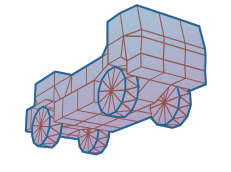 |
RigsofRods
2023.09
Soft-body Physics Simulation
|
Go to the documentation of this file.
25 #ifndef _Hydrax_Modules_SimpleGrid_H_
26 #define _Hydrax_Modules_SimpleGrid_H_
40 namespace Hydrax{
namespace Module
81 const Size &_MeshSize)
100 const Size &_MeshSize,
101 const float &_Strength,
103 const bool &_ChoppyWaves,
104 const float &_ChoppyStrength)
145 void update(
const Ogre::Real &timeSinceLastFrame);
155 void saveCfg(Ogre::String &Data);
160 bool loadCfg(Ogre::ConfigFile &CfgFile);
166 float getHeigth(
const Ogre::Vector2 &Position);
bool ChoppyWaves
Choppy waves.
SimpleGrid(Hydrax *h, Noise::Noise *n, const MaterialManager::NormalMode &NormalMode)
Constructor.
Options(const int &_Complexity, const Size &_MeshSize)
Constructor.
Mesh::POS_NORM_VERTEX * mVerticesChoppyBuffer
Use it to store vertex positions when choppy displacement is enabled.
Struct wich contains an especific width and height value.
void _calculeNormals()
Calcule current normals.
float getHeigth(const Ogre::Vector2 &Position)
Get the current heigth at a especified world-space point.
Options(const int &_Complexity, const Size &_MeshSize, const float &_Strength, const bool &_Smooth, const bool &_ChoppyWaves, const float &_ChoppyStrength)
Constructor.
void _performChoppyWaves()
Perform choppy waves.
Options()
Default constructor.
const Options & getOptions() const
Get current options.
bool loadCfg(Ogre::ConfigFile &CfgFile)
Load config.
void setOptions(const Options &Options)
Set options.
Base noise class, Override it for create different ways of create water noise.
Hydrax simple grid module.
NormalMode
Normal generation mode.
Struct wich contains Hydrax simple grid module options.
Base module class, Override it for create different ways of create water noise.
int Complexity
Projected grid complexity (N*N)
Hydrax * mHydrax
Our Hydrax pointer.
void saveCfg(Ogre::String &Data)
Save config.
void update(const Ogre::Real &timeSinceLastFrame)
Call it each frame.
Options mOptions
Our projected grid options.
Vertex struct for position and normals data.
void * mVertices
Vertex pointer (Mesh::POS_NORM_VERTEX or Mesh::POS_VERTEX)
float ChoppyStrength
Choppy waves strength.