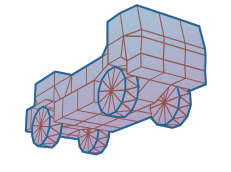 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
119 Str<200> msg; msg <<
_L(
"Terrain not found: ") << filename_part;
130 std::string
const& filename = terrn_entry->
fname;
133 Ogre::DataStreamPtr stream = Ogre::ResourceGroupManager::getSingleton().openResource(filename);
134 LOG(
" ===== LOADING TERRAIN " + filename);
141 catch (Ogre::Exception& e)
212 rq.
asr_rotation = Ogre::Quaternion(Ogre::Degree(180) - player_character->
getRotation(), Ogre::Vector3::UNIT_Y);
273 bool fresh_actor_seat_player =
false;
276 if (def->slide_nodes_connect_instantly)
286 fresh_actor_seat_player =
true;
300 fresh_actor_seat_player =
true;
343 fresh_actor_seat_player =
true;
347 if (fresh_actor_seat_player)
393 Str<500> msg; msg <<
"Cannot reload vehicle; file '" << actor->
ar_filename <<
"' not found in ModCache.";
401 srq->
asr_rotation = Ogre::Quaternion(Ogre::Degree(270) - Ogre::Radian(actor->
getRotation()), Ogre::Vector3::UNIT_Y);
448 actorx->hookToggle();
472 if (prev_player_actor && prev_player_actor->
ar_dashboard)
482 if (prev_player_actor)
496 if (prev_player_actor)
507 float rotation = prev_player_actor->
getRotation() - Ogre::Math::HALF_PI;
508 Ogre::Vector3 position = prev_player_actor->
getPosition();
512 Ogre::Vector3 l = position - 2.0f * prev_player_actor->
GetCameraRoll();
513 Ogre::Vector3 r = position + 2.0f * prev_player_actor->
GetCameraRoll();
516 position = std::abs(r.y - r_h) * 1.2f < std::abs(l.y - l_h) ? r : l;
521 if (player_character)
524 player_character->
setRotation(Ogre::Radian(rotation));
551 if (player_character)
587 ev_src_instance_name, box_name);
647 for (
int i = 0; i < actor->ar_num_nodes; i++)
685 bool spawn_now =
false;
737 if (!entry->
guid.empty())
752 if (!default_skin_entry)
757 if (default_skin_entry && num_skins == 1)
765 default_skin_entry->
dname =
"Default skin";
766 default_skin_entry->
description =
"Original, unmodified skin";
825 float spawn_rot = 0.0f;
866 for (
int i = 0; i < 100; i++)
893 std::vector<ActorPtr> actorsToBeamUp;
897 float src_agl = std::numeric_limits<float>::max();
898 float dst_agl = std::numeric_limits<float>::max();
899 for (
ActorPtr& actor : actorsToBeamUp)
901 for (
int i = 0; i < actor->ar_num_nodes; i++)
903 Ogre::Vector3 pos = actor->ar_nodes[i].AbsPosition;
910 translation += Ogre::Vector3::UNIT_Y * (std::max(0.0f, src_agl) - dst_agl);
912 for (
ActorPtr& actor : actorsToBeamUp)
914 actor->resetPosition(actor->ar_nodes[0].AbsPosition + translation,
false);
1007 Ogre::Vector3 position(Ogre::Vector3::ZERO);
1008 Ogre::Radian rotation(0);
1021 LOG(
"Position: " + pos +
", " + rot);
1049 float mindist = 1000.0;
1053 if (!actor->ar_driveable)
1057 LOG(
"cinecam missing, cannot enter the actor!");
1063 len = actor->ar_nodes[actor->ar_cinecam_node[0]].AbsPosition.distance(this->
GetPlayerCharacter()->getPosition() + Ogre::Vector3(0.0, 2.0, 0.0));
1068 nearest_actor = actor;
1079 if (this->
GetPlayerActor()->ar_nodes[0].Velocity.squaredLength() < 1.0f ||
1081 this->GetPlayerActor()->ar_driveable ==
AI)
1126 float min_squared_distance = std::numeric_limits<float>::max();
1129 float squared_distance = position.squaredDistance(actor->ar_nodes[0].AbsPosition);
1130 if (squared_distance < min_squared_distance)
1132 min_squared_distance = squared_distance;
1133 nearest_actor = actor;
1138 if (nearest_actor !=
nullptr &&
1155 if (
m_timer.getMilliseconds() > 1000)
1164 if (waypoint.
