pos)
-1=R, 0=N, 1... ('engine' attrs #5[R],#6[N],#7[1]...)
void SetEngineOptions(float einertia, char etype, float eclutch, float ctime, float stime, float pstime, float irpm, float srpm, float maximix, float minimix, float ebraking)
void setGear(int v)
low level gear changing (bypasses shifting logic)
Ogre::Vector3 GetCameraDir()
ActorInstanceID_t ar_instance_id
Static attr; session-unique ID.
float m_cur_turbo_rpm[MAXTURBO]
Truck file format(technical spec)
SimGearboxMode getAutoMode()
TorqueCurve * m_torque_curve
bool m_engine_is_priming
Engine.
void TRIGGER_EVENT_ASYNC(scriptEvents type, int arg1, int arg2ex=0, int arg3ex=0, int arg4ex=0, std::string arg5ex="", std::string arg6ex="", std::string arg7ex="", std::string arg8ex="")
Asynchronously (via MSG_SIM_SCRIPT_EVENT_TRIGGERED) invoke script function eventCallbackEx(),...
Ogre::Real ar_brake
Physics state; braking intensity.
int m_engine_turbo_mode
Engine attribute.
float m_min_idle_mixture
Minimum throttle to maintain the idle RPM ('engoption' attr #10)
float m_braking_torque
Engine attribute.
void RequestUpdateHudFeatures()
bool m_turbo_has_wastegate
System integration layer; inspired by OgreBites::ApplicationContext.
void putMessage(MessageArea area, MessageType type, std::string const &msg, std::string icon="")
float m_turbo_inertia_factor
void shift(int val)
Changes gear by a relative offset. Plays sounds.
float m_min_wastegate_psi
This class loads and processes a torque curve for a vehicle.
float m_engine_stall_rpm
('engoption' attr #7)
float approx_exp(const float x)
float m_one_third_rpm_range
void UpdateEngine(float dt, int doUpdate)
void pushNetworkState(float engine_rpm, float acc, float clutch, int gear, bool running, bool contact, char auto_mode, char auto_select=-1)
float m_turbo_bov_rpm[MAXTURBO]
void setAutoMode(SimGearboxMode mode)
void shiftTo(int val)
Changes gear to given value. Plays sounds.
float m_max_idle_mixture
Maximum throttle to maintain the idle RPM ('engoption' attr #9)
@ SE_TRUCK_ENGINE_DIED
triggered when the vehicle's engine dies (from underrev, water, etc), the argument refers to the acto...
float m_shift_time
Time (in seconds) that it takes to shift ('engoption' attr #4)
float m_turbo_wg_threshold_p
float m_cur_engine_rpm
Engine.
float m_ref_wheel_revolutions
Gears; estimated wheel revolutions based on current vehicle speed along the longi....
@ MANUAL_STICK
Fully manual: stick shift.
void setWheelSpin(float rpm)
float m_antilag_power_factor
void offStart()
Quick start of vehicle engine.
std::string ToLocalizedString(SimGearboxMode e)
int m_kickdown_delay_counter
float m_clutch_time
Time (in seconds) the clutch takes to apply ('engoption' attr #5)
static const float RAD_PER_SEC_TO_RPM
Convert radian/second to RPM (60/2*PI)
std::deque< float > m_accs
CVar * io_arcade_controls
void updateShifts()
Changes gears. Plays sounds.
std::deque< float > m_rpms
int getNumGearsRanges() const
bool m_engine_has_turbo
Engine attribute.
#define SOUND_START(_ACTOR_, _TRIG_)
void autoSetAcc(float val)
float m_diff_ratio
Global gear ratio ('engine' attr #4)
float m_engine_torque
Torque in N/m ('engine' attr #3)
@ SEMI_AUTO
Manual shift with auto clutch.
float m_cur_wheel_revolutions
Gears; measured wheel revolutions.
std::deque< float > m_brakes
void setHydroPump(float work)
void setTCaseRatio(float ratio)
Set current transfer case gear (reduction) ratio.
Ogre::Vector3 getDirection()
average actor velocity, calculated using the actor positions of the last two frames
#define SOUND_STOP(_ACTOR_, _TRIG_)
float m_engine_idle_rpm
('engoption' attr #8)
char m_engine_type
't' = truck (default), 'c' = car ('engoption' attr #2)
float m_turbo_engine_rpm_operation
float m_engine_max_rpm
Shift up RPM ('engine' attr #2)
@ MANUAL
Fully manual: sequential shift.
void stopEngine()
stall engine
#define SOUND_MODULATE(_ACTOR_, _MOD_, _VALUE_)
float m_engine_inertia
('engoption' attr #1)
int m_upshift_delay_counter
float m_engine_addi_torque[MAXTURBO]
bool m_engine_is_electric
Engine attribute.
float m_tcase_ratio
Engine.
void UpdateInputEvents(float dt)
int m_cur_gear
Gears; Current gear {-1 = reverse, 0 = neutral, 1...21 = forward}.
bool m_starter
Ignition switch is in START position.
#define SOUND_PLAY_ONCE(_ACTOR_, _TRIG_)
Ogre::Real getEngineTorque(Ogre::Real rpm)
Returns the calculated engine torque based on the given RPM, interpolating the torque curve spline.
SimGearboxMode m_auto_mode
bool m_engine_is_running
Engine state.
InputEngine * GetInputEngine()
float getAccToHoldRPM()
estimate required throttle input to hold the current rpm
float m_cur_clutch_torque
@ MANUAL_RANGES
Fully manual: stick shift with ranges.
float m_hydropump_state
Engine.
std::vector< float > m_gear_ratios
[R|N|1|...] ('engine' attrs #4[global],#5[R],#6[N],#7[1]...)
@ CONSOLE_MSGTYPE_INFO
Generic message.
wheel_t ar_wheels[MAX_WHEELS]
float m_clutch_force
('engoption' attr #3)
bool m_engine_has_air
Engine attribute.
int m_cur_gear_range
Gears.
Ogre::Vector3 getGForces()
void SetTurboOptions(int type, float tinertiaFactor, int nturbos, float param1, float param2, float param3, float param4, float param5, float param6, float param7, float param8, float param9, float param10, float param11)
void setManualClutch(float val)
void startEngine()
Quick engine start. Plays sounds.
float m_post_shift_time
Time (in seconds) until full torque is transferred ('engoption' attr #6)
float m_antilag_rand_chance
float m_engine_shiftup_rpm
Shift down RPM ('engine' attr #1)
int m_num_gears
Num. forward gears.