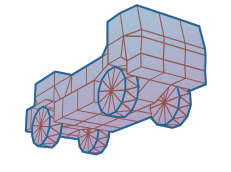 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
33 #include <OgreStringConverter.h>
35 #include <unordered_map>
44 m_inertia_function_width(10),
46 m_command_key_width(2),
54 <<
"; ---------------------------------------------------------------------------- ;" << endl
55 <<
"; Project: Rigs of Rods (http://www.rigsofrods.org) ;" << endl
56 <<
"; File format: https://docs.rigsofrods.org/vehicle-creation/fileformat-truck ;" << endl
57 <<
"; ---------------------------------------------------------------------------- ;" << endl
77 for (
auto module_itor:
m_rig_def->user_modules)
92 m_stream <<
"section -1 " << m->name << endl;
181 for (
auto itor = module->
pistonprops.begin(); itor != end_itor; ++itor)
193 <<
", " << setw(
m_node_id_width) << (def.couple_node.IsValidAnyState() ? def.couple_node.Str() :
"-1")
196 <<
", " << def.airfoil
210 for (
auto itor = module->
turbojets.begin(); itor != end_itor; ++itor)
219 <<
", " << setw(2) << def.is_reversable
238 for (
auto itor = module->
screwprops.begin(); itor != end_itor; ++itor)
284 for (
auto itor = module->
turboprops2.begin(); itor != end_itor; ++itor)
311 for (
auto itor = module->
airbrakes.begin(); itor != end_itor; ++itor)
339 if (module->
wings.empty())
344 auto end_itor = module->
wings.end();
345 for (
auto itor = module->
wings.begin(); itor != end_itor; ++itor)
386 for (
auto itor = module->
soundsources.begin(); itor != end_itor; ++itor)
404 m_stream <<
"soundsources2" << endl;
406 for (
auto itor = module->
soundsources2.begin(); itor != end_itor; ++itor)
413 <<
", " << setw(2) << def.
mode
431 case ExtCameraMode::NODE:
434 case ExtCameraMode::CINECAM:
437 case ExtCameraMode::CLASSIC:
453 for (
auto itor = module->
videocameras.begin(); itor != end_itor; ++itor)
521 auto end_itor = module->
exhausts.end();
522 for (
auto itor = module->
exhausts.begin(); itor != end_itor; ++itor)
556 for (
auto texcoord_itor = def.
texcoords.begin(); texcoord_itor != texcoord_end; ++texcoord_itor)
565 for (
auto cab_itor = def.
cab_triangles.begin(); cab_itor != cab_end; ++cab_itor)
593 inline void PropAnimFlag(std::stringstream& out,
int flags,
bool& join,
unsigned int mask,
const char* name,
char joiner =
'|')
679 for (
auto itor = module->
flexbodies.begin(); itor != end_itor; ++itor)
701 auto forset_itor = def->
node_list.begin();
703 for (; forset_itor != forset_end; ++forset_itor)
717 for (
auto anim_itor = def->
animations.begin(); anim_itor != anim_end; ++anim_itor)
727 if (module->
props.empty())
732 auto end_itor = module->
props.end();
733 for (
auto itor = module->
props.begin(); itor != end_itor; ++itor)
776 for (
auto anim_itor = def.
animations.begin(); anim_itor != anim_end; ++anim_itor)
790 m_stream <<
"materialflarebindings" << endl;
808 auto end_itor = module->
flares2.end();
809 for (
auto itor = module->
flares2.begin(); itor != end_itor; ++itor)
821 <<
", " << (char)def.
type
839 size_t name_w = 0, type_w = 0, tex1_w = 0, tex2_w = 1, tex3_w = 1;
842 name_w = std::max(name_w, mm.
name.length());
849 m_stream <<
"managedmaterials" << std::left << endl;
862 << setw(name_w) << def.
name <<
" "
873 m_stream << setw(tex2_w) <<
"" <<
" ";
892 m_stream <<
"collisionboxes" << endl;
894 for (
auto itor = module->
collisionboxes.begin(); itor != end_itor; ++itor)
900 auto nodes_end = def.
nodes.end();
901 auto node_itor = def.
nodes.begin();
904 for (; node_itor != nodes_end; ++node_itor)
906 m_stream <<
", " << node_itor->Str();
916 if (module->
axles.empty())
921 auto end_itor = module->
axles.end();
922 for (
auto itor = module->
axles.begin(); itor != end_itor; ++itor)
935 for (
auto itor = def.
options.begin(); itor != end; ++itor)
954 for (
auto itor = module->
interaxles.begin(); itor != end_itor; ++itor)
967 for (
auto itor = def.
