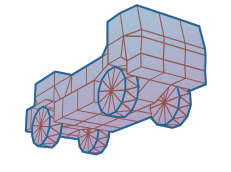 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
48 bool show_translucent =
false;
53 fmt::format(
_LC(
"VehicleButtons",
"Hover the mouse on the left to see controls")),
"lightbulb.png");
58 show_translucent =
true;
65 && (ImGui::GetIO().MousePos.x <=
MIN_PANEL_WIDTH + ImGui::GetStyle().WindowPadding.x*2))
67 show_translucent =
true;
88 ImGuiWindowFlags flags = ImGuiWindowFlags_NoCollapse |
89 ImGuiWindowFlags_NoMove | ImGuiWindowFlags_AlwaysAutoResize | ImGuiWindowFlags_NoTitleBar;
97 ImGui::PushStyleColor(ImGuiCol_TextDisabled, ImGui::GetStyle().Colors[ImGuiCol_TextDisabled]);
108 ImGui::Begin(
"VehicleInfoTPanel",
nullptr, flags);
112 int tabflags_basics = ImGuiTabItemFlags_None;
113 int tabflags_stats = ImGuiTabItemFlags_None;
114 int tabflags_commands = ImGuiTabItemFlags_None;
115 int tabflags_diag = ImGuiTabItemFlags_None;
122 case TPANELFOCUS_DIAG: tabflags_diag = ImGuiTabItemFlags_SetSelected;
break;
133 ImVec2 name_pos = ImGui::GetCursorPos();
137 Ogre::TexturePtr preview_tex = Ogre::TextureManager::getSingleton().load(
141 ImVec2 size(preview_tex->getWidth(), preview_tex->getHeight());
148 ImGui::Image(
reinterpret_cast<ImTextureID
>(preview_tex->getHandle()), size);
149 tabs_pos = ImGui::GetCursorPos();
151 name_pos.x += size.x + ImGui::GetStyle().ItemSpacing.x;
152 ImGui::SetCursorPos(name_pos);
161 ImGui::SetCursorPos(tabs_pos);
163 ImGui::BeginTabBar(
"VehicleInfoTPanelTabs", ImGuiTabBarFlags_None);
164 if (ImGui::BeginTabItem(
_LC(
"TPanel",
"Basics"),
nullptr, tabflags_basics))
171 if (ImGui::BeginTabItem(
_LC(
"TPanel",
"Stats"),
nullptr, tabflags_stats))
178 if (ImGui::BeginTabItem(
_LC(
"TPanel",
"Commands"),
nullptr, tabflags_commands))
185 if (ImGui::BeginTabItem(
_LC(
"TPanel",
"Diag"),
nullptr, tabflags_diag))
196 ImGui::PopStyleColor(2);
207 ImGui::TextDisabled(
"%s",
_LC(
"VehicleDescription",
"Description text:"));
210 ImGui::TextWrapped(
"%s", line.c_str());
218 ImGui::TextDisabled(
"%s",
_LC(
"VehicleDescription",
"Help image:"));
220 ImGui::SetCursorPosX(
MIN_PANEL_WIDTH - (ImGui::CalcTextSize(
_LC(
"VehicleDescription",
"Full size")).
x + 25.f));
223 ImTextureID im_tex =
reinterpret_cast<ImTextureID
>(actorx->
GetHelpTex()->getHandle());
241 ImGui::TextDisabled(
"%s",
_LC(
"VehicleDescription",
"Command controls:"));
243 ImGui::Text(
"%s",
_LC(
"VehicleDescription",
"Hover controls for on-vehicle highlight"));
244 ImGui::PopStyleColor(1);
249 ImVec2 initial_cursor = ImGui::GetCursorScreenPos();
252 std::string desc = qpair.uckp_description;
253 if (qpair.uckp_description ==
"")
255 desc =
_LC(
"VehicleDescription",
"~unlabeled~");
259 ImVec2 desc_size = ImGui::CalcTextSize(desc.c_str());
260 const bool single_line = ImGui::GetWindowContentRegionMax().x > desc_size.x + key1_size.x + key2_size.x;
261 static const float BUMP_HEIGHT = 3.f;
262 static const float MOUSEHIGHLIGHT_MAGICPADRIGHT = 4.f;
265 ImVec2 highlight_mouse_min = initial_cursor - ImGui::GetStyle().ItemSpacing/2;
266 ImVec2 highlight_mouse_max = highlight_mouse_min + ImVec2(ImGui::GetWindowContentRegionWidth()+MOUSEHIGHLIGHT_MAGICPADRIGHT, ImGui::GetTextLineHeightWithSpacing());
269 highlight_mouse_max.y += ImGui::GetTextLineHeightWithSpacing() - BUMP_HEIGHT;
272 if ((ImGui::GetMousePos().
