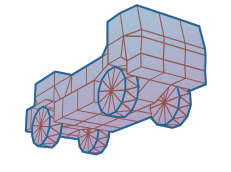 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
25 #ifndef _Hydrax_GodRaysManager_H_
26 #define _Hydrax_GodRaysManager_H_
80 void update(
const Ogre::Real& timeSinceLastFrame);
99 void addDepthTechnique(Ogre::Technique *Technique,
const bool& AutoUpdate =
true);
const Ogre::Real & getRaysSize() const
Get god rays size.
void _updateMaterialsParameters()
Update materials parameters.
void _createDepthRTT()
Create depth RTT.
const Ogre::Real & getSimulationSpeed() const
Get god rays simulation speed.
Ogre::SceneNode * mProjectorSN
Projector scene node.
void setNoiseParameters(Ogre::Vector4 Params)
Set noise params.
void addDepthTechnique(Ogre::Technique *Technique, const bool &AutoUpdate=true)
Add god rays depth technique to an especified material.
const bool & areObjectsIntersectionsEnabled() const
Are rays objects intersections enabled?
std::queue< std::string > mMaterials
std::string to store entity's original materials name
GodRaysManager(Hydrax *h)
Constructor.
MaterialType
God rays material enumeration.
Ogre::Real mNoiseDerivation
Noise parameters (Used in _calculateRayPosition(...))
GodRaysManager::DepthMapListener class.
bool _isComponent(const HydraxComponent &List, const HydraxComponent &ToCheck) const
Is component in the given list?
Ogre::ManualObject * mManualGodRays
Manual object to create god rays.
Ogre::Real mNoisePositionMultiplier
PositionMultiplier value.
Struct wich contains an especific width and height value.
const bool isVisible() const
Is visible?
Hydrax * mHydrax
Hydrax parent pointer.
Ogre::TexturePtr mProjectorRTT
For rays intersection with objects we use a depth map based technique Depth RTT texture.
void setObjectIntersectionsEnabled(const bool &Enable)
Set objects intersections enabled.
int mNumberOfRays
Number of rays.
void _createMaterials(const HydraxComponent &HC)
Create materials that we need(God rays depth too if it's needed)
void _createGodRays()
Create god rays manual object.
Ogre::Camera * mProjectorCamera
Camera used to project rays.
Ogre::Vector2 _calculateRayPosition(const int &RayNumber)
Calculate the current position of a ray.
Ogre::Real mNoiseNormalMultiplier
Normal multiplier.
Ogre::Real mRaysSize
God rays size.
void create(const HydraxComponent &HC)
Create.
void _updateProjector()
Update projector.
Ogre::MaterialPtr mMaterials[2]
God rays materials 0-God rays, 1-Depth.
void _updateRays()
Update god rays.
Underwater god rays manager class God rays.
void SetSimulationSpeed(const Ogre::Real &Speed)
Set god rays simulation speed.
const int & getNumberOfRays() const
Get number of god rays.
const bool & isCreated() const
Has been create() already called?
Ogre::Real mSimulationSpeed
God rays simulation speed.
void update(const Ogre::Real &timeSinceLastFrame)
Call each frame.
Noise::Perlin * getPerlin()
Get perlin noise module.
bool mCreated
Has been create() already called?
void setNumberOfRays(const int &NumberOfRays)
Set the number of god rays.
HydraxComponent
Hydrax flags to select components wich we want to use.
Ogre::SceneNode * getSceneNode()
Get good rays scene node.
Noise::Perlin * mPerlin
Our Perlin noise module.
GodRaysManager * mGodRaysManager
God rays manager pointer.
void postRenderTargetUpdate(const Ogre::RenderTargetEvent &evt)
Funtion that is called after the Rtt will render.
bool mObjectsIntersections
Are god rays objects intersections active?
Perlin noise module class.
const Ogre::Vector4 getNoiseParameters() const
Get noise params.
Ogre::Real mNoiseYNormalMultiplier
Y normal component multiplier.
void setVisible(const bool &Visible)
Set visible.
~GodRaysManager()
Destructor.
void setRaysSize(const Ogre::Real &Size)
Set god rays size.
std::vector< Ogre::Technique * > mDepthTechniques
Technique vector for addDepthTechnique(...)
DepthMapListener mDepthMapListener
Depth RTT listener.
void preRenderTargetUpdate(const Ogre::RenderTargetEvent &evt)
Funtion that is called before the Rtt will render.