options.begin(); itor != end; ++itor)
1032 if (module->
torquecurve[0].predefined_func_name.empty())
1034 auto itor_end = module->
torquecurve[0].samples.end();
1035 auto itor = module->
torquecurve[0].samples.begin();
1036 for (; itor != itor_end; ++itor)
1055 auto end_itor = module->
particles.end();
1056 for (
auto itor = module->
particles.begin(); itor != end_itor; ++itor)
1078 auto end_itor = module->
ropables.end();
1079 for (
auto itor = module->
ropables.begin(); itor != end_itor; ++itor)
1086 <<
", " << def.
group
1095 if (module->
ties.empty())
1100 auto end_itor = module->
ties.end();
1101 for (
auto itor = module->
ties.begin(); itor != end_itor; ++itor)
1115 <<
", " << def.
group;
1123 if (module->
fixes.empty())
1128 auto end_itor = module->
fixes.end();
1129 for (
auto itor = module->
fixes.begin(); itor != end_itor; ++itor)
1138 if (module->
ropes.empty())
1143 auto end_itor = module->
ropes.end();
1146 for (
auto itor = module->
ropes.begin(); itor != end_itor; ++itor)
1176 m_stream <<
"railgroups" << endl << endl;
1178 for (
auto itor = module->
railgroups.begin(); itor != end_itor; ++itor)
1186 for (
auto node_itor = def.
node_list.begin(); node_itor != node_end; ++node_itor)
1188 m_stream <<
", " << node_itor->start.Str();
1189 if (node_itor->IsRange())
1191 m_stream <<
" - " << node_itor->end.Str();
1205 m_stream <<
"slidenodes" << endl << endl;
1207 for (
auto itor = module->
slidenodes.begin(); itor != end_itor; ++itor)
1220 for (; itor != end; ++itor)
1222 m_stream <<
", " << itor->start.Str();
1223 if (itor->IsRange())
1225 m_stream <<
" - " << itor->end.Str();
1254 if (module->
hooks.empty())
1258 m_stream <<
"hooks" << endl << endl;
1259 auto end_itor = module->
hooks.end();
1260 for (
auto itor = module->
hooks.begin(); itor != end_itor; ++itor)
1296 m_stream <<
"lockgroups" << endl << endl;
1298 for (
auto itor = module->
lockgroups.begin(); itor != end_itor; ++itor)
1305 auto nodes_end = def.
nodes.end();
1306 for (
auto nodes_itor = def.
nodes.begin(); nodes_itor != nodes_end; ++nodes_itor)
1308 m_stream <<
", " << nodes_itor->Str();
1321 m_stream <<
"animators" << endl << endl;
1322 auto end_itor = module->
triggers.end();
1323 for (
auto itor = module->
triggers.begin(); itor != end_itor; ++itor)
1355 #define ANIMATOR_ADD_FLAG(DEF_VAR, AND_VAR, BITMASK_CONST, NAME_STR) \
1356 if (AND_VAR) { m_stream << " | "; } \
1357 if (BITMASK_IS_1((DEF_VAR).flags, RigDef::Animator::BITMASK_CONST)) { \
1359 m_stream << NAME_STR; \
1362 #define ANIMATOR_ADD_AERIAL_FLAG(DEF_VAR, AND_VAR, BITMASK_CONST, NAME_STR) \
1363 if (AND_VAR) { m_stream << " | "; } \
1364 if (BITMASK_IS_1((DEF_VAR).aero_animator.flags, RigDef::AeroAnimator::BITMASK_CONST)) { \
1366 m_stream << NAME_STR << DEF_VAR.aero_animator.engine_idx + 1; \
1369 #define ANIMATOR_ADD_LIMIT(DEF_VAR, AND_VAR, BITMASK_CONST, NAME_STR, VALUE) \
1370 if (AND_VAR) { m_stream << " | "; } \
1371 if (BITMASK_IS_1((DEF_VAR).aero_animator.flags, RigDef::Animator::BITMASK_CONST)) { \
1373 m_stream << NAME_STR << ": " << VALUE; \
1382 m_stream <<
"animators" << endl << endl;
1383 auto end_itor = module->
animators.end();
1384 for (
auto itor = module->
animators.begin(); itor != end_itor; ++itor)
1446 m_stream <<
"contacters" << endl << endl;
1462 m_stream <<
"rotators" << endl << endl;
1463 auto end_itor = module->
rotators.end();
1464 for (
auto itor = module->
rotators.begin(); itor != end_itor; ++itor)
1476 for (
int i = 0; i < 4; ++i)
1482 for (
int i = 0; i < 4; ++i)
1513 size_t desc_w = 0, startf_w = 0, stopf_w = 0;
1516 desc_w = std::max(desc_w, def.
description.length());
1522 m_stream <<
"rotators2" << endl << endl;
1523 auto end_itor = module->
rotators2.end();
1524 for (
auto itor = module->
rotators2.begin(); itor != end_itor; ++itor)
1535 for (
int i = 0; i < 4; ++i)
1541 for (
int i = 0; i < 4; ++i)
1577 m_stream <<
"flexbodywheels" << endl << endl;
1579 for (
auto itor = module->
flexbodywheels.begin(); itor != end_itor; ++itor)
1581 this->
UpdatePresets(itor->beam_defaults.get(), itor->node_defaults.get(),
nullptr);
1588 << setw(3) << itor->num_rays <<
", "
1592 << setw(3) << (int)itor->braking <<
", "
1593 << setw(3) << (int)itor->propulsion <<
", "
1600 << (
static_cast<char>(itor->side)) <<
", "
1601 << itor->rim_mesh_name <<
" "
1602 << itor->tyre_mesh_name
1620 <<
"mode: " << (def.
attr_is_on ?
