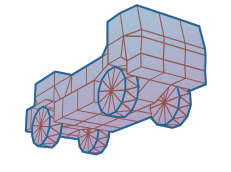 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
62 const ActorPtr ActorManager::ACTORPTR_NULL;
64 ActorManager::ActorManager()
65 : m_dt_remainder(0.0f)
66 , m_forced_awake(false)
67 , m_physics_steps(2000)
68 , m_simulation_speed(1.0f)
88 LOG(
" == Spawning vehicle: " + def->name);
123 vehicle_position.x += vehicle_position.x - actor->
ar_bounding_box.getCenter().x;
124 vehicle_position.z += vehicle_position.z - actor->
ar_bounding_box.getCenter().z;
130 miny = vehicle_position.y;
141 actor->
resetPosition(vehicle_position.x, vehicle_position.z,
true, miny);
152 Vector3 gpos = Vector3(vehicle_position.x, 0.0f, vehicle_position.z);
200 if (!subMeshGroundModelName.empty())
316 for (
RigDef::Script const& script_def : def->root_module->scripts)
321 LOG(
" ===== DONE LOADING VEHICLE");
342 if (actor->ar_net_source_id == sourceid)
353 std::stable_sort(packet_buffer.begin(), packet_buffer.end(),
355 { return a.header.source > b.header.source; });
357 auto it = std::unique(packet_buffer.rbegin(), packet_buffer.rend(),
359 { return !memcmp(&a.header, &b.header, sizeof(RoRnet::Header)) &&
360 a.header.command == RoRnet::MSG2_STREAM_DATA; });
361 packet_buffer.erase(packet_buffer.begin(), it.base());
362 for (
auto& packet : packet_buffer)
372 std::string filename;
373 std::string bundlename;
382 else if (filename.empty())
390 text <<
_L(
"spawned a new vehicle: ") << filename;
402 _L(
"Mod not installed: ") + filename);
403 RoR::LogFormat(
"[RoR] Cannot create remote actor (not installed), filename: '%s'", filename_maybe_bundlequalified.c_str());
418 if (
strnlen(actor_reg->skin, 60) < 60 && actor_reg->skin[0] !=
'\0')
422 if (
strnlen(actor_reg->sectionconfig, 60) < 60)
448 int sourceid = packet.header.source;
449 actor->ar_net_stream_results[sourceid] = reg->
status;
454 case 1: message =
"successfully loaded stream";
break;
455 case -2: message =
"detected mismatch stream";
break;
456 default: message =
"could not load stream";
break;
486 if (packet.header.source == actor->ar_net_source_id && packet.header.streamid == actor->ar_net_stream_id)
488 actor->pushNetwork(packet.buffer, packet.header.size);
495 #endif // USE_SOCKETW
502 return search->second;
525 if (actor->ar_net_source_id == sourceid)
543 int stream_result = actor->ar_net_stream_results[sourceid];
544 if (stream_result == -1 || stream_result == -2)
546 if (stream_result == 1)
557 if (actor->ar_net_source_id == source_id && actor->ar_net_stream_id == stream_id)
568 if (
m_actors[a]->ar_collision_bounding_boxes.empty() &&
m_actors[b]->ar_collision_bounding_boxes.empty())
570 return m_actors[a]->ar_bounding_box.intersects(
m_actors[b]->ar_bounding_box);
572 else if (
m_actors[a]->ar_collision_bounding_boxes.empty())
574 for (
const auto& bbox_b :
m_actors[b]->ar_collision_bounding_boxes)
575 if (bbox_b.intersects(
m_actors[a]->ar_bounding_box))
578 else if (
m_actors[b]->ar_collision_bounding_boxes.empty())
580 for (
const auto& bbox_a :
m_actors[a]->ar_collision_bounding_boxes)
581 if (bbox_a.intersects(
m_actors[b]->ar_bounding_box))
586 for (
const auto& bbox_a :
m_actors[a]->ar_collision_bounding_boxes)
587 for (
const auto& bbox_b :
m_actors[b]->ar_collision_bounding_boxes)
588 if (bbox_a.intersects(bbox_b))
597 if (
m_actors[a]->ar_predicted_coll_bounding_boxes.empty() &&
