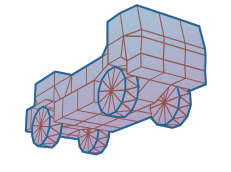 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
24 #ifndef WAVE_H_INCLUDED
25 #define WAVE_H_INCLUDED
53 namespace Hydrax{
namespace Noise
68 Wave(Ogre::Vector2 dir,
float A,
float T,
float p=0.f);
77 void update(
const Ogre::Real &timeSinceLastFrame);
85 float getValue(
const float &
x,
const float &
y);
142 #endif // WAVE_H_INCLUDED
float mK
Dispersion factor.
Ogre::Vector2 getDirection() const
Returns direction of the wave.
float getValue(const float &x, const float &y)
Get the especified x/y noise value.
float getSpeed() const
Returns phase speed of the wave.
float mL
Longitude (calculated)
Ogre::Vector2 mDir
Direction (must be normalised)
void update(const Ogre::Real &timeSinceLastFrame)
Call it each frame.
float getPhase() const
Returns phase of the wave.
Wave(Ogre::Vector2 dir, float A, float T, float p=0.f)
Default constructor.
float mC
Speed (calculated)
A wave defined by the direction, amplitude, period, and optionally phase.
float getAmplitude() const
Returns amplitude of the wave.
float getPeriod() const
Returns period of the wave.
float getLongitude() const
Returns longitude of the wave.
double mTime
Elapsed time.