"ON" :
"OFF");
1631 if (module->
brakes.size() == 0) {
return; }
1652 <<
"mode: " << (alb->
attr_is_on ?
"ON" :
"OFF");
1662 if (module->
engine.size() == 0)
1677 " Forward gears...\n\t"
1680 << setw(10) << engine.
torque <<
", "
1687 for (; itor != end; ++itor)
1691 m_stream <<
", -1.0" << endl << endl;
1717 << setw(10) << engoption.
inertia <<
", "
1718 << setw(10) << (char)engoption.
type <<
", "
1723 << setw( 8) << engoption.
stall_rpm <<
", "
1724 << setw( 7) << engoption.
idle_rpm <<
", "
1734 if (module->
help.size() == 0)
1738 m_stream <<
"help\n\t" << module->
help[module->
help.size() - 1].material << endl << endl;
1749 m_stream <<
"wheels2" << endl << endl;
1750 auto end_itor = module->
wheels2.end();
1751 for (
auto itor = module->
wheels2.begin(); itor != end_itor; ++itor)
1753 this->
UpdatePresets(itor->beam_defaults.get(), itor->node_defaults.get(),
nullptr);
1760 << setw(3) << itor->num_rays <<
", "
1764 << setw(3) << (int)itor->braking <<
", "
1765 << setw(3) << (int)itor->propulsion <<
", "
1772 << itor->face_material_name <<
" "
1773 << itor->band_material_name <<
" "
1783 if (module->
wheels.empty())
1789 m_stream <<
"wheels" << endl << endl;
1793 << setw(3) <<
"nrays, "
1797 << setw(3) <<
"brk, "
1798 << setw(3) <<
"pwr, "
1806 auto end_itor = module->
wheels.end();
1807 for (
auto itor = module->
wheels.begin(); itor != end_itor; ++itor)
1809 this->
UpdatePresets(itor->beam_defaults.get(), itor->node_defaults.get(),
nullptr);
1815 << setw(3) << itor->num_rays <<
", "
1819 << setw(3) << (int)itor->braking <<
", "
1820 << setw(3) << (int)itor->propulsion <<
", "
1825 << itor->face_material_name <<
" "
1826 << itor->band_material_name <<
" "
1846 << setw(3) << (int)def.
braking <<
", "
1852 << (
static_cast<char>(def.
side)) <<
", "
1864 m_stream <<
"meshwheels" <<
"\n\n";
1881 m_stream <<
"meshwheels2" <<
"\n\n";
1899 for (
auto& camera: module->
cameras)
1916 m_stream <<
"cinecam" << endl << endl;
1918 for (
auto itor = module->
cinecam.begin(); itor != module->
cinecam.end(); ++itor)
1945 if (module->
beams.empty())
1951 m_stream <<
"beams" << endl << endl;
1954 for (
size_t i = 0; i < module->
beams.size(); i++)
1957 if (prev_defaults != beam.
defaults.get())
1960 prev_defaults = beam.
defaults.get();
1972 if (module->
shocks.empty())
1978 m_stream <<
"shocks" << endl << endl;
1981 for (
size_t i = 0; i < module->
shocks.size(); i++)
2005 m_stream <<
"shocks2" << endl << endl;
2008 for (
size_t i = 0; i < module->
shocks2.size(); i++)
2032 m_stream <<
"shocks3" << endl << endl;
2035 for (
size_t i = 0; i < module->
shocks3.size(); i++)
2054 if (module->
hydros.empty())
2060 m_stream <<
"hydros" << endl << endl;
2063 for (
size_t i = 0; i < module->
hydros.size(); i++)
2087 m_stream <<
"commands" << endl << endl;
2090 for (
size_t i = 0; i < module->
commands2.size(); i++)
2174 if (inertia.start_delay_factor != 0 && inertia.stop_delay_factor != 0)
2179 if (!inertia.start_function.empty())
2182 if (!inertia.stop_function.empty())
2329 if (beam_defaults !=
nullptr)
2385 if (module->
nodes.empty())
2391 m_stream <<
"nodes" << endl << endl;
2393 size_t num_named = 0;
2396 for (
size_t i = 0; i < module->
nodes.size(); i++)
2399 if (node.