x > highlight_mouse_min.x && ImGui::GetMousePos().y > highlight_mouse_min.y)
273 && (ImGui::GetMousePos().x < highlight_mouse_max.x && ImGui::GetMousePos().y < highlight_mouse_max.y))
281 ImDrawList* draw_list = ImGui::GetWindowDrawList();
282 draw_list->AddRectFilled(highlight_mouse_min, highlight_mouse_max, ImColor(col));
290 ImGui::Text(
"%s", desc.c_str());
300 ImGui::SetCursorPos(ImGui::GetCursorPos() + ImVec2(0.f, -BUMP_HEIGHT));
304 bool key1_hovered =
false;
305 bool key1_active =
false;
306 ImGui::SetCursorPosX((ImGui::GetWindowContentRegionMax().
x) - key1_size.x - key2_size.x - ImGui::GetStyle().ItemSpacing.x);
319 ImGui::SetCursorPosX((ImGui::GetWindowContentRegionMax().
x) - key2_size.x);
320 bool key2_hovered =
false;
321 bool key2_active =
false;
340 ImVec2 label_size = ImGui::CalcTextSize(name);
341 ImVec2 value_size = ImGui::CalcTextSize(value.c_str());
342 float cursor_x_desired = - ImGui::CalcTextSize(value.c_str()).x;
343 if (label_size.x + value_size.x + ImGui::GetStyle().ItemSpacing.x < ImGui::GetWindowContentRegionWidth())
347 ImGui::SetCursorPosX(ImGui::GetWindowContentRegionMax().
x - value_size.x);
348 ImGui::TextColored(value_color,
"%s", value.c_str());
361 ImGui::PopStyleColor();
381 ImGui::PushStyleVar(ImGuiStyleVar_ItemSpacing, ImVec2(ImGui::GetStyle().ItemSpacing.x, 0));
402 const float avg_deform =
static_cast<float>(
Round((
float)
m_stat_avg_deform / (
float)num_beams, 4) * 100.0f );
421 const double PI = 3.14159265358979323846;
429 const ImVec4 rpm_color = (cur_rpm > max_rpm) ? theme.
value_red_text_color : ImGui::GetStyle().Colors[ImGuiCol_Text];
437 const float currentKw = (((cur_rpm * (torque + ((turbo_psi * 6.8) * torque) / 100) * ( PI / 30)) / 1000));
450 float speedKN = n0_velo_len * 1.94384449f;
461 std::string label =
fmt::format(
"{} #{}:",
_LC(
"SimActorStats",
"Engine "), engine_num);
478 std::string label =
fmt::format(
"{} #{}:",
_LC(
"SimActorStats",
"Engine "), engine_num);
496 ImGui::PopStyleVar();
501 ImGui::TextDisabled(
"%s",
_LC(
"TopMenubar",
"Live diagnostic views:"));
505 int debug_view_type =
static_cast<int>(actorx->
GetDebugView());
532 if ((current_actor !=
nullptr) && (debug_view_type !=
static_cast<int>(current_actor->
GetGfxActor()->
GetDebugView())))
540 ImGui::TextDisabled(
"%s",
_LC(
"TopMenubar",
"Settings:"));
545 if (debug_view_type >= 2)
563 float average_deformation = 0.0f;
564 float beamstress = 0.0f;
567 int beamdeformed = 0;
577 beamstress += std::abs(beam->
stress);
578 float current_deformation = fabs(beam->
L - beam->
refL);
583 average_deformation += current_deformation;
610 ImGui::Dummy(
BUTTONDUMMY_SIZE); ImGui::SameLine(); ImGui::Bullet(); ImGui::Text(
"%s", name);
613 ImGui::SetCursorPosX(ImGui::GetWindowContentRegionWidth() - btn_size.x);
626 ImGui::TextDisabled(
"Simulation:");
636 if (num_headlights || num_taillights || num_blinkleft || num_blinkright || num_beacons || has_horn)
638 ImGui::TextDisabled(
"Lights and signals:");
639 if (num_headlights || num_taillights)
651 if (num_blinkright || num_blinkleft)
673 ImGui::TextDisabled(
"Engine:");
679 if (has_transfercase && has_4wd && has_2wd)
683 if (has_transfercase && num_tc_gears > 1)
720 if (num_axlediffs || num_wheeldiffs || tc_visible || alb_visible || has_parkingbrake || has_engine)
722 ImGui::TextDisabled(
"Traction:");
739 if (has_parkingbrake)
751 if (num_locks || num_ties)
753 ImGui::TextDisabled(
"Connections:");
766 ImGui::TextDisabled(
"View:");
790 ImVec2 p1_pos, p2_pos;
802 ImGui::PushStyleColor(ImGuiCol_Button, ImGui::GetStyle().Colors[ImGuiCol_ButtonActive]);
806 ImGui::PushStyleColor(ImGuiCol_Button, ImGui::GetStyle().Colors[ImGuiCol_Button]);
809 ImGui::GetWindowDrawList()->AddRectFilled(
811 ImColor(ImGui::GetStyle().Colors[ImGuiCol_Button]), ImGui::GetStyle().FrameRounding);
812 ImGui::GetWindowDrawList()->AddImage(
reinterpret_cast<ImTextureID
>(icon->getHandle()),
814 ImGui::SetCursorPosX(ImGui::GetCursorPosX() +
BUTTON_SIZE.x + 2*ImGui::GetStyle().ItemSpacing.x);
815 ImGui::PopStyleColor();
817 ImGui::Text(
"%s", name);
820 ImGui::SetCursorPosX(ImGui::GetWindowContentRegionMax().
x - btn_size.x);
992 const char* msg =
nullptr;
1027 int num_custom_flares = 0;
1034 num_custom_flares++;
1036 if (i == 5 || i == 9)
1041 std::string label =
"L" + std::to_string(i + 1);
1045 ImGui::PushStyleColor(ImGuiCol_Button, ImGui::GetStyle().Colors[ImGuiCol_ButtonActive]);
1049 ImGui::PushStyleColor(ImGuiCol_Button, ImGui::GetStyle().Colors[ImGuiCol_Button]);
1052 if (ImGui::Button(label.c_str(), ImVec2(32, 0)))
1056 if (ImGui::IsItemHovered())
1058 ImGui::BeginTooltip();
1060 ImGui::EndTooltip();
1064 ImGui::PopStyleColor();
1068 if (num_custom_flares > 0)
int getAxleDiffMode()
Writes info to console/notify box.
Game state manager and message-queue provider.
Ogre::TexturePtr m_m_icon
int tr_ax_2
This axle is only driven in 4WD mode.
void DrawEngineButton(RoR::GfxActor *actorx)
int m_stat_deformed_beams
void SetDebugView(DebugViewType dv)
void DrawRepairButton(RoR::GfxActor *actorx)
bool cc_mode
Cruise Control.
void DrawLockButton(RoR::GfxActor *actorx)
int getWheelDiffMode()
Writes info to console/notify box.
CVar * gfx_speedo_imperial
bool getBeaconMode() const
void ImTextWrappedColorMarked(std::string const &text)
Prints multiline text with '#rrggbb' color markers. Behaves like ImGui::Text* functions.
Ogre::TexturePtr m_a_icon
ImVec2 ImCalcEventHighlightedSize(events input_event)
void DrawBeaconButton(RoR::GfxActor *actorx)
ImVec4 value_red_text_color
@ MSG_SIM_MODIFY_ACTOR_REQUESTED
Payload = RoR::ActorModifyRequest* (owner)
void DrawStatsLineColored(const char *name, const std::string &value, ImVec4 value_color)
Ogre::TexturePtr FetchIcon(const char *name)
Ogre::TexturePtr m_physics_icon
size_t getNumVideoCameras() const
bool ar_physics_paused
Sim state.
bool isLocked()
Are hooks locked?
@ TRUCK
its a truck (or other land vehicle)
void DrawWarnBlinkerButton(RoR::GfxActor *actorx)
void toggleAxleDiffMode()
static const int MAX_CLIGHTS
See RoRnet::Lightmask and enum events in InputEngine.h.
TPanelMode m_visibility_mode
CameraManager * GetCameraManager()
Ogre::Vector3 AbsPosition
absolute position in the world (shaky)
GUIManager * GetGuiManager()
CVar * diag_hide_broken_beams
ImVec4 value_blue_text_color
Estabilishing a physics linkage between 2 actors modifies a global linkage table and triggers immedia...
NodeSB * GetSimNodeBuffer()
void ToggleAutoShiftMode()
bool getCustomParticleMode()
ActorInstanceID_t ar_instance_id
Static attr; session-unique ID.
bool GetScreenPosFromWorldPos(Ogre::Vector3 const &world_pos, ImVec2 &out_screen)
const float MIN_PANEL_WIDTH
std::vector< UniqueCommandKeyPair > ar_unique_commandkey_pairs
UI helper for displaying command control keys to user (must be built at spawn).
Ogre::TexturePtr m_particle_icon
void DrawAntiLockBrakeButton(RoR::GfxActor *actorx)
const ImVec2 BUTTONDUMMY_SIZE(18, 1)
void toggleWheelDiffMode()
Truck file format(technical spec)
std::vector< ScrewpropSB > simbuf_screwprops
Ogre::TexturePtr m_w_icon
bool tr_2wd
Does it support 2WD mode?
int getWheelNodeCount() const
Ogre::TexturePtr m_warning_light_icon
int countBeaconProps() const
void DrawActorPhysicsButton(RoR::GfxActor *actorx)
void DrawSecureButton(RoR::GfxActor *actorx)
void UpdateStats(float dt, ActorPtr actor)
Caution: touches live data, must be synced with sim. thread.
ActorLinkingRequestType alr_type
Ogre::Vector3 simbuf_node0_velo
Ogre::Real Round(Ogre::Real value, unsigned short ndigits=0)
void RequestUpdateHudFeatures()
std::vector< std::string > getDescription()
void toggleCustomParticles()
void DrawMirrorButton(RoR::GfxActor *actorx)
void putMessage(MessageArea area, MessageType type, std::string const &msg, std::string icon="")
std::vector< hook_t > ar_hooks
std::vector< AeroEngineSB > simbuf_aeroengines
Ogre::TexturePtr m_beacons_icon
std::vector< tie_t > ar_ties
Ogre::TexturePtr m_abs_icon
void DrawHornButton(RoR::GfxActor *actorx)
CommandkeyID_t m_active_commandkey
std::vector< float > tr_gear_ratios
Gear reduction ratios.
void DrawRightBlinkerButton(RoR::GfxActor *actorx)
CVar * diag_hide_beam_stress
TPanelFocus m_requested_focus
Requested by SetVisible()
@ NOT_DRIVEABLE
not drivable at all
void displayAxleDiffMode()
Cycles through the available inter axle diff modes.
ImVec4 semitransparent_window_bg
void tractioncontrolToggle()
ImVec4 m_command_hovered_text_color
void DrawVehicleCommandsUI(RoR::GfxActor *actorx)
void DrawStatsLine(const char *name, const std::string &value)
void SetVideoCamState(VideoCamState state)
void DrawTransferCaseModeButton(RoR::GfxActor *actorx)
CVar * diag_hide_wheel_info
bool tc_nodash
Traction control attribute; Hide the dashboard indicator?
float refL
reference length
void DrawWheelDiffButton(RoR::GfxActor *actorx)
void DrawStatsBullet(const char *name, const std::string &value)
Ogre::Vector3 getMaxGForces()
ImDrawList * GetImDummyFullscreenWindow(const std::string &name="RoR_TransparentFullscreenWindow")
ActorInstanceID_t amr_actor
@ MANUAL_STICK
Fully manual: stick shift.
Ogre::TexturePtr m_headlight_icon
VideoCamState GetVideoCamState() const
Simulation: An edge in the softbody structure.
Ogre::TexturePtr m_left_blinker_icon
Ogre::TexturePtr m_horn_icon
Ogre::TexturePtr m_actor_physics_icon
ImVec4 m_cmdbeam_highlight_color
Core data structures for simulation; Everything affected by by either physics, network or user intera...
Ogre::String filecachename
preview image filename
Ogre::TexturePtr GetHelpTex()
TransferCase * getTransferCaseMode()
Toggles between 2WD and 4WD mode.
int ar_num_custom_particles
float m_cmdbeam_highlight_thickness
void PushMessage(Message m)
Doesn't guarantee order! Use ChainMessage() if order matters.
void DrawSingleBulletRow(const char *name, RoR::events ev)
float simbuf_inputshaft_rpm
void DrawVehicleDiagUI(RoR::GfxActor *actorx)
const float HELP_TEXTURE_WIDTH
Ogre::TexturePtr m_parking_brake_icon
void DrawVehicleCommandHighlights(RoR::GfxActor *actorx)
void antilockbrakeToggle()
bool getHeadlightsVisible() const
RoR::SimGearboxMode GetAutoShiftMode()
CmdKeyArray ar_command_key
BEWARE: commandkeys are indexed 1-MAX_COMMANDS!
void DrawParticlesButton(RoR::GfxActor *actorx)
const ImVec2 BUTTON_SIZE(18, 18)
ImVec4 m_transluc_textdis_color
Central state/object manager and communications hub.
@ SEMI_AUTO
Manual shift with auto clutch.
TPanelFocus m_current_focus
Updated based on currently open tab.
const ImVec2 BUTTON_OFFSET(0, 3.f)
bool ImDrawEventHighlightedButton(events input_event, bool *btn_hovered=nullptr, bool *btn_active=nullptr)
GameContext * GetGameContext()
Ogre::TexturePtr m_engine_icon
const float HELP_TEXTURE_HEIGHT
@ AIRPLANE
its an airplane
Ogre::TexturePtr m_camera_icon
std::string FormatVelocityBySpeedoPreset(float velocity)
Ogre::TexturePtr m_cruise_control_icon
void DrawParkingBrakeButton(RoR::GfxActor *actorx)
void DrawCustomLightButton(RoR::GfxActor *actorx)
float getTotalMass(bool withLocked=true)
Ogre::TexturePtr m_shift_icon
CacheEntryPtr & getUsedActorEntry()
The actor entry itself.
bool tc_mode
Traction control state; Enabled? {1/0}.
void DrawTransferCaseGearRatioButton(RoR::GfxActor *actorx)
@ MANUAL
Fully manual: sequential shift.
Ogre::Vector3 AbsPosition
void toggleTransferCaseMode()
void parkingbrakeToggle()
ImVec2 screen_edge_padding
bool ar_is_police
Gfx/sfx attr.
Ogre::Timer m_startupdemo_timer
bool alb_mode
Anti-lock brake state; Enabled? {1/0}.
CVar * ui_show_vehicle_buttons
UI helper for displaying command control keys to user.
float simbuf_engine_max_rpm
Ogre::TexturePtr m_g_icon
void toggleBlinkType(BlinkType blink)
void DrawTractionControlButton(RoR::GfxActor *actorx)
@ MSG_SIM_ACTOR_LINKING_REQUESTED
Payload = RoR::ActorLinkingRequest* (owner)
Unified game event system - all requests and state changes are reported using a message.
void DrawShiftModeButton(RoR::GfxActor *actorx)
bool alb_nodash
Anti-lock brake attribute: Hide the dashboard indicator?
void DrawVehicleBasicsUI(RoR::GfxActor *actorx)
void DrawAxleDiffButton(RoR::GfxActor *actorx)
void StartEngine()
Quick engine start. Plays sounds.
void toggleTransferCaseGearRatio()
InputEngine * GetInputEngine()
ActorInstanceID_t alr_actor_instance_id
float simbuf_engine_turbo_psi
Ogre::TexturePtr m_mirror_icon
void setCustomLightVisible(int number, bool visible)
const ImVec2 MAX_PREVIEW_SIZE(100.f, 100.f)
void Draw(RoR::GfxActor *actorx)
ImVec4 m_panel_translucent_color
@ MANUAL_RANGES
Fully manual: stick shift with ranges.
Manager for all visuals belonging to a single actor.
Ogre::TexturePtr m_lock_icon
int countFlaresByType(FlareType type)
Ogre::TexturePtr m_repair_icon
int countCustomLights(int control_number)
bool DrawGCheckbox(CVar *cvar, const char *label)
Ogre::TexturePtr m_secure_icon
DebugViewType GetDebugView() const
void ImDrawEventHighlighted(events input_event)
@ CONSOLE_MSGTYPE_INFO
Generic message.
std::string getTruckName()
void SetVisible(TPanelMode mode, TPanelFocus focus=TPANELFOCUS_NONE)
void DrawLeftBlinkerButton(RoR::GfxActor *actorx)
Ogre::Vector3 getGForces()
void displayWheelDiffMode()
Cycles through the available inter wheel diff modes.
void DrawCruiseControlButton(RoR::GfxActor *actorx)
Ogre::TexturePtr m_right_blinker_icon
#define SOUND_GET_STATE(_ACTOR_, _TRIG_)
void DrawVehicleStatsUI(RoR::GfxActor *actorx)
CommandkeyID_t m_hovered_commandkey
Ogre::TexturePtr m_traction_control_icon
void cruisecontrolToggle()
Defined in 'gameplay/CruiseControl.cpp'.
ActorSB & GetSimDataBuffer()
int ar_num_shocks
Number of shock absorbers.
float simbuf_engine_torque
bool DrawSingleButtonRow(bool active, const Ogre::TexturePtr &icon, const char *name, RoR::events ev, bool *btn_active=nullptr)
static const CommandkeyID_t COMMANDKEYID_INVALID
#define SOUND_TOGGLE(_ACTOR_, _TRIG_)
void DrawHeadLightButton(RoR::GfxActor *actorx)
void switchToNextBehavior()
bool getCustomLightVisible(int number)
void displayTransferCaseMode()
Gets the current transfer case mode name (4WD Hi, ...)
const float BUTTON_Y_OFFSET