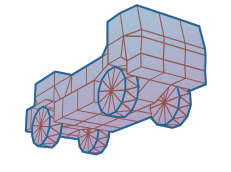 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
37 EngineSim::EngineSim(
float _min_rpm,
float _max_rpm,
float torque, std::vector<float> gears,
float dratio,
ActorPtr actor) :
39 , m_auto_cur_acc(0.0f)
40 , m_auto_mode(AUTOMATIC)
42 , m_braking_torque(-torque / 5.0f)
43 , m_clutch_force(10000.0f)
48 , m_cur_clutch_torque(0.0f)
49 , m_cur_engine_rpm(0.0f)
50 , m_cur_engine_torque(0.0f)
53 , m_cur_wheel_revolutions(0.0f)
54 , m_ref_wheel_revolutions(0.0f)
55 , m_diff_ratio(dratio)
57 , m_engine_torque(torque)
58 , m_gear_ratios(gears)
59 , m_engine_has_air(true)
60 , m_engine_has_turbo(true)
61 , m_hydropump_state(0.0f)
62 , m_engine_idle_rpm(std::min(std::abs(_min_rpm), 800.0f))
63 , m_engine_inertia(10.0f)
64 , m_kickdown_delay_counter(0)
65 , m_max_idle_mixture(0.1f)
66 , m_engine_max_rpm(std::abs(_max_rpm))
67 , m_min_idle_mixture(0.0f)
68 , m_engine_min_rpm(std::abs(_min_rpm))
69 , m_num_gears((int)gears.size() - 2)
70 , m_post_shift_time(0.2f)
71 , m_post_shift_clock(0.0f)
73 , m_engine_is_priming(false)
74 , m_engine_is_running(false)
75 , m_shift_behaviour(0.0f)
80 , m_engine_stall_rpm(300.0f)
84 , m_engine_turbo_mode(OLD)
86 , m_upshift_delay_counter(0)
87 , m_engine_is_electric(false)
88 , m_turbo_inertia_factor(1)
90 , m_max_turbo_rpm(200000.0f)
91 , m_turbo_engine_rpm_operation(0.0f)
94 , m_min_wastegate_psi(20)
95 , m_turbo_has_wastegate(false)
96 , m_turbo_has_bov(false)
97 , m_turbo_flutters(false)
98 , m_turbo_wg_threshold_p(0)
99 , m_turbo_wg_threshold_n(0)
100 , m_turbo_has_antilag(false)
101 , m_antilag_rand_chance(0.9975)
102 , m_antilag_min_rpm(3000)
103 , m_antilag_power_factor(170)
129 void EngineSim::SetTurboOptions(
int type,
float tinertiaFactor,
int nturbos,
float param1,
float param2,
float param3,
float param4,
float param5,
float param6,
float param7,
float param8,
float param9,
float param10,
float param11)
137 LOG(
"Turbo: No more than 4 turbos allowed");
155 float turbo_max_psi = param1;
197 void EngineSim::SetEngineOptions(
float einertia,
char etype,
float eclutch,
float ctime,
float stime,
float pstime,
float irpm,
float srpm,
float maximix,
float minimix,
float ebraking)
238 else if (etype ==
'e')
287 float turbotorque = 0.0f;
288 float turboInertia = 0.000003f;
312 float turbotorque = 0.0f;
313 float turboBOVtorque = 0.0f;
360 turboInertia = turboInertia * 0.7;
362 turboInertia = turboInertia * 1.3;
371 turbotorque += (turbotorque * 2.5);
426 float totaltorque = 0.0f;
587 else if (m_cur_engine_rpm < m_engine_min_rpm && m_engine_min_rpm > declutchRPM)
603 float torqueDiff = std::min(engineTorque, std::abs(reTorque));
604 float clutch = torqueDiff / reTorque;
618 if (hdir != Vector3::ZERO)
650 float avgRPM50 = 0.0f;
651 float avgRPM200 = 0.0f;
652 float avgAcc50 = 0.0f;
653 float avgAcc200 = 0.0f;
654 float avgBrake50 = 0.0f;
655 float avgBrake200 = 0.0f;
657 for (
unsigned int i = 0; i <
m_accs.size(); i++)
671 avgRPM50 /= std::min(
m_rpms.size(), (std::deque<float>::size_type)50);
672 avgAcc50 /= std::min(