id.IsTypeNamed())
2405 if (preset != prev_preset)
2408 prev_preset = preset;
2412 if (dminimass != prev_dminimass)
2415 prev_dminimass = dminimass;
2424 m_stream << endl << endl <<
"nodes2" << endl;
2426 for (
size_t i = 0; i < module->
nodes.size(); i++)
2429 if (!node.
id.IsTypeNamed())
2435 if (preset != prev_preset)
2438 prev_preset = preset;
2442 if (dminimass != prev_dminimass)
2445 prev_dminimass = dminimass;
2459 if (node_defaults !=
nullptr)
2519 if (
m_rig_def->enable_advanced_deformation)
2521 m_stream <<
"enable_advanced_deformation" << endl << endl;
2525 m_stream <<
"hideInChooser" << endl << endl;
2527 if (
m_rig_def->slide_nodes_connect_instantly)
2529 m_stream <<
"slidenode_connect_instantly" << endl << endl;
2531 if (
m_rig_def->lockgroup_default_nolock)
2533 m_stream <<
"lockgroup_default_nolock" << endl << endl;
2537 m_stream <<
"rollon" << endl << endl;
2541 m_stream <<
"rescuer" << endl << endl;
2545 m_stream <<
"disabledefaultsounds" << endl << endl;
2549 m_stream <<
"forwardcommands" << endl << endl;
2553 m_stream <<
"importcommands" << endl << endl;
2574 if (! module->
guid.empty())
2576 m_stream <<
"guid " << module->
guid[module->
guid.size() - 1].guid << endl << endl;
2587 std::string line = *itor;
2590 m_stream << endl <<
"end_description" << endl << endl;
2596 for (
auto itor = module->
author.begin(); itor != module->
author.end(); ++itor)
2615 if (module->
globals.size() == 0)
2621 << module->
globals[0].dry_mass <<
", "
2622 << module->
globals[0].cargo_mass;
2623 if (!module->
globals[0].material_name.empty())
2661 [
keyword, vectorpos](
const RigDef::DocComment& dc) { return dc.commented_keyword == keyword && dc.commented_datapos == (int)vectorpos; });
#define ANIMATOR_ADD_LIMIT(DEF_VAR, AND_VAR, BITMASK_CONST, NAME_STR, VALUE)
void ProcessDefaultMinimass(DefaultMinimass *default_minimass)
float min_mass_Kg
minimum node mass in Kg
static const BitMask_t OPTION_u_INPUT_AILERON_ELEVATOR
static const BitMask64_t SOURCE_EVENT
std::shared_ptr< BeamDefaults > beam_defaults
std::vector< Globals > globals
DifferentialTypeVec options
Order matters!
float spring_out
spring value applied when shock extending
static const BitMask64_t SOURCE_ANGLE_OF_ATTACK
static const BitMask_t OPTION_c_COMMAND_STYLE
#define ANIMATOR_ADD_AERIAL_FLAG(DEF_VAR, AND_VAR, BITMASK_CONST, NAME_STR)
static const BitMask_t OPTION_e_INPUT_ELEVATOR
void ProcessWings(Document::Module *module)
std::shared_ptr< BeamDefaults > beam_defaults
static const BitMask_t CONSTRAINT_ATTACH_FOREIGN
BitMask_t constraint_flags
static const BitMask64_t SOURCE_FLAP
static const BitMask64_t SOURCE_VERTICAL_VELOCITY
static const BitMask_t OPTION_A_INV_TRIGGER_BLOCKER
Ogre::String material_name
float damp_out_fast
Damping value applied when shock is commpressing faster than split out velocity.
float extension_break_limit
void ProcessBrakes(Document::Module *module)
Node::Ref blade_tip_nodes[4]
static const BitMask_t OPTION_p_10xTOUGHER
void ProcessFileinfo(Document::Module *module)
void ProcessBeamDefaults(BeamDefaults *beam_defaults)
std::vector< Screwprop > screwprops
void ProcessMeshWheels2(Document::Module *module)
std::shared_ptr< BeamDefaults > beam_defaults
void ProcessFlexBodyWheels(Document::Module *module)
static const BitMask_t OPTION_x_EXHAUST_POINT
void ProcessNodes(Document::Module *)
NodeDefaults * m_current_node_defaults
Legacy parser resolved references on-the-fly and the condition to check named nodes was "are there an...
void ProcessRotators2(Document::Module *module)
void ProcessVideocamera(Document::Module *module)
unsigned int options
Bit flags.
std::vector< Hook > hooks
float default_braking_force
bool option_o_1press_center
std::vector< Fusedrag > fusedrag
std::vector< Brakes > brakes
WingControlSurface control_surface
static const BitMask_t OPTION_s_SOFT_BUMP_BOUNDS
int m_inertia_function_width
void ProcessTurboprops(Document::Module *module)
void ProcessGuiSettings(Document::Module *module)
void ProcessShocks3(Document::Module *)
Ogre::String start_function
unsigned int texture_width
void ProcessTurbojets(Document::Module *module)
float post_shift_time
Seconds.
