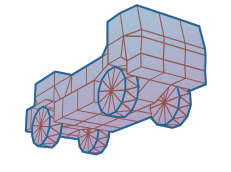 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
51 Ogre::Vector3
NearestPointOnLine(
const Ogre::Vector3& pt1,
const Ogre::Vector3& pt2,
const Ogre::Vector3& tp)
53 Ogre::Vector3 a = tp - pt1;
54 Ogre::Vector3 b = pt2 - pt1;
55 Ogre::Real len = b.normalise();
57 len = std::max(0.0f, std::min(a.dotProduct(b), len));
65 m_sliding_node(slidingNode),
67 m_initial_railgroup(slidingRail),
68 m_cur_railgroup(m_initial_railgroup),
70 m_node_forces_ratio(0.0f),
72 m_initial_threshold(0.0f),
73 m_cur_threshold(0.0f),
74 m_spring_rate(9000000),
75 m_break_force(std::numeric_limits<
Ogre::Real>::infinity()),
78 m_attach_distance(0.1f),
79 sn_attach_foreign(false),
80 sn_attach_self(false),
81 sn_slide_broken(false)
117 size_t closest_seg = 0;
119 for (
size_t i = 1; i < this->
rg_segments.size(); ++i)
122 if (dist_sq < closest_dist_sq)
124 closest_dist_sq = dist_sq;
140 if (dist_sq < closest_dist_sq)
143 closest_dist_sq = dist_sq;
150 if (dist_sq < closest_dist_sq)
181 const Ogre::Real bLen = b.normalise();
187 const Ogre::Real len = std::max(0.0f, std::min(aDotBUnit, bLen));
213 return std::numeric_limits<Ogre::Real>::infinity();
221 return std::numeric_limits<Ogre::Real>::infinity();
229 return std::numeric_limits<Ogre::Real>::infinity();
252 const Ogre::Real beamLen = std::max(0.0f, force.normalise() -
m_cur_threshold);
254 return (force * forceLen);
#define ROR_ASSERT(_EXPR)
Ogre::Vector3 m_ideal_position
Where the node SHOULD be. (World, m)
Ogre::Vector3 AbsPosition
absolute position in the world (shaky)
float m_initial_threshold
Distance from beam calculating corrective forces (m)
A series of RailSegment-s for SlideNode to slide along. Can be closed in a loop.
float m_spring_rate
Spring rate holding node to rail (N/m)
RailSegment * CheckCurSlideSegment(Ogre::Vector3 const &point)
Check if the slidenode should skip to a neighbour rail segment.
float m_node_forces_ratio
Ratio of length along the slide beam where the virtual node is "0.0f = p1, 1.0f = p2".
RailGroup * m_cur_railgroup
Current Rail group, used for attachments.
Ogre::Vector3 NearestPointOnLine(const Ogre::Vector3 &pt1, const Ogre::Vector3 &pt2, const Ogre::Vector3 &tp)
Find the point on a line defined by pt1 and pt2 that is nearest to a given point tp tp /| A / | / | /...
float m_cur_threshold
Distance away from beam before corrective forces begin to act on the node (m)
A single beam in a chain.
Simulation: An edge in the softbody structure.
void UpdatePosition()
Checks for current rail segment and updates ideal position of the node.
Core data structures for simulation; Everything affected by by either physics, network or user intera...
int GetSlideNodeId()
Returns the node index of the slide node.
float m_attach_rate
How fast the cur threshold changes when attaching (i.e. how long it will take for springs to fully at...
std::vector< RailSegment > rg_segments
static Ogre::Real getLenTo(const RailGroup *group, const Ogre::Vector3 &point)
NodeNum_t pos
This node's index in Actor::ar_nodes array.
RailSegment * m_cur_rail_seg
Current rail segment we are sliding on.
Central state/object manager and communications hub.
Physics: A vertex in the softbody structure.
void ResetPositions()
Recalculates the closest position on current RailGroup.
bool sn_slide_broken
The slidenode was pulled away from the rail.
SlideNode(node_t *sliding_node, RailGroup *rail)
const Ogre::Vector3 & GetSlideNodePosition() const
Ogre::Vector3 CalcCorrectiveForces()
Calculate forces between the ideal and actual position of the sliding node.
void UpdateForces(float dt)
Updates the corrective forces and applies these forces to the beam.
node_t * m_sliding_node
Pointer to node that is sliding.
beam_t * m_sliding_beam
Pointer to current beam sliding on.
RailSegment * FindClosestSegment(Ogre::Vector3 const &point)
Search for closest rail segment (the one with closest node in it) in the entire RailGroup.
float m_break_force
Force at which Slide Node breaks away from the rail (N)