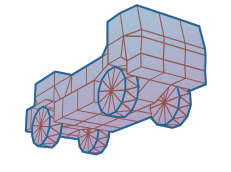 |
RigsofRods
2023.09
Soft-body Physics Simulation
|
Go to the documentation of this file.
33 #define CHECK_SECTION_IN_ALL_MODULES(_CLASS_, _FIELD_, _FUNCTION_) \
35 std::list<std::shared_ptr<RigDef::Document::Module>>::iterator module_itor = m_selected_modules.begin(); \
36 for (; module_itor != m_selected_modules.end(); module_itor++) \
38 std::vector<_CLASS_>::iterator section_itor = module_itor->get()->_FIELD_.begin(); \
39 for (; section_itor != module_itor->get()->_FIELD_.end(); section_itor++) \
41 if (! _FUNCTION_(*section_itor))\
43 section_itor = module_itor->get()->_FIELD_.erase(section_itor); \
44 if (section_itor == module_itor->get()->_FIELD_.end()) \
62 valid &= CheckSectionSubmeshGroundmodel();
64 valid &= CheckGearbox();
88 m_selected_modules.push_back(
file->root_module);
97 case Message::TYPE_FATAL_ERROR:
98 cm_type = RoR::Console::MessageType::CONSOLE_SYSTEM_ERROR;
101 case Message::TYPE_ERROR:
102 case Message::TYPE_WARNING:
103 cm_type = RoR::Console::MessageType::CONSOLE_SYSTEM_WARNING;
107 cm_type = RoR::Console::MessageType::CONSOLE_SYSTEM_NOTICE;
116 Ogre::String *containing_module_name =
nullptr;
118 std::list<std::shared_ptr<RigDef::Document::Module>>::iterator module_itor = m_selected_modules.begin();
119 for (; module_itor != m_selected_modules.end(); module_itor++)
121 if (! module_itor->get()->submesh_groundmodel.empty())
123 if (containing_module_name ==
nullptr)
125 containing_module_name = & module_itor->get()->name;
129 std::stringstream text;
130 text <<
"Duplicate inline-section 'submesh_groundmodel'; found in modules: '"
131 << *containing_module_name <<
"' & '" << module_itor->get()->name <<
"'";
132 AddMessage(Message::TYPE_FATAL_ERROR, text.str());
143 std::map< Ogre::String, std::shared_ptr<RigDef::Document::Module> >::iterator result
144 = m_file->user_modules.find(module_name);
146 if (result != m_file->user_modules.end())
148 m_selected_modules.push_back(result->second);
157 std::shared_ptr<RigDef::Engine> engine;
158 std::list<std::shared_ptr<RigDef::Document::Module>>::iterator module_itor = m_selected_modules.begin();
159 for (; module_itor != m_selected_modules.end(); module_itor++)
161 if (module_itor->get()->engine.size() > 0)
163 if (module_itor->get()->engine[module_itor->get()->engine.size() - 1].gear_ratios.size() > 0)
169 AddMessage(Message::TYPE_FATAL_ERROR,
"Engine must have at least 1 forward gear.");
179 std::list<Ogre::String> bad_fields;
185 bad_fields.push_back(
"spring_in_rate");
189 bad_fields.push_back(
"damping_in_rate");
193 bad_fields.push_back(
"spring_out_rate");
197 bad_fields.push_back(
"damping_out_rate");
201 bad_fields.push_back(
"spring_in_progression_factor");
205 bad_fields.push_back(
"damping_in_progression_factor");
209 bad_fields.push_back(
"spring_out_progression_factor");
213 bad_fields.push_back(
"damping_out_progression_factor");
217 bad_fields.push_back(
"max_contraction");
221 bad_fields.push_back(
"max_extension");
225 bad_fields.push_back(
"precompression");
228 if (bad_fields.size() > 0)
230 std::stringstream msg;
231 msg <<
"Invalid values in section 'shocks2', fields: ";
232 std::list<Ogre::String>::iterator itor = bad_fields.begin();
234 for( ; itor != bad_fields.end(); itor++)
236 msg << (first ?
