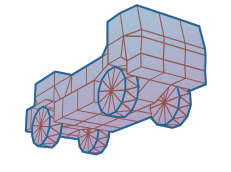 |
RigsofRods
2023.09
Soft-body Physics Simulation
|
Go to the documentation of this file.
24 #ifndef _SkyX_VClouds_GeometryBlock_H_
25 #define _SkyX_VClouds_GeometryBlock_H_
27 #include "Prerequisites.h"
29 namespace SkyX {
namespace VClouds{
64 const float& Height,
const Ogre::Radian& Alpha,
const Ogre::Radian& Beta,
65 const float& Radius,
const Ogre::Radian& Phi,
const int& Na,
66 const int& Nb,
const int& Nc,
const int& A,
67 const int& B,
const int& C,
const int& Position);
86 void updateGeometry(Ogre::Camera* c,
const Ogre::Vector3& displacement,
const Ogre::Vector3& distance);
154 const Ogre::AxisAlignedBox
_buildAABox(
const float& fd)
const;
188 void _setVertexData(
const int& index,
const Ogre::Vector3& p,
const float& o);
int mPosition
Number of block(Position)
Ogre::Radian mAlpha
Angles.
Ogre::Vector2 mV2Cos
Precomputed Cos/Sin vectors.
Ogre::Vector3 mDisplacement
Displacement.
Ogre::Vector2 mWorldOffset
World coords offset.
const bool isInFrustum(Ogre::Camera *c) const
Is the geometry block inside the camera frustum?
Ogre::Vector3 mDistance
Current distance.
Ogre::HardwareVertexBufferSharedPtr mVertexBuffer
Vertex buffer.
Ogre::SubMesh * getSubMesh()
Get sub mesh.
void _updateZoneCSlice(const int &n)
Update zone C slice.
const Ogre::AxisAlignedBox _buildAABox(const float &fd) const
Build axis aligned box.
const bool & isCreated() const
Has been create() already called?
int mNumberOfTriangles
Current number of triangles.
Ogre::Radian mPhi
Acimutal angle.
float mA
A, B and C radius.
VClouds * mVClouds
VClouds pointer.
float mLastFallingDistance
Last falling distance.
Ogre::MeshPtr getMesh()
Get mesh.
void updateGeometry(Ogre::Camera *c, const Ogre::Vector3 &displacement, const Ogre::Vector3 &distance)
Update geometry.
Ogre::HardwareIndexBufferSharedPtr mIndexBuffer
Index buffer.
GeometryBlock(VClouds *vc, const float &Height, const Ogre::Radian &Alpha, const Ogre::Radian &Beta, const float &Radius, const Ogre::Radian &Phi, const int &Na, const int &Nb, const int &Nc, const int &A, const int &B, const int &C, const int &Position)
Constructor.
Ogre::HardwareIndexBufferSharedPtr & getHardwareIndexBuffer()
Get hardware index buffer reference.
void _updateGeometry()
Update geometry.
Ogre::Entity * getEntity()
Get entity.
Ogre::SubMesh * mSubMesh
Ogre::Submesh pointer.
float mBetaSin
PI - Beta, PI - Alpha Sin.
void setWorldOffset(const Ogre::Vector2 &WorldOffset)
Set world offset.
void _setVertexData(const int &index, const Ogre::Vector3 &p, const float &o)
Set vertex data.
bool mCreated
Has been create() already called?
void _createGeometry()
Create geometry.
~GeometryBlock()
Destructor.
Ogre::MeshPtr mMesh
Ogre::MeshPtr.
Ogre::Camera * mCamera
Current rendering camera.
int mNa
Number of slices per geometry zone.
void _updateZoneBSlice(const int &n)
Update zone B slice.
void _updateZoneASlice(const int &n)
Update zone A slice.
void _calculateDataSize()
Calculate data size.
VERTEX * mVertices
Vertices pointer.
int mVertexCount
Vertex count.
Ogre::HardwareVertexBufferSharedPtr & getHardwareVertexBuffer()
Get hardware vertex buffer reference.
Ogre::Entity * mEntity
Ogre::Entity pointer.