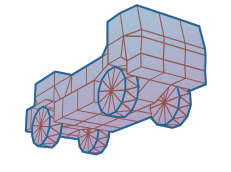 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
31 void DrawRowSlider(
const char* nameStr, std::string codeStr,
const char* descStr,
float min,
float max,
float &var_ref);
32 void DrawRowText(
const char* nameStr, std::string codeStr,
const char* descStr);
33 void DrawRowCheckbox(
const char* nameStr, std::string codeStr,
const char* descStr,
bool &var_ref,
const char* label);
34 void DrawRowInt(
const char* nameStr, std::string codeStr,
const char* descStr,
int &var_ref);
35 void DrawRowIntCheckbox(
const char* nameStr, std::string codeStr,
const char* descStr,
int &var_ref,
bool &on,
const char* label);
36 void DrawRowIntNode(
const char* nameStr, std::string codeStr,
const char* descStr,
int &var_ref,
int &node_x,
int &node_y,
int &node_z);
void DrawRowText(const char *nameStr, std::string codeStr, const char *descStr)
void ExecuteString(std::string const &code)
Runs code using 'as' console command.
void DrawRowCheckbox(const char *nameStr, std::string codeStr, const char *descStr, bool &var_ref, const char *label)
Generic console rendering.
void DrawRowIntNode(const char *nameStr, std::string codeStr, const char *descStr, int &var_ref, int &node_x, int &node_y, int &node_z)
void DrawRowInt(const char *nameStr, std::string codeStr, const char *descStr, int &var_ref)
Central state/object manager and communications hub.
void DrawRowIntCheckbox(const char *nameStr, std::string codeStr, const char *descStr, int &var_ref, bool &on, const char *label)
void DrawRowSlider(const char *nameStr, std::string codeStr, const char *descStr, float min, float max, float &var_ref)