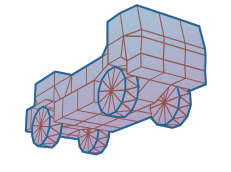 |
RigsofRods
Soft-body Physics Simulation
|
Ogre::TexturePtr m_m_icon
bool IsVisible(TPanelFocus focus=TPANELFOCUS_NONE) const
void DrawEngineButton(RoR::GfxActor *actorx)
int m_stat_deformed_beams
void DrawRepairButton(RoR::GfxActor *actorx)
void DrawLockButton(RoR::GfxActor *actorx)
Ogre::TexturePtr m_a_icon
Global forward declarations.
void DrawBeaconButton(RoR::GfxActor *actorx)
Ogre::TexturePtr m_physics_icon
void DrawWarnBlinkerButton(RoR::GfxActor *actorx)
TPanelMode m_visibility_mode
Ogre::TexturePtr m_particle_icon
void DrawAntiLockBrakeButton(RoR::GfxActor *actorx)
Ogre::TexturePtr m_w_icon
Ogre::TexturePtr m_warning_light_icon
void DrawActorPhysicsButton(RoR::GfxActor *actorx)
void DrawSecureButton(RoR::GfxActor *actorx)
void UpdateStats(float dt, ActorPtr actor)
Caution: touches live data, must be synced with sim. thread.
void DrawMirrorButton(RoR::GfxActor *actorx)
Ogre::TexturePtr m_beacons_icon
Ogre::TexturePtr m_abs_icon
void DrawHornButton(RoR::GfxActor *actorx)
CommandkeyID_t m_active_commandkey
void DrawRightBlinkerButton(RoR::GfxActor *actorx)
TPanelFocus m_requested_focus
Requested by SetVisible()
ImVec4 m_command_hovered_text_color
void DrawVehicleCommandsUI(RoR::GfxActor *actorx)
void DrawTransferCaseModeButton(RoR::GfxActor *actorx)
void DrawWheelDiffButton(RoR::GfxActor *actorx)
Ogre::TexturePtr m_headlight_icon
int CommandkeyID_t
Index into Actor::ar_commandkeys (BEWARE: indexed 1-MAX_COMMANDKEYS, 0 is invalid value,...
Ogre::TexturePtr m_left_blinker_icon
Ogre::TexturePtr m_horn_icon
Ogre::TexturePtr m_actor_physics_icon
ImVec4 m_cmdbeam_highlight_color
float m_cmdbeam_highlight_thickness
void DrawVehicleDiagUI(RoR::GfxActor *actorx)
Ogre::TexturePtr m_parking_brake_icon
void DrawVehicleCommandHighlights(RoR::GfxActor *actorx)
void DrawParticlesButton(RoR::GfxActor *actorx)
ImVec4 m_transluc_textdis_color
bool IsHornButtonActive() const
TPanelFocus m_current_focus
Updated based on currently open tab.
Ogre::TexturePtr m_engine_icon
Ogre::TexturePtr m_camera_icon
Ogre::TexturePtr m_cruise_control_icon
void DrawParkingBrakeButton(RoR::GfxActor *actorx)
void DrawCustomLightButton(RoR::GfxActor *actorx)
Ogre::TexturePtr m_shift_icon
void DrawTransferCaseGearRatioButton(RoR::GfxActor *actorx)
float m_cmdbeam_ui_rect_thickness
Ogre::Timer m_startupdemo_timer
Ogre::TexturePtr m_g_icon
void DrawTractionControlButton(RoR::GfxActor *actorx)
void DrawShiftModeButton(RoR::GfxActor *actorx)
void DrawVehicleBasicsUI(RoR::GfxActor *actorx)
void DrawAxleDiffButton(RoR::GfxActor *actorx)
Ogre::TexturePtr m_mirror_icon
void Draw(RoR::GfxActor *actorx)
ImVec4 m_panel_translucent_color
Ogre::TexturePtr m_lock_icon
Ogre::TexturePtr m_repair_icon
Ogre::TexturePtr m_secure_icon
void SetVisible(TPanelMode mode, TPanelFocus focus=TPANELFOCUS_NONE)
void DrawLeftBlinkerButton(RoR::GfxActor *actorx)
void DrawCruiseControlButton(RoR::GfxActor *actorx)
Ogre::TexturePtr m_right_blinker_icon
void DrawVehicleStatsUI(RoR::GfxActor *actorx)
CommandkeyID_t m_hovered_commandkey
Ogre::TexturePtr m_traction_control_icon
static const CommandkeyID_t COMMANDKEYID_INVALID
CommandkeyID_t GetActiveCommandKey() const
void DrawHeadLightButton(RoR::GfxActor *actorx)