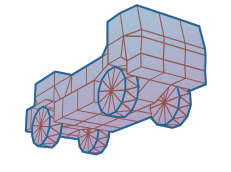 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
32 void AddInline(ImU32 color, ImVec2 text_size,
const char* text,
const char* text_end);
34 void AddWrapped(ImU32 color,
float wrap_width,
const char* text,
const char* text_end);
36 void AddMultiline(ImU32 color,
float wrap_width,
const char* text,
const char* text_end);
46 void LoadingIndicatorCircle(
const char* label,
const float indicator_radius,
const ImVec4& main_color,
const ImVec4& backdrop_color,
const int circle_count,
const float speed);
49 void DrawImageRotated(ImTextureID tex_id, ImVec2 center, ImVec2 size,
float angle);
52 ImVec2
DrawColorMarkedText(ImDrawList* drawlist, ImVec2 text_cursor, ImVec4 default_color,
float override_alpha,
float wrap_width, std::string
const& line);
73 void DrawGCombo(
CVar* cvar,
const char* label,
const char* values);
75 Ogre::TexturePtr
FetchIcon(
const char* name);
92 bool ImButtonHoldToConfirm(
const std::string& btn_idstr,
const bool smallbutton,
const float time_limit);
void ImTextWrappedColorMarked(std::string const &text)
Prints multiline text with '#rrggbb' color markers. Behaves like ImGui::Text* functions.
ImVec2 ImCalcEventHighlightedSize(events input_event)
void ImAddItemToComboboxString(std::string &target, std::string const &item)
std::string StripColorMarksFromText(std::string const &text)
void DrawGFloatSlider(CVar *cvar, const char *label, float v_min, float v_max)
Ogre::TexturePtr FetchIcon(const char *name)
ImVec2 cursor
Next draw position, screen space.
void AddMultiline(ImU32 color, float wrap_width, const char *text, const char *text_end)
Wraps substrings separated by blanks. wrap_width=-1.f disables wrapping.
void DrawGCombo(CVar *cvar, const char *label, const char *values)
void DrawGTextEdit(CVar *cvar, const char *label, Str< 1000 > &buf)
void DrawGIntBox(CVar *cvar, const char *label)
bool GetScreenPosFromWorldPos(Ogre::Vector3 const &world_pos, ImVec2 &out_screen)
ImVec2 size
Accumulated text size.
ImVec2 DrawColorMarkedText(ImDrawList *drawlist, ImVec2 text_cursor, ImVec4 default_color, float override_alpha, float wrap_width, std::string const &line)
Draw multiline text with '#rrggbb' color markers. Returns total text size.
void ImDrawModifierKeyHighlighted(OIS::KeyCode key)
void AddInline(ImU32 color, ImVec2 text_size, const char *text, const char *text_end)
No wrapping or trimming.
void DrawGFloatBox(CVar *cvar, const char *label)
void DrawGIntSlider(CVar *cvar, const char *label, int v_min, int v_max)
Helper for drawing multiline wrapped & colored text.
ImDrawList * GetImDummyFullscreenWindow(const std::string &name="RoR_TransparentFullscreenWindow")
bool ImButtonHoldToConfirm(const std::string &btn_idstr, const bool smallbutton, const float time_limit)
Central state/object manager and communications hub.
bool ImDrawEventHighlightedButton(events input_event, bool *btn_hovered=nullptr, bool *btn_active=nullptr)
void DrawGIntCheck(CVar *cvar, const char *label)
Quake-style console variable, defined in RoR.cfg or crated via Console UI and scripts.
void AddWrapped(ImU32 color, float wrap_width, const char *text, const char *text_end)
Wraps entire input. Trims leading blanks on extra lines. wrap_width=-1.f disables wrapping.
ImTextFeeder(ImDrawList *_drawlist, ImVec2 _origin)
ImVec2 origin
First draw position, screen space.
void ImTerminateComboboxString(std::string &target)
bool DrawGCheckbox(CVar *cvar, const char *label)
void ImDrawEventHighlighted(events input_event)
void LoadingIndicatorCircle(const char *label, const float indicator_radius, const ImVec4 &main_color, const ImVec4 &backdrop_color, const int circle_count, const float speed)
Draws animated loading spinner.
void DrawImageRotated(ImTextureID tex_id, ImVec2 center, ImVec2 size, float angle)
Add rotated textured quad to ImDrawList, source: https://github.com/ocornut/imgui/issues/1982#issueco...