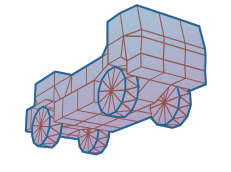 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
25 #ifndef _Hydrax_MaterialManager_H_
26 #define _Hydrax_MaterialManager_H_
191 const Ogre::String GpuProgramNames[2],
193 const Ogre::String EntryPoints[2],
194 const Ogre::String Data[2]);
206 const Ogre::String& EntryPoint,
207 const Ogre::String& Data);
223 return mMaterials[
static_cast<int>(Material)];
267 void addDepthTechnique(Ogre::Technique *Technique,
const bool& AutoUpdate =
true);
280 void addDepthTextureTechnique(Ogre::Technique *Technique,
const Ogre::String& TextureName,
const Ogre::String& AlphaChannel =
"w",
const bool& AutoUpdate =
true);
354 bool _createSimpleColorMaterial(
const Ogre::ColourValue& MaterialColor,
const MaterialType& MT,
const Ogre::String& MaterialName,
const bool& DepthCheck =
true,
const bool& DepthWrite =
true);
void removeCompositor()
Remove compositor.
Options mOptions
Actual material options.
Ogre::MaterialPtr mMaterials[6]
Hydrax materials vector.
Ogre::CompositorPtr mCompositors[1]
Hydrax compositors vector.
Underwater compositor listener.
Ogre::MaterialPtr & getMaterial(const MaterialType &Material)
Get material.
bool _createDepthMaterial(const HydraxComponent &Components, const Options &Options)
Create depth material.
bool _createUnderwaterCompositor(const HydraxComponent &Components, const Options &Options)
Create underwater compositor.
MaterialType
Material type enum.
bool mCompositorsNeedToBeReloaded[1]
Hydrax compositors boolean: Need to be reloaded?
bool _createDepthTextureGPUPrograms(const HydraxComponent &Components, const Options &Options, const Ogre::String &AlphaChannel)
Create depth texture gpu programs.
Material/Shader manager class.
Hydrax * mHydrax
Hydrax main pointer.
std::vector< Ogre::Technique * > & getDepthTechniques()
Get external depth techniques.
bool createMaterials(const HydraxComponent &Components, const Options &Options)
Create materials.
bool _createUnderwaterMaterial(const HydraxComponent &Components, const Options &Options)
Create underwater material.
void notifyMaterialRender(Ogre::uint32 pass_id, Ogre::MaterialPtr &mat)
On material render.
bool mCreated
Is createMaterials() already called?
bool fillGpuProgramsToPass(Ogre::Pass *Pass, const Ogre::String GpuProgramNames[2], const ShaderMode &SM, const Ogre::String EntryPoints[2], const Ogre::String Data[2])
Fill GPU vertex and fragment program to a pass.
void setCompositorEnable(const CompositorType &Compositor, const bool &Enable)
Set a compositor enable/disable.
void addDepthTextureTechnique(Ogre::Technique *Technique, const Ogre::String &TextureName, const Ogre::String &AlphaChannel="w", const bool &AutoUpdate=true)
Add depth texture technique to an especified material.
MaterialManager * mMaterialManager
Material manager parent pointer.
void removeMaterials()
Remove materials.
const Options & getLastOptions() const
Get the last MaterialManager::Options used in a material generation.
NormalMode
Normal generation mode.
UnderwaterCompositorListener mUnderwaterCompositorListener
Underwater compositor listener.
void setGpuProgramParameter(const GpuProgram &GpuP, const MaterialType &MType, const Ogre::String &Name, const Ogre::Real &Value)
Set gpu program Ogre::Real parameter.
const bool & isCreated() const
Is createMaterials() already called?
void reload(const MaterialType &Material)
Reload material.
void addDepthTechnique(Ogre::Technique *Technique, const bool &AutoUpdate=true)
Add depth technique to an especified material.
bool _createSimpleColorMaterial(const Ogre::ColourValue &MaterialColor, const MaterialType &MT, const Ogre::String &MaterialName, const bool &DepthCheck=true, const bool &DepthWrite=true)
HydraxComponent mComponents
Actual material components.
bool createGpuProgram(const Ogre::String &Name, const ShaderMode &SM, const GpuProgram &GPUP, const Ogre::String &EntryPoint, const Ogre::String &Data)
Create GPU program.
HydraxComponent
Hydrax flags to select components wich we want to use.
@ MAT_UNDERWATER_COMPOSITOR
NormalMode NM
Normal map generation mode.
Options(const ShaderMode &_SM, const NormalMode &_NM)
Constructor.
~MaterialManager()
Destructor.
bool _isComponent(const HydraxComponent &List, const HydraxComponent &ToCheck) const
Is component in the given list?
ShaderMode SM
Shader mode.
std::vector< Ogre::Technique * > mDepthTechniques
Technique vector for addDepthTechnique(...)
Options()
Default constructor.
GpuProgram
Gpu program enum.
Ogre::CompositorPtr & getCompositor(const CompositorType &Compositor)
Get compositor.
bool _createWaterMaterial(const HydraxComponent &Components, const Options &Options)
Create water material.
void notifyMaterialSetup(Ogre::uint32 pass_id, Ogre::MaterialPtr &mat)
On material setup.
CompositorType
Compositor type enum.
bool mCompositorsEnable[1]
Hydrax compostor enable vector.
MaterialManager(Hydrax *h)
Constructor.
const bool & isCompositorEnable(const CompositorType &Compositor) const
Is the compositor enable?