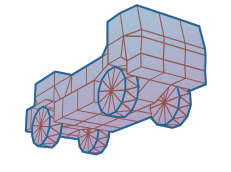 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
24 #ifndef _SkyX_VClouds_DataManager_H_
25 #define _SkyX_VClouds_DataManager_H_
27 #include "Prerequisites.h"
31 namespace SkyX {
namespace VClouds{
78 void create(
const int& nx,
const int& ny,
const int& nz);
83 void update(
const Ogre::Real &timeSinceLastFrame);
130 void setWheater(
const float& Humidity,
const float& AverageCloudsSize,
const bool& delayedResponse =
true);
150 void _initData(
const int& nx,
const int& ny,
const int& nz);
159 Cell ***
_create3DCellArray(
const int& nx,
const int& ny,
const int& nz,
const bool& init =
true);
185 void _performCalculations(
const int& nx,
const int& ny,
const int& nz,
const int& step,
const int& xStart,
const int& xEnd);
207 const float _getDensityAt(Cell ***c,
const int& nx,
const int& ny,
const int& nz,
const int&
x,
const int&
y,
const int&
z,
const int& r,
const float& strength)
const;
215 const float _getDensityAt(Cell ***c,
const int&
x,
const int&
y,
const int&
z)
const;
226 const bool _fact(Cell ***c,
const int& nx,
const int& ny,
const int& nz,
const int&
x,
const int&
y,
const int&
z)
const;
235 void _clearProbabilities(Cell*** c,
const int& nx,
const int& ny,
const int& nz,
const bool& clearData);
244 void _updateProbabilities(Cell*** c,
const int& nx,
const int& ny,
const int& nz,
const bool& delayedResponse);
257 const Ogre::Real
_getLightAbsorcionAt(Cell*** c,
const int& nx,
const int& ny,
const int& nz,
const int&
x,
const int&
y,
const int&
z,
const Ogre::Vector3& d,
const float& att)
const;
void _createVolTexture(const VolTextureId &TexId, const int &nx, const int &ny, const int &nz)
Create volumetric texture.
const bool & isCreated() const
Has been create(...) already called?
void create(const int &nx, const int &ny, const int &nz)
Create.
void _delete3DCellArray(Cell ***c, const int &nx, const int &ny)
Delete tridimensional cell array.
bool mVolTexToUpdate
Current texture.
void _performCalculations(const int &nx, const int &ny, const int &nz, const int &step, const int &xStart, const int &xEnd)
Perform celullar automata simulation.
float dens
Continous density.
void setUpdateTime(const float &UpdateTime)
Set update time.
const Ogre::Real & getUpdateTime() const
Get update time.
const Ogre::Real _getInterpolation() const
Get current interpolation factor.
void _updateVolTextureData(Cell ***c, const VolTextureId &TexId, const int &nx, const int &ny, const int &nz)
Update volumetric texture data.
bool hum
Humidity, phase and cloud.
float mUpdateTime
Update time.
bool mCreated
Has been create(...) already called?
DataManager(VClouds *vc)
Constructor.
int mMaxNumberOfClouds
Max number of clouds(Ellipsoids)
const Ogre::Real _getLightAbsorcionAt(Cell ***c, const int &nx, const int &ny, const int &nz, const int &x, const int &y, const int &z, const Ogre::Vector3 &d, const float &att) const
Get light absorcion factor at a point.
Cell *** mCellsCurrent
Simulation data.
Cell *** _create3DCellArray(const int &nx, const int &ny, const int &nz, const bool &init=true)
Create tridimensional cell array.
VolTextureId
Volumetric textures enumeration.
void forceToUpdateData()
Forces the data manager to calculate the next step right now.
void _updateProbabilities(Cell ***c, const int &nx, const int &ny, const int &nz, const bool &delayedResponse)
Update probabilities based from the Ellipsoid vector.
void _clearProbabilities(Cell ***c, const int &nx, const int &ny, const int &nz, const bool &clearData)
Clear probabilities.
int mStep
Current calculation state.
const float _getDensityAt(Cell ***c, const int &nx, const int &ny, const int &nz, const int &x, const int &y, const int &z, const int &r, const float &strength) const
Get continous density at a point.
void addEllipsoid(Ellipsoid *e, const bool &UpdateProbabilities=true)
Add ellipsoid: clouds are modelled as ellipsoids in our simulation approach, so.
void _initData(const int &nx, const int &ny, const int &nz)
Initialize data.
float light
Light absorcion.
std::vector< Ellipsoid * > mEllipsoids
Ellipsoids.
FastFakeRandom * mFFRandom
Fast fake random.
VClouds * mVClouds
SkyX parent pointer.
const bool _fact(Cell ***c, const int &nx, const int &ny, const int &nz, const int &x, const int &y, const int &z) const
Fact funtion.
void setWheater(const float &Humidity, const float &AverageCloudsSize, const bool &delayedResponse=true)
Set wheater parameters Use this funtion to update the cloud field parameters, you'll get a smart and ...
Ellipsoid class x^2 y^2 z^2 / + / + / = 1 a^2 b^2 c^2.
Ogre::TexturePtr mVolTextures[2]
Volumetric textures array.
~DataManager()
Destructor.
void _copy3DCellArraysData(Cell ***src, Cell ***dest, const int &nx, const int &ny, const int &nz)
Copy 3d cells arrays data.
float mCurrentTransition
Current transition.
void update(const Ogre::Real &timeSinceLastFrame)
Update.