"" :
", ") << *itor;
240 AddMessage(Message::TYPE_ERROR, msg.str());
248 std::list<Ogre::String> bad_fields;
254 bad_fields.push_back(
"spring_in_rate");
258 bad_fields.push_back(
"damping_in_rate");
262 bad_fields.push_back(
"spring_out_rate");
266 bad_fields.push_back(
"damping_out_rate");
270 bad_fields.push_back(
"damp_in_slow");
274 bad_fields.push_back(
"split_in");
278 bad_fields.push_back(
"damp_in_fast");
282 bad_fields.push_back(
"damp_out_slow");
286 bad_fields.push_back(
"split_out");
290 bad_fields.push_back(
"damp_out_fast");
294 bad_fields.push_back(
"max_contraction");
298 bad_fields.push_back(
"max_extension");
302 bad_fields.push_back(
"precompression");
305 if (bad_fields.size() > 0)
307 std::stringstream msg;
308 msg <<
"Invalid values in section 'shocks3', fields: ";
309 std::list<Ogre::String>::iterator itor = bad_fields.begin();
311 for( ; itor != bad_fields.end(); itor++)
313 msg << (first ?
"" :
", ") << *itor;
317 AddMessage(Message::TYPE_ERROR, msg.str());
326 unsigned int source_check = def.
flags;
337 AddMessage(Message::TYPE_ERROR,
"Animator: No animator source defined");
350 std::stringstream msg;
351 msg <<
"Section 'commands' or 'commands2': Invalid 'extend_key': ";
353 msg <<
"; valid range is <1 - " <<
MAX_COMMANDS <<
"> (plus any negative number is acceptable)";
354 AddMessage(Message::TYPE_ERROR, msg.str());
360 std::stringstream msg;
361 msg <<
"Section 'commands' or 'commands2': Invalid 'contract_key': ";
363 msg <<
"; valid range is <1 - " <<
MAX_COMMANDS <<
"> (plus any negative number is acceptable)";
364 AddMessage(Message::TYPE_ERROR, msg.str());
377 std::stringstream msg;
378 msg <<
"Wrong parameter 'control_number' (" << def.
control_number <<
"), must be in range <-1, 500>";
379 AddMessage(Message::TYPE_ERROR, msg.str());
385 std::stringstream msg;
386 msg <<
"Wrong parameter 'blink_delay_milis' (" << def.
blink_delay_milis <<
"), must be in range <-2, 60000>";
387 AddMessage(Message::TYPE_ERROR, msg.str());
407 if (! trigger_blocker && ! inv_trigger_blocker && ! hook_toggle )
412 std::stringstream msg;
414 msg <<
"; Alloved range is <0 - " <<
MAX_COMMANDS <<
">. ";
415 msg <<
"Trigger deactivated.";
416 AddMessage(Message::TYPE_ERROR, msg.str());
420 else if (! hook_toggle)
425 std::stringstream msg;
427 msg <<
"; Alloved range is <0 - " <<
MAX_COMMANDS <<
">. ";
428 msg <<
"Trigger deactivated.";
429 AddMessage(Message::TYPE_ERROR, msg.str());
434 std::stringstream msg;
436 msg <<
"; Alloved range is <0 - " <<
MAX_COMMANDS <<
">. ";
437 msg <<
"Trigger deactivated.";
438 AddMessage(Message::TYPE_ERROR, msg.str());
448 AddMessage(Message::TYPE_ERROR,
"Wrong command-eventnumber. Engine trigger deactivated.");
463 AddMessage(Message::TYPE_ERROR,
"Wrong texture size definition.");
469 AddMessage(Message::TYPE_ERROR,
"Wrong clipping sizes definition.");
475 AddMessage(Message::TYPE_ERROR,
"Camera Mode setting incorrect.");
481 AddMessage(Message::TYPE_ERROR,
"Camera Role (camera, trace, mirror) setting incorrect.");
static const int MAX_COMMANDS
maximum number of commands per actor
float spring_out
spring value applied when shock extending
bool CheckGearbox()
Checks there's at least 1 forward gear.
static const BitMask_t OPTION_A_INV_TRIGGER_BLOCKER
float damp_out_fast
Damping value applied when shock is commpressing faster than split out velocity.
