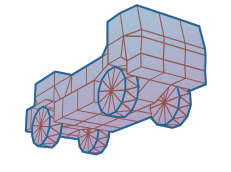 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
50 void query(
const Ogre::Vector3& vec1,
const Ogre::Vector3& vec2,
const Ogre::Vector3& vec3,
const float enlargeBB);
76 Ogre::Vector3
m_bbmin = Ogre::Vector3::ZERO;
77 Ogre::Vector3
m_bbmax = Ogre::Vector3::ZERO;
80 void queryrec(
int kdindex,
int axis);
82 void partintwo(
const int start,
const int median,
const int end,
const int axis,
float& minex,
float& maxex);
std::vector< pointid_t > hit_pointid_list
int RefelemID_t
index to PointColDetector::m_ref_list, use RoR::REFELEMID_INVALID as empty value.
static const NodeNum_t NODENUM_INVALID
int PointidID_t
index to PointColDetector::hit_pointid_list, use RoR::POINTIDID_INVALID as empty value.
std::vector< kdnode_t > m_kdtree
PointColDetector(ActorPtr actor)
void UpdateInterPoint(bool ignorestate=false)
std::vector< refelem_t > m_ref_list
int ActorInstanceID_t
Unique sequentially generated ID of an actor in session. Use ActorManager::GetActorById()
uint16_t NodeNum_t
Node position within Actor::ar_nodes; use RoR::NODENUM_INVALID as empty value.
ActorInstanceID_t actorid
static const PointidID_t POINTIDID_INVALID
static const ActorInstanceID_t ACTORINSTANCEID_INVALID
void setPoint(const Ogre::Vector3 pos)
Central state/object manager and communications hub.
void refresh_node_positions()
std::array< float, 3 > point
std::vector< PointidID_t > hit_list
void queryrec(int kdindex, int axis)
static const RefelemID_t REFELEMID_INVALID
std::unordered_set< ActorInstanceID_t > hit_list_actorset
void UpdateIntraPoint(bool contactables=false)
void update_structures_for_contacters(bool ignoreinternal)
void partintwo(const int start, const int median, const int end, const int axis, float &minex, float &maxex)
std::vector< ActorInstanceID_t > m_collision_partners
IntraPoint: always just owning actor; InterPoint: all colliding actors.
void query(const Ogre::Vector3 &vec1, const Ogre::Vector3 &vec2, const Ogre::Vector3 &vec3, const float enlargeBB)
void build_kdtree_incr(int axis, int index)