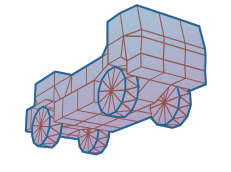 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
25 #ifndef _Hydrax_DecalsManager_H_
26 #define _Hydrax_DecalsManager_H_
50 Decal(
Hydrax *h,
const Ogre::String &TextureName,
const int& Id);
223 Decal*
add(
const Ogre::String& TextureName);
234 void remove(
const int& Id);
void update()
Update decal manager.
DecalsManager(Hydrax *h)
Constructor.
Ogre::Vector3 mLastPosition
Last camera position, orientation, underwater.
Ogre::Real mTransparency
Transparency.
const bool & isVisible() const
Is decal visile?
Ogre::Frustum * getProjector()
Get the decal projector.
Hydrax * mHydrax
Hydrax parent pointer.
Ogre::String mTextureName
Decal texture name.
void _setWaterStrength(const Ogre::Real &WaterStrength)
Set water strength (used for decals culling)
Ogre::SceneNode * getSceneNode()
Get the decal scene node.
Struct wich contains an especific width and height value.
Ogre::Quaternion mLastOrientation
Decal * get(const int &Id)
Get decal.
void _forceToUpdate()
Call to force to update decals.
Ogre::Vector2 mPosition
Position.
const Ogre::Vector2 & getPosition() const
Get decal position.
void setTransparency(const Ogre::Real &Transparency)
Set decal transparency.
~DecalsManager()
Destructor.
const Ogre::Real & getTransparency() const
Get decal transparency.
void setOrientation(const Ogre::Radian &Orientation)
Set decal orientation.
void removeAll()
Remove all decals.
const Ogre::Radian & getOrientation() const
Get decal orientation.
void setVisible(const bool &Visible)
Set decal visibile or not.
const Ogre::String & getTextureName() const
Get decal texture name.
const Ogre::Real _getWaterStrength() const
Get water strength (used for decals culling)
const Ogre::Vector2 & getSize() const
Get decal size.
Ogre::Frustum * mProjector
Decal projector.
Ogre::Pass * getRegisteredPass()
Get the pass the decal is in.
void setSize(const Ogre::Vector2 &Size)
Set decal size.
void unregister()
Unregister from current technique.
bool mVisible
Is decal visible?
std::vector< Decal * > getDecals()
Get decals std::vector.
std::vector< Decal * >::iterator DecalIt
Decal iterator.
const int & getId() const
Get the decal Id.
Ogre::Real mWaterStrength
Water strength (For decals culling)
Hydrax * mHydrax
Hydrax parent pointer.
void registerPass(Ogre::Pass *_Pass)
Register the decal int the specified pass.
void remove(const int &Id)
Remove decal.
Decal * add(const Ogre::String &TextureName)
Add decal.
void registerAll()
Register all decals.
Decal(Hydrax *h, const Ogre::String &TextureName, const int &Id)
Constructor.
Ogre::Radian mOrientation
Orientation.
Ogre::SceneNode * mSceneNode
Decal scene node.
void setPosition(const Ogre::Vector2 &Position)
Set decal position.
Ogre::Pass * mRegisteredPass
Registered pass.
std::vector< Decal * > mDecals
Decals std::vector.