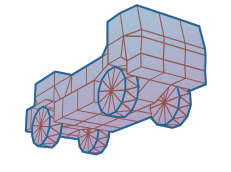 |
RigsofRods
Soft-body Physics Simulation
|
float m_colum_widths[3]
body->header width sync
Str< 1000 > m_active_buffer
eventtypes m_selected_evtype
int m_active_mapping_file
bool m_interactive_keybinding_expl
void DrawControlsTab(const char *prefix)
Draws table with events matching prefix.
RoR::events m_active_event
event_trigger_t * m_active_trigger
void DrawControlsTabItem(const char *name, const char *prefix)
Wraps DrawControlsTab() with scrollbar and tabs-bar logic.
void DrawEvent(RoR::events ev_code)
One line in table.
bool IsInteractiveKeyBindingActive()
const ImVec4 GRAY_HINT_TEXT
bool ShouldDisplay(event_trigger_t &trig)
void SetVisible(bool visible)
void DrawEventEditBox()
Only the editing UI, embeddable.
bool m_interactive_keybinding_active