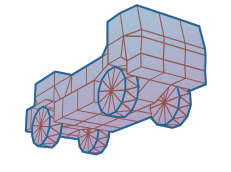 |
RigsofRods
Soft-body Physics Simulation
|
Ogre::Vector3 CalcWavesVelocity(Ogre::Vector3 pos) override
void WaterSetSunPosition(Ogre::Vector3) override
< TODO: Mixed gfx+physics (waves) - must be separated ~ only_a_ptr, 02/2018
void SetWaterVisible(bool value) override
Hydrax::Hydrax * GetHydrax()
HydraxWater(float waterHeight, Ogre::String configFile="HydraxDefault.hdx")
bool IsUnderWater(Ogre::Vector3 pos) override
Hydrax::Noise::Perlin * waternoise
void SetStaticWaterHeight(float value) override
float GetStaticWaterHeight() override
Returns static water level configured in 'terrn2'.
void UpdateWater() override
Hydrax projected grid module.
Ogre::String CurrentConfigFile
float CalcWavesHeight(Ogre::Vector3 pos) override
Hydrax::Module::ProjectedGrid * mModule
Perlin noise module class.
void FrameStepWater(float dt) override