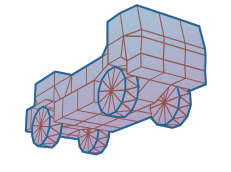 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
25 #ifndef _SkyX_CfgFileManager_H_
26 #define _SkyX_CfgFileManager_H_
35 class BasicController;
56 const bool load(
const Ogre::String& File)
const;
63 const bool save(
const Ogre::String& File,
const Ogre::String& Path =
"")
const;
65 static Ogre::String
_getCfgString(
const Ogre::String& Name,
const int& Value);
66 static Ogre::String
_getCfgString(
const Ogre::String& Name,
const Ogre::Real& Value);
67 static Ogre::String
_getCfgString(
const Ogre::String& Name,
const bool& Value);
68 static Ogre::String
_getCfgString(
const Ogre::String& Name,
const Ogre::Vector2& Value);
69 static Ogre::String
_getCfgString(
const Ogre::String& Name,
const Ogre::Vector3& Value);
70 static Ogre::String
_getCfgString(
const Ogre::String &Name,
const Ogre::Vector4 &Value);
71 static Ogre::String
_getCfgString(
const Ogre::String &Name,
const Ogre::Degree &Value);
76 static int _getIntValue(Ogre::ConfigFile& CfgFile,
const Ogre::String Name);
81 static Ogre::Real
_getFloatValue(Ogre::ConfigFile& CfgFile,
const Ogre::String Name);
86 static bool _getBoolValue(Ogre::ConfigFile& CfgFile,
const Ogre::String Name);
91 static Ogre::Vector2
_getVector2Value(Ogre::ConfigFile& CfgFile,
const Ogre::String Name);
96 static Ogre::Vector3
_getVector3Value(Ogre::ConfigFile& CfgFile,
const Ogre::String Name);
98 static Ogre::Vector4
_getVector4Value(Ogre::ConfigFile& CfgFile,
const Ogre::String Name);
100 static Ogre::Degree
_getDegreeValue(Ogre::ConfigFile& CfgFile,
const Ogre::String Name);
102 static bool _isStringInList(
const Ogre::StringVector &List,
const Ogre::String &Find);
108 const bool _saveToFile(
const Ogre::String& Data,
const Ogre::String& File,
const Ogre::String& Path)
const;
114 const void _loadCfgFile(
const Ogre::String& File, std::pair<bool,Ogre::ConfigFile> &Result)
const;
BasicController * mController
static bool _isStringInList(const Ogre::StringVector &List, const Ogre::String &Find)
static bool _getBoolValue(Ogre::ConfigFile &CfgFile, const Ogre::String Name)
Get bool value.
const bool _saveToFile(const Ogre::String &Data, const Ogre::String &File, const Ogre::String &Path) const
Save a string in file.
static Ogre::Vector4 _getVector4Value(Ogre::ConfigFile &CfgFile, const Ogre::String Name)
static Ogre::Vector2 _getVector2Value(Ogre::ConfigFile &CfgFile, const Ogre::String Name)
Get vector2 value.
static Ogre::Real _getFloatValue(Ogre::ConfigFile &CfgFile, const Ogre::String Name)
Get float value.
const bool load(const Ogre::String &File) const
Load hydrax cfg file.
static Ogre::Vector3 _getVector3Value(Ogre::ConfigFile &CfgFile, const Ogre::String Name)
Get vector3 value.
const void _loadCfgFile(const Ogre::String &File, std::pair< bool, Ogre::ConfigFile > &Result) const
Load a cfg file in an Ogre::ConfigFile.
CfgFileManager(SkyX *s, BasicController *c, Ogre::Camera *d)
Constructor.
const Ogre::String _getVersionCfgString() const
Get hydrax version cfg string.
static Ogre::String _getCfgString(const Ogre::String &Name, const int &Value)
const bool _checkVersion(Ogre::ConfigFile &CfgFile) const
Check hydrax version cfg file.
static Ogre::Degree _getDegreeValue(Ogre::ConfigFile &CfgFile, const Ogre::String Name)
SkyX * mSkyX
Hydrax parent pointer.
~CfgFileManager()
Destructor.
static int _getIntValue(Ogre::ConfigFile &CfgFile, const Ogre::String Name)
Get int value.
const bool save(const Ogre::String &File, const Ogre::String &Path="") const
Save current hydrax config to a file.