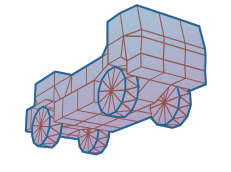 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
24 #ifndef REAL_H_INCLUDED
25 #define REAL_H_INCLUDED
59 namespace Hydrax{
namespace Noise
94 int addWave(Ogre::Vector2 dir,
float A,
float T,
float p=0.f);
132 bool modifyWave(
int id,Ogre::Vector2 dir,
float A,
float T,
float p=0.f);
143 void update(
const Ogre::Real &timeSinceLastFrame);
148 void saveCfg(Ogre::String &Data);
153 bool loadCfg(Ogre::ConfigFile &CfgFile);
161 float getValue(
const float &
x,
const float &
y);
193 #endif // REAL_H_INCLUDED
GPUNormalMapManager * mGPUNormalMapManager
GPUNormalMapManager pointer.
bool eraseWave(int id)
Removes a wave from the system.
float getValue(const float &x, const float &y)
Get the especified x/y noise value.
int addWave(Ogre::Vector2 dir, float A, float T, float p=0.f)
Adds a wave to the system.
std::vector< PressurePoint > mPressurePoints
Vector of pressure points.
double mTime
Elapsed time.
bool loadCfg(Ogre::ConfigFile &CfgFile)
Load config.
Class to manager GPU normal maps.
bool createGPUNormalMapResources(GPUNormalMapManager *g)
Create GPUNormalMap resources.
void saveCfg(Ogre::String &Data)
Save config.
Base noise class, Override it for create different ways of create water noise.
bool modifyWave(int id, Ogre::Vector2 dir, float A, float T, float p=0.f)
Modify a wave from the system.
Perlin * getPerlinNoise() const
Get current Real noise options.
int addPressurePoint(Ogre::Vector2 Orig, float p, float T, float L)
Adds a pressure point to the system.
A wave defined by the direction, amplitude, period, and optionally phase.
This class is a sea elevation module that combines several effects: Waves, defined by direction,...
Real()
Default constructor.
Perlin * mPerlinNoise
Perlin noise.
void update(const Ogre::Real &timeSinceLastFrame)
Call it each frame.
Perlin noise module class.
std::vector< Wave > mWaves
Vector of waves.
Wave getWave(int id) const
Returns a wave to the system.