bool CheckTrigger(RigDef::Trigger &def)
bool AddModule(Ogre::String const &module_name)
Adds a vehicle module to the validated configuration.
unsigned int texture_width
#define CHECK_SECTION_IN_ALL_MODULES(_CLASS_, _FIELD_, _FUNCTION_)
bool CheckCommand(RigDef::Command2 &def)
This is a raw Ogre binding for Imgui No project cmake file
float split_vel_out
Split velocity in (m/s) - threshold for slow / fast damping during extension.
int longbound_trigger_action
float spring_in
Spring value applied when the shock is compressing.
float precompression
Changes compression or extension of the suspension when the truck spawns. This can be used to "level"...
void putMessage(MessageArea area, MessageType type, std::string const &msg, std::string icon="")
float long_bound
Maximum extension limit, in percentage ( 1.00 = 100% )
bool CheckShock3(RigDef::Shock3 &shock3)
static const BitMask_t OPTION_B_TRIGGER_BLOCKER
RoR::CommandkeyID_t extend_key
static const BitMask_t OPTION_INVISIBLE
float damp_in
Damping value applied when the shock is compressing.
float damp_out
damping value applied when shock extending
float spring_out
Spring value applied when shock extending.
RoR::VideoCamRole camera_role
#define BITMASK_SET_0(VAR, FLAGS)
#define BITMASK_IS_0(VAR, FLAGS)
static const BitMask_t OPTION_LONG_LIMIT
unsigned int texture_height
#define BITMASK_IS_1(VAR, FLAGS)
float short_bound
Maximum contraction limit, in percentage ( 1.00 = 100% )
float spring_in
Spring value applied when the shock is compressing.
float precompression
Changes compression or extension of the suspension when the truck spawns. This can be used to "level"...
float progress_factor_spring_out
Progression factor springout, 0 = disabled, 1...x as multipliers, example:maximum springrate == sprin...
void Setup(RigDef::DocumentPtr file)
Prepares the validation.
Central state/object manager and communications hub.
float long_bound
Maximum extension limit, in percentage ( 1.00 = 100% )
float damp_in_fast
Damping value applied when shock is commpressing faster than split in velocity.
AeroAnimator aero_animator
static const BitMask_t OPTION_s_CMD_NUM_SWITCH
float progress_factor_spring_in
Progression factor for springin. A value of 0 disables this option. 1...x as multipliers,...
int shortbound_trigger_action
static const BitMask_t OPTION_h_UNLOCKS_HOOK_GROUP
static const BitMask_t OPTION_VISIBLE
bool CheckSectionSubmeshGroundmodel()
Inline-ection 'submesh_groundmodel', unique across all modules.
static const BitMask_t OPTION_E_ENGINE_TRIGGER
float split_vel_in
Split velocity in (m/s) - threshold for slow / fast damping during compression.
bool CheckAnimator(RigDef::Animator &def)
float short_bound
Maximum contraction limit, in percentage ( 1.00 = 100% )
float progress_factor_damp_in
Progression factor for dampin. 0 = disabled, 1...x as multipliers, example:maximum dampingrate == spr...
bool CheckFlare2(RigDef::Flare2 &def)
RoR::CommandkeyID_t contract_key
int control_number
Only 'u' type flares.
float damp_in
Damping value applied when the shock is compressing.
void AddMessage(Validator::Message::Type type, Ogre::String const &text)
bool CheckVideoCamera(RigDef::VideoCamera &def)
Section 'videocamera'.
@ CONSOLE_MSGTYPE_ACTOR
Parsing/spawn/simulation messages for actors.
std::shared_ptr< Document > DocumentPtr
static const BitMask_t OPTION_SHORT_LIMIT
static const BitMask_t OPTION_H_LOCKS_HOOK_GROUP
float damp_out
Damping value applied when shock extending.
float damp_in_slow
Damping value applied when shock is commpressing slower than split in velocity.
bool CheckShock2(RigDef::Shock2 &shock2)
float progress_factor_damp_out
Progression factor dampout, 0 = disabled, 1...x as multipliers, example:maximum dampingrate == spring...
static const CommandkeyID_t COMMANDKEYID_INVALID
float damp_out_slow
Damping value applied when shock is commpressing slower than split out velocity.