m_accs.size(), (std::deque<float>::size_type)50);
673 avgBrake50 /= std::min(
m_brakes.size(), (std::deque<float>::size_type)50);
675 avgRPM200 /=
m_rpms.size();
676 avgAcc200 /=
m_accs.size();
679 if (avgAcc50 > 0.8f || avgAcc200 > 0.8f || avgBrake50 > 0.8f || avgBrake200 > 0.8f)
683 else if (acc < 0.5f && avgAcc50 < 0.5f && avgAcc200 < 0.5f && m_actor->ar_brake < 0.5f && avgBrake50 < 0.5f && avgBrake200 < 0.5)
754 if (newGear < m_cur_gear && m_kickdown_delay_counter > 0)
897 if (autoselect != -1)
962 rpmRatio = std::max(0.0f, rpmRatio);
963 rpmRatio = std::min(rpmRatio, 1.0f);
965 float crankfactor = 5.0f * rpmRatio;
1193 val = std::max(0.0f, val);
1205 float atValue = 0.0f;
1225 float tqValue = 1.0f;
1248 if (crankfactor < 0.9f)
1253 else if (crankfactor < 1.0f)
1256 return 10.0f * (1.0f - crankfactor);
1271 float acclModifier = 0.0f;
1274 acclModifier += 0.25f;
1278 acclModifier += 0.50f;
1280 accl *= acclModifier;
1286 float brakeModifier = 0.0f;
1289 brakeModifier += 0.25f;
1293 brakeModifier += 0.50f;
1295 brake *= brakeModifier;
1334 if (velocity < 1.0f && brake > 0.5f && accl < 0.5f && this->
GetGear() > 0)
1346 else if (velocity > -1.0f && brake < 0.5f && accl > 0.5f && this->
GetGear() < 0)
1437 for (
int i = 1; i < 19; i++)
1449 bool gear_changed =
false;
1451 int curgear = this->
GetGear();
1453 int gearoffset = std::max(0, curgear - curgearrange * 6);
1462 gear_changed =
true;
1468 gear_changed =
true;
1474 gear_changed =
true;
1483 else if (curgear > 0 && curgear < 19)
1488 if (gear_changed || curgear == 0)
1504 for (
int i = 1; i < 19 && !found; i++)
1515 for (
int i = 1; i < 7 && !found; i++)
1519 this->
shiftTo(i + curgearrange * 6);
float ar_avg_wheel_speed
Physics state; avg wheel speed in m/s.
float m_braking_torque
Engine attribute.
float m_tcase_ratio
Engine.
float m_ref_wheel_revolutions
Gears; estimated wheel revolutions based on current vehicle speed along the longi....
void SetEngineRpm(float rpm)
Set current engine RPM.
void PushNetworkState(float engine_rpm, float acc, float clutch, int gear, bool running, bool contact, char auto_mode, char auto_select=-1)
Sets current engine state;.
void setManualClutch(float val)
void SetClutch(float clutch)
void shift(int val)
Changes gear by a relative offset. Plays sounds.
float m_hydropump_state
Engine.
int GetGearRange()
low level gear changing
int m_engine_turbo_mode
Engine attribute.
Ogre::Vector3 GetCameraDir()
void autoShiftSet(int mode)
void ToggleAutoShiftMode()
ActorInstanceID_t ar_instance_id
Static attr; session-unique ID.
float m_turbo_bov_rpm[MAXTURBO]
bool m_engine_has_air
Engine attribute.
float m_min_wastegate_psi
float m_antilag_rand_chance
float m_cur_engine_rpm
Engine.
int m_kickdown_delay_counter
TorqueCurve * m_torque_curve
void TRIGGER_EVENT_ASYNC(scriptEvents type, int arg1, int arg2ex=0, int arg3ex=0, int arg4ex=0, std::string arg5ex="", std::string arg6ex="", std::string arg7ex="", std::string arg8ex="")
Asynchronously (via MSG_SIM_SCRIPT_EVENT_TRIGGERED) invoke script function eventCallbackEx(),...
Ogre::Real ar_brake
Physics state; braking intensity.
int m_cur_gear_range
Gears.
float m_turbo_engine_rpm_operation
void RequestUpdateHudFeatures()
std::deque< float > m_rpms
float m_turbo_wg_threshold_n
System integration layer; inspired by OgreBites::ApplicationContext.
void updateShifts()
Changes gears. Plays sounds.
void putMessage(MessageArea area, MessageType type, std::string const &msg, std::string icon="")
This class loads and processes a torque curve for a vehicle.
void SetAutoMode(RoR::SimGearboxMode mode)
float approx_exp(const float x)
float m_engine_max_rpm
Engine attribute.
void SetWheelSpin(float rpm)
Set current wheel spinning speed.
void autoSetAcc(float val)
void SetAcceleration(float val)
@ SE_TRUCK_ENGINE_DIED
triggered when the vehicle's engine dies (from underrev, water, etc), the argument refers to the acto...
char m_engine_type
Engine attribute {'t' = truck (default), 'c' = car}.
bool m_turbo_has_wastegate
void UpdateEngineSim(float dt, int doUpdate)
float m_engine_idle_rpm
Engine attribute.
@ MANUAL_STICK
Fully manual: stick shift.
std::string ToLocalizedString(SimGearboxMode e)
float m_one_third_rpm_range
void SetTurboOptions(int type, float tinertiaFactor, int nturbos, float param1, float param2, float param3, float param4, float param5, float param6, float param7, float param8, float param9, float param10, float param11)
Sets turbo options.
static const float RAD_PER_SEC_TO_RPM
Convert radian/second to RPM (60/2*PI)
float m_engine_inertia
Engine attribute.
CVar * io_arcade_controls
float m_post_shift_time
Shift attribute.
float m_engine_min_rpm
Engine attribute.
void SetTCaseRatio(float ratio)
Set current transfer case gear (reduction) ratio.
float m_engine_stall_rpm
Engine attribute.
int m_upshift_delay_counter
void UpdateInputEvents(float dt)
float m_cur_turbo_rpm[MAXTURBO]
RoR::SimGearboxMode GetAutoShiftMode()
#define SOUND_START(_ACTOR_, _TRIG_)
bool m_engine_is_electric
Engine attribute.
int m_auto_mode
Transmission mode (.
@ SEMI_AUTO
Manual shift with auto clutch.
float m_max_idle_mixture
Engine attribute.
Ogre::Vector3 getDirection()
average actor velocity, calculated using the actor positions of the last two frames
#define SOUND_STOP(_ACTOR_, _TRIG_)
float GetAccToHoldRPM()
estimate required throttle input to hold the current rpm
float m_min_idle_mixture
Engine attribute.
void SetGear(int v)
low level gear changing
int GetGear()
low level gear changing
bool m_starter
Ignition switch is in START position.
void SetEngineOptions(float einertia, char etype, float eclutch, float ctime, float stime, float pstime, float irpm, float srpm, float maximix, float minimix, float ebraking)
Sets engine options.
@ MANUAL
Fully manual: sequential shift.
#define SOUND_MODULATE(_ACTOR_, _MOD_, _VALUE_)
float m_cur_wheel_revolutions
Gears; measured wheel revolutions.
void StopEngine()
stall engine
bool m_engine_has_turbo
Engine attribute.
float m_turbo_inertia_factor
float m_cur_clutch_torque
#define SOUND_PLAY_ONCE(_ACTOR_, _TRIG_)
Ogre::Real getEngineTorque(Ogre::Real rpm)
Returns the calculated engine torque based on the given RPM, interpolating the torque curve spline.
void StartEngine()
Quick engine start. Plays sounds.
bool m_engine_is_priming
Engine.
InputEngine * GetInputEngine()
int m_cur_gear
Gears; Current gear {-1 = reverse, 0 = neutral, 1...21 = forward}.
float m_diff_ratio
Engine.
std::vector< float > m_gear_ratios
Gears.
void OffStart()
Quick start of vehicle engine.
@ MANUAL_RANGES
Fully manual: stick shift with ranges.
void shiftTo(int val)
Changes gear to given value. Plays sounds.
float m_clutch_force
Clutch attribute.
@ CONSOLE_MSGTYPE_INFO
Generic message.
bool m_contact
Ignition switch is in ON/RUN position.
wheel_t ar_wheels[MAX_WHEELS]
int getNumGearsRanges() const
float m_antilag_power_factor
Ogre::Vector3 getGForces()
float m_engine_addi_torque[MAXTURBO]
std::deque< float > m_accs
void SetHydroPumpWork(float work)
Set current hydro pump work.
float m_clutch_time
Clutch attribute.
void SetGearRange(int v)
low level gear changing
float m_turbo_wg_threshold_p
float m_shift_time
Shift attribute.
float m_cur_engine_torque
Engine.
void SetEnginePriming(bool p)
Set current engine prime.
std::deque< float > m_brakes
bool m_engine_is_running
Engine state.
float m_engine_torque
Engine attribute.