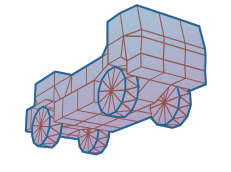 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
31 #include <Overlay/OgreFontManager.h>
33 #include <OgreUTFString.h>
39 class MovableText :
public Ogre::MovableObject,
public Ogre::Renderable
78 MovableText(
const Ogre::UTFString &name,
const Ogre::UTFString &caption,
79 const Ogre::UTFString &fontName =
"highcontrast_black",
80 Ogre::Real charHeight = 1.0,
const Ogre::ColourValue &color = Ogre::ColourValue::Black);
84 virtual void visitRenderables(Ogre::Renderable::Visitor* visitor,
bool debugRenderables =
false) {};
88 void setCaption(
const Ogre::UTFString &caption);
89 void setColor(
const Ogre::ColourValue &color);
122 const Ogre::String &
getMovableType(
void)
const {
static Ogre::String movType =
"MovableText";
return movType;};
void setTextAlignment(const HorizontalAlignment &horizontalAlignment, const VerticalAlignment &verticalAlignment)
#define ROR_ASSERT(_EXPR)
void setColor(const Ogre::ColourValue &color)
Ogre::MaterialPtr mpBackgroundMaterial
void setCaption(const Ogre::UTFString &caption)
virtual void visitRenderables(Ogre::Renderable::Visitor *visitor, bool debugRenderables=false)
Ogre::AxisAlignedBox GetAABB(void)
const Ogre::AxisAlignedBox & getBoundingBox(void) const
Ogre::uint getSpaceWidth() const
void showOnTop(bool show=true)
void setSpaceWidth(Ogre::Real width)
VerticalAlignment mVerticalAlignment
Ogre::Real getAdditionalHeight() const
void setFontName(const Ogre::UTFString &fontName)
const Ogre::Vector3 & getWorldPosition(void) const
const Ogre::LightList & getLights(void) const
Ogre::UTFString mFontName
Ogre::RenderWindow * mpWin
Ogre::Real getSquaredViewDepth(const Ogre::Camera *cam) const
Ogre::uint getCharacterHeight() const
MovableText(const Ogre::UTFString &name, const Ogre::UTFString &caption, const Ogre::UTFString &fontName="highcontrast_black", Ogre::Real charHeight=1.0, const Ogre::ColourValue &color=Ogre::ColourValue::Black)
void getRenderOperation(Ogre::RenderOperation &op)
const Ogre::Quaternion & getWorldOrientation(void) const
Ogre::AxisAlignedBox mAABB
Ogre::MaterialPtr mpMaterial
void getWorldTransforms(Ogre::Matrix4 *xform) const
Central state/object manager and communications hub.
const Ogre::MaterialPtr & getMaterial(void) const
Ogre::RenderOperation mRenderOp
const Ogre::ColourValue & getColor() const
const Ogre::UTFString & getFontName() const
void setCharacterHeight(Ogre::Real height)
const Ogre::String & getMovableType(void) const
void _updateRenderQueue(Ogre::RenderQueue *queue)
HorizontalAlignment mHorizontalAlignment
void _notifyCurrentCamera(Ogre::Camera *cam)
void setAdditionalHeight(Ogre::Real height)
Ogre::Real getBoundingRadius(void) const
const Ogre::String & getName(void) const
Ogre::Real mAdditionalHeight
Ogre::Real mTimeUntilNextToggle
bool getShowOnTop() const
const Ogre::UTFString & getCaption() const