m_actors[b]->ar_predicted_coll_bounding_boxes.empty())
599 return m_actors[a]->ar_predicted_bounding_box.intersects(
m_actors[b]->ar_predicted_bounding_box);
601 else if (
m_actors[a]->ar_predicted_coll_bounding_boxes.empty())
603 for (
const auto& bbox_b :
m_actors[b]->ar_predicted_coll_bounding_boxes)
604 if (bbox_b.intersects(
m_actors[a]->ar_predicted_bounding_box))
607 else if (
m_actors[b]->ar_predicted_coll_bounding_boxes.empty())
609 for (
const auto& bbox_a :
m_actors[a]->ar_predicted_coll_bounding_boxes)
610 if (bbox_a.intersects(
m_actors[b]->ar_predicted_bounding_box))
615 for (
const auto& bbox_a :
m_actors[a]->ar_predicted_coll_bounding_boxes)
616 for (
const auto& bbox_b :
m_actors[b]->ar_predicted_coll_bounding_boxes)
617 if (bbox_a.intersects(bbox_b))
631 for (
unsigned int t = 0; t <
m_actors.size(); t++)
633 if (t == j || visited[t])
637 m_actors[t]->ar_sleep_counter = 0.0f;
642 m_actors[t]->ar_sleep_counter = 0.0f;
658 (actor->getPosition().distance(source_actor->
getPosition()) <
664 actor->ar_sleep_counter = 0.0f;
670 if (std::find(linked_actors.begin(), linked_actors.end(), actor) == linked_actors.end())
677 actor->ar_command_key[j].playerInputValue = std::max(source_actor->
ar_command_key[j].playerInputValue,
702 for (
auto hook : source_actor->
ar_hooks)
704 if (!hook.hk_locked_actor || hook.hk_locked_actor == source_actor)
708 hook.hk_locked_actor->ar_brake = source_actor->
ar_brake;
711 hook.hk_locked_actor->parkingbrakeToggle();
724 auto& actor_pair = entry.second;
725 if ((actor_pair.first == a1 && actor_pair.second == a2) ||
726 (actor_pair.first == a2 && actor_pair.second == a1))
742 if (actor->ar_driveable ==
AI)
744 if (actor->getVelocity().squaredLength() > 0.01f)
746 actor->ar_sleep_counter = 0.0f;
750 actor->ar_sleep_counter += dt;
752 if (actor->ar_sleep_counter >= 10.0f)
764 std::vector<bool> visited(
m_actors.size());
772 for (
unsigned int t = 0; t <
m_actors.size(); t++)
786 actor->ar_sleep_counter = 0.0f;
809 if (collisions->
isInside(actor->ar_nodes[0].AbsPosition, inst, box))
825 if (actor !=
nullptr)
840 actor->muteAllSounds();
848 actor->unmuteAllSounds();
855 float min_squared_distance = std::numeric_limits<float>::max();
858 float squared_distance = position.squaredDistance(actor->ar_nodes[0].AbsPosition);
859 if (squared_distance < min_squared_distance)
861 min_squared_distance = squared_distance;
862 nearest_actor = actor;
865 return std::make_pair(nearest_actor, std::sqrt(min_squared_distance));
896 { return b->ar_net_source_id == actor->ar_net_source_id; }) == 1)
902 #endif // USE_SOCKETW
905 std::vector<ScriptUnitId_t> unload_list;
908 if (pair.second.associatedActor == actor)
909 unload_list.push_back(pair.first);
921 [actor](
FreeForce& item) { return item.ffc_base_actor == actor || item.ffc_target_actor == actor; }),
930 for (
unsigned int i = 0; i <
m_actors.size(); i++)
939 if (player !=
nullptr)
941 else if (prev_player !=
nullptr)
966 for (
int i = pivot_index + 1; i <
m_actors.size(); i++)
972 for (
int i = 0; i < pivot_index; i++)
978 if (pivot_index >= 0)
991 for (
int i = pivot_index - 1; i >= 0; i--)
997 for (
int i =
static_cast<int>(
m_actors.size()) - 1; i > pivot_index; i--)
1003 if (pivot_index >= 0)
1018 if (actor->ar_rescuer_flag)
1031 dt = std::min(dt, 1.0f / 20.0f);
1051 actor->HandleInputEvents(dt);
1052 actor->HandleAngelScriptEvents(dt);
