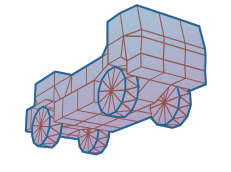 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
61 class SkyX :
public Ogre::FrameListener,
public Ogre::RenderTargetListener
74 inline RenderQueueGroups(
const Ogre::uint8& s,
const Ogre::uint8& vc,
const Ogre::uint8& vclu,
const Ogre::uint8& vclo)
75 : skydome(s), vclouds(vc), vcloudsLightningsUnder(vclu), vcloudsLightningsOver(vclo)
129 void update(
const Ogre::Real &timeSinceLastFrame);
138 void notifyCameraRender(Ogre::Camera* c);
151 void setVisible(
const bool& visible);
168 mTimeMultiplier = TimeMultiplier;
169 mVCloudsManager->_updateWindSpeedConfig();
177 return mTimeMultiplier;
193 return mAtmosphereManager;
217 return mCloudsManager;
225 return mVCloudsManager;
233 if (mController->getDeleteBySkyX())
252 void setRenderQueueGroups(
const RenderQueueGroups& rqg);
259 return mRenderQueueGroups;
270 void setLightingMode(
const LightingMode& lm);
277 return mLightingMode;
283 void setStarfieldEnabled(
const bool& Enabled);
301 mInfiniteCameraFarClipDistance = d;
309 return mInfiniteCameraFarClipDistance;
317 return mSceneManager;
331 bool frameStarted(
const Ogre::FrameEvent& evt);
336 void preViewportUpdate(
const Ogre::RenderTargetViewportEvent& evt);
349 return mCfgFileManager;
352 inline const bool loadCfg(
const Ogre::String &File)
const
354 return mCfgFileManager->load(File);
const RenderQueueGroups & getRenderQueueGroups() const
Get render queue groups.
Ogre::Real mInfiniteCameraFarClipDistance
Infinite camera far clip distance.
LightingMode mLightingMode
Lighting mode.
LightingMode
Lighting mode enumeration SkyX is designed for true HDR rendering, but there is a big number of appli...
const LightingMode & getLightingMode() const
Get lighting mode.
Controller * getController() const
Get current controller.
Ogre::Real mLastCameraFarClipDistance
Last camera far clip distance.
Ogre::SceneManager * getSceneManager()
Get scene manager.
bool mCreated
Is SkyX created?
MeshManager * getMeshManager()
Get mesh manager.
const Ogre::Real & _getTimeOffset() const
Get time offset.
Ogre::uint8 vcloudsLightningsUnder
VClouds lightnings render queue group (when the camera is under the cloud field)
const bool & isStarfieldEnabled() const
Is the starfield enable?
Ogre::Real mTimeMultiplier
Time multiplier.
const Ogre::Real & getInfiniteCameraFarClipDistance() const
Get infinite cmaera far clip distance.
void setController(Controller *c)
Set controller.
GPUManager * getGPUManager()
Get GPU manager.
GPUManager * mGPUManager
GPU manager.
CloudsManager * getCloudsManager()
Get clouds manager.
RenderQueueGroups mRenderQueueGroups
Render queue groups.
Ogre::Camera * getCamera()
Get current rendering camera.
AtmosphereManager * mAtmosphereManager
Atmosphere manager.
RenderQueueGroups(const Ogre::uint8 &s, const Ogre::uint8 &vc, const Ogre::uint8 &vclu, const Ogre::uint8 &vclo)
Constructor.
AtmosphereManager * getAtmosphereManager()
Get atmosphere manager.
void setTimeMultiplier(const Ogre::Real &TimeMultiplier)
Set time multiplier.
Ogre::Camera * mCamera
Current rendering camera.
Ogre::SceneManager * mSceneManager
Scene manager.
const Ogre::Real & getTimeMultiplier() const
Get time multiplier.
const bool loadCfg(const Ogre::String &File) const
Ogre::Vector3 mLastCameraPosition
Last camera position.
bool mVisible
Is SkyX visible?
bool mStarfield
Enable starfield?
CfgFileManager * getCfgFileManager()
MeshManager * mMeshManager
Mesh manager.
CloudsManager * mCloudsManager
Clouds manager.
Ogre::Real mTimeOffset
Time offset.
const bool isCreated() const
Is SkyX created?
Ogre::uint8 skydome
Skydome render queue group (Note: Moon = skydome_render_queue+1)
Ogre::uint8 vcloudsLightningsOver
VClouds lightnings render queue group (when the camera is over the cloud field)
const bool & isVisible() const
Is SkyX visible?
MoonManager * mMoonManager
Moon manager.
VCloudsManager * getVCloudsManager()
Get volumetric clouds manager.
VCloudsManager * mVCloudsManager
Volumetric clouds manager.
Ogre::uint8 vclouds
VClouds render queue group.
Controller * mController
Controller.
MoonManager * getMoonManager()
Get moon manager.
void setInfiniteCameraFarClipDistance(const Ogre::Real &d)
Set infinite camera far clip distance.
CfgFileManager * mCfgFileManager