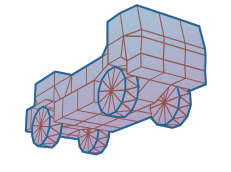 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
25 #ifndef _Hydrax_Noise_FFT_H_
26 #define _Hydrax_Noise_FFT_H_
40 namespace Hydrax{
namespace Noise
105 const float& _PhysicalResolution,
107 const Ogre::Vector2& _WindDirection,
108 const float& _AnimationSpeed,
109 const float& _KwPower,
110 const float& _Amplitude)
135 const float& _PhysicalResolution,
137 const Ogre::Vector2& _WindDirection,
138 const float& _AnimationSpeed,
139 const float& _KwPower,
140 const float& _Amplitude,
141 const float &_GPU_Strength,
142 const Ogre::Vector3 &_GPU_LODParameters)
163 FFT(
const Options &Options);
186 void update(
const Ogre::Real &timeSinceLastFrame);
191 void saveCfg(Ogre::String &Data);
196 bool loadCfg(Ogre::ConfigFile &CfgFile);
203 float getValue(
const float &
x,
const float &
y);
243 const float _getPhillipsSpectrum(
const Ogre::Vector2& waveVector,
const Ogre::Vector2& wind,
const float& kwPower_ = 2.0f)
const;
std::complex< float > * currentWaves
the data of the simulation at time t, which is formed using the data at time 0 and the angular freque...
void _executeInverseFFT()
Execute inverse fast fourier transform.
void _initNoise()
Initialize noise.
const float _getGaussianRandomFloat() const
Get a Gaussian random number with mean 0 and standard deviation 1, using Box - muller transform.
float AnimationSpeed
Animation speed.
Ogre::Vector2 WindDirection
Wind direction.
float Amplitude
Noise amplitude.
const float _getPhillipsSpectrum(const Ogre::Vector2 &waveVector, const Ogre::Vector2 &wind, const float &kwPower_=2.0f) const
Get the Philipps Spectrum, used to create the amplitudes and phases.
const Options & getOptions() const
Get current FFT noise options.
bool createGPUNormalMapResources(GPUNormalMapManager *g)
Create GPUNormalMap resources.
float maximalValue
The minimal value of the result data of the fft transformation.
void _updateGPUNormalMapResources()
Update gpu normal map resources.
Class to manager GPU normal maps.
GPUNormalMapManager * mGPUNormalMapManager
GPUNormalMapManager pointer.
void saveCfg(Ogre::String &Data)
Save config.
void update(const Ogre::Real &timeSinceLastFrame)
Call it each frame.
Ogre::Vector3 GPU_LODParameters
LOD Parameters, in order to obtain a smooth normal map we need to decrease the detail level when the ...
int resolution
FFT resolution.
void _calculeNoise(const float &delta)
Calcule noise.
float GPU_Strength
GPU Normal map generator parameters Only if GPU normal map generation is active.
void setOptions(const Options &Options)
Set/Update fft noise options.
Options mOptions
Perlin noise options.
FFT()
Default constructor.
Base noise class, Override it for create different ways of create water noise.
Options()
Default constructor.
float getValue(const float &x, const float &y)
Get the especified x/y noise value.
float * angularFrequencies
the angular frequencies
float PhysicalResolution
Physical resolution.
Options(const int &_Resolution, const float &_PhysicalResolution, const float &_Scale, const Ogre::Vector2 &_WindDirection, const float &_AnimationSpeed, const float &_KwPower, const float &_Amplitude, const float &_GPU_Strength, const Ogre::Vector3 &_GPU_LODParameters)
User constructor.
bool loadCfg(Ogre::ConfigFile &CfgFile)
Load config.
std::complex< float > * initialWaves
the data which is referred as h0{x,t), that is, the data of the simulation at the time 0.
Options(const int &_Resolution, const float &_PhysicalResolution, const float &_Scale, const Ogre::Vector2 &_WindDirection, const float &_AnimationSpeed, const float &_KwPower, const float &_Amplitude)
User constructor.
float * re
Pointers to resolution*resolution float size arrays.
int Resolution
Noise resolution (2^n)
void _normalizeFFTData(const float &scale)
Normalize fft data.
Struct wich contains fft noise module options.