speed < 5)
1166 waypoint.
speed = -1;
1197 float time_factor = 1.0f;
1201 time_factor = 1000.0f;
1205 time_factor = 10000.0f;
1209 time_factor = -1000.0f;
1213 time_factor = -10000.0f;
1228 #endif // USE_CAELUM
1408 if (actor->ar_rescuer_flag)
1413 if (rescuer ==
nullptr)
1533 else if (ref < value)
1549 float commandrate = 4.0;
1552 float sum_steer = -tmp_left + tmp_right;
1560 float sum_pitch = tmp_pitch_down - tmp_pitch_up;
1566 float sum_rudder = tmp_rudder_left - tmp_rudder_right;
1666 if (tmp_throttle > 0)
1753 float sum_steer = (tmp_steer_left - tmp_steer_right) * dt;
1755 if (fabs(sum_steer) > 0 && stime <= 0)
1765 sum_steer = (tmp_steer_left - tmp_steer_right);
1789 #ifdef USE_ANGELSCRIPT
1792 #endif // USE_ANGELSCRIPT
1812 float sum = -std::max(tmp_left_digital, tmp_left_analog) + std::max(tmp_right_digital, tmp_right_analog);
@ MSG_EDI_MODIFY_PROJECT_REQUESTED
Payload = RoR::UpdateProjectRequest* (owner)
void setHighBeamsVisible(bool val)
#define ROR_ASSERT(_EXPR)
Game state manager and message-queue provider.
GUI::VehicleInfoTPanel VehicleInfoTPanel
void UpdateInputEvents(float dt)
static const int MAX_COMMANDS
maximum number of commands per actor
Ogre::Radian getRotation() const
void SetDebugView(DebugViewType dv)
void LoadAssetPack(CacheEntryPtr &t_dest, Ogre::String const &assetpack_filename)
Adds asset pack to the requesting cache entry's resource group.
void setPosition(Ogre::Vector3 position)
float getMinCameraRadius()
float getThrottle(float thrtl, float dt)
VehicleAIPtr ar_vehicle_ai
Ogre::String dname
name parsed from the file
float getWheelSpeed() const
@ NETWORK
Remote controlled.
@ MSG_SIM_MODIFY_ACTOR_REQUESTED
Payload = RoR::ActorModifyRequest* (owner)
void ModifyActor(ActorModifyRequest &rq)
bool ar_physics_paused
Sim state.
@ MSG_EDI_RELOAD_BUNDLE_REQUESTED
Payload = RoR::CacheEntryPtr* (owner)
@ TRUCK
its a truck (or other land vehicle)
std::string ar_filename
Attribute; filled at spawn.
std::list< std::string > assetpack_files
Ogre::Vector3 getMaxTerrainSize()
GUI::FrictionSettings FrictionSettings
void toggleAxleDiffMode()
GameContextSB & GetSimDataBuffer()
static const int MAX_CLIGHTS
See RoRnet::Lightmask and enum events in InputEngine.h.
CacheEntryPtr m_last_skin_selection
void setHeadlightsVisible(bool val)
void UndoRemoteActorCoupling(ActorPtr actor)
CameraManager * GetCameraManager()
@ CONFIG_FILE
'Preselected vehicle' in RoR.cfg or command line
RefCountingObjectPtr< CacheEntry > CacheEntryPtr
Ogre::Vector3 AbsPosition
absolute position in the world (shaky)
GUIManager * GetGuiManager()
CVar * sim_soft_reset_mode
std::shared_ptr< RoR::SkinDef > skin_def
Cached skin info, added on first use or during cache rebuild.
Estabilishing a physics linkage between 2 actors modifies a global linkage table and triggers immedia...
ActorPtrVec ar_linked_actors
BEWARE: Includes indirect links, see DetermineLinkedActors(); Other actors linked using 'hooks/ties/r...