1054 #ifdef USE_ANGELSCRIPT
1055 if (actor->ar_vehicle_ai && actor->ar_vehicle_ai->isActive())
1056 actor->ar_vehicle_ai->update(dt, 0);
1057 #endif // USE_ANGELSCRIPT
1059 if (actor->ar_engine)
1061 if (actor->ar_driveable ==
TRUCK)
1067 actor->ar_engine->UpdateEngineSim(dt, 1);
1069 actor->ar_engine->UpdateEngineAudio();
1073 actor->updateDashBoards(dt);
1076 actor->updateFlareStates(dt);
1080 actor->updateVisual(dt);
1083 actor->updateSkidmarks();
1090 actor->calcNetwork();
1092 actor->sendStreamData();
1096 if (player_actor !=
nullptr)
1130 auto func = std::function<void()>([
this]()
1146 if (actor->ar_instance_id == actor_id)
1158 actor->UpdatePhysicsOrigin();
1163 std::vector<std::function<void()>> tasks;
1166 if (actor->ar_update_physics = actor->CalcForcesEulerPrepare(i == 0))
1168 auto func = std::function<void()>([
this, i, &actor]()
1172 tasks.push_back(func);
1178 if (actor->ar_update_physics)
1180 actor->CalcBeamsInterActor();
1185 std::vector<std::function<void()>> tasks;
1188 if (actor->m_inter_point_col_detector !=
nullptr && (actor->ar_update_physics ||
1191 auto func = std::function<void()>([
this, &actor]()
1193 actor->m_inter_point_col_detector->UpdateInterPoint();
1194 if (actor->ar_collision_relevant)
1196 ResolveInterActorCollisions(PHYSICS_DT,
1197 *actor->m_inter_point_col_detector,
1198 actor->ar_num_collcabs,
1201 actor->ar_inter_collcabrate,
1203 actor->ar_collision_range,
1204 *actor->ar_submesh_ground_model);
1207 tasks.push_back(func);
1218 actor->m_ongoing_reset =
false;
1221 Vector3 camera_gforces = actor->m_camera_gforces_accu /
m_physics_steps;
1222 actor->m_camera_gforces_accu = Vector3::ZERO;
1223 actor->m_camera_gforces = actor->m_camera_gforces * 0.5f + camera_gforces * 0.5f;
1224 actor->calculateLocalGForces();
1225 actor->calculateAveragePosition();
1226 actor->m_avg_node_velocity = actor->m_avg_node_position - actor->m_avg_node_position_prev;
1228 actor->m_avg_node_position_prev = actor->m_avg_node_position;
1229 actor->ar_top_speed = std::max(actor->ar_top_speed, actor->ar_nodes[0].Velocity.length());
1243 msg <<
"Failed to load '" << filename <<
"' (type: '" << type <<
"'), message: " << exception_msg;
1274 if (stream.isNull() || !stream->isReadable())
1289 LOG(
" == Validating vehicle: " + def->name);
1292 validator.
Setup(def);
1301 Ogre::StringUtil::toLowerCase(file_extension);
1302 if ((file_extension ==
".load") || (file_extension ==
".fixed"))
1310 def->hash =
Sha1Hash(stream->getAsString());
1315 catch (Ogre::Exception& oex)
1320 catch (std::exception& stex)
1334 std::vector<ActorPtr> actors;
1338 actors.push_back(actor);
1353 String ssmsg =
_L(
"New simulation speed: ") +
TOSTRING(
Round(simulation_speed * 100.0f, 1)) +
"%";
1362 String ssmsg =
_L(
"New simulation speed: ") +
TOSTRING(
Round(simulation_speed * 100.0f, 1)) +
"%";
1370 if (simulation_speed != 1.0f)
1374 UTFString ssmsg =
_L(
"Simulation speed reset.");
1400 String ssmsg =
_L(
"Physics paused");
1405 String ssmsg =
_L(
"Physics unpaused");
1437 #ifdef USE_ANGELSCRIPT
1440 #endif // USE_ANGELSCRIPT
1449 Ogre::Degree pitchAngle = Ogre::Radian(asin(dirDiff.dotProduct(Ogre::Vector3::UNIT_Y)));
1451 if (std::abs(pitchAngle.valueDegrees()) > 2.0f)
1459 float downhill_force = std::abs(sin(pitchAngle.valueRadians()) * vehicle->
getTotalMass()) * g;
