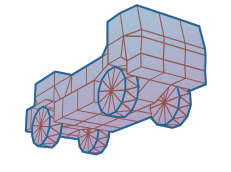 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
34 #define _def_PackedNoise true
36 namespace Hydrax{
namespace Noise{
42 , mGPUNormalMapManager(0)
89 return static_cast<int>(
mWaves.size());
105 if( (
id < 0) || (
id >= (
int)
mWaves.size()) ){
106 HydraxLOG(
"Error (Real::eraseWave):\tCan't delete the wave.");
107 HydraxLOG(
"\tIdentifier exceed the waves vector dimensions.");
113 HydraxLOG(
"Error (Real::eraseWave):\tCan't delete the wave.");
114 HydraxLOG(
"\tThe size before deletion matchs with the size after the operation.");
122 if( (
id < 0) || (
id >= (
int)
mWaves.size()) ){
123 HydraxLOG(
"Error (Real::eraseWave):\tCan't delete the wave.");
124 HydraxLOG(
"\tIdentifier exceed the waves vector dimensions.");
166 mTime += timeSinceLastFrame;
168 for(i=(
int)
mWaves.size()-1;i>=0;i--) {
169 mWaves.at(i).update(timeSinceLastFrame);
184 for(i=0;i<(int)
mWaves.size();i++) {
float getValue(const float &x, const float &y)
Get the especified x/y noise value.
GPUNormalMapManager * mGPUNormalMapManager
GPUNormalMapManager pointer.
virtual void remove()
Remove.
bool eraseWave(int id)
Removes a wave from the system.
virtual void create()
Create.
float getValue(const float &x, const float &y)
Get the especified x/y noise value.
int addWave(Ogre::Vector2 dir, float A, float T, float p=0.f)
Adds a wave to the system.
std::vector< PressurePoint > mPressurePoints
Vector of pressure points.
double mTime
Elapsed time.
Struct wich contains an especific width and height value.
virtual void removeGPUNormalMapResources(GPUNormalMapManager *g)
Remove GPUNormalMap resources.
virtual bool loadCfg(Ogre::ConfigFile &CfgFile)
Load config.
bool loadCfg(Ogre::ConfigFile &CfgFile)
Load config.
Class to manager GPU normal maps.
void saveCfg(Ogre::String &Data)
Save config.
bool createGPUNormalMapResources(GPUNormalMapManager *g)
Create GPUNormalMap resources.
static Ogre::Vector3 _getVector3Value(Ogre::ConfigFile &CfgFile, const Ogre::String Name)
Get vector3 value.
void saveCfg(Ogre::String &Data)
Save config.
const Ogre::String & getName() const
Get noise name.
Base noise class, Override it for create different ways of create water noise.
bool modifyWave(int id, Ogre::Vector2 dir, float A, float T, float p=0.f)
Modify a wave from the system.
void update(const Ogre::Real &timeSinceLastFrame)
Call it each frame.
int addPressurePoint(Ogre::Vector2 Orig, float p, float T, float L)
Adds a pressure point to the system.
A wave defined by the direction, amplitude, period, and optionally phase.
const bool & areGPUNormalMapResourcesCreated() const
Are GPU normal map resources created?
Real()
Default constructor.
Perlin * mPerlinNoise
Perlin noise.
Struct wich contains Perlin noise module options.
void update(const Ogre::Real &timeSinceLastFrame)
Call it each frame.
A PressurePoint defined by the origen, pressure pulse, Maximum time of perturbation and wave longitud...
static int _getIntValue(Ogre::ConfigFile &CfgFile, const Ogre::String Name)
Get int value.
const bool & isCreated() const
Is created() called?
void setOptions(const Options &Options)
Set/Update perlin noise options.
static Ogre::Real _getFloatValue(Ogre::ConfigFile &CfgFile, const Ogre::String Name)
Get float value.
Perlin noise module class.
std::vector< Wave > mWaves
Vector of waves.
Wave getWave(int id) const
Returns a wave to the system.