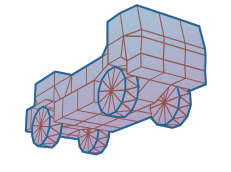 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
24 #ifndef _SkyX_VClouds_Lightning_H_
25 #define _SkyX_VClouds_Lightning_H_
27 #include "Prerequisites.h"
29 namespace SkyX {
namespace VClouds{
51 Segment(
const Ogre::Vector3& a_,
const Ogre::Vector3& b_)
75 Lightning(Ogre::SceneManager* sm, Ogre::SceneNode* sn,
const Ogre::Vector3& orig,
const Ogre::Vector3& dir,
const Ogre::Real& l,
76 const Ogre::uint32& d,
const Ogre::uint32& rec,
const Ogre::Real& tm,
const Ogre::Real& wm,
const Ogre::Vector2& b = Ogre::Vector2(0,1));
93 void update(Ogre::Real timeSinceLastFrame);
155 void _updateData(
const Ogre::Real& alpha,
const Ogre::Real& currentPos,
const Ogre::Real& parentTime);
Ogre::Vector3 a
Segment start.
Ogre::BillboardSet * getBillboardSet() const
Get billboard set.
void update(Ogre::Real timeSinceLastFrame)
Update.
const Ogre::Real & getIntensity() const
Get lightning intensity.
Ogre::SceneManager * mSceneManager
Scene manager.
Ogre::Real mIntensity
Lightning intensity.
Ogre::Real mTimeMultiplier
Global time multiplier.
const Ogre::Real & getLength() const
Get ray length.
const bool & isFinished() const
Has the ray finished?
Ogre::Real mRealLength
Real ray length (total segments length amount)
Ogre::SceneNode * mSceneNode
Scene node.
Ogre::Vector3 mTimeMultipliers
Per step time multipliers.
void _updateData(const Ogre::Real &alpha, const Ogre::Real ¤tPos, const Ogre::Real &parentTime)
Update data.
Ogre::Real mWidthMultiplier
Width multiplier.
bool mCreated
Has been create() already called?
bool mFinished
Has the ray finished?
std::vector< Segment > mSegments
Segments.
std::vector< Lightning * > mChildren
Children lightnings.
Ogre::uint32 mRecursivity
Recursivity level.
void _updateRenderQueueGroup(const Ogre::uint8 &rqg)
Update render queue group.
Ogre::Real mLength
Ray length.
Ogre::uint32 mDivisions
Number of divisions.
Ogre::Vector2 mBounds
Ray bounds (for internal visual calculations)
Ogre::Vector3 mDirection
Ray direction.
Lightning(Ogre::SceneManager *sm, Ogre::SceneNode *sn, const Ogre::Vector3 &orig, const Ogre::Vector3 &dir, const Ogre::Real &l, const Ogre::uint32 &d, const Ogre::uint32 &rec, const Ogre::Real &tm, const Ogre::Real &wm, const Ogre::Vector2 &b=Ogre::Vector2(0, 1))
Constructor.
Ogre::SceneNode * getSceneNode() const
Get scene node.
const Ogre::Vector3 & getDirection() const
Get ray direction.
Ogre::Vector2 mAngleRange
Angle range (Little values -> Less derivations, bigger values -> More derivations)
Ogre::Vector3 mOrigin
Ray origin.
Ogre::Real mTime
Current elapsed time.
Ogre::BillboardSet * mBillboardSet
Billboard set.
Segment()
Default constructor.
Segment(const Ogre::Vector3 &a_, const Ogre::Vector3 &b_)
Constructor.
Ogre::Vector3 b
Segment end.