static const BitMask_t OPTION_R_ACTIVE_RIGHT
const std::string & Str() const
float long_bound
Maximum extension. The longest length a shock can be, as a proportion of its original length....
std::vector< DocComment > _comments
int mode
A special constant or cinecam index.
static const float BEAM_DEFORM
std::vector< Wheel > wheels
@ y_INPUT_InvAILERON_RUDDER
std::vector< float > gear_ratios
unsigned int flare_number
std::vector< Pistonprop > pistonprops
float split_vel_out
Split velocity in (m/s) - threshold for slow / fast damping during extension.
@ s_CMD_NUM_SWITCH
switch that exchanges cmdshort/cmdshort for all triggers with the same commandnumbers,...
std::vector< Lockgroup > lockgroups
static const BitMask_t MODE_BOUNCE
std::vector< Shock2 > shocks2
static const BitMask64_t SOURCE_TACHO
std::vector< Command2 > commands2
void ProcessCommands2(Document::Module *)
Ogre::String damaged_diffuse_map
void ProcessCollisionBoxes(Document::Module *module)
std::vector< Wing > wings
Truck file format(technical spec)
float deformation_threshold
Ogre::String particle_system_name
std::shared_ptr< BeamDefaults > beam_defaults
static const BitMask_t CONSTRAINT_ATTACH_SELF
static const BitMask64_t SOURCE_PARKING
int longbound_trigger_action
DefaultMinimass * m_current_default_minimass
static const BitMask64_t SOURCE_BOAT_RUDDER
float spring_in
Spring value applied when the shock is compressing.
std::vector< CruiseControl > cruisecontrol
std::vector< Rope > ropes
static const BitMask_t OPTION_m_METRIC
void ProcessRopables(Document::Module *module)
static const BitMask_t OPTION_L_ACTIVE_LEFT
void ProcessHydro(Hydro &def)
std::vector< Node::Range > rail_node_ranges
static const BitMask64_t SOURCE_PITCH
std::vector< Airbrake > airbrakes
std::vector< Node::Ref > nodes
float precompression
Changes compression or extension of the suspension when the truck spawns. This can be used to "level"...
std::vector< Node > nodes
static const BitMask_t OPTION_n_INPUT_NORMAL
void ExportBaseMeshWheel(BaseMeshWheel &def)
static const BitMask64_t SOURCE_ALTIMETER_10K
unsigned int spin_right_key
std::vector< SlideNode > slidenodes
RoR::WheelPropulsion propulsion
static const BitMask_t OPTION_p_NO_PARTICLES
static const BitMask_t OPTION_u_INVULNERABLE
std::list< Animation > animations
@ c_COMMAND_STYLE
trigger is set with commandstyle boundaries instead of shocksytle
std::string NodeOptionsToStr(BitMask_t options)
void ProcessShock2(Shock2 &def)
@ B_TRIGGER_BLOCKER
Blocker that enable/disable other triggers.
void ProcessAuthors(Document::Module *module)
static const BitMask_t MODE_EVENT_LOCK
void ProcessShock(Shock &def)
std::vector< Axle > axles
float beam_thickness_meters
std::vector< Author > author
static const BitMask64_t SOURCE_SHIFTERLIN
@ t_CONTINUOUS
this trigger sends values between 0 and 1
static const BitMask64_t SOURCE_AIR_RUDDER
float long_bound
Maximum extension limit, in percentage ( 1.00 = 100% )
bool IsValidAnyState() const
void ProcessEngine(Document::Module *module)
static const BitMask_t OPTION_m_NO_MOUSE_GRAB
static const BitMask64_t SOURCE_AIRSPEED
void ProcessManagedMaterialsAndOptions(Document::Module *module)
void ProcessFusedrag(Document::Module *module)
@ u_INPUT_AILERON_ELEVATOR
std::vector< Submesh > submeshes
static const BitMask_t OPTION_B_TRIGGER_BLOCKER
std::vector< GuiSettings > guisettings
static const BitMask64_t SOURCE_ELEVATOR
RoR::CommandkeyID_t extend_key
static const BitMask64_t SOURCE_ROLL
float damp_in
Damping value applied when the shock is compressing.
float damp_out
damping value applied when shock extending
@ x_START_DISABLED
this trigger is disabled on startup, default is enabled
float clutch_time
Seconds.
float spring_out
Spring value applied when shock extending.
