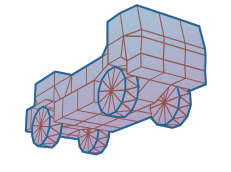 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
56 #include <Bites/OgreWindowEventUtilities.h>
58 #include <MyGUI_OgrePlatform.h>
59 #include <MyGUI_UString.h>
60 #include <OgreOverlay.h>
128 void ShowMessageBox(
const char* title,
const char* text,
bool allow_close =
true,
const char* btn1_text =
"OK",
const char* btn2_text =
nullptr);
163 void eventRequestTag(
const MyGUI::UString& _tag, MyGUI::UString& _result);
GUI::VehicleInfoTPanel VehicleInfoTPanel
ImVec4 selected_entry_text_color
MyGUI::OgrePlatform * m_mygui_platform
void SupressCursor(bool do_supress)
Overlay showing chat/console messages on screen, with optional entry field.
ImVec4 value_red_text_color
GUI::FrictionSettings FrictionSettings
void ApplyGuiCaptureKeyboard()
Call after rendered frame to apply queued value.
Generic UI dialog (not modal). Invocable from scripting. Any number of buttons. Configurable to fire ...
ImVec4 in_progress_text_color
ImVec4 value_blue_text_color
GUI::SimPerfStats SimPerfStats
GUI::TextureToolWindow TextureToolWindow
GUI::GameControls GameControls
GUI::MpClientList MpClientList
Race direction arrow and text info (using OGRE Overlay)
ImVec4 semitrans_text_bg_color
GUI::GameSettings GameSettings
ImVec4 semitransparent_window_bg
GUI::DirectionArrow DirectionArrow
void eventRequestTag(const MyGUI::UString &_tag, MyGUI::UString &_result)
GUI::LoadingWindow LoadingWindow
GUI::RepositorySelector RepositorySelector
void NewImGuiFrame(float dt)
bool AreStaticMenusAllowed()
i.e. top menubar / vehicle UI buttons
GUI::MultiplayerSelector MultiplayerSelector
Flexbody and prop diagnostic.
Ogre::Overlay * MenuWallpaper
GUI::CollisionsDebug CollisionsDebug
void DrawSimulationGui(float dt)
Touches live data; must be called in sync with sim. thread.
bool m_gui_kb_capture_queued
Resets and accumulates every frame.
ImVec4 warning_text_color
void SetGuiHidden(bool visible)
bool IsGuiCaptureKeyboardRequested() const
Central state/object manager and communications hub.
ImVec4 color_mark_max_darkness
If all RGB components are darker than this, text is auto-lightened.
@ SUPRESSED
Hidden manually, will not re-appear until explicitly set VISIBLE.
ImVec4 no_entries_text_color
ImVec4 success_text_color
GUI::NodeBeamUtils NodeBeamUtils
GUI::ConsoleWindow ConsoleWindow
void DrawSimGuiBuffered(GfxActor *player_gfx_actor)
Reads data from simbuffer.
GUI::TopMenubar TopMenubar
void SetUpMenuWallpaper()
void SetMouseCursorVisibility(MouseCursorVisibility visi)
ImVec2 screen_edge_padding
GUI::FlexbodyDebug FlexbodyDebug
Ogre::Timer m_last_mousemove_time
ImVec4 highlight_text_color
void ShowMessageBox(const char *title, const char *text, bool allow_close=true, const char *btn1_text="OK", const char *btn2_text=nullptr)
Diagnostic view for static terrain collisions and script event-boxes.
GUI::MessageBoxDialog MessageBoxDialog
void UpdateMouseCursorVisibility()
GUI::MainSelector MainSelector
ImVec2 semitrans_text_bg_padding
bool m_gui_kb_capture_requested
Effective value, persistent.
@ HIDDEN
Hidden as inactive, will re-appear the moment user moves mouse.
void UpdateInputEvents(float dt)
bool m_is_cursor_supressed
True if cursor was manually hidden.
void SetSceneManagerForGuiRendering(Ogre::SceneManager *scene_manager)
void RequestGuiCaptureKeyboard(bool val)
Pass true during frame to prevent input passing to application.
In-game map widget Has 3 display modes (see SurveyMapMode), cycled using input SURVEY_MAP_TOGGLE_VIEW
GUI::GameMainMenu GameMainMenu
@ VISIBLE
Visible, will be auto-hidden if not moving for a while.