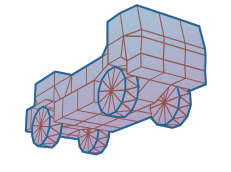 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
27 namespace Hydrax{
namespace Module
55 , mVerticesChoppyBuffer(0)
64 , mVerticesChoppyBuffer(0)
304 Ogre::Vector3 p = Ogre::Vector3(0,0,0);
305 Ogre::Affine3 mWorldMatrix;
379 Ogre::Vector3 vec1, vec2, normal;
387 vec1 = Ogre::Vector3(
392 vec2 = Ogre::Vector3(
397 normal = vec2.crossProduct(vec1);
bool ChoppyWaves
Choppy waves.
void create()
Create our water mesh, geometry, entity, etc...
VertexType
Mesh vertex type enum.
virtual void saveCfg(Ogre::String &Data)
Save config.
SimpleGrid(Hydrax *h, Noise::Noise *n, const MaterialManager::NormalMode &NormalMode)
Constructor.
float MeshStrength
Water strength.
virtual bool createGPUNormalMapResources(GPUNormalMapManager *g)
Create GPUNormalMap resources.
Vertex struct for position data.
const bool & isCreated() const
Is created() called?
const Ogre::Vector3 & getPosition() const
Get water position.
Mesh::VertexType _SG_getVertexTypeFromNormalMode(const MaterialManager::NormalMode &NormalMode)
Mesh * getMesh()
Get Hydrax::Mesh.
bool updateGeometry(const int &numVer, void *verArray)
Update geomtry.
Mesh::POS_NORM_VERTEX * mVerticesChoppyBuffer
Use it to store vertex positions when choppy displacement is enabled.
Struct wich contains an especific width and height value.
void _calculeNormals()
Calcule current normals.
static Size _getSizeValue(Ogre::ConfigFile &CfgFile, const Ogre::String Name)
Get size value.
float getHeigth(const Ogre::Vector2 &Position)
Get the current heigth at a especified world-space point.
void _setStrength(const Ogre::Real &Strength)
Set water strength GPU param.
static bool _getBoolValue(Ogre::ConfigFile &CfgFile, const Ogre::String Name)
Get bool value.
virtual void create()
Create.
void _performChoppyWaves()
Perform choppy waves.
virtual float getValue(const float &x, const float &y)=0
Get the especified x/y noise value.
const Ogre::String & getMaterialName() const
Get material name.
Ogre::String _SG_getNormalModeString(const MaterialManager::NormalMode &NormalMode)
Ogre::Vector2 getGridPosition(const Ogre::Vector2 &Position)
Get the [0,1] range x/y grid position from a 2D world space x/z point.
void setMaterialName(const Ogre::String &MaterialName)
Set mesh material.
GPUNormalMapManager * getGPUNormalMapManager()
Get Hydrax::GPUNormalMapManager.
const Ogre::String & getName() const
Get noise name.
bool loadCfg(Ogre::ConfigFile &CfgFile)
Load config.
void setOptions(const Options &Options)
Set options.
Base noise class, Override it for create different ways of create water noise.
const Mesh::Options & getMeshOptions() const
Get the mesh options for this module.
NormalMode
Normal generation mode.
Struct wich contains Hydrax simple grid module options.
void remove()
Remove all resources.
Base module class, Override it for create different ways of create water noise.
int Complexity
Projected grid complexity (N*N)
Hydrax * mHydrax
Our Hydrax pointer.
Class wich contains all funtions/variables related to Hydrax water mesh.
virtual void update(const Ogre::Real &timeSinceLastFrame)
Call it each frame.
void saveCfg(Ogre::String &Data)
Save config.
static Ogre::String _getCfgString(const Ogre::String &Name, const int &Value)
virtual bool loadCfg(Ogre::ConfigFile &CfgFile)
Load config.
int MeshComplexity
Mesh complexity.
void update(const Ogre::Real &timeSinceLastFrame)
Call it each frame.
Size MeshSize
Grid size (X/Z) world space.
Options mOptions
Our projected grid options.
MaterialManager::NormalMode mNormalMode
Normal map generation mode.
Vertex struct for position and normals data.
void * mVertices
Vertex pointer (Mesh::POS_NORM_VERTEX or Mesh::POS_VERTEX)
void setOptions(const Options &Options)
Update options.
const MaterialManager::NormalMode & getNormalMode() const
Get the normal generation mode.
static int _getIntValue(Ogre::ConfigFile &CfgFile, const Ogre::String Name)
Get int value.
const bool & _isCurrentFrameUnderwater() const
Is current frame underwater?
const Ogre::String & getName() const
Get module name.
Noise::Noise * mNoise
Noise generator pointer.
float ChoppyStrength
Choppy waves strength.
virtual void remove()
Remove.
static Ogre::Real _getFloatValue(Ogre::ConfigFile &CfgFile, const Ogre::String Name)
Get float value.
Ogre::Entity * getEntity()
Get entity.
Mesh::Options mMeshOptions
Module mesh options.