1461 float ratio = std::max(0.0f, 1.0f - (engine_force / downhill_force));
1483 if (engine && engine->
GetGear() != 0)
1499 ROR_ASSERT(freeforce.ffc_base_actor !=
nullptr);
1502 ROR_ASSERT(freeforce.ffc_base_node <= freeforce.ffc_base_actor->ar_num_nodes);
1505 switch (freeforce.ffc_type)
1508 freeforce.ffc_base_actor->ar_nodes[freeforce.ffc_base_node].Forces += freeforce.ffc_force_magnitude * freeforce.ffc_force_const_direction;
1513 const Vector3 force_direction = (freeforce.ffc_target_coords - freeforce.ffc_base_actor->ar_nodes[freeforce.ffc_base_node].AbsPosition).normalisedCopy();
1514 freeforce.ffc_base_actor->ar_nodes[freeforce.ffc_base_node].Forces += freeforce.ffc_force_magnitude * force_direction;
1521 ROR_ASSERT(freeforce.ffc_target_actor !=
nullptr);
1524 ROR_ASSERT(freeforce.ffc_target_node <= freeforce.ffc_target_actor->ar_num_nodes);
1526 const Vector3 force_direction = (freeforce.ffc_target_actor->ar_nodes[freeforce.ffc_target_node].AbsPosition - freeforce.ffc_base_actor->ar_nodes[freeforce.ffc_base_node].AbsPosition).normalisedCopy();
1527 freeforce.ffc_base_actor->ar_nodes[freeforce.ffc_base_node].Forces += freeforce.ffc_force_magnitude * force_direction;
1579 fmt::format(
"Cannot add free force of type 'TOWARDS_NODE' with ID {} to actor {}: Target actor not found or disposed", freeforce.
ffc_id, rq->
ffr_target_actor));
1610 fmt::format(
"Cannot add free force with ID {}: ID already in use", rq->
ffr_id));
1644 fmt::format(
"Cannot remove free force with ID {}: ID not found",
id));
#define ROR_ASSERT(_EXPR)
Game state manager and message-queue provider.
float ar_avg_wheel_speed
Physics state; avg wheel speed in m/s.
static const int MAX_COMMANDS
maximum number of commands per actor
void SetDebugView(DebugViewType dv)
void resetPosition(Ogre::Vector3 translation, bool setInitPosition)
Moves the actor to given world coords.
bool cc_mode
Cruise Control.
< Must preserve mem. layout of RoRnet::StreamRegister
float GetSimulationSpeed() const
int CheckNetworkStreamsOk(int sourceid)
Softbody object; can be anything from soda can to a space shuttle Constructed from a truck definition...
VehicleAIPtr ar_vehicle_ai
bool asr_free_position
Disables the automatic spawn position adjustment.
@ MSG2_STREAM_UNREGISTER
remove stream
void BITMASK_SET(BitMask_t &mask, BitMask_t flag, bool val)
Ogre::String m_section_config
@ NETWORK
Remote controlled.
@ MSG_SIM_MODIFY_ACTOR_REQUESTED
Payload = RoR::ActorModifyRequest* (owner)
NodeNum_t ffc_target_node
bool ar_physics_paused
Sim state.
int32_t time
initial time stamp
void UpdateSimDataBuffer()
Copies sim. data from Actor to GfxActor for later update.
@ TRUCK
its a truck (or other land vehicle)
std::string ar_filename
Attribute; filled at spawn.
int GetNetTimeOffset(int sourceid)
static const ActorPtr ACTORPTR_NULL
void ConfigureSections(Ogre::String const §ionconfig, RigDef::DocumentPtr def)
bool ModifyTyrePressure(float v)
@ CONFIG_FILE
'Preselected vehicle' in RoR.cfg or command line
unsigned int ar_vector_index
Sim attr; actor element index in std::vector<m_actors>
Ogre::Vector3 AbsPosition
absolute position in the world (shaky)
Estabilishing a physics linkage between 2 actors modifies a global linkage table and triggers immedia...
ActorPtrVec ar_linked_actors
BEWARE: Includes indirect links, see DetermineLinkedActors(); Other actors linked using 'hooks/ties/r...
