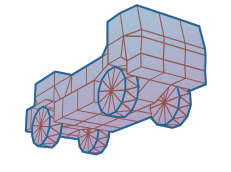 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
41 const int flags = ImGuiWindowFlags_NoCollapse;
42 ImGui::SetNextWindowSize(ImVec2(600.f, 675.f), ImGuiCond_FirstUseEver);
43 bool keep_open =
true;
44 ImGui::Begin(
_LC(
"NodeBeamUtils",
"Node/Beam Utils"), &keep_open, flags);
46 ImGui::PushItemWidth(500.f);
49 if (ImGui::SliderFloat(
_LC(
"NodeBeamUtils",
"Total mass"), &cur_mass, ref_mass * 0.5f, ref_mass * 2.0f,
"%.2f kg"))
57 if (ImGui::SliderFloat(
"Spring##Beams", &actor->
ar_nb_beams_scale.first, 0.1f, 10.0f,
"%.5f"))
61 if (ImGui::SliderFloat(
"Damping##Beams", &actor->
ar_nb_beams_scale.second, 0.1f, 10.0f,
"%.5f"))
67 if (ImGui::SliderFloat(
"Spring##Shocks", &actor->
ar_nb_shocks_scale.first, 0.1f, 10.0f,
"%.5f"))
71 if (ImGui::SliderFloat(
"Damping##Shocks", &actor->
ar_nb_shocks_scale.second, 0.1f, 10.0f,
"%.5f"))
77 if (ImGui::SliderFloat(
"Spring##Wheels", &actor->
ar_nb_wheels_scale.first, 0.1f, 10.0f,
"%.5f"))
81 if (ImGui::SliderFloat(
"Damping##Wheels", &actor->
ar_nb_wheels_scale.second, 0.1f, 10.0f,
"%.5f"))
87 if (ImGui::Button(
_LC(
"NodeBeamUtils",
"Reset to default settings"), ImVec2(280.f, 25.f)))
96 if (ImGui::Button(
_LC(
"NodeBeamUtils",
"Update initial node positions"), ImVec2(280.f, 25.f)))
100 ImGui::PopItemWidth();
102 ImGui::PushItemWidth(235.f);
107 ImGui::PopItemWidth();
108 ImGui::PushItemWidth(138.f);
110 ImGui::TextColored(
GRAY_HINT_TEXT,
"%s",
_LC(
"NodeBeamUtils",
"Beams (spring & damping search interval):"));
118 ImGui::TextColored(
GRAY_HINT_TEXT,
"%s",
_LC(
"NodeBeamUtils",
"Shocks (spring & damping search interval):"));
126 ImGui::TextColored(
GRAY_HINT_TEXT,
"%s",
_LC(
"NodeBeamUtils",
"Wheels (spring & damping search interval):"));
134 ImGui::PopItemWidth();
138 ImVec2(280.f, 25.f)))
147 if (ImGui::Button(
_LC(
"NodeBeamUtils",
"Reset search"), ImVec2(280.f, 25.f)))
156 ImGui::Columns(2,
_LC(
"NodeBeamUtils",
"Search results"));
157 ImGui::SetColumnOffset(1, 290.f);
158 ImGui::Text(
"%s",
_LC(
"NodeBeamUtils",
"Reference"));
160 ImGui::Text(
"%s",
_LC(
"NodeBeamUtils",
"Optimum"));
178 m_is_hovered = ImGui::IsWindowHovered(ImGuiHoveredFlags_RootAndChildWindows);
Game state manager and message-queue provider.
void searchBeamDefaults()
Searches for more stable beam defaults.
std::vector< float > ar_nb_optimum
Temporary storage of the optimum search result.
std::vector< float > ar_nb_reference
Temporary storage of the reference search result.
GUIManager * GetGuiManager()
const ImVec4 GRAY_HINT_TEXT
std::pair< float, float > ar_nb_shocks_k_interval
Search interval for springiness & damping of shock beams.
std::pair< float, float > ar_nb_shocks_scale
Scales for springiness & damping of shock beams.
int ar_nb_measure_steps
Amount of physics steps to be measured.
std::pair< float, float > ar_nb_wheels_scale
Scales for springiness & damping of wheel / rim beams.
std::pair< float, float > ar_nb_beams_d_interval
Search interval for springiness & damping of regular beams.
std::pair< float, float > ar_nb_shocks_d_interval
Search interval for springiness & damping of shock beams.
std::pair< float, float > ar_nb_beams_k_interval
Search interval for springiness & damping of regular beams.
void SyncReset(bool reset_position)
this one should be called only synchronously (without physics running in background)
Central state/object manager and communications hub.
GameContext * GetGameContext()
float ar_initial_total_mass
float getTotalMass(bool withLocked=true)
std::pair< float, float > ar_nb_wheels_k_interval
Search interval for springiness & damping of wheel / rim beams.
float ar_nb_mass_scale
Global mass scale (affects all nodes the same way)
std::pair< float, float > ar_nb_wheels_d_interval
Search interval for springiness & damping of wheel / rim beams.
std::pair< float, float > ar_nb_beams_scale
Scales for springiness & damping of regular beams.
void SetVisible(bool visible)
int ar_nb_skip_steps
Amount of physics steps to be skipped before measuring.
const ActorPtr & GetPlayerActor()
void updateInitPosition()
void RequestGuiCaptureKeyboard(bool val)
Pass true during frame to prevent input passing to application.
void applyNodeBeamScales()
For GUI::NodeBeamUtils.