static const BitMask_t MODE_ROTATION_Y
static const float BEAM_BREAK
void ProcessSlideNodes(Document::Module *module)
static const BitMask64_t SOURCE_AILERON
static const BitMask_t OPTION_g_INPUT_ELEVATOR_RUDDER
std::string RigidityNodeToStr(Node::Ref node)
void ProcessTriggers(Document::Module *module)
RoR::VideoCamRole camera_role
std::vector< MeshWheel2 > meshwheels2
float short_bound
Maximum contraction. The shortest length the shock can be, as a proportion of its original length....
static const BitMask_t OPTION_m_METRIC
std::vector< SkeletonSettings > set_skeleton_settings
Node::Ref alt_reference_node
float spring_rate
The 'stiffness' of the shock. The higher the value, the less the shock will move for a given bump.
std::vector< Turbojet > turbojets
void ProcessEngoption(Document::Module *module)
void ProcessRopes(Document::Module *module)
static const BitMask_t MODE_AUTO_ANIMATE
static const BitMask_t OPTION_l_LOAD_WEIGHT
std::stringstream m_stream
ManagedMaterialsOptions options
void ProcessCinecam(Document::Module *)
Ogre::String material_name
std::vector< Node::Ref > contacters
std::vector< Cinecam > cinecam
void ProcessNodeDefaults(NodeDefaults *node_defaults)
bool _has_load_weight_override
std::vector< Wheel2 > wheels2
static const BitMask_t OPTION_i_INVISIBLE
static const BitMask_t OPTION_i_INVISIBLE
bool _has_extension_break_limit
static const BitMask64_t SOURCE_BOAT_THROTTLE
RoR::WheelBraking braking
unsigned int texture_height
std::vector< TransferCase > transfercase
static const BitMask64_t SOURCE_DIFFLOCK
static const BitMask64_t SOURCE_AIR_BRAKE
static const BitMask_t OPTION_M_ABSOLUTE_METRIC
void ProcessPropsAndAnimations(Document::Module *module)
void ProcessContacters(Document::Module *module)
void ProcessTorqueCurve(Document::Module *module)
std::vector< Rotator2 > rotators2
std::vector< Ogre::String > description
void ProcessAnimators(Document::Module *module)
float load_weight_override
void ProcessExtCamera(Document::Module *module)
static const BitMask_t OPTION_x_START_DISABLED
void ProcessWheels2(Document::Module *module)
void ProcessFlares2(Document::Module *module)
const std::string & Str() const
void ProcessPistonprops(Document::Module *module)
static const BitMask_t OPTION_a_INPUT_AILERON
#define BITMASK_IS_1(VAR, FLAGS)
static const BitMask_t MODE_OFFSET_Z
void ProcessCameras(Document::Module *module)
std::vector< Animator > animators
std::vector< Turboprop2 > turboprops2
static const BitMask_t OPTION_c_CONTACT
Node::Ref alt_orientation_node
std::vector< Trigger > triggers
void ProcessGuid(Document::Module *module)
Ogre::String specular_map
void ProcessAntiLockBrakes(Document::Module *module)
float short_bound
Maximum contraction limit, in percentage ( 1.00 = 100% )
float spring_in
Spring value applied when the shock is compressing.
float precompression
Changes compression or extension of the suspension when the truck spawns. This can be used to "level"...
static const BitMask_t MODE_OFFSET_X
static const BitMask_t MODE_ROTATION_Z
std::vector< ExtCamera > extcamera
static const BitMask_t OPTION_v_INPUT_InvAILERON_ELEVATOR
static const BitMask64_t SOURCE_STEERING_WHEEL
std::vector< Rotator > rotators
void ProcessMeshWheels(Document::Module *module)
void ProcessBeams(Document::Module *)
static const BitMask_t OPTION_L_LOG
float progress_factor_spring_out
Progression factor springout, 0 = disabled, 1...x as multipliers, example:maximum springrate == sprin...
float expansion_trigger_limit
@ A_INV_TRIGGER_BLOCKER
Blocker that enable/disable other triggers, reversed activation method (inverted Blocker style,...
float long_bound
Maximum extension limit, in percentage ( 1.00 = 100% )
void ProcessLockgroups(Document::Module *module)
static const BitMask64_t SOURCE_SHIFT_LEFT_RIGHT
void ProcessSoundsources2(Document::Module *module)
std::vector< float > gear_ratios
float damp_in_fast
Damping value applied when shock is commpressing faster than split in velocity.
std::vector< Flare2 > flares2
float max_inclination_angle
static const BitMask_t OPTION_s_CMD_NUM_SWITCH
static const BitMask64_t SOURCE_HEADING
DifferentialTypeVec options
Order matters!
std::vector< Shock > shocks
float progress_factor_spring_in
Progression factor for springin. A value of 0 disables this option. 1...x as multipliers,...
