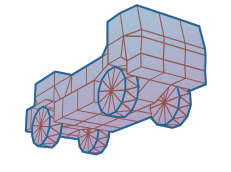 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
130 const vector3& translate = vector3(0.f, 0.f, 0.f),
160 void setFixedYawAxis(
bool useFixed,
const vector3& fixedAxis = vector3(0.f, 1.f, 0.f) );
164 void yaw(
const Radian& angle, TransformSpace relativeTo = TS_LOCAL);
175 void setDirection(
const vector3& vec, TransformSpace relativeTo = TS_LOCAL,
176 const vector3& localDirectionVector = vector3(0.f, 0.f, -1.f));
184 void lookAt(
const vector3& targetPoint, TransformSpace relativeTo,
185 const vector3& localDirectionVector = vector3(0.f, 0.f, -1.f));
206 const vector3& localDirectionVector = vector3(0.f, 0.f, -1.f),
207 const vector3& offset = vector3(0.f, 0.f, 0.f));
229 void setVisible(
bool visible,
bool cascade =
true);
MovableObjectArray getAttachedObjects()
The MovableObjects attached to this node.
void setVisible(bool visible, bool cascade=true)
Makes all objects attached to this node become visible / invisible.
void setDebugDisplayEnabled(bool enabled, bool cascade=true)
Tells all objects attached to this node whether to display their debug information or not.
void removeAndDestroyAllChildren(void)
Removes and destroys all children of this node.
void setDirection(const vector3 &vec, TransformSpace relativeTo=TS_LOCAL, const vector3 &localDirectionVector=vector3(0.f, 0.f, -1.f))
Sets the node's direction vector ie it's local -z.
SceneManager getCreator(void) const
Gets the creator of this scene node.
const vector3 & getAutoTrackLocalDirection(void)
Get the auto tracking local direction for this node, if it is auto tracking.
const vector3 & getAutoTrackOffset(void)
Get the auto tracking offset for this node, if the node is auto tracking.
void attachObject(MovableObject@ obj)
Adds an instance of a scene object to this node.
void yaw(const Radian &angle, TransformSpace relativeTo=TS_LOCAL)
Rotate the node around the Y-axis.
void removeAndDestroyChild(const string &name)
This method removes and destroys the named child and all of its children.
SceneNode getParentSceneNode(void)
Gets the parent of this SceneNode.
void showBoundingBox(bool bShow)
Allows the showing of the node's bounding box.
void hideBoundingBox(bool bHide)
Allows the overriding of the node's bounding box over the SceneManager's bounding box setting.
CReadonlyScriptArrayView< Ogre::MovableObject * > MovableObjectArray
void lookAt(const vector3 &targetPoint, TransformSpace relativeTo, const vector3 &localDirectionVector=vector3(0.f, 0.f, -1.f))
Points the local -Z direction of this node at a point in space.
void setFixedYawAxis(bool useFixed, const vector3 &fixedAxis=vector3(0.f, 1.f, 0.f))
Tells the node whether to yaw around it's own local Y axis or a fixed axis of choice.
Class representing a node in the scene graph.
SceneNode getAutoTrackTarget(void)
Get the auto tracking target for this node, if any.
bool getShowBoundingBox() const
This allows scene managers to determine if the node's bounding box should be added to the rendering q...
void detachAllObjects(void)
Detaches all objects attached to this node.
OGRE-AngelScript bindings; Actually named Ogre in the scripts, just changed for docs to separate thin...
MovableObject detachObject(uint16 index)
Detaches the indexed object from this scene node.
MovableObject getAttachedObject(const string &name)
Retrieves a pointer to an attached object.
bool isInSceneGraph(void) const
Determines whether this node is in the scene graph, i.e.
SceneNode createChildSceneNode(const vector3 &translate=vector3(0.f, 0.f, 0.f), const Quaternion &rotate=quaternion())
Creates an unnamed new SceneNode as a child of this node.
Abstract class defining a movable object in a scene.
Encapsulates everything renderable by OGRE - use game.getSceneManager() to get it.
void setAutoTracking(bool enabled, SceneNode@ const target=0, const vector3 &localDirectionVector=vector3(0.f, 0.f, -1.f), const vector3 &offset=vector3(0.f, 0.f, 0.f))
Enables / disables automatic tracking of another SceneNode.
void flipVisibility(bool cascade=true)
Inverts the visibility of all objects attached to this node.