ActorInstanceID_t ar_instance_id
Static attr; session-unique ID.
int m_net_first_wheel_node
Network attr; Determines data buffer layout; calculated on spawn.
void HandleErrorLoadingTruckfile(std::string filename, std::string exception_msg)
Ogre::AxisAlignedBox ar_bounding_box
standard bounding box (surrounds all nodes of an actor)
RigDef::DocumentPtr GetFile()
CVar * gfx_skidmarks_mode
static const NodeNum_t NODENUM_INVALID
ground_model_t * defaultgm
Truck file format(technical spec)
void AddFreeForce(FreeForceRequest *rq)
void HandleErrorLoadingFile(std::string type, std::string filename, std::string exception_msg)
Ogre::Vector3 lo
absolute collision box
std::map< beam_t *, std::pair< ActorPtr, ActorPtr > > inter_actor_links
@ DISPOSED
removed from simulation, still in memory to satisfy pointers.
@ LIGHTMASK_REVERSE
reverse light on
void RemoveStreamSource(int sourceid)
ActorLinkingRequestType alr_type
Ogre::Real m_min_camera_radius
void TRIGGER_EVENT_ASYNC(scriptEvents type, int arg1, int arg2ex=0, int arg3ex=0, int arg4ex=0, std::string arg5ex="", std::string arg6ex="", std::string arg7ex="", std::string arg8ex="")
Asynchronously (via MSG_SIM_SCRIPT_EVENT_TRIGGERED) invoke script function eventCallbackEx(),...
Ogre::Real ar_brake
Physics state; braking intensity.
bool sl_enabled
Speed limiter;.
ActorPtr FindActorInsideBox(Collisions *collisions, const Ogre::String &inst, const Ogre::String &box)
Checks the rig-def file syntax and pulls data to File object.
bool CheckActorCollAabbIntersect(int a, int b)
Returns whether or not the bounding boxes of truck a and truck b intersect. Based on the truck collis...
Ogre::Real Round(Ogre::Real value, unsigned short ndigits=0)
float ffc_force_magnitude
void LogFormat(const char *format,...)
Improved logging utility. Uses fixed 2Kb buffer.
void SplitBundleQualifiedFilename(const std::string &bundleQualifiedFilename, std::string &out_bundleName, std::string &out_filename)
int32_t origin_sourceid
origin sourceid
Ogre::Vector3 hi
absolute collision box
bool ar_toggle_ropes
Sim state.
void putMessage(MessageArea area, MessageType type, std::string const &msg, std::string icon="")
std::vector< hook_t > ar_hooks
A land vehicle engine + transmission.
bool ar_trailer_parking_brake
Ogre::String resource_group
Resource group of the loaded bundle. Empty if not loaded yet.
ActorPtrVec & GetActors()
This creates a billboarding object that displays a text.
float sl_speed_limit
Speed limiter;.
void ConfigureAddonParts(TuneupDefPtr &tuneup_def)
const ActorPtr & GetActorByNetworkLinks(int source_id, int stream_id)
@ SE_GENERIC_NEW_TRUCK
triggered when the user spawns a new actor, the argument refers to the actor ID
const ActorPtr & FetchPreviousVehicleOnList(ActorPtr player, ActorPtr prev_player)
void FinishFlexbodyTasks()
ActorPtr ffc_target_actor
ScriptUnitId_t loadScript(Ogre::String scriptname, ScriptCategory category=ScriptCategory::TERRAIN, ActorPtr associatedActor=nullptr, std::string buffer="")
Loads a script.
std::pair< ActorPtr, float > GetNearestActor(Ogre::Vector3 position)
void Setup(RigDef::DocumentPtr file)
Prepares the validation.
@ LOCAL_SIMULATED
simulated (local) actor
bool AreActorsDirectlyLinked(const ActorPtr &a1, const ActorPtr &a2)
std::string asr_filename
Can be in "Bundle-qualified" format, i.e. "mybundle.zip:myactor.truck".
Ogre::Vector3 RelPosition
relative to the local physics origin (one origin per actor) (shaky)
void SetAcceleration(float val)
std::map< int, int > m_stream_time_offsets
Networking: A network time offset for each stream source.
void ProcessOgreStream(Ogre::DataStream *stream, Ogre::String resource_group)
bool ar_forward_commands
Sim state.
static const NodeNum_t NODENUM_MAX
Ogre::Vector3 center
center of rotation
ScriptEngine * GetScriptEngine()
CVar * mp_cyclethru_net_actors
Include remote actors when cycling through with CTRL + [ and CTRL + ].