std::vector< Camera > cameras
void ProcessScrewprops(Document::Module *module)
int shortbound_trigger_action
static const BitMask_t OPTION_y_INPUT_InvAILERON_RUDDER
static const BitMask64_t SOURCE_PERMANENT
static const BitMask_t OPTION_s_SUPPORT
void ProcessRotators(Document::Module *module)
float visual_beam_diameter
static const char * TypeToStr(ManagedMaterialType type)
@ g_INPUT_ELEVATOR_RUDDER
static const BitMask_t OPTION_b_KEY_BLOCKER
void ProcessSoundsources(Document::Module *module)
void ProcessHooks(Document::Module *module)
@ b_KEY_BLOCKER
Set the CommandKeys that are set in a commandkeyblocker or trigger to blocked on startup,...
static const BitMask_t OPTION_i_INVISIBLE
static const BitMask64_t SOURCE_ALTIMETER_1K
std::vector< Engine > engine
void ExportDocComment(Document::Module *module, RigDef::Keyword keyword, ptrdiff_t vectorpos)
void ProcessWheels(Document::Module *module)
std::shared_ptr< BeamDefaults > defaults
std::vector< Exhaust > exhausts
void UpdatePresets(BeamDefaults *beam, NodeDefaults *node, DefaultMinimass *minimass)
float parking_brake_force
void ProcessCommand2(Command2 &def)
std::vector< RailGroup > railgroups
float option_min_range_meters
static const BitMask_t OPTION_h_UNLOCKS_HOOK_GROUP
static const BitMask_t OPTION_D_CONTACT_BUOYANT
static const BitMask_t OPTION_t_CONTINUOUS
static const BitMask64_t SOURCE_ACCEL
std::vector< Texcoord > texcoords
std::vector< Cab > cab_triangles
float precompression
Changes compression or extension of the suspension when the truck spawns. This can be used to "level"...
std::shared_ptr< BeamDefaults > beam_defaults
void ProcessSubmeshGroundmodel(Document::Module *module)
RigDef::DocumentPtr m_rig_def
std::vector< Prop > props
void ProcessFlexbodies(Document::Module *module)
void ProcessAirbrakes(Document::Module *module)
void ProcessShocks2(Document::Module *)
void ProcessTies(Document::Module *module)
static const BitMask_t OPTION_s_BUOYANT_NO_DRAG
static const BitMask_t OPTION_s_DISABLE_ON_HIGH_SPEED
std::vector< Fileinfo > fileinfo
static const BitMask_t OPTION_E_ENGINE_TRIGGER
static const BitMask_t OPTION_y_EXHAUST_DIRECTION
void ProcessExhausts(Document::Module *module)
static const BitMask64_t SOURCE_TURBO
Node::Ref rotating_plate_nodes[4]
std::vector< Node::Ref > node_list
static const BitMask_t OPTION_b_EXTRA_BUOYANCY
void ProcessAxles(Document::Module *module)
std::vector< Shock3 > shocks3
std::vector< Ogre::String > submesh_groundmodel
float visibility_range_meters
static const BitMask_t OPTION_c_NO_GROUND_CONTACT
Ogre::String particle_name
static const BitMask_t CONSTRAINT_ATTACH_NONE
static const BitMask_t OPTION_h_INPUT_InvELEVATOR_RUDDER
void SerializeModule(std::shared_ptr< RigDef::Document::Module > m)
std::vector< FlexBodyWheel > flexbodywheels
std::vector< MaterialFlareBinding > materialflarebindings
static const BitMask_t OPTION_h_HOOK_POINT
float plastic_deform_coef
void ProcessSetSkeletonSettings(Document::Module *module)
std::string m_dataline_indentstr
a node or a beam line for example
static const BitMask64_t SOURCE_BRAKES
void ProcessHelp(Document::Module *module)
std::vector< VideoCamera > videocameras
std::string m_setdefaults_indentstr
'set_beam_defaults' for example
void ProcessBeam(Beam &beam)
float contraction_trigger_limit
std::shared_ptr< NodeDefaults > node_defaults
@ E_ENGINE_TRIGGER
this trigger is used to control an engine
void ProcessDirectiveAddAnimation(RigDef::Animation &anim)
float split_vel_in
Split velocity in (m/s) - threshold for slow / fast damping during compression.
BeamDefaults * m_current_beam_defaults
void ProcessSpeedLimiter(Document::Module *module)
static const float DEFAULT_SPRING
std::vector< AntiLockBrakes > antilockbrakes
static const BitMask_t OPTION_j_INVISIBLE
float short_bound
Maximum contraction limit, in percentage ( 1.00 = 100% )
float progress_factor_damp_in
Progression factor for dampin. 0 = disabled, 1...x as multipliers, example:maximum dampingrate == spr...
