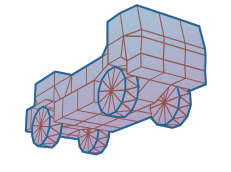 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
31 #include <OgreVector3.h>
100 void SetSpringRate(
float rate ) {
m_spring_rate = fabs( rate); }
101 void SetBreakForce(
float breakRate ) {
m_break_force = fabs( breakRate); }
102 void SetAttachmentRate(
float rate ) {
m_attach_rate = fabs( rate); }
152 static Ogre::Real
getLenTo(
const RailGroup* group,
const Ogre::Vector3& point );
166 static Ogre::Real
getLenTo(
const beam_t* beam,
const Ogre::Vector3& point );
Global forward declarations.
Ogre::Vector3 m_ideal_position
Where the node SHOULD be. (World, m)
void AttachToRail(RailGroup *toAttach)
float m_initial_threshold
Distance from beam calculating corrective forces (m)
void ResetSlideNode()
Move back to initial rail, reset 'broken' flag and recalculate closest position.
bool sn_attach_foreign
Attach/detach to rails only on other vehicles.
A series of RailSegment-s for SlideNode to slide along. Can be closed in a loop.
float m_spring_rate
Spring rate holding node to rail (N/m)
RailSegment * CheckCurSlideSegment(Ogre::Vector3 const &point)
Check if the slidenode should skip to a neighbour rail segment.
bool sn_attach_self
Attach/detach to rails on the current vehicle only.
float m_node_forces_ratio
Ratio of length along the slide beam where the virtual node is "0.0f = p1, 1.0f = p2".
RailGroup * m_cur_railgroup
Current Rail group, used for attachments.
float m_cur_threshold
Distance away from beam before corrective forces begin to act on the node (m)
A single beam in a chain.
Simulation: An edge in the softbody structure.
void UpdatePosition()
Checks for current rail segment and updates ideal position of the node.
RailGroup * m_initial_railgroup
Initial Rail group on spawn.
int GetSlideNodeId()
Returns the node index of the slide node.
float m_attach_rate
How fast the cur threshold changes when attaching (i.e. how long it will take for springs to fully at...
std::vector< RailSegment > rg_segments
static Ogre::Real getLenTo(const RailGroup *group, const Ogre::Vector3 &point)
RailSegment * m_cur_rail_seg
Current rail segment we are sliding on.
Physics: A vertex in the softbody structure.
void ResetPositions()
Recalculates the closest position on current RailGroup.
bool sn_slide_broken
The slidenode was pulled away from the rail.
SlideNode(node_t *sliding_node, RailGroup *rail)
const Ogre::Vector3 & GetSlideNodePosition() const
Ogre::Vector3 CalcCorrectiveForces()
Calculate forces between the ideal and actual position of the sliding node.
void UpdateForces(float dt)
Updates the corrective forces and applies these forces to the beam.
node_t * m_sliding_node
Pointer to node that is sliding.
int rg_id
Spawn context - matching separately defined rails with slidenodes.
float m_attach_distance
Maximum distance slide node will attach to a beam (m)
void SetDefaultRail(RailGroup *rail)
Sets rail to initially use when spawned or reset.
beam_t * m_sliding_beam
Pointer to current beam sliding on.
RailSegment * FindClosestSegment(Ogre::Vector3 const &point)
Search for closest rail segment (the one with closest node in it) in the entire RailGroup.
float m_break_force
Force at which Slide Node breaks away from the rail (N)
RailSegment(beam_t *beam)