Processes a RigDef::Document (parsed from 'truck' file format) into a simulated gameplay object (Acto...
size_t m_net_wheel_buf_size
For incoming/outgoing traffic; calculated on spawn.
float ar_wheel_speed
Physics state; wheel speed in m/s.
void SendAllActorsSleeping()
float initial_beam_strength
for reset
void AddStreamMismatch(int sourceid, int streamid)
void FinishWheelUpdates()
Ogre::Vector3 ffr_force_const_direction
ActorInstanceID_t amr_actor
void UpdateProps(float dt, bool is_player_actor)
bool m_disable_default_sounds
Spawner context; TODO: remove.
float getAvgPropedWheelRadius()
bool isPreloadedWithTerrain() const
bool isActive()
Returns the status of the AI.
float m_simulation_speed
slow motion < 1.0 < fast motion
std::map< int, std::set< int > > m_stream_mismatches
Networking: A set of streams without a corresponding actor in the actor-array for each stream source.
@ TOWARDS_COORDS
Constant force directed towards ffc_target_coords
int ActorInstanceID_t
Unique sequentially generated ID of an actor in session. Use ActorManager::GetActorById()
void CalcFreeForces()
Apply FreeForces - intentionally as a separate pass over all actors.
void UpdateSleepingState(ActorPtr player_actor, float dt)
bool PredictActorCollAabbIntersect(int a, int b)
Returns whether or not the bounding boxes of truck a and truck b might intersect during the next fram...
@ MSG2_STREAM_DATA
stream data
uint16_t NodeNum_t
Node position within Actor::ar_nodes; use RoR::NODENUM_INVALID as empty value.
float m_dt_remainder
Keeps track of the rounding error in the time step calculation.
CacheEntryPtr FindEntryByFilename(RoR::LoaderType type, bool partial, const std::string &_filename_maybe_bundlequalified)
Returns NULL if none found; "Bundle-qualified" format also specifies the ZIP/directory in modcache,...
ground_model_t * getGroundModelByString(const Ogre::String name)
void RemoveFreeForce(FreeForceID_t id)
Performs a formal validation of the file (missing required parts, conflicts of modules,...
FreeForceVec_t::iterator FindFreeForce(FreeForceID_t id)
Ogre::Vector3 ffc_force_const_direction
Expected to be normalized; only effective with FreeForceType::CONSTANT
void unloadScript(ScriptUnitId_t unique_id)
Unloads a script.
void RegisterGfxActor(RoR::GfxActor *gfx_actor)
A database of user-installed content alias 'mods' (vehicles, terrains...)
Ogre::Vector3 relo
relative collision box
std::shared_ptr< Task > m_sim_task
void ConfigureAssetPacks(ActorPtr actor, RigDef::DocumentPtr def)
void HandleActorStreamData(std::vector< RoR::NetRecvPacket > packet)
@ MSG2_STREAM_REGISTER
create new stream
CacheEntryPtr asr_cache_entry
Optional, overrides 'asr_filename' and 'asr_cache_entry_num'.
TyrePressure & getTyrePressure()
void PushMessage(Message m)
Doesn't guarantee order! Use ChainMessage() if order matters.
std::vector< ActorPtr > GetLocalActors()
std::unique_ptr< ThreadPool > m_sim_thread_pool
Facilitates execution of (small) tasks on separate threads.
int ScriptUnitId_t
Unique sequentially generated ID of a loaded and running scriptin session. Use ScriptEngine::getScrip...
static ActorInstanceID_t m_actor_counter
void updateVisual(float dt=0)
void ProcessNewActor(ActorPtr actor, ActorSpawnRequest rq, RigDef::DocumentPtr def)
Ogre::Vector3 ar_origin
Physics state; base position for softbody nodes.
const char * ToCStr() const
@ LOCAL_SLEEPING
sleeping (local) actor
int FreeForceID_t
Unique sequentially generated ID of FreeForce; use ActorManager::GetFreeForceNextId().
