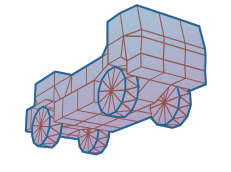 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
25 #ifndef _Hydrax_Module_H_
26 #define _Hydrax_Module_H_
41 namespace Hydrax{
namespace Module
55 Module(
const Ogre::String &Name,
84 virtual void update(
const Ogre::Real &timeSinceLastFrame);
89 virtual void saveCfg(Ogre::String &Data);
94 virtual bool loadCfg(Ogre::ConfigFile &CfgFile);
99 inline const Ogre::String&
getName()
const
150 virtual float getHeigth(
const Ogre::Vector2 &Position);
virtual void saveCfg(Ogre::String &Data)
Save config.
const bool & isCreated() const
Is created() called?
virtual const bool _createGeometry(Mesh *mMesh) const
Create geometry in module(If special geometry is needed)
virtual ~Module()
Destructor.
Base Hydrax mesh options.
Class to manager GPU normal maps.
virtual void create()
Create.
Noise::Noise * getNoise()
Get the Hydrax::Noise module pointer.
void setNoise(Noise::Noise *Noise, GPUNormalMapManager *g=0, const bool &DeleteOldNoise=true)
Set noise.
Base noise class, Override it for create different ways of create water noise.
const Mesh::Options & getMeshOptions() const
Get the mesh options for this module.
virtual float getHeigth(const Ogre::Vector2 &Position)
Get the current heigth at a especified world-space point.
NormalMode
Normal generation mode.
Central state/object manager and communications hub.
Base module class, Override it for create different ways of create water noise.
bool mCreated
Is create() called?
Class wich contains all funtions/variables related to Hydrax water mesh.
virtual void update(const Ogre::Real &timeSinceLastFrame)
Call it each frame.
virtual bool loadCfg(Ogre::ConfigFile &CfgFile)
Load config.
MaterialManager::NormalMode mNormalMode
Normal map generation mode.
Ogre::String mName
Module name.
const MaterialManager::NormalMode & getNormalMode() const
Get the normal generation mode.
const Ogre::String & getName() const
Get module name.
Noise::Noise * mNoise
Noise generator pointer.
virtual void remove()
Remove.
Mesh::Options mMeshOptions
Module mesh options.
Module(const Ogre::String &Name, Noise::Noise *n, const Mesh::Options &MeshOptions, const MaterialManager::NormalMode &NormalMode)
Constructor.