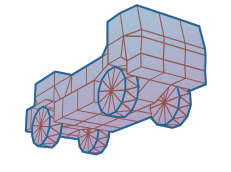 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
37 change = Ogre::Math::Clamp(change, -dt * 10.0f, -dt * 1.0f);
46 change = Ogre::Math::Clamp(change, +dt * 1.0f, +dt * 10.0f);
float m_ref_tyre_pressure
bool ModifyTyrePressure(float v)
Wheel 'pressure adjustment' logic (only for 'wheels2')
float m_pressure_pressed_timer
std::vector< int > m_pressure_beams
#define SOUND_START(_ACTOR_, _TRIG_)
#define SOUND_STOP(_ACTOR_, _TRIG_)
InputEngine * GetInputEngine()
void UpdateInputEvents(float dt)