RoR::SimGearboxMode GetAutoShiftMode()
CmdKeyArray ar_command_key
BEWARE: commandkeys are indexed 1-MAX_COMMANDS!
@ CONSTANT
Constant force given by direction and magnitude.
Collisions * GetCollisions()
RigDef::DocumentPtr actor_def
Cached actor definition (aka truckfile) after first spawn.
Ogre::Vector3 getPosition()
Ogre::Vector3 ffr_target_coords
bool nd_tyre_node
Attr; This node is part of a tyre (note some wheel types don't use rim nodes at all)
void NotifyActorCameraChanged()
Logic: sound, display; Notify this vehicle that camera changed;.
void ForceFeedbackStep(int steps)
void WriteDiagnosticDump(std::string const &filename)
bool ar_import_commands
Sim state.
int FindPivotActorId(ActorPtr player, ActorPtr prev_player)
void UpdateNetTimeOffset(int sourceid, int offset)
Central state/object manager and communications hub.
size_t m_net_propanimkey_buf_size
For incoming/outgoing traffic; calculated on spawn.
@ MSG2_STREAM_REGISTER_RESULT
result of a stream creation
std::vector< std::pair< float, float > > ar_initial_beam_defaults
GameContext * GetGameContext()
void UpdateCruiseControl(float dt)
Defined in 'gameplay/CruiseControl.cpp'.
const ActorPtr & FetchRescueVehicle()
BitMask_t m_lightmask
RoRnet::Lightmask.
float m_dry_mass
Physics attr;.
double ffr_force_magnitude
void SetCheckBeams(bool check_beams)
Global force affecting particular (base) node of particular (base) actor; added ad-hoc by scripts.
std::vector< PropAnimKeyState > m_prop_anim_key_states
float ar_initial_total_mass
float ar_sleep_counter
Sim state; idle time counter.
Ogre::Vector3 getDirection()
average actor velocity, calculated using the actor positions of the last two frames
@ TERRN_DEF
Preloaded with terrain.
CacheEntryPtr asr_skin_entry
@ LIGHTMASK_BRAKES
brake lights on
float m_simulation_time
Amount of time the physics simulation is going to be advanced.
void SetSimulationSpeed(float speed)
bool m_preloaded_with_terrain
Spawn context (TODO: remove!)
Replay * m_replay_handler
float getTotalMass(bool withLocked=true)
int GetGear()
low level gear changing
size_t m_net_total_buffer_size
For incoming/outgoing traffic; calculated on spawn.
float m_last_simulation_speed
previously used time ratio between real time (evt.timeSinceLastFrame) and physics time ('dt' used in ...
void RecalculateNodeMasses(Ogre::Real total)
Previously 'calc_masses2()'.
int ar_num_cinecams
Sim attr;.
int32_t status
initial stream status
void RecursiveActivation(int j, std::vector< bool > &visited)
std::string SanitizeUtf8CString(const char *start, const char *end=nullptr)
float m_total_mass
Physics state; total mass in Kg.
int32_t type
0 = Actor, 1 = Character, 3 = ChatSystem
void DeleteActorInternal(ActorPtr actor)
Do not call directly; use GameContext::DeleteActor()
size_t m_net_node_buf_size
For incoming/outgoing traffic; calculated on spawn.
CVar * sim_realistic_commands
std::vector< Ogre::Vector3 > ar_initial_node_positions
CacheSystem * GetCacheSystem()
std::string Sha1Hash(std::string const &data)
CacheEntryPtr FetchSkinByName(std::string const &skin_name)
static bool ProcessFreeForce(FreeForceRequest *rq, FreeForce &freeforce)
int32_t colournum
colour set by server
Common for ADD and MODIFY requests; tailored for use with AngelScript thru GameScript::pushMessage().
char username[RORNET_MAX_USERNAME_LEN]
the nickname of the user (UTF-8)
bool ar_toggle_ties
Sim state.
bool nd_rim_node
Attr; This node is part of a rim (only wheel types with separate rim nodes)
void LoadResource(CacheEntryPtr &t)
Loads the associated resource bundle if not already done.
