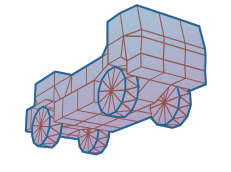 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
35 , mMeshOptions(MeshOptions)
36 , mNormalMode(NormalMode)
94 void Module::update(
const Ogre::Real &timeSinceLastFrame)
99 void Module::saveCfg(Ogre::String &Data)
101 Data +=
"#Module options\n";
102 Data +=
"Module="+
mName+
"\n\n";
105 bool Module::loadCfg(Ogre::ConfigFile &CfgFile)
107 if (CfgFile.getSetting(
"Module") ==
mName)
113 HydraxLOG(
"Error (Module::loadCfg):\t" +
mName +
" options entry can not be found.");
117 float Module::getHeigth(
const Ogre::Vector2 &Position)
virtual void remove()
Remove.
virtual bool createGPUNormalMapResources(GPUNormalMapManager *g)
Create GPUNormalMap resources.
virtual void create()
Create.
virtual void removeGPUNormalMapResources(GPUNormalMapManager *g)
Remove GPUNormalMap resources.
Base Hydrax mesh options.
Class to manager GPU normal maps.
const Ogre::String & getName() const
Get noise name.
Base noise class, Override it for create different ways of create water noise.
NormalMode
Normal generation mode.
Base module class, Override it for create different ways of create water noise.
bool mCreated
Is create() called?
virtual void update(const Ogre::Real &timeSinceLastFrame)=0
Call it each frame.
Ogre::String mName
Module name.
const MaterialManager::NormalMode & getNormalMode() const
Get the normal generation mode.
const bool & isCreated() const
Is created() called?
Noise::Noise * mNoise
Noise generator pointer.