static const BitMask_t OPTION_M_ABSOLUTE_METRIC
std::shared_ptr< BeamDefaults > beam_defaults
void ProcessSubmesh(Document::Module *module)
unsigned int forum_account_id
void ProcessTransferCase(Document::Module *module)
static const BitMask_t CONSTRAINT_ATTACH_ALL
std::vector< Flexbody > flexbodies
#define ANIMATOR_ADD_FLAG(DEF_VAR, AND_VAR, BITMASK_CONST, NAME_STR)
RoR::CommandkeyID_t contract_key
static const BitMask_t MODE_ROTATION_X
std::vector< Hydro > hydros
static const float DEFAULT_DAMP
std::vector< Ropable > ropables
@ s_DISABLE_ON_HIGH_SPEED
std::vector< ManagedMaterial > managedmaterials
DashboardSpecial special_prop_dashboard
static const BitMask_t OPTION_r_ROPE
static const BitMask64_t SOURCE_CLUTCH
static const BitMask_t MODE_OFFSET_Y
Ogre::String airfoil_name
static const BitMask_t OPTION_F_10xTOUGHER_BUOYANT
int control_number
Only 'u' type flares.
float damp_in
Damping value applied when the shock is compressing.
std::vector< Node::Ref > nodes
static const BitMask_t OPTION_i_INVISIBLE
void ProcessHydros(Document::Module *)
bool _attachment_rate_set
std::vector< CollisionBox > collisionboxes
void ProcessShock3(Shock3 &def)
void PropAnimFlag(std::stringstream &out, int flags, bool &join, unsigned int mask, const char *name, char joiner='|')
std::shared_ptr< Document > DocumentPtr
static const BitMask_t OPTION_r_BUOYANT_ONLY_DRAG
static const BitMask_t OPTION_e_TERRAIN_EDIT_POINT
std::vector< Node::Range > node_list
static const BitMask_t OPTION_m_METRIC
std::vector< TorqueCurve > torquecurve
std::vector< SpeedLimiter > speedlimiter
@ h_INPUT_InvELEVATOR_RUDDER
std::shared_ptr< NodeDefaults > node_defaults
static const BitMask64_t SOURCE_SHIFT_BACK_FORTH
static const BitMask_t OPTION_H_LOCKS_HOOK_GROUP
static const BitMask_t OPTION_S_INVULNERABLE_BUOYANT
void ProcessTractionControl(Document::Module *module)
static const BitMask_t OPTION_i_INVISIBLE
float damping
The 'resistance to motion' of the shock. The best value is given by this equation: 2 * sqrt(suspended...
std::vector< SoundSource > soundsources
bool _max_attach_dist_set
std::shared_ptr< BeamDefaults > beam_defaults
Node::Ref base_plate_nodes[4]
void ProcessParticles(Document::Module *module)
void ProcessMaterialFlareBindings(Document::Module *module)
float damp_out
Damping value applied when shock extending.
bool option_c_auto_center
unsigned int spin_left_key
void ProcessRailGroups(Document::Module *module)
float damp_in_slow
Damping value applied when shock is commpressing slower than split in velocity.
Ogre::String beam_material_name
void ProcessDescription(Document::Module *module)
Ogre::String material_name
const char * ROOT_MODULE_NAME
void ProcessCruiseControl(Document::Module *module)
static const BitMask64_t SOURCE_TORQUE
void ProcessFixes(Document::Module *module)
void ProcessNode(Node &node)
Ogre::String stop_function
static const BitMask_t OPTION_s_DISABLE_SELF_LOCK
static const BitMask64_t SOURCE_ALTIMETER_100K
std::vector< Particle > particles
std::vector< Beam > beams
Ogre::String material_name
static const BitMask_t OPTION_r_INPUT_RUDDER
static const BitMask_t MODE_NO_FLIP
void ProcessInterAxles(Document::Module *module)
Node::Ref reference_arm_node
std::shared_ptr< DefaultMinimass > default_minimass
Data structures representing 'truck' file format, see https://docs.rigsofrods.org/vehicle-creation/fi...
void ProcessGlobals(Document::Module *module)
std::vector< InterAxle > interaxles
BeaconSpecial special_prop_beacon
float progress_factor_damp_out
Progression factor dampout, 0 = disabled, 1...x as multipliers, example:maximum dampingrate == spring...
static const BitMask_t OPTION_i_INVISIBLE
static const BitMask_t OPTION_b_BUOYANT
static const BitMask_t OPTION_x_INPUT_AILERON_RUDDER
std::vector< MeshWheel > meshwheels
std::vector< Engoption > engoption
Ogre::String sound_script_name
@ v_INPUT_InvAILERON_ELEVATOR
std::vector< TractionControl > tractioncontrol
float damp_out_slow
Damping value applied when shock is commpressing slower than split out velocity.
Ogre::String flare_material_name
void ProcessShocks(Document::Module *)
std::vector< Node::Ref > fixes
std::list< Animation > animations
std::vector< SoundSource2 > soundsources2
static const BitMask_t OPTION_f_NO_SPARKS
static const BitMask64_t SOURCE_SEQUENTIAL_SHIFT
static const BitMask64_t SOURCE_SPEEDO