@ MSG_SIM_ACTOR_LINKING_REQUESTED
Payload = RoR::ActorLinkingRequest* (owner)
Unified game event system - all requests and state changes are reported using a message.
std::vector< float > ar_initial_node_masses
void putNetMessage(int user_id, MessageType type, const char *text)
#define SOUND_PLAY_ONCE(_ACTOR_, _TRIG_)
void dispose()
Effectively destroys the object but keeps it in memory to satisfy shared pointers.
Ogre::UTFString asr_net_username
void StartEngine()
Quick engine start. Plays sounds.
Ogre::UTFString m_net_username
void UpdateInputEvents(float dt)
TuneupDefPtr m_working_tuneup_def
Each actor gets unique instance, even if loaded from .tuneup file in modcache.
@ TOWARDS_NODE
Constant force directed towards ffc_target_node
@ MSG_SIM_SPAWN_ACTOR_REQUESTED
Payload = RoR::ActorSpawnRequest* (owner)
int32_t origin_streamid
origin streamid
InputEngine * GetInputEngine()
void ModifyFreeForce(FreeForceRequest *rq)
void EraseIf(std::vector< T, A > &c, Predicate pred)
Ogre::Vector3 m_avg_node_position
average node position
RefCountingObjectPtr< Actor > ActorPtr
ActorInstanceID_t alr_actor_instance_id
@ MSG_SIM_DELETE_ACTOR_REQUESTED
Payload = RoR::ActorPtr* (owner)
bool ShouldIncludeActorInList(const ActorPtr &actor)
@ ACTOR
Defined in truck file under 'scripts', contains global variable BeamClass@ thisActor.
void UpdateTruckFeatures(ActorPtr vehicle, float dt)
void ForwardCommands(ActorPtr source_actor)
Fowards things to trailers.
bool isInside(Ogre::Vector3 pos, const Ogre::String &inst, const Ogre::String &box, float border=0)
ground_model_t * ar_submesh_ground_model
void OffStart()
Quick start of vehicle engine.
@ NETWORKED_HIDDEN
not simulated, not updated (remote)
RigDef::DocumentPtr FetchActorDef(RoR::ActorSpawnRequest &rq)
int m_wheel_node_count
Static attr; filled at spawn.
static void SetupDefaultSoundSources(ActorPtr const &actor)
void RepairActor(Collisions *collisions, const Ogre::String &inst, const Ogre::String &box, bool keepPosition=false)
std::shared_ptr< Document > DocumentPtr
@ CONSOLE_MSGTYPE_INFO
Generic message.
void calculateAveragePosition()
bool m_forced_awake
disables sleep counters
Ogre::Vector3 asr_position
std::string GetSubmeshGroundmodelName()
int ar_net_source_id
Unique ID of remote player who spawned this actor.
@ NETWORKED_OK
not simulated (remote) actor
void Parallelize(const std::vector< std::function< void()>> &task_funcs)
Run collection of tasks in parallel and wait until all have finished.
ThreadPool * GetThreadPool()
void UpdateActors(ActorPtr player_actor)
Ogre::UTFString tryConvertUTF(const char *buffer)
const ActorPtr & FetchNextVehicleOnList(ActorPtr player, ActorPtr prev_player)
BitMask_t getLightStateMask() const
void UpdateWheelVisuals()
@ AI
machine controlled by an Artificial Intelligence
ActorMemoryRequirements const & GetMemoryRequirements()
Ogre::Vector3 ffc_target_coords
void AddPacket(int streamid, int type, int len, const char *content)
collision_box_t * asr_spawnbox
Ogre::Quaternion asr_rotation
void UpdateBoundingBoxes()
const ActorPtr & GetActorById(ActorInstanceID_t actor_id)
FreeForceVec_t m_free_forces
Global forces added ad-hoc by scripts.
float default_beam_deform
for reset
void CleanUpSimulation()
Call this after simulation loop finishes.
ActorManager * GetActorManager()
@ MSG2_USER_LEAVE
user leaves
ActorPtr CreateNewActor(ActorSpawnRequest rq, RigDef::DocumentPtr def)
void UpdatePhysicsSimulation()
CVar * sim_replay_enabled
int CheckNetRemoteStreamsOk(int sourceid)
CVar * mp_pseudo_collisions
bool isBeingReset() const
const TerrainPtr & GetTerrain()
Ogre::String fname
filename
void calcNodeConnectivityGraph()
< Sent from the client to server and vice versa, to broadcast a new stream