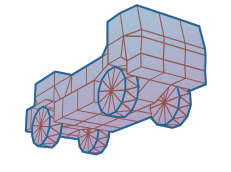 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
61 ss <<
"[RoR|MessageBox] Showing a message box\n<MessageBox title> " << cfg.
mbc_title <<
"\n<MessageBox text> " << cfg.
mbc_text <<
"'";
69 void MessageBoxDialog::Show(
const char* title,
const char* text,
bool allow_close,
const char* button1_text,
const char* button2_text)
103 ImGui::SetNextWindowPosCenter(ImGuiCond_Appearing);
118 if (ImGui::Checkbox(
_LC(
"MessageBox",
"Always ask"), &ask))
std::vector< MessageBoxButton > mbc_buttons
#define ROR_ASSERT(_EXPR)
Game state manager and message-queue provider.
void DrawButton(MessageBoxButton const &button)
Generic UI dialog (not modal). Invocable from scripting. Any number of buttons. Configurable to fire ...
GUIManager * GetGuiManager()
@ SE_GENERIC_MESSAGEBOX_CLICK
triggered when the user clicks on a message box button, the argument refers to the button pressed
bool * m_close_handle
If nullptr, close button is hidden. Otherwise visible.
void TRIGGER_EVENT_ASYNC(scriptEvents type, int arg1, int arg2ex=0, int arg3ex=0, int arg4ex=0, std::string arg5ex="", std::string arg6ex="", std::string arg7ex="", std::string arg8ex="")
Asynchronously (via MSG_SIM_SCRIPT_EVENT_TRIGGERED) invoke script function eventCallbackEx(),...
const char * MsgTypeToString(MsgType type)
void PushMessage(Message m)
Doesn't guarantee order! Use ChainMessage() if order matters.
Central state/object manager and communications hub.
GameContext * GetGameContext()
void Show(MessageBoxConfig const &cfg)
bool * mbc_close_handle
External close handle - not required for mbc_allow_close.
CVar * mbc_always_ask_conf
If set, displays classic checkbox "[x] Always ask".
Unified game event system - all requests and state changes are reported using a message.
float mbc_content_width
Parameter to ImGui::SetContentWidth() - hard limit on content size.
bool mbc_allow_close
Show close handle even if dbc_close_handle isn't set.
void RequestGuiCaptureKeyboard(bool val)
Pass true during frame to prevent input passing to application.