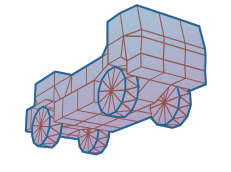 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
46 inline float computeVolume(Ogre::Vector3 o, Ogre::Vector3 a, Ogre::Vector3 b, Ogre::Vector3 c);
49 Ogre::Vector3
computePressureForceSub(Ogre::Vector3 a, Ogre::Vector3 b, Ogre::Vector3 c, Ogre::Vector3 vel,
int type);
52 Ogre::Vector3
computePressureForce(Ogre::Vector3 a, Ogre::Vector3 b, Ogre::Vector3 c, Ogre::Vector3 vel,
int type);
void computeNodeForce(node_t *a, node_t *b, node_t *c, bool doUpdate, int type)
float computeVolume(Ogre::Vector3 o, Ogre::Vector3 a, Ogre::Vector3 b, Ogre::Vector3 c)
Buoyance(DustPool *splash, DustPool *ripple)
Ogre::Vector3 computePressureForce(Ogre::Vector3 a, Ogre::Vector3 b, Ogre::Vector3 c, Ogre::Vector3 vel, int type)
Ogre::Vector3 computePressureForceSub(Ogre::Vector3 a, Ogre::Vector3 b, Ogre::Vector3 c, Ogre::Vector3 vel, int type)
Central state/object manager and communications hub.
Physics: A vertex in the softbody structure.