CharacterFactory m_character_factory
void autoShiftSet(int mode)
ActorInstanceID_t ar_instance_id
Static attr; session-unique ID.
float getSurfaceHeightBelow(float x, float z, float height)
void RemoveGfxActor(RoR::GfxActor *gfx_actor)
Ogre::String m_last_section_config
AppContext * GetAppContext()
static const NodeNum_t NODENUM_INVALID
bool getFogLightsVisible() const
void toggleWheelDiffMode()
Truck file format(technical spec)
@ MSG_SIM_UNPAUSE_REQUESTED
SkyManager * getSkyManager()
void UpdateCommonInputEvents(float dt)
ActorLinkingRequestType alr_type
Character * GetPlayerCharacter()
void NotifyVehicleChanged(ActorPtr new_vehicle)
void TRIGGER_EVENT_ASYNC(scriptEvents type, int arg1, int arg2ex=0, int arg3ex=0, int arg4ex=0, std::string arg5ex="", std::string arg6ex="", std::string arg7ex="", std::string arg8ex="")
Asynchronously (via MSG_SIM_SCRIPT_EVENT_TRIGGERED) invoke script function eventCallbackEx(),...
Ogre::Real ar_brake
Physics state; braking intensity.
ActorPtr FindActorInsideBox(Collisions *collisions, const Ogre::String &inst, const Ogre::String &box)
void OnLoaderGuiApply(RoR::LoaderType type, CacheEntryPtr entry, std::string sectionconfig)
GUI callback.
OverlayWrapper * GetOverlayWrapper()
RoR::TuneupDefPtr tuneup_def
Cached tuning info, added on first use or during cache rebuild.
Character * CreateLocalCharacter()
void LogFormat(const char *format,...)
Improved logging utility. Uses fixed 2Kb buffer.
CacheEntryPtr m_last_tuneup_selection
Ogre::Vector3 getPosition(const Ogre::String &inst, const Ogre::String &box)
void toggleCustomParticles()
bool ar_toggle_ropes
Sim state.
System integration layer; inspired by OgreBites::ApplicationContext.
void putMessage(MessageArea area, MessageType type, std::string const &msg, std::string icon="")
GUI::GameControls GameControls
void TeleportPlayer(float x, float z)
bool ar_trailer_parking_brake
const ActorPtr & FetchPreviousVehicleOnList(ActorPtr player, ActorPtr prev_player)
TuneupDefPtr asr_working_tuneup
Only filled when editing tuneup via Tuning menu.
void SetAutoMode(RoR::SimGearboxMode mode)
CVar * cli_preset_spawn_rot
ActorManager m_actor_manager
@ TUNEUP_USE_ADDONPART_SET
'subject' is addonpart filename.
GUI::GameSettings GameSettings
int ar_aerial_flap
Sim state; state of aircraft flaps (values: 0-5)
void setRudder(float val)
@ LOCAL_SIMULATED
simulated (local) actor
std::vector< Ogre::String > sectionconfigs
Ogre::SceneNode * GetCameraNode()
std::string asr_filename
Can be in "Bundle-qualified" format, i.e. "mybundle.zip:myactor.truck".
@ NOT_DRIVEABLE
not drivable at all
void Show(LoaderType type, std::string const &filter_guid="", CacheEntryPtr advertised_entry=nullptr)
void smoothValue(float &ref, float value, float rate)
void displayAxleDiffMode()
Cycles through the available inter axle diff modes.
ActorPtr simbuf_player_actor
DashBoardManager * ar_dashboard
void tractioncontrolToggle()
@ MSG_APP_DISPLAY_WINDOWED_REQUESTED
@ SAVEGAME
User spawned and part of a savegame.
@ SE_TRUCK_EXIT
triggered when switching from vehicle mode to person mode, the argument refers to the actor ID of the...
CVar * diag_preset_spawn_rot
bool ar_forward_commands
Sim state.
float ar_left_mirror_angle
Sim state; rear view mirror angle.
virtual void toggleReverse()=0
void SetVideoCamState(VideoCamState state)
float ar_right_mirror_angle
Sim state; rear view mirror angle.
GUI::RepositorySelector RepositorySelector
virtual void flipStart()=0
bool LoadTerrn2(Terrn2Def &def, Ogre::DataStreamPtr &ds)
CVar * sim_tuning_enabled
@ MSG_APP_DISPLAY_FULLSCREEN_REQUESTED
RoR::ForceFeedback & GetForceFeedback()
ActorPtr SpawnActor(ActorSpawnRequest &rq)
float GetHeightAt(float x, float z)
ActorInstanceID_t amr_actor
void UpdateGlobalInputEvents()
@ VCSTATE_ENABLED_OFFLINE
VideoCamState GetVideoCamState() const
AeroEngine * ar_aeroengines[MAX_AEROENGINES]
Message * m_msg_chain_end
bool isActive()
Returns the status of the AI.
