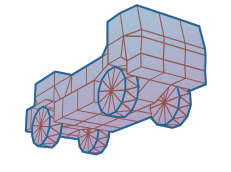 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
39 HydraxWater::HydraxWater(
float water_height, Ogre::String conf_file):
42 , waterHeight(water_height)
43 , waveHeight(water_height)
44 , CurrentConfigFile(conf_file)
67 Ogre::Plane(Ogre::Vector3::UNIT_Y, 0),
78 if (Root::getSingleton().getRenderSystem()->getName() ==
"Direct3D9 Rendering Subsystem" || Root::getSingleton().getRenderSystem()->getName() ==
"Direct3D11 Rendering Subsystem")
105 sunPosition -= sky->GetCaelumSys()->getSun()->getLightDirection() * 80000;
107 mHydrax->
setSunColor(Ogre::Vector3(sky->GetCaelumSys()->getSun()->getBodyColour().r, sky->GetCaelumSys()->getSun()->getBodyColour().g, sky->GetCaelumSys()->getSun()->getBodyColour().b));
141 return Vector3(0, 0, 0);
143 return Vector3(0, 0, 0);
Game state manager and message-queue provider.
void setSunColor(const Ogre::Vector3 &SunColor)
Set sun color.
Ogre::Vector3 CalcWavesVelocity(Ogre::Vector3 pos) override
CameraManager * GetCameraManager()
void update(const Ogre::Real &timeSinceLastFrame)
Call every frame.
void WaterSetSunPosition(Ogre::Vector3) override
AppContext * GetAppContext()
void setSunPosition(const Ogre::Vector3 &SunPosition)
Set sun position.
SkyManager * getSkyManager()
void SetWaterVisible(bool value) override
System integration layer; inspired by OgreBites::ApplicationContext.
Ogre::SceneNode * GetCameraNode()
void create()
Create all resources according with current Hydrax components and add Hydrax to the scene.
bool IsUnderWater(Ogre::Vector3 pos) override
Hydrax::Noise::Perlin * waternoise
void SetStaticWaterHeight(float value) override
Ogre::Camera * GetCamera()
float GetStaticWaterHeight() override
Returns static water level configured in 'terrn2'.
void setModule(Module::Module *Module, const bool &DeleteOldModule=true)
Set Hydrax module.
void UpdateWater() override
Base module class, Override it for create different ways of create water noise.
GameContext * GetGameContext()
Hydrax projected grid module.
const bool loadCfg(const Ogre::String &File) const
Load config from file.
void remove()
Remove hydrax, you can call this method to remove Hydrax from the scene or release (secondary) Hydrax...
Ogre::String CurrentConfigFile
void setPosition(const Ogre::Vector3 &Position)
Set water position.
Struct wich contains Hydrax projected grid module options.
void setVisible(const bool &Visible)
Show/Hide hydrax water.
float CalcWavesHeight(Ogre::Vector3 pos) override
Hydrax::Module::ProjectedGrid * mModule
void setShaderMode(const MaterialManager::ShaderMode &ShaderMode)
Set shader mode.
Perlin noise module class.
void FrameStepWater(float dt) override
float getHeigth(const Ogre::Vector2 &Position)
Get the current heigth at a especified world-space point.
const TerrainPtr & GetTerrain()