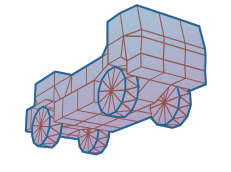 |
RigsofRods
2023.09
Soft-body Physics Simulation
|
Go to the documentation of this file.
33 #include "PagedGeometry.h"
34 #include "TreeLoader2D.h"
35 #include "TreeLoader3D.h"
36 #include "BatchPage.h"
37 #include "GrassLoader.h"
38 #include "ImpostorPage.h"
42 #include <unordered_map>
69 bool LoadTerrainObject(
const Ogre::String& name,
const Ogre::Vector3& pos,
const Ogre::Vector3& rot,
const Ogre::String& instancename,
const Ogre::String& type,
float rendering_distance = 0,
bool enable_collisions =
true,
int scripthandler = -1,
bool uniquifyMaterial =
false);
71 void moveObjectVisuals(
const Ogre::String& instancename,
const Ogre::Vector3& pos);
81 float yawfrom,
float yawto,
82 float scalefrom,
float scaleto,
83 char* ColorMap,
char* DensityMap,
char* treemesh,
char* treeCollmesh,
84 float gridspacing,
float highdens,
85 int minDist,
int maxDist,
int mapsizex,
int mapsizez);
88 float SwaySpeed,
float SwayLength,
float SwayDistribution,
float Density,
89 float minx,
float miny,
float minH,
float maxx,
float maxy,
float maxH,
90 char* grassmat,
char* colorMapFilename,
char* densityMapFilename,
91 int growtechnique,
int techn,
int range,
int mapsizex,
int mapsizez);
112 Ogre::ParticleSystem*
psys =
nullptr;
113 Ogre::SceneNode*
node =
nullptr;
133 std::unordered_map<std::string, std::shared_ptr<RoR::ODefDocument>>
m_odef_cache;
150 std::vector<Forests::PagedGeometry*> m_paged_geometry;
LocalizerVec & GetLocalizers()
void ProcessPredefinedActor(int tobj_cache_id, const std::string &name, const Ogre::Vector3 position, const Ogre::Vector3 rotation, const TObjSpecialObject type)
bool m_has_predefined_actors
void UpdateParticleEffectObjects()
std::vector< TObjDocumentPtr > m_tobj_cache
ProceduralManagerPtr & getProceduralManager()
SurveyMapEntityVec m_map_entities
void LoadTObjFile(Ogre::String filename)
std::vector< TerrainEditorObjectPtr > TerrainEditorObjectPtrVec
Ogre::ParticleSystem * psys
Ogre::SceneNode * getGroupingSceneNode()
LocalizerVec m_localizers
void destroyObject(const Ogre::String &instancename)
bool LoadTerrainObject(const Ogre::String &name, const Ogre::Vector3 &pos, const Ogre::Vector3 &rot, const Ogre::String &instancename, const Ogre::String &type, float rendering_distance=0, bool enable_collisions=true, int scripthandler=-1, bool uniquifyMaterial=false)
bool LoadTerrainScript(const Ogre::String &filename)
std::vector< MeshObject * > m_mesh_objects
void LoadPredefinedActors()
bool HasPredefinedActors()
std::unordered_map< std::string, std::shared_ptr< RoR::ODefDocument > > m_odef_cache
std::vector< SurveyMapEntity > SurveyMapEntityVec
void ProcessGrass(float SwaySpeed, float SwayLength, float SwayDistribution, float Density, float minx, float miny, float minH, float maxx, float maxy, float maxH, char *grassmat, char *colorMapFilename, char *densityMapFilename, int growtechnique, int techn, int range, int mapsizex, int mapsizez)
RoR::ODefDocument * FetchODef(std::string const &odef_name)
Ogre::SceneNode * m_terrn2_grouping_node
For a readable scene graph (via inspector script)
bool GetEditorObjectFlagRotYXZ(TerrainEditorObjectPtr const &object)
void UpdateAnimatedObjects(float dt)
Central state/object manager and communications hub.
ProceduralManagerPtr m_procedural_manager
std::vector< AnimatedObject > m_animated_objects
TerrainEditorObjectPtrVec & GetEditorObjects()
TerrainEditorObjectPtrVec m_editor_objects
Ogre::AnimationState * anim
TerrainObjectManager(Terrain *terrainManager)
int TerrainEditorObjectID_t
Offset into RoR::TerrainObjectManager::m_editor_objects, use RoR::TERRAINEDITOROBJECTID_INVALID as em...
bool UpdateTerrainObjects(float dt)
void ProcessTree(float yawfrom, float yawto, float scalefrom, float scaleto, char *ColorMap, char *DensityMap, char *treemesh, char *treeCollmesh, float gridspacing, float highdens, int minDist, int maxDist, int mapsizex, int mapsizez)
void ProcessODefCollisionBoxes(TerrainEditorObjectPtr obj, ODefDocument *odef, const TerrainEditorObjectPtr ¶ms, bool race_event)
void moveObjectVisuals(const Ogre::String &instancename, const Ogre::Vector3 &pos)
std::vector< ParticleEffectObject > m_particle_effect_objects
Ogre::SceneNode * m_angelscript_grouping_node
For even more readable scene graph (via inspector script)
Self reference-counting objects, as requred by AngelScript garbage collector.
std::vector< Localizer > LocalizerVec
Ogre::SceneNode * m_tobj_grouping_node
For even more readable scene graph (via inspector script)
std::vector< TObjDocumentPtr > & GetTobjCache()
Ogre::Quaternion rotation
void SpawnSinglePredefinedActor(TerrainEditorObjectPtr const &object)
int m_tobj_cache_active_id
TerrainEditorObjectID_t FindEditorObjectByInstanceName(std::string const &instance_name)
Returns offset to m_editor_objects or -1 if not found.