Ogre::Camera * GetCamera()
Screwprop * ar_screwprops[MAX_SCREWPROPS]
void UpdateSleepingState(ActorPtr player_actor, float dt)
void ChainMessage(Message m)
Add to last pushed message's chain.
ActorSpawnRequest m_current_selection
Context of the loader UI.
@ CAELUM
Caelum (best looking, slower)
float getMinHeight(bool skip_virtual_nodes=true)
GUI::MultiplayerSelector MultiplayerSelector
int ar_airbrake_intensity
Physics state; values 0-5.
CacheEntryPtr FindEntryByFilename(RoR::LoaderType type, bool partial, const std::string &_filename_maybe_bundlequalified)
Returns NULL if none found; "Bundle-qualified" format also specifies the ZIP/directory in modcache,...
void SetVisible(bool visible)
bool LoadTerrain(std::string const &filename_part)
@ MSG_SIM_SEAT_PLAYER_REQUESTED
Payload = RoR::ActorPtr (owner) | nullptr.
A database of user-installed content alias 'mods' (vehicles, terrains...)
ActorPtr m_player_actor
Actor (vehicle or machine) mounted and controlled by player.
collision_box_t * getBox(const Ogre::String &inst, const Ogre::String &box)
CacheEntryPtr asr_tuneup_entry
Only filled when user selected a saved/downloaded .tuneup mod in SelectorUI.
void ChangePlayerActor(ActorPtr actor)
void resolveCollisions(Ogre::Vector3 direction)
Moves the actor at most 'direction.length()' meters towards 'direction' to resolve any collisions.
void setVisible3d(bool visibility)
CacheEntryPtr asr_cache_entry
Optional, overrides 'asr_filename' and 'asr_cache_entry_num'.
TyrePressure & getTyrePressure()
void DeleteActor(ActorPtr actor)
void PushMessage(Message m)
Doesn't guarantee order! Use ChainMessage() if order matters.
std::vector< ActorPtr > GetLocalActors()
void UpdateInputEvents(float dt)
Ogre::String getSectionConfig()
const char * ToCStr() const
@ LOCAL_SLEEPING
sleeping (local) actor
void setFogLightsVisible(bool val)
void SyncReset(bool reset_position)
this one should be called only synchronously (without physics running in background)
void antilockbrakeToggle()
bool ar_hydro_speed_coupling
bool getHeadlightsVisible() const
@ SE_TRUCK_ENTER
triggered when switching from person mode to vehicle mode, the argument refers to the actor ID of the...
CmdKeyArray ar_command_key
BEWARE: commandkeys are indexed 1-MAX_COMMANDS!
Collisions * GetCollisions()
Ogre::Vector3 getPosition()
CacheEntryPtr m_last_cache_selection
Vehicle/load.
void hookToggle(int group=-1, ActorLinkingRequestType mode=ActorLinkingRequestType::HOOK_TOGGLE, NodeNum_t mousenode=NODENUM_INVALID, ActorInstanceID_t forceunlock_filter=ACTORINSTANCEID_INVALID)
void setTimeMultiplier(const Ogre::Real &TimeMultiplier)
Set time multiplier.
void SetPrevPlayerActor(ActorPtr actor)
@ MSG_GUI_OPEN_SELECTOR_REQUESTED
Payload = LoaderType* (owner), Description = GUID | empty.
#define SOUND_START(_ACTOR_, _TRIG_)
LoaderType
< Search mode for ModCache::Query() & Operation mode for GUI::MainSelector
@ MSG_GUI_REFRESH_TUNING_MENU_REQUESTED
void showDashboardOverlays(bool show, ActorPtr actor)
ActorPtr mpr_target_actor
bool ar_import_commands
Sim state.
void setThrottle(float val)
RoRnet::UserInfo GetLocalUserData()
GameContext * GetGameContext()
@ MSG_APP_SCREENSHOT_REQUESTED
Ogre::Vector3 getPosition()
@ AIRPLANE
its an airplane
bool getSideLightsVisible() const
void AnalyzeFlexbodies()
populates the combobox
float ar_sleep_counter
Sim state; idle time counter.
std::vector< Message > chain
Posted after the message is processed.
float getHeightAboveGroundBelow(float height, bool skip_virtual_nodes=true)
#define SOUND_STOP(_ACTOR_, _TRIG_)
@ TERRN_DEF
Preloaded with terrain.
bool asr_terrn_machine
This is a fixed machinery.
CacheEntryPtr asr_skin_entry
float getSurfaceHeight(float x, float z)
std::shared_ptr< rapidjson::Document > amr_saved_state
void toggleSlideNodeLock()
GUI::TopMenubar TopMenubar
@ MSG_EDI_ENTER_TERRN_EDITOR_REQUESTED
Ogre::Vector3 GetCameraRoll()
Character * GetLocalCharacter()
void toggleTransferCaseMode()
void parkingbrakeToggle()
CVar * diag_preset_spawn_pos
void DeleteActorInternal(ActorPtr actor)
Do not call directly; use GameContext::DeleteActor()
bool ar_is_police
Gfx/sfx attr.
CacheSystem * GetCacheSystem()
@ SKYX
SkyX (best looking, slower)
CVar * gfx_sky_time_speed
float ar_elevator
Sim state; aerial controller.
void UpdateAirplaneInputEvents(float dt)
int32_t colournum
colour set by server
GUI::FlexbodyDebug FlexbodyDebug
or anywhere else will not be considered a but parsed as regular data ! Each line is treated as values separated by separators Possible i e animators Multiline description Single instance
char username[RORNET_MAX_USERNAME_LEN]
the nickname of the user (UTF-8)
bool ar_toggle_ties
Sim state.
RoR::LoaderType cqy_filter_type
void toggleBlinkType(BlinkType blink)
void UpdateEnvMap(Ogre::Vector3 center, GfxActor *gfx_actor, bool full=false)
const ActorPtr & FetchPrevVehicleOnList()
void LoadResource(CacheEntryPtr &t)
Loads the associated resource bundle if not already done.
void OnLoaderGuiCancel()
GUI callback.
@ MSG_SIM_ACTOR_LINKING_REQUESTED
Payload = RoR::ActorLinkingRequest* (owner)
Unified game event system - all requests and state changes are reported using a message.
float ar_hydro_dir_command
ActorType ar_driveable
Sim attr; marks vehicle type and features.
@ SE_TRUCK_TELEPORT
triggered when the user teleports the truck, the argument refers to the actor ID of the vehicle
@ MSG_GUI_OPEN_MENU_REQUESTED
ActorPtr m_prev_player_actor
Previous actor (vehicle or machine) mounted and controlled by player.
void UpdatePropAnimInputEvents()
CacheEntryPtr & getUsedSkinEntry()
Ogre::Vector3 getSpawnPos()
bool getHighBeamsVisible() const
SkyXManager * getSkyXManager()
void tieToggle(int group=-1, ActorLinkingRequestType mode=ActorLinkingRequestType::TIE_TOGGLE, ActorInstanceID_t forceunlock_filter=ACTORINSTANCEID_INVALID)
Ogre::UTFString asr_net_username
void ShowMessageBox(const char *title, const char *text, bool allow_close=true, const char *btn1_text="OK", const char *btn2_text=nullptr)
void SetRenderdashActive(bool active)
CVar * cli_preset_spawn_pos
Ogre::Vector3 getRotationCenter()
void toggleTransferCaseGearRatio()
@ MSG_SIM_SPAWN_ACTOR_REQUESTED
Payload = RoR::ActorSpawnRequest* (owner)
void SetActorCoupling(bool enabled, ActorPtr actor)
Ogre::Quaternion getDirection(const Ogre::String &inst, const Ogre::String &box)
InputEngine * GetInputEngine()
RefCountingObjectPtr< Actor > ActorPtr
ActorInstanceID_t alr_actor_instance_id
@ MSG_SIM_DELETE_ACTOR_REQUESTED
Payload = RoR::ActorPtr* (owner)
void UpdateSimInputEvents(float dt)
void UpdateBoatInputEvents(float dt)
void setCustomLightVisible(int number, bool visible)
virtual void setThrottle(float val)=0
bool isInside(Ogre::Vector3 pos, const Ogre::String &inst, const Ogre::String &box, float border=0)
void ShowLoaderGUI(int type, const Ogre::String &instance, const Ogre::String &box)
@ NETWORKED_HIDDEN
not simulated, not updated (remote)
RigDef::DocumentPtr FetchActorDef(RoR::ActorSpawnRequest &rq)
std::string cqy_filter_guid
Exact match (case-insensitive); leave empty to disable.
GUI::MainSelector MainSelector
float getMaxHeight(bool skip_virtual_nodes=true)
int countFlaresByType(FlareType type)
@ USER
Direct selection by user via GUI.
void RestoreSavedState(ActorPtr actor, rapidjson::Value const &j_entry)
@ CONSOLE_MSGTYPE_ACTOR
Parsing/spawn/simulation messages for actors.
DebugViewType GetDebugView() const
std::shared_ptr< Document > DocumentPtr
CVar * diag_preset_veh_enter
@ CONSOLE_MSGTYPE_INFO
Generic message.
void SpawnPreselectedActor(std::string const &preset_vehicle, std::string const &preset_veh_config)
needs Character to exist
CacheEntryPtr m_dummy_cache_selection
ActorPtr m_last_spawned_actor
Last actor spawned by user and still alive.
@ MSG_GUI_CLOSE_MENU_REQUESTED
Ogre::Vector3 asr_position
void SetVisible(bool visible)
ModifyProjectRequestType mpr_type
int ar_net_source_id
Unique ID of remote player who spawned this actor.
@ MSG_SIM_PAUSE_REQUESTED
@ NETWORKED_OK
not simulated (remote) actor
void displayWheelDiffMode()
Cycles through the available inter wheel diff modes.
void UpdateActors(ActorPtr player_actor)
float ar_aileron
Sim state; aerial controller.
Ogre::UTFString tryConvertUTF(const char *buffer)
const ActorPtr & FetchNextVehicleOnList(ActorPtr player, ActorPtr prev_player)
void setAirbrakeIntensity(float intensity)
const ActorPtr & GetPlayerActor()
@ AI
machine controlled by an Artificial Intelligence
void UpdateTruckInputEvents(float dt)
CVar * gfx_sky_time_cycle
void prepareInside(bool inside)
Prepares vehicle for in-cabin camera use.
float ar_rudder
Sim state; aerial/marine controller.
void cruisecontrolToggle()
Defined in 'gameplay/CruiseControl.cpp'.
collision_box_t * asr_spawnbox
Ogre::Quaternion asr_rotation
TuneupDefPtr & getWorkingTuneupDef()
virtual float getThrottle()=0
void setSideLightsVisible(bool val)
@ SE_GENERIC_DELETED_TRUCK
triggered when the user deletes an actor, the argument refers to the actor ID
const ActorPtr & GetActorById(ActorInstanceID_t actor_id)
size_t Query(CacheQuery &query)
const ActorPtr & FetchNextVehicleOnList()
void Log(const char *msg)
The ultimate, application-wide logging function. Adds a line (any length) in 'RoR....
ActorManager * GetActorManager()
ActorPtr FindActorByCollisionBox(std::string const &ev_src_instance_name, std::string const &box_name)
void setStr(std::string const &str)
std::vector< CacheQueryResult > cqy_results
@ MSG_APP_SHUTDOWN_REQUESTED
static const CommandkeyID_t COMMANDKEYID_INVALID
ActorPtr CreateNewActor(ActorSpawnRequest rq, RigDef::DocumentPtr def)
CommandkeyID_t GetActiveCommandKey() const
#define SOUND_TOGGLE(_ACTOR_, _TRIG_)
void setRotation(Ogre::Radian rotation)
NodeNum_t ar_cinecam_node[MAX_CAMERAS]
Sim attr; Cine-camera node indexes.
Ogre::String guid
global unique id; Type "addonpart" leaves this empty and uses addonpart_guids; Always lowercase.
bool getCustomLightVisible(int number)
void displayTransferCaseMode()
Gets the current transfer case mode name (4WD Hi, ...)
void CreatePlayerCharacter()
Terrain must be loaded.
void UpdateSkyInputEvents(float dt)
bool isBeingReset() const
std::shared_ptr< rapidjson::Document > asr_saved_state
Pushes msg MODIFY_ACTOR (type RESTORE_SAVED) after spawn.
Ogre::String fname
filename
void UpdateInputEvents(float dt)
bool HasPredefinedActors()
@ RELOAD
Full reload from filesystem, requested by user.