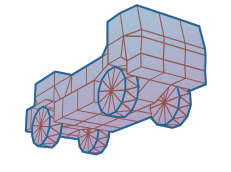 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
25 #ifndef _Hydrax_Mesh_H_
26 #define _Hydrax_Mesh_H_
124 Options(
const int &meshComplexity,
const Size &meshSize,
const float &meshStrength,
const VertexType &meshVertexType)
void create()
Create our water mesh, geometry, entity, etc...
const Options & getOptions() const
Get options.
Options mOptions
Mesh options.
VertexType
Mesh vertex type enum.
Ogre::SubMesh * mSubMesh
Ogre::Submesh pointer.
float MeshStrength
Water strength.
Vertex struct for position data.
Ogre::Entity * mEntity
Ogre::Entity pointer.
Ogre::String mMaterialName
Material name.
VertexType MeshVertexType
Vertex type.
bool updateGeometry(const int &numVer, void *verArray)
Update geomtry.
Vertex struct for position and uv data.
Struct wich contains an especific width and height value.
Mesh(Hydrax *h)
Constructor.
int mNumFaces
Number of faces.
Base Hydrax mesh options.
Ogre::HardwareIndexBufferSharedPtr & getHardwareIndexBuffer()
Get hardware index buffer reference.
Ogre::SubMesh * getSubMesh()
Get sub mesh.
const Ogre::String & getMaterialName() const
Get material name.
Ogre::MeshPtr mMesh
Ogre::MeshPtr.
Options(const int &meshComplexity, const Size &meshSize, const VertexType &meshVertexType)
Constructor.
Ogre::SceneNode * mSceneNode
Ogre::SceneNode pointer.
int mNumVertices
Number of vertices.
Options(const int &meshComplexity, const Size &meshSize, const float &meshStrength, const VertexType &meshVertexType)
Constructor.
Ogre::Vector2 getGridPosition(const Ogre::Vector2 &Position)
Get the [0,1] range x/y grid position from a 2D world space x/z point.
void setMaterialName(const Ogre::String &MaterialName)
Set mesh material.
const VertexType & getVertexType() const
Get vertex type return Mesh vertex type.
Ogre::SceneNode * getSceneNode()
Get the Ogre::SceneNode pointer where Hydrax mesh is attached.
const int & getNumFaces() const
Get number of faces.
const Ogre::Vector3 getObjectSpacePosition(const Ogre::Vector3 &WorldSpacePosition) const
Get the object-space position from world-space position.
void _createGeometry()
Create mesh geometry.
void remove()
Remove all resources.
Hydrax * mHydrax
Hydrax pointer.
Ogre::HardwareVertexBufferSharedPtr & getHardwareVertexBuffer()
Get hardware vertex buffer reference.
Class wich contains all funtions/variables related to Hydrax water mesh.
int MeshComplexity
Mesh complexity.
Size MeshSize
Grid size (X/Z) world space.
const int & getNumVertices() const
Get number of vertices.
bool mCreated
Is _createGeometry() called?
const bool & isCreated() const
Is _createGeometry() called?
Ogre::HardwareVertexBufferSharedPtr mVertexBuffer
Vertex buffer.
Vertex struct for position and normals data.
bool isPointInGrid(const Ogre::Vector2 &Position)
Get if a Position point is inside of the grid.
const Ogre::Vector3 getWorldSpacePosition(const Ogre::Vector3 &ObjectSpacePosition) const
Get the world-space position from object-space position.
Ogre::MeshPtr getMesh()
Get mesh.
void setOptions(const Options &Options)
Update options.
Vertex struct for position, normals and uv data.
Ogre::Entity * getEntity()
Get entity.
Ogre::HardwareIndexBufferSharedPtr mIndexBuffer
Index buffer.
